Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / clr / src / BCL / System / Threading / Timer.cs / 2 / Timer.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================================== ** ** Class: TimerQueue ** ** ** Purpose: Class for creating and managing a threadpool ** ** =============================================================================*/ namespace System.Threading { using System.Threading; using System; using System.Security; using System.Security.Permissions; using Microsoft.Win32; using System.Runtime.CompilerServices; using System.Runtime.InteropServices; using System.Runtime.ConstrainedExecution; internal class _TimerCallback { TimerCallback _timerCallback; ExecutionContext _executionContext; Object _state; static internal ContextCallback _ccb = new ContextCallback(TimerCallback_Context); static internal void TimerCallback_Context(Object state) { _TimerCallback helper = (_TimerCallback) state; helper._timerCallback(helper._state); } internal _TimerCallback(TimerCallback timerCallback, Object state, ref StackCrawlMark stackMark) { _timerCallback = timerCallback; _state = state; if (!ExecutionContext.IsFlowSuppressed()) { _executionContext = ExecutionContext.Capture(ref stackMark); ExecutionContext.ClearSyncContext(_executionContext); } } // call back helper static internal void PerformTimerCallback(Object state) { _TimerCallback helper = (_TimerCallback)state; BCLDebug.Assert(helper != null, "Null state passed to PerformTimerCallback!"); // call directly if EC flow is suppressed if (helper._executionContext == null) { TimerCallback callback = helper._timerCallback; callback(helper._state); } else { // From this point on we can use useExecutionContext for this callback ExecutionContext.Run(helper._executionContext.CreateCopy(), _ccb, helper); } } } [System.Runtime.InteropServices.ComVisible(true)] public delegate void TimerCallback(Object state); [HostProtection(Synchronization=true, ExternalThreading=true)] internal sealed class TimerBase : CriticalFinalizerObject, IDisposable { #pragma warning disable 169 private IntPtr timerHandle; private IntPtr delegateInfo; #pragma warning restore 169 private int timerDeleted; private int m_lock = 0; ~TimerBase() { // lock(this) cannot be used reliably in Cer since thin lock could be // promoted to syncblock and that is not a guaranteed operation bool bLockTaken = false; do { if (Interlocked.CompareExchange(ref m_lock, 1, 0) == 0) { bLockTaken = true; try { DeleteTimerNative(null); } finally { m_lock = 0; } } Thread.SpinWait(1); // yield to processor } while (!bLockTaken); } internal void AddTimer(TimerCallback callback, Object state, UInt32 dueTime, UInt32 period, ref StackCrawlMark stackMark ) { if (callback != null) { _TimerCallback callbackHelper = new _TimerCallback(callback, state, ref stackMark); state = (Object)callbackHelper; AddTimerNative(state, dueTime, period, ref stackMark); timerDeleted = 0; } else { throw new ArgumentNullException("TimerCallback"); } } [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] internal bool ChangeTimer(UInt32 dueTime,UInt32 period) { bool status = false; bool bLockTaken = false; // prepare here to prevent threadabort from occuring which could // destroy m_lock state. lock(this) can't be used due to critical // finalizer and thinlock/syncblock escalation. RuntimeHelpers.PrepareConstrainedRegions(); try { } finally { do { if (Interlocked.CompareExchange(ref m_lock, 1, 0) == 0) { bLockTaken = true; try { if (timerDeleted != 0) throw new ObjectDisposedException(null, Environment.GetResourceString("ObjectDisposed_Generic")); status = ChangeTimerNative(dueTime,period); } finally { m_lock = 0; } } Thread.SpinWait(1); // yield to processor } while (!bLockTaken); } return status; } [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] internal bool Dispose(WaitHandle notifyObject) { bool status = false; bool bLockTaken = false; RuntimeHelpers.PrepareConstrainedRegions(); try { } finally { do { if (Interlocked.CompareExchange(ref m_lock, 1, 0) == 0) { bLockTaken = true; try { status = DeleteTimerNative(notifyObject.SafeWaitHandle); } finally { m_lock = 0; } } Thread.SpinWait(1); // yield to processor } while (!bLockTaken); GC.SuppressFinalize(this); } return status; } [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] public void Dispose() { bool bLockTaken = false; RuntimeHelpers.PrepareConstrainedRegions(); try { } finally { do { if (Interlocked.CompareExchange(ref m_lock, 1, 0) == 0) { bLockTaken = true; try { DeleteTimerNative(null); } finally { m_lock = 0; } } Thread.SpinWait(1); // yield to processor } while (!bLockTaken); GC.SuppressFinalize(this); } } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern void AddTimerNative(Object state, UInt32 dueTime, UInt32 period, ref StackCrawlMark stackMark ); [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern bool ChangeTimerNative(UInt32 dueTime,UInt32 period); [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern bool DeleteTimerNative(SafeHandle notifyObject); } [HostProtection(Synchronization=true, ExternalThreading=true)] [System.Runtime.InteropServices.ComVisible(true)] public sealed class Timer : MarshalByRefObject, IDisposable { private const UInt32 MAX_SUPPORTED_TIMEOUT = (uint)0xfffffffe; private TimerBase timerBase; [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public Timer(TimerCallback callback, Object state, int dueTime, int period) { if (dueTime < -1) throw new ArgumentOutOfRangeException("dueTime", Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegOrNegative1")); if (period < -1 ) throw new ArgumentOutOfRangeException("period", Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegOrNegative1")); StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; TimerSetup(callback,state,(UInt32)dueTime,(UInt32)period,ref stackMark); } [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public Timer(TimerCallback callback, Object state, TimeSpan dueTime, TimeSpan period) { long dueTm = (long)dueTime.TotalMilliseconds; if (dueTm < -1) throw new ArgumentOutOfRangeException("dueTm",Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegOrNegative1")); if (dueTm > MAX_SUPPORTED_TIMEOUT) throw new ArgumentOutOfRangeException("dueTm",Environment.GetResourceString("ArgumentOutOfRange_TimeoutTooLarge")); long periodTm = (long)period.TotalMilliseconds; if (periodTm < -1) throw new ArgumentOutOfRangeException("periodTm",Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegOrNegative1")); if (periodTm > MAX_SUPPORTED_TIMEOUT) throw new ArgumentOutOfRangeException("periodTm",Environment.GetResourceString("ArgumentOutOfRange_PeriodTooLarge")); StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; TimerSetup(callback,state,(UInt32)dueTm,(UInt32)periodTm,ref stackMark); } [CLSCompliant(false)] [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public Timer(TimerCallback callback, Object state, UInt32 dueTime, UInt32 period) { StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; TimerSetup(callback,state,dueTime,period,ref stackMark); } [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public Timer(TimerCallback callback, Object state, long dueTime, long period) { if (dueTime < -1) throw new ArgumentOutOfRangeException("dueTime",Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegOrNegative1")); if (period < -1) throw new ArgumentOutOfRangeException("period",Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegOrNegative1")); if (dueTime > MAX_SUPPORTED_TIMEOUT) throw new ArgumentOutOfRangeException("dueTime",Environment.GetResourceString("ArgumentOutOfRange_TimeoutTooLarge")); if (period > MAX_SUPPORTED_TIMEOUT) throw new ArgumentOutOfRangeException("period",Environment.GetResourceString("ArgumentOutOfRange_PeriodTooLarge")); StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; TimerSetup(callback,state,(UInt32) dueTime, (UInt32) period,ref stackMark); } [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public Timer(TimerCallback callback) { int dueTime = -1; // we want timer to be registered, but not activated. Requires caller to call int period = -1; // Change after a timer instance is created. This is to avoid the potential // for a timer to be fired before the returned value is assigned to the variable, // potentially causing the callback to reference a bogus value (if passing the timer to the callback). StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; TimerSetup(callback, this, (UInt32)dueTime, (UInt32)period, ref stackMark); } private void TimerSetup(TimerCallback callback, Object state, UInt32 dueTime, UInt32 period, ref StackCrawlMark stackMark ) { timerBase = new TimerBase(); timerBase.AddTimer(callback, state,(UInt32) dueTime, (UInt32) period, ref stackMark); } public bool Change(int dueTime, int period) { if (dueTime < -1 ) throw new ArgumentOutOfRangeException("dueTime",Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegOrNegative1")); if (period < -1) throw new ArgumentOutOfRangeException("period",Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegOrNegative1")); return timerBase.ChangeTimer((UInt32)dueTime,(UInt32)period); } public bool Change(TimeSpan dueTime, TimeSpan period) { return Change((long) dueTime.TotalMilliseconds, (long) period.TotalMilliseconds); } [CLSCompliant(false)] public bool Change(UInt32 dueTime, UInt32 period) { return timerBase.ChangeTimer(dueTime,period); } public bool Change(long dueTime, long period) { if (dueTime < -1 ) throw new ArgumentOutOfRangeException("dueTime", Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegOrNegative1")); if (period < -1) throw new ArgumentOutOfRangeException("period", Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegOrNegative1")); if (dueTime > MAX_SUPPORTED_TIMEOUT) throw new ArgumentOutOfRangeException("dueTime", Environment.GetResourceString("ArgumentOutOfRange_TimeoutTooLarge")); if (period > MAX_SUPPORTED_TIMEOUT) throw new ArgumentOutOfRangeException("period", Environment.GetResourceString("ArgumentOutOfRange_PeriodTooLarge")); return timerBase.ChangeTimer((UInt32)dueTime,(UInt32)period); } public bool Dispose(WaitHandle notifyObject) { if (notifyObject==null) throw new ArgumentNullException("notifyObject"); return timerBase.Dispose(notifyObject); } public void Dispose() { timerBase.Dispose(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================================== ** ** Class: TimerQueue ** ** ** Purpose: Class for creating and managing a threadpool ** ** =============================================================================*/ namespace System.Threading { using System.Threading; using System; using System.Security; using System.Security.Permissions; using Microsoft.Win32; using System.Runtime.CompilerServices; using System.Runtime.InteropServices; using System.Runtime.ConstrainedExecution; internal class _TimerCallback { TimerCallback _timerCallback; ExecutionContext _executionContext; Object _state; static internal ContextCallback _ccb = new ContextCallback(TimerCallback_Context); static internal void TimerCallback_Context(Object state) { _TimerCallback helper = (_TimerCallback) state; helper._timerCallback(helper._state); } internal _TimerCallback(TimerCallback timerCallback, Object state, ref StackCrawlMark stackMark) { _timerCallback = timerCallback; _state = state; if (!ExecutionContext.IsFlowSuppressed()) { _executionContext = ExecutionContext.Capture(ref stackMark); ExecutionContext.ClearSyncContext(_executionContext); } } // call back helper static internal void PerformTimerCallback(Object state) { _TimerCallback helper = (_TimerCallback)state; BCLDebug.Assert(helper != null, "Null state passed to PerformTimerCallback!"); // call directly if EC flow is suppressed if (helper._executionContext == null) { TimerCallback callback = helper._timerCallback; callback(helper._state); } else { // From this point on we can use useExecutionContext for this callback ExecutionContext.Run(helper._executionContext.CreateCopy(), _ccb, helper); } } } [System.Runtime.InteropServices.ComVisible(true)] public delegate void TimerCallback(Object state); [HostProtection(Synchronization=true, ExternalThreading=true)] internal sealed class TimerBase : CriticalFinalizerObject, IDisposable { #pragma warning disable 169 private IntPtr timerHandle; private IntPtr delegateInfo; #pragma warning restore 169 private int timerDeleted; private int m_lock = 0; ~TimerBase() { // lock(this) cannot be used reliably in Cer since thin lock could be // promoted to syncblock and that is not a guaranteed operation bool bLockTaken = false; do { if (Interlocked.CompareExchange(ref m_lock, 1, 0) == 0) { bLockTaken = true; try { DeleteTimerNative(null); } finally { m_lock = 0; } } Thread.SpinWait(1); // yield to processor } while (!bLockTaken); } internal void AddTimer(TimerCallback callback, Object state, UInt32 dueTime, UInt32 period, ref StackCrawlMark stackMark ) { if (callback != null) { _TimerCallback callbackHelper = new _TimerCallback(callback, state, ref stackMark); state = (Object)callbackHelper; AddTimerNative(state, dueTime, period, ref stackMark); timerDeleted = 0; } else { throw new ArgumentNullException("TimerCallback"); } } [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] internal bool ChangeTimer(UInt32 dueTime,UInt32 period) { bool status = false; bool bLockTaken = false; // prepare here to prevent threadabort from occuring which could // destroy m_lock state. lock(this) can't be used due to critical // finalizer and thinlock/syncblock escalation. RuntimeHelpers.PrepareConstrainedRegions(); try { } finally { do { if (Interlocked.CompareExchange(ref m_lock, 1, 0) == 0) { bLockTaken = true; try { if (timerDeleted != 0) throw new ObjectDisposedException(null, Environment.GetResourceString("ObjectDisposed_Generic")); status = ChangeTimerNative(dueTime,period); } finally { m_lock = 0; } } Thread.SpinWait(1); // yield to processor } while (!bLockTaken); } return status; } [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] internal bool Dispose(WaitHandle notifyObject) { bool status = false; bool bLockTaken = false; RuntimeHelpers.PrepareConstrainedRegions(); try { } finally { do { if (Interlocked.CompareExchange(ref m_lock, 1, 0) == 0) { bLockTaken = true; try { status = DeleteTimerNative(notifyObject.SafeWaitHandle); } finally { m_lock = 0; } } Thread.SpinWait(1); // yield to processor } while (!bLockTaken); GC.SuppressFinalize(this); } return status; } [ReliabilityContract(Consistency.WillNotCorruptState, Cer.MayFail)] public void Dispose() { bool bLockTaken = false; RuntimeHelpers.PrepareConstrainedRegions(); try { } finally { do { if (Interlocked.CompareExchange(ref m_lock, 1, 0) == 0) { bLockTaken = true; try { DeleteTimerNative(null); } finally { m_lock = 0; } } Thread.SpinWait(1); // yield to processor } while (!bLockTaken); GC.SuppressFinalize(this); } } [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern void AddTimerNative(Object state, UInt32 dueTime, UInt32 period, ref StackCrawlMark stackMark ); [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern bool ChangeTimerNative(UInt32 dueTime,UInt32 period); [MethodImplAttribute(MethodImplOptions.InternalCall)] private extern bool DeleteTimerNative(SafeHandle notifyObject); } [HostProtection(Synchronization=true, ExternalThreading=true)] [System.Runtime.InteropServices.ComVisible(true)] public sealed class Timer : MarshalByRefObject, IDisposable { private const UInt32 MAX_SUPPORTED_TIMEOUT = (uint)0xfffffffe; private TimerBase timerBase; [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public Timer(TimerCallback callback, Object state, int dueTime, int period) { if (dueTime < -1) throw new ArgumentOutOfRangeException("dueTime", Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegOrNegative1")); if (period < -1 ) throw new ArgumentOutOfRangeException("period", Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegOrNegative1")); StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; TimerSetup(callback,state,(UInt32)dueTime,(UInt32)period,ref stackMark); } [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public Timer(TimerCallback callback, Object state, TimeSpan dueTime, TimeSpan period) { long dueTm = (long)dueTime.TotalMilliseconds; if (dueTm < -1) throw new ArgumentOutOfRangeException("dueTm",Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegOrNegative1")); if (dueTm > MAX_SUPPORTED_TIMEOUT) throw new ArgumentOutOfRangeException("dueTm",Environment.GetResourceString("ArgumentOutOfRange_TimeoutTooLarge")); long periodTm = (long)period.TotalMilliseconds; if (periodTm < -1) throw new ArgumentOutOfRangeException("periodTm",Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegOrNegative1")); if (periodTm > MAX_SUPPORTED_TIMEOUT) throw new ArgumentOutOfRangeException("periodTm",Environment.GetResourceString("ArgumentOutOfRange_PeriodTooLarge")); StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; TimerSetup(callback,state,(UInt32)dueTm,(UInt32)periodTm,ref stackMark); } [CLSCompliant(false)] [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public Timer(TimerCallback callback, Object state, UInt32 dueTime, UInt32 period) { StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; TimerSetup(callback,state,dueTime,period,ref stackMark); } [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public Timer(TimerCallback callback, Object state, long dueTime, long period) { if (dueTime < -1) throw new ArgumentOutOfRangeException("dueTime",Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegOrNegative1")); if (period < -1) throw new ArgumentOutOfRangeException("period",Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegOrNegative1")); if (dueTime > MAX_SUPPORTED_TIMEOUT) throw new ArgumentOutOfRangeException("dueTime",Environment.GetResourceString("ArgumentOutOfRange_TimeoutTooLarge")); if (period > MAX_SUPPORTED_TIMEOUT) throw new ArgumentOutOfRangeException("period",Environment.GetResourceString("ArgumentOutOfRange_PeriodTooLarge")); StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; TimerSetup(callback,state,(UInt32) dueTime, (UInt32) period,ref stackMark); } [MethodImplAttribute(MethodImplOptions.NoInlining)] // Methods containing StackCrawlMark local var has to be marked non-inlineable public Timer(TimerCallback callback) { int dueTime = -1; // we want timer to be registered, but not activated. Requires caller to call int period = -1; // Change after a timer instance is created. This is to avoid the potential // for a timer to be fired before the returned value is assigned to the variable, // potentially causing the callback to reference a bogus value (if passing the timer to the callback). StackCrawlMark stackMark = StackCrawlMark.LookForMyCaller; TimerSetup(callback, this, (UInt32)dueTime, (UInt32)period, ref stackMark); } private void TimerSetup(TimerCallback callback, Object state, UInt32 dueTime, UInt32 period, ref StackCrawlMark stackMark ) { timerBase = new TimerBase(); timerBase.AddTimer(callback, state,(UInt32) dueTime, (UInt32) period, ref stackMark); } public bool Change(int dueTime, int period) { if (dueTime < -1 ) throw new ArgumentOutOfRangeException("dueTime",Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegOrNegative1")); if (period < -1) throw new ArgumentOutOfRangeException("period",Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegOrNegative1")); return timerBase.ChangeTimer((UInt32)dueTime,(UInt32)period); } public bool Change(TimeSpan dueTime, TimeSpan period) { return Change((long) dueTime.TotalMilliseconds, (long) period.TotalMilliseconds); } [CLSCompliant(false)] public bool Change(UInt32 dueTime, UInt32 period) { return timerBase.ChangeTimer(dueTime,period); } public bool Change(long dueTime, long period) { if (dueTime < -1 ) throw new ArgumentOutOfRangeException("dueTime", Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegOrNegative1")); if (period < -1) throw new ArgumentOutOfRangeException("period", Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegOrNegative1")); if (dueTime > MAX_SUPPORTED_TIMEOUT) throw new ArgumentOutOfRangeException("dueTime", Environment.GetResourceString("ArgumentOutOfRange_TimeoutTooLarge")); if (period > MAX_SUPPORTED_TIMEOUT) throw new ArgumentOutOfRangeException("period", Environment.GetResourceString("ArgumentOutOfRange_PeriodTooLarge")); return timerBase.ChangeTimer((UInt32)dueTime,(UInt32)period); } public bool Dispose(WaitHandle notifyObject) { if (notifyObject==null) throw new ArgumentNullException("notifyObject"); return timerBase.Dispose(notifyObject); } public void Dispose() { timerBase.Dispose(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
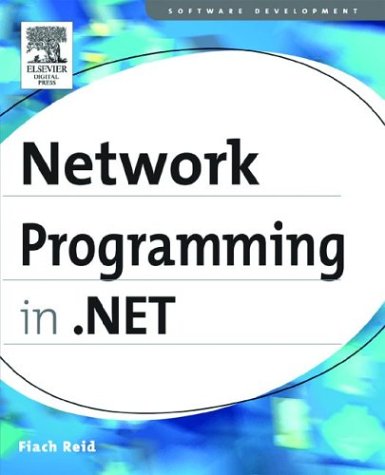
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ArcSegment.cs
- Rect.cs
- SmtpClient.cs
- TypedTableGenerator.cs
- AttributeProviderAttribute.cs
- ComponentFactoryHelpers.cs
- IUnknownConstantAttribute.cs
- SubqueryTrackingVisitor.cs
- Timer.cs
- FolderBrowserDialog.cs
- SqlTopReducer.cs
- SelectorItemAutomationPeer.cs
- CacheForPrimitiveTypes.cs
- CapabilitiesUse.cs
- FocusManager.cs
- FirstQueryOperator.cs
- ExceptionDetail.cs
- SizeAnimationBase.cs
- ToolboxItemWrapper.cs
- StrokeDescriptor.cs
- PingReply.cs
- SqlServices.cs
- InvalidWMPVersionException.cs
- CompoundFileReference.cs
- SoundPlayer.cs
- X509ServiceCertificateAuthentication.cs
- SqlFormatter.cs
- MouseWheelEventArgs.cs
- WindowsListViewGroupHelper.cs
- LocalizedNameDescriptionPair.cs
- Registry.cs
- SoapSchemaMember.cs
- DirectoryGroupQuery.cs
- NetMsmqSecurityMode.cs
- WindowsStatic.cs
- ToolStripContainerActionList.cs
- InternalException.cs
- Adorner.cs
- MessageDesigner.cs
- WpfGeneratedKnownProperties.cs
- Font.cs
- HeaderPanel.cs
- HealthMonitoringSectionHelper.cs
- SinglePageViewer.cs
- ImpersonateTokenRef.cs
- WeakEventTable.cs
- XmlProcessingInstruction.cs
- Adorner.cs
- ReverseComparer.cs
- TheQuery.cs
- SqlDataAdapter.cs
- LinkConverter.cs
- DoubleAnimationBase.cs
- XmlElementElement.cs
- PopOutPanel.cs
- ElementProxy.cs
- BookmarkEventArgs.cs
- _SslSessionsCache.cs
- DataBinding.cs
- ComboBox.cs
- TrackingMemoryStreamFactory.cs
- _HelperAsyncResults.cs
- SetMemberBinder.cs
- unsafenativemethodsother.cs
- ProviderConnectionPointCollection.cs
- DesignerWithHeader.cs
- MgmtConfigurationRecord.cs
- WmfPlaceableFileHeader.cs
- TemplateLookupAction.cs
- ProcessHostMapPath.cs
- BamlStream.cs
- GlyphInfoList.cs
- IdentityReference.cs
- BrushConverter.cs
- EventBookmark.cs
- SelectedDatesCollection.cs
- ItemMap.cs
- TraceHandler.cs
- DesignDataSource.cs
- EdmSchemaError.cs
- SoapMessage.cs
- DeclarativeCatalogPart.cs
- FormattedText.cs
- ObjectQuery.cs
- ConfigurationLocationCollection.cs
- util.cs
- SoapSchemaMember.cs
- DelegateCompletionCallbackWrapper.cs
- MaskedTextProvider.cs
- WorkflowElementDialog.cs
- PartManifestEntry.cs
- RangeBase.cs
- Currency.cs
- ListSourceHelper.cs
- FlowchartSizeFeature.cs
- GenerateHelper.cs
- EndpointIdentityConverter.cs
- TraceInternal.cs
- SerializerWriterEventHandlers.cs
- DesignTimeVisibleAttribute.cs