Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / CompMod / System / CodeDOM / Compiler / CompilerParameters.cs / 1 / CompilerParameters.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.CodeDom.Compiler { using System; using System.CodeDom; using System.Collections; using System.Collections.Specialized; using Microsoft.Win32; using Microsoft.Win32.SafeHandles; using System.Runtime.InteropServices; using System.Security.Permissions; using System.Security.Policy; using System.Runtime.Serialization; ////// [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] [PermissionSet(SecurityAction.InheritanceDemand, Name="FullTrust")] [Serializable] public class CompilerParameters { private StringCollection assemblyNames = new StringCollection(); [OptionalField] private StringCollection embeddedResources = new StringCollection(); [OptionalField] private StringCollection linkedResources = new StringCollection(); private string outputName; private string mainClass; private bool generateInMemory = false; private bool includeDebugInformation = false; private int warningLevel = -1; // -1 means not set (use compiler default) private string compilerOptions; private string win32Resource; private bool treatWarningsAsErrors = false; private bool generateExecutable = false; private TempFileCollection tempFiles; [NonSerializedAttribute] private SafeUserTokenHandle userToken; private Evidence evidence = null; ////// Represents the parameters used in to invoke the compiler. /// ////// public CompilerParameters() : this(null, null) { } ////// Initializes a new instance of ///. /// /// public CompilerParameters(string[] assemblyNames) : this(assemblyNames, null, false) { } ////// Initializes a new instance of ///using the specified /// assembly names. /// /// public CompilerParameters(string[] assemblyNames, string outputName) : this(assemblyNames, outputName, false) { } ////// Initializes a new instance of ///using the specified /// assembly names and output name. /// /// public CompilerParameters(string[] assemblyNames, string outputName, bool includeDebugInformation) { if (assemblyNames != null) { ReferencedAssemblies.AddRange(assemblyNames); } this.outputName = outputName; this.includeDebugInformation = includeDebugInformation; } ////// Initializes a new instance of ///using the specified /// assembly names, output name and a whether to include debug information flag. /// /// public bool GenerateExecutable { get { return generateExecutable; } set { generateExecutable = value; } } ////// Gets or sets whether to generate an executable. /// ////// public bool GenerateInMemory { get { return generateInMemory; } set { generateInMemory = value; } } ////// Gets or sets whether to generate in memory. /// ////// public StringCollection ReferencedAssemblies { get { return assemblyNames; } } ////// Gets or sets the assemblies referenced by the source to compile. /// ////// public string MainClass { get { return mainClass; } set { mainClass = value; } } ////// Gets or sets the main class. /// ////// public string OutputAssembly { get { return outputName; } set { outputName = value; } } ////// Gets or sets the output assembly. /// ////// public TempFileCollection TempFiles { get { if (tempFiles == null) tempFiles = new TempFileCollection(); return tempFiles; } set { tempFiles = value; } } ////// Gets or sets the temp files. /// ////// public bool IncludeDebugInformation { get { return includeDebugInformation; } set { includeDebugInformation = value; } } ////// Gets or sets whether to include debug information in the compiled /// executable. /// ////// public bool TreatWarningsAsErrors { get { return treatWarningsAsErrors; } set { treatWarningsAsErrors = value; } } ///[To be supplied.] ////// public int WarningLevel { get { return warningLevel; } set { warningLevel = value; } } ///[To be supplied.] ////// public string CompilerOptions { get { return compilerOptions; } set { compilerOptions = value; } } ///[To be supplied.] ////// public string Win32Resource { get { return win32Resource; } set { win32Resource = value; } } ///[To be supplied.] ////// [System.Runtime.InteropServices.ComVisible(false)] public StringCollection EmbeddedResources { get { return embeddedResources; } } ////// Gets or sets the resources to be compiled into the target /// ////// [System.Runtime.InteropServices.ComVisible(false)] public StringCollection LinkedResources { get { return linkedResources; } } ////// Gets or sets the linked resources /// ////// public IntPtr UserToken { get { if (userToken != null) return userToken.DangerousGetHandle(); else return IntPtr.Zero; } set { if (userToken != null) userToken.Close(); userToken = new SafeUserTokenHandle(value, false); } } internal SafeUserTokenHandle SafeUserToken { get { return userToken; } } ////// Gets or sets user token to be employed when creating the compiler process. /// ////// public Evidence Evidence { get { Evidence e = null; if (evidence != null) e = CompilerResults.CloneEvidence(evidence); return e; } [SecurityPermissionAttribute( SecurityAction.Demand, ControlEvidence = true )] set { if (value != null) evidence = CompilerResults.CloneEvidence(value); else evidence = null; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Set the evidence for partially trusted scenarios. /// ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.CodeDom.Compiler { using System; using System.CodeDom; using System.Collections; using System.Collections.Specialized; using Microsoft.Win32; using Microsoft.Win32.SafeHandles; using System.Runtime.InteropServices; using System.Security.Permissions; using System.Security.Policy; using System.Runtime.Serialization; ////// [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] [PermissionSet(SecurityAction.InheritanceDemand, Name="FullTrust")] [Serializable] public class CompilerParameters { private StringCollection assemblyNames = new StringCollection(); [OptionalField] private StringCollection embeddedResources = new StringCollection(); [OptionalField] private StringCollection linkedResources = new StringCollection(); private string outputName; private string mainClass; private bool generateInMemory = false; private bool includeDebugInformation = false; private int warningLevel = -1; // -1 means not set (use compiler default) private string compilerOptions; private string win32Resource; private bool treatWarningsAsErrors = false; private bool generateExecutable = false; private TempFileCollection tempFiles; [NonSerializedAttribute] private SafeUserTokenHandle userToken; private Evidence evidence = null; ////// Represents the parameters used in to invoke the compiler. /// ////// public CompilerParameters() : this(null, null) { } ////// Initializes a new instance of ///. /// /// public CompilerParameters(string[] assemblyNames) : this(assemblyNames, null, false) { } ////// Initializes a new instance of ///using the specified /// assembly names. /// /// public CompilerParameters(string[] assemblyNames, string outputName) : this(assemblyNames, outputName, false) { } ////// Initializes a new instance of ///using the specified /// assembly names and output name. /// /// public CompilerParameters(string[] assemblyNames, string outputName, bool includeDebugInformation) { if (assemblyNames != null) { ReferencedAssemblies.AddRange(assemblyNames); } this.outputName = outputName; this.includeDebugInformation = includeDebugInformation; } ////// Initializes a new instance of ///using the specified /// assembly names, output name and a whether to include debug information flag. /// /// public bool GenerateExecutable { get { return generateExecutable; } set { generateExecutable = value; } } ////// Gets or sets whether to generate an executable. /// ////// public bool GenerateInMemory { get { return generateInMemory; } set { generateInMemory = value; } } ////// Gets or sets whether to generate in memory. /// ////// public StringCollection ReferencedAssemblies { get { return assemblyNames; } } ////// Gets or sets the assemblies referenced by the source to compile. /// ////// public string MainClass { get { return mainClass; } set { mainClass = value; } } ////// Gets or sets the main class. /// ////// public string OutputAssembly { get { return outputName; } set { outputName = value; } } ////// Gets or sets the output assembly. /// ////// public TempFileCollection TempFiles { get { if (tempFiles == null) tempFiles = new TempFileCollection(); return tempFiles; } set { tempFiles = value; } } ////// Gets or sets the temp files. /// ////// public bool IncludeDebugInformation { get { return includeDebugInformation; } set { includeDebugInformation = value; } } ////// Gets or sets whether to include debug information in the compiled /// executable. /// ////// public bool TreatWarningsAsErrors { get { return treatWarningsAsErrors; } set { treatWarningsAsErrors = value; } } ///[To be supplied.] ////// public int WarningLevel { get { return warningLevel; } set { warningLevel = value; } } ///[To be supplied.] ////// public string CompilerOptions { get { return compilerOptions; } set { compilerOptions = value; } } ///[To be supplied.] ////// public string Win32Resource { get { return win32Resource; } set { win32Resource = value; } } ///[To be supplied.] ////// [System.Runtime.InteropServices.ComVisible(false)] public StringCollection EmbeddedResources { get { return embeddedResources; } } ////// Gets or sets the resources to be compiled into the target /// ////// [System.Runtime.InteropServices.ComVisible(false)] public StringCollection LinkedResources { get { return linkedResources; } } ////// Gets or sets the linked resources /// ////// public IntPtr UserToken { get { if (userToken != null) return userToken.DangerousGetHandle(); else return IntPtr.Zero; } set { if (userToken != null) userToken.Close(); userToken = new SafeUserTokenHandle(value, false); } } internal SafeUserTokenHandle SafeUserToken { get { return userToken; } } ////// Gets or sets user token to be employed when creating the compiler process. /// ////// public Evidence Evidence { get { Evidence e = null; if (evidence != null) e = CompilerResults.CloneEvidence(evidence); return e; } [SecurityPermissionAttribute( SecurityAction.Demand, ControlEvidence = true )] set { if (value != null) evidence = CompilerResults.CloneEvidence(value); else evidence = null; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Set the evidence for partially trusted scenarios. /// ///
Link Menu
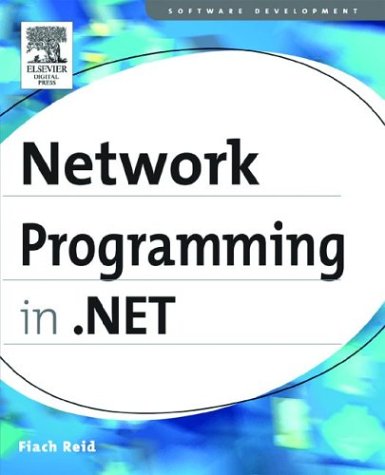
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BaseResourcesBuildProvider.cs
- DataBindingHandlerAttribute.cs
- SafeEventLogWriteHandle.cs
- SelectionItemPattern.cs
- HttpContextBase.cs
- MessageBuffer.cs
- TextTreeRootNode.cs
- Operators.cs
- RefreshPropertiesAttribute.cs
- Atom10FormatterFactory.cs
- DmlSqlGenerator.cs
- RegisteredArrayDeclaration.cs
- ExponentialEase.cs
- SafeNativeMemoryHandle.cs
- SafeFileMappingHandle.cs
- SevenBitStream.cs
- BaseDataBoundControl.cs
- OciEnlistContext.cs
- PublishLicense.cs
- OpacityConverter.cs
- BrushConverter.cs
- BaseParser.cs
- Internal.cs
- FileLoadException.cs
- Crypto.cs
- COM2PictureConverter.cs
- NegotiateStream.cs
- UriTemplateLiteralPathSegment.cs
- RoutedEventArgs.cs
- UnionCqlBlock.cs
- FamilyTypeface.cs
- CodeAccessPermission.cs
- ResourcePart.cs
- ISFClipboardData.cs
- DependencyPropertyKind.cs
- ProcessModelInfo.cs
- XpsSerializerWriter.cs
- InvariantComparer.cs
- SettingsPropertyCollection.cs
- SafeTokenHandle.cs
- RelationshipFixer.cs
- HandlerWithFactory.cs
- StaticFileHandler.cs
- XmlILCommand.cs
- DataGridViewButtonColumn.cs
- SqlCacheDependencySection.cs
- ResourceDescriptionAttribute.cs
- ScrollBar.cs
- TrackingServices.cs
- RelationshipType.cs
- ContractDescription.cs
- DefaultObjectMappingItemCollection.cs
- DataGridRowDetailsEventArgs.cs
- XNodeValidator.cs
- Graphics.cs
- NestPullup.cs
- RsaKeyIdentifierClause.cs
- DrawingCollection.cs
- ChooseAction.cs
- MarkupCompilePass1.cs
- ClaimSet.cs
- FixedSOMContainer.cs
- TypedDatasetGenerator.cs
- ToolStripOverflowButton.cs
- AlignmentXValidation.cs
- SqlFunctionAttribute.cs
- TypeToArgumentTypeConverter.cs
- TaiwanCalendar.cs
- BitmapScalingModeValidation.cs
- Brush.cs
- FileSystemWatcher.cs
- TypeLoadException.cs
- ConfigXmlDocument.cs
- WebBrowsableAttribute.cs
- Rules.cs
- NativeRightsManagementAPIsStructures.cs
- WasNotInstalledException.cs
- SystemUnicastIPAddressInformation.cs
- SettingsSavedEventArgs.cs
- WriteTimeStream.cs
- PathFigureCollectionConverter.cs
- SudsParser.cs
- PermissionRequestEvidence.cs
- ValidatingReaderNodeData.cs
- FixedStringLookup.cs
- LongAverageAggregationOperator.cs
- IList.cs
- UriExt.cs
- QuaternionValueSerializer.cs
- SafeArrayTypeMismatchException.cs
- ObjectListFieldCollection.cs
- RoleServiceManager.cs
- GlobalizationSection.cs
- WsdlParser.cs
- DesignTimeSiteMapProvider.cs
- HtmlUtf8RawTextWriter.cs
- TraceXPathNavigator.cs
- SettingsBindableAttribute.cs
- EnumBuilder.cs
- TreeViewTemplateSelector.cs