Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / WinForms / Managed / System / WinForms / ListBindingConverter.cs / 1 / ListBindingConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Diagnostics; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Collections; using System.Globalization; using System.Reflection; ////// /// public class ListBindingConverter : TypeConverter { private static Type[] ctorTypes = null; // the list of type of our ctor parameters. private static string[] ctorParamProps = null; // the name of each property to check to see if we need to init with a ctor. ///[To be supplied.] ////// Creates our array of types on demand. /// private static Type[] ConstructorParamaterTypes { get { if (ctorTypes == null) { ctorTypes = new Type[]{typeof(string), typeof(object), typeof(string), typeof(bool), typeof(DataSourceUpdateMode), typeof(object), typeof(string), typeof(IFormatProvider)}; } return ctorTypes; } } ////// Creates our array of param names on demand. /// private static string[] ConstructorParameterProperties { get { if (ctorParamProps == null) { ctorParamProps = new string[]{null, null, null, "FormattingEnabled", "DataSourceUpdateMode", "NullValue", "FormatString", "FormatInfo", }; } return ctorParamProps; } } ////// /// public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(InstanceDescriptor)) { return true; } return base.CanConvertTo(context, destinationType); } ///Gets a value indicating whether this converter can /// convert an object to the given destination type using the context. ////// /// Converts the given object to another type. The most common types to convert /// are to and from a string object. The default implementation will make a call /// to ToString on the object if the object is valid and if the destination /// type is string. If this cannot convert to the desitnation type, this will /// throw a NotSupportedException. /// public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } if (destinationType == typeof(InstanceDescriptor) && value is Binding) { Binding b = (Binding)value; return GetInstanceDescriptorFromValues(b); } return base.ConvertTo(context, culture, value, destinationType); } ////// /// Creates an instance of this type given a set of property values /// for the object. This is useful for objects that are immutable, but still /// want to provide changable properties. /// public override object CreateInstance(ITypeDescriptorContext context, IDictionary propertyValues) { try { return new Binding((string)propertyValues["PropertyName"], propertyValues["DataSource"], (string)propertyValues["DataMember"]); } catch (InvalidCastException invalidCast) { throw new ArgumentException(SR.GetString(SR.PropertyValueInvalidEntry), invalidCast); } catch (NullReferenceException nullRef) { throw new ArgumentException(SR.GetString(SR.PropertyValueInvalidEntry), nullRef); } } ////// /// Determines if changing a value on this object should require a call to /// CreateInstance to create a new value. /// public override bool GetCreateInstanceSupported(ITypeDescriptorContext context) { return true; } ////// Gets the best matching ctor for a given binding and fills it out, based on the /// state of the Binding and the optimal ctor. /// private InstanceDescriptor GetInstanceDescriptorFromValues(Binding b) { // The BindingFormattingDialog turns on Binding::FormattingEnabled property. // however, when the user data binds a property using the PropertyBrowser, Binding::FormattingEnabled is set to false // The Binding class is not a component class, so we don't have the ComponentInitialize method where we can set FormattingEnabled to true // so we set it here. VsWhidbey 241361 b.FormattingEnabled = true; bool isComplete = true; int lastItem = ConstructorParameterProperties.Length - 1; for (; lastItem >= 0; lastItem--) { // null means no prop is available, we quit here. // if (ConstructorParameterProperties[lastItem] == null) { break; } // get the property and see if it needs to be serialized. // PropertyDescriptor prop = TypeDescriptor.GetProperties(b)[ConstructorParameterProperties[lastItem]]; if (prop != null && prop.ShouldSerializeValue(b)) { break; } } // now copy the type array up to the point we quit. // Type[] ctorParams = new Type[lastItem + 1]; Array.Copy(ConstructorParamaterTypes, 0, ctorParams, 0, ctorParams.Length); // Get the ctor info. // ConstructorInfo ctor = typeof(Binding).GetConstructor(ctorParams); Debug.Assert(ctor != null, "Failed to find Binding ctor for types!"); if (ctor == null) { isComplete = false; ctor = typeof(Binding).GetConstructor(new Type[] { typeof(string), typeof(object), typeof(string)}); } // now fill in the values. // object[] values = new object[ctorParams.Length]; for (int i = 0; i < values.Length; i++) { object val = null; switch (i) { case 0: val = b.PropertyName; break; case 1: val = b.BindToObject.DataSource; break; case 2: val = b.BindToObject.BindingMemberInfo.BindingMember; break; default: val = TypeDescriptor.GetProperties(b)[ConstructorParameterProperties[i]].GetValue(b); break; } values[i] = val; } return new InstanceDescriptor(ctor, values, isComplete); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Diagnostics; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Collections; using System.Globalization; using System.Reflection; ////// /// public class ListBindingConverter : TypeConverter { private static Type[] ctorTypes = null; // the list of type of our ctor parameters. private static string[] ctorParamProps = null; // the name of each property to check to see if we need to init with a ctor. ///[To be supplied.] ////// Creates our array of types on demand. /// private static Type[] ConstructorParamaterTypes { get { if (ctorTypes == null) { ctorTypes = new Type[]{typeof(string), typeof(object), typeof(string), typeof(bool), typeof(DataSourceUpdateMode), typeof(object), typeof(string), typeof(IFormatProvider)}; } return ctorTypes; } } ////// Creates our array of param names on demand. /// private static string[] ConstructorParameterProperties { get { if (ctorParamProps == null) { ctorParamProps = new string[]{null, null, null, "FormattingEnabled", "DataSourceUpdateMode", "NullValue", "FormatString", "FormatInfo", }; } return ctorParamProps; } } ////// /// public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(InstanceDescriptor)) { return true; } return base.CanConvertTo(context, destinationType); } ///Gets a value indicating whether this converter can /// convert an object to the given destination type using the context. ////// /// Converts the given object to another type. The most common types to convert /// are to and from a string object. The default implementation will make a call /// to ToString on the object if the object is valid and if the destination /// type is string. If this cannot convert to the desitnation type, this will /// throw a NotSupportedException. /// public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } if (destinationType == typeof(InstanceDescriptor) && value is Binding) { Binding b = (Binding)value; return GetInstanceDescriptorFromValues(b); } return base.ConvertTo(context, culture, value, destinationType); } ////// /// Creates an instance of this type given a set of property values /// for the object. This is useful for objects that are immutable, but still /// want to provide changable properties. /// public override object CreateInstance(ITypeDescriptorContext context, IDictionary propertyValues) { try { return new Binding((string)propertyValues["PropertyName"], propertyValues["DataSource"], (string)propertyValues["DataMember"]); } catch (InvalidCastException invalidCast) { throw new ArgumentException(SR.GetString(SR.PropertyValueInvalidEntry), invalidCast); } catch (NullReferenceException nullRef) { throw new ArgumentException(SR.GetString(SR.PropertyValueInvalidEntry), nullRef); } } ////// /// Determines if changing a value on this object should require a call to /// CreateInstance to create a new value. /// public override bool GetCreateInstanceSupported(ITypeDescriptorContext context) { return true; } ////// Gets the best matching ctor for a given binding and fills it out, based on the /// state of the Binding and the optimal ctor. /// private InstanceDescriptor GetInstanceDescriptorFromValues(Binding b) { // The BindingFormattingDialog turns on Binding::FormattingEnabled property. // however, when the user data binds a property using the PropertyBrowser, Binding::FormattingEnabled is set to false // The Binding class is not a component class, so we don't have the ComponentInitialize method where we can set FormattingEnabled to true // so we set it here. VsWhidbey 241361 b.FormattingEnabled = true; bool isComplete = true; int lastItem = ConstructorParameterProperties.Length - 1; for (; lastItem >= 0; lastItem--) { // null means no prop is available, we quit here. // if (ConstructorParameterProperties[lastItem] == null) { break; } // get the property and see if it needs to be serialized. // PropertyDescriptor prop = TypeDescriptor.GetProperties(b)[ConstructorParameterProperties[lastItem]]; if (prop != null && prop.ShouldSerializeValue(b)) { break; } } // now copy the type array up to the point we quit. // Type[] ctorParams = new Type[lastItem + 1]; Array.Copy(ConstructorParamaterTypes, 0, ctorParams, 0, ctorParams.Length); // Get the ctor info. // ConstructorInfo ctor = typeof(Binding).GetConstructor(ctorParams); Debug.Assert(ctor != null, "Failed to find Binding ctor for types!"); if (ctor == null) { isComplete = false; ctor = typeof(Binding).GetConstructor(new Type[] { typeof(string), typeof(object), typeof(string)}); } // now fill in the values. // object[] values = new object[ctorParams.Length]; for (int i = 0; i < values.Length; i++) { object val = null; switch (i) { case 0: val = b.PropertyName; break; case 1: val = b.BindToObject.DataSource; break; case 2: val = b.BindToObject.BindingMemberInfo.BindingMember; break; default: val = TypeDescriptor.GetProperties(b)[ConstructorParameterProperties[i]].GetValue(b); break; } values[i] = val; } return new InstanceDescriptor(ctor, values, isComplete); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
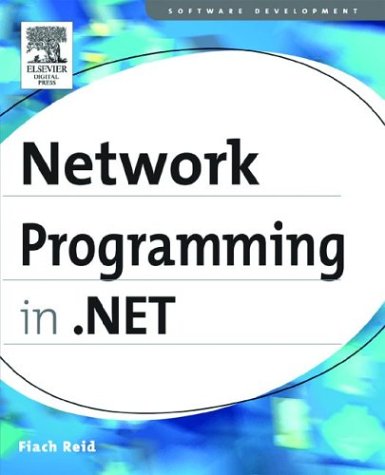
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CrossSiteScriptingValidation.cs
- OleDbSchemaGuid.cs
- printdlgexmarshaler.cs
- DocumentXmlWriter.cs
- CounterSampleCalculator.cs
- _Events.cs
- GridViewCellAutomationPeer.cs
- TraceContext.cs
- PromptBuilder.cs
- Shape.cs
- LayoutUtils.cs
- GridToolTip.cs
- ValidationSummary.cs
- DPCustomTypeDescriptor.cs
- _AuthenticationState.cs
- Simplifier.cs
- EntityContainerRelationshipSet.cs
- SchemaElement.cs
- IgnoreDeviceFilterElement.cs
- DelayedRegex.cs
- PerfService.cs
- Camera.cs
- XmlDesigner.cs
- BaseTemplateBuildProvider.cs
- CompiledXpathExpr.cs
- ObjRef.cs
- GlyphRunDrawing.cs
- EncoderReplacementFallback.cs
- COM2PictureConverter.cs
- LayoutSettings.cs
- Exceptions.cs
- indexingfiltermarshaler.cs
- PasswordDeriveBytes.cs
- MobileListItemCollection.cs
- DataBinding.cs
- Base64Decoder.cs
- TransformerInfoCollection.cs
- Matrix3DConverter.cs
- DataReceivedEventArgs.cs
- SafeNativeMethods.cs
- PagesSection.cs
- PassportAuthenticationEventArgs.cs
- SqlGatherConsumedAliases.cs
- SubtreeProcessor.cs
- Avt.cs
- CompositeClientFormatter.cs
- UnknownWrapper.cs
- ReplyAdapterChannelListener.cs
- BinaryObjectInfo.cs
- SqlServer2KCompatibilityCheck.cs
- WebPartVerbCollection.cs
- HttpStaticObjectsCollectionBase.cs
- Camera.cs
- DocumentViewerConstants.cs
- SByteConverter.cs
- Lasso.cs
- StylusOverProperty.cs
- ExtentJoinTreeNode.cs
- ThemeableAttribute.cs
- LogEntryUtils.cs
- ClickablePoint.cs
- QueryOutputWriter.cs
- HtmlSelect.cs
- RegularExpressionValidator.cs
- MissingFieldException.cs
- IgnorePropertiesAttribute.cs
- LinqDataView.cs
- ProcessRequestArgs.cs
- _SSPIWrapper.cs
- x509store.cs
- HostExecutionContextManager.cs
- DesignTimeParseData.cs
- CompiledQuery.cs
- DelegatingConfigHost.cs
- RoutedEventArgs.cs
- CodePageUtils.cs
- AndMessageFilter.cs
- SuppressMessageAttribute.cs
- StylusOverProperty.cs
- DynamicRendererThreadManager.cs
- SubMenuStyle.cs
- WindowsScrollBarBits.cs
- Ops.cs
- NullableFloatAverageAggregationOperator.cs
- XmlSchema.cs
- ClientSponsor.cs
- FillRuleValidation.cs
- SafeArrayTypeMismatchException.cs
- SplineKeyFrames.cs
- NativeMethods.cs
- RIPEMD160Managed.cs
- QueryStringParameter.cs
- QilFactory.cs
- ReverseComparer.cs
- KoreanLunisolarCalendar.cs
- AnnotationHelper.cs
- EntityAdapter.cs
- UpdateRecord.cs
- FormViewDeletedEventArgs.cs
- NumericUpDown.cs