Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / UIAutomation / UIAutomationTypes / System / Windows / Automation / AutomationIdentifier.cs / 1 / AutomationIdentifier.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Base class for Automation Idenfitiers (Property, Event, etc.) // // History: // 06/02/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Diagnostics; using MS.Internal.Automation; namespace System.Windows.Automation { ////// Base class for object identity based identifiers. /// Implement ISerializable to ensure that it remotes propertly /// This class is effectively abstract, only derived classes are /// instantiated. /// #if (INTERNAL_COMPILE) internal class AutomationIdentifier : IComparable #else public class AutomationIdentifier : IComparable #endif { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors // Internal so only our own derived classes can actually // use this class. (3rd party classes can try deriving, // but the internal ctor will prevent instantiation.) internal AutomationIdentifier(UiaCoreTypesApi.AutomationIdType type, int id, Guid guid, string programmaticName) { Debug.Assert(id != 0); _id = id; _type = type; _guid = guid; _programmaticName = programmaticName; } #endregion Constructors //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// Returns underlying identifier as used by provider interfaces. /// ////// Use LookupById method to convert back from Id to an /// AutomationIdentifier /// public int Id { get { return _id; } } ////// Returns the programmatic name passed in on registration. /// ////// Appends the type to the programmatic name. /// public string ProgrammaticName { get { return _programmaticName; } } #endregion Public Properties //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public Methods ////// Tests whether two AutomationIdentifier objects are equivalent /// public override bool Equals( object obj ) { return obj == (object)this; } ////// Overrides Object.GetHashCode() /// public override int GetHashCode() { return base.GetHashCode(); } ////// For IComparable() /// public int CompareTo(object obj) { Debug.Assert(obj != null, "Null obj!"); if (obj == null) throw new ArgumentNullException("obj"); // Ordering allows arrays of references to these to be sorted - though the sort order is undefined. Debug.Assert(obj is AutomationIdentifier, "CompareTo called with unexpected type"); return GetHashCode() - obj.GetHashCode(); } #endregion Public Methods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods internal static AutomationIdentifier Register(UiaCoreTypesApi.AutomationIdType type, Guid guid, string programmaticName) { // Get internal id from the guid... int id = UiaCoreTypesApi.UiaLookupId(type, ref guid); if (id == 0) { Debug.Assert(id != 0, "Identifier not recognized by core - " + guid); return null; } lock (_idTable) { // See if instance already exists... AutomationIdentifier autoid = (AutomationIdentifier)_idTable[guid]; if (autoid != null) { return autoid; } // If not, create one... switch (type) { case UiaCoreTypesApi.AutomationIdType.Property: autoid = new AutomationProperty(id, guid, programmaticName); break; case UiaCoreTypesApi.AutomationIdType.Event: autoid = new AutomationEvent(id, guid, programmaticName); break; case UiaCoreTypesApi.AutomationIdType.TextAttribute: autoid = new AutomationTextAttribute(id, guid, programmaticName); break; case UiaCoreTypesApi.AutomationIdType.Pattern: autoid = new AutomationPattern(id, guid, programmaticName); break; case UiaCoreTypesApi.AutomationIdType.ControlType: autoid = new ControlType(id, guid, programmaticName); break; default: Debug.Assert(false, "Invalid type specified for AutomationIdentifier"); throw new InvalidOperationException("Invalid type specified for AutomationIdentifier"); } _idTable[id] = autoid; return autoid; } } internal static AutomationIdentifier LookupById(UiaCoreTypesApi.AutomationIdType type, int id) { AutomationIdentifier autoid; lock (_idTable) { autoid = (AutomationIdentifier) _idTable[id]; } if(autoid == null) { return null; } if(autoid._type != type) { return null; } return autoid; } #endregion Internal Methods //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private Guid _guid; private UiaCoreTypesApi.AutomationIdType _type; private int _id; // value used in core private string _programmaticName; // As of 8/18/03 there were 187 entries added in a normal loading private static Hashtable _idTable = new Hashtable(200,1.0f); #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Base class for Automation Idenfitiers (Property, Event, etc.) // // History: // 06/02/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Diagnostics; using MS.Internal.Automation; namespace System.Windows.Automation { ////// Base class for object identity based identifiers. /// Implement ISerializable to ensure that it remotes propertly /// This class is effectively abstract, only derived classes are /// instantiated. /// #if (INTERNAL_COMPILE) internal class AutomationIdentifier : IComparable #else public class AutomationIdentifier : IComparable #endif { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors // Internal so only our own derived classes can actually // use this class. (3rd party classes can try deriving, // but the internal ctor will prevent instantiation.) internal AutomationIdentifier(UiaCoreTypesApi.AutomationIdType type, int id, Guid guid, string programmaticName) { Debug.Assert(id != 0); _id = id; _type = type; _guid = guid; _programmaticName = programmaticName; } #endregion Constructors //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// Returns underlying identifier as used by provider interfaces. /// ////// Use LookupById method to convert back from Id to an /// AutomationIdentifier /// public int Id { get { return _id; } } ////// Returns the programmatic name passed in on registration. /// ////// Appends the type to the programmatic name. /// public string ProgrammaticName { get { return _programmaticName; } } #endregion Public Properties //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public Methods ////// Tests whether two AutomationIdentifier objects are equivalent /// public override bool Equals( object obj ) { return obj == (object)this; } ////// Overrides Object.GetHashCode() /// public override int GetHashCode() { return base.GetHashCode(); } ////// For IComparable() /// public int CompareTo(object obj) { Debug.Assert(obj != null, "Null obj!"); if (obj == null) throw new ArgumentNullException("obj"); // Ordering allows arrays of references to these to be sorted - though the sort order is undefined. Debug.Assert(obj is AutomationIdentifier, "CompareTo called with unexpected type"); return GetHashCode() - obj.GetHashCode(); } #endregion Public Methods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods internal static AutomationIdentifier Register(UiaCoreTypesApi.AutomationIdType type, Guid guid, string programmaticName) { // Get internal id from the guid... int id = UiaCoreTypesApi.UiaLookupId(type, ref guid); if (id == 0) { Debug.Assert(id != 0, "Identifier not recognized by core - " + guid); return null; } lock (_idTable) { // See if instance already exists... AutomationIdentifier autoid = (AutomationIdentifier)_idTable[guid]; if (autoid != null) { return autoid; } // If not, create one... switch (type) { case UiaCoreTypesApi.AutomationIdType.Property: autoid = new AutomationProperty(id, guid, programmaticName); break; case UiaCoreTypesApi.AutomationIdType.Event: autoid = new AutomationEvent(id, guid, programmaticName); break; case UiaCoreTypesApi.AutomationIdType.TextAttribute: autoid = new AutomationTextAttribute(id, guid, programmaticName); break; case UiaCoreTypesApi.AutomationIdType.Pattern: autoid = new AutomationPattern(id, guid, programmaticName); break; case UiaCoreTypesApi.AutomationIdType.ControlType: autoid = new ControlType(id, guid, programmaticName); break; default: Debug.Assert(false, "Invalid type specified for AutomationIdentifier"); throw new InvalidOperationException("Invalid type specified for AutomationIdentifier"); } _idTable[id] = autoid; return autoid; } } internal static AutomationIdentifier LookupById(UiaCoreTypesApi.AutomationIdType type, int id) { AutomationIdentifier autoid; lock (_idTable) { autoid = (AutomationIdentifier) _idTable[id]; } if(autoid == null) { return null; } if(autoid._type != type) { return null; } return autoid; } #endregion Internal Methods //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private Guid _guid; private UiaCoreTypesApi.AutomationIdType _type; private int _id; // value used in core private string _programmaticName; // As of 8/18/03 there were 187 entries added in a normal loading private static Hashtable _idTable = new Hashtable(200,1.0f); #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
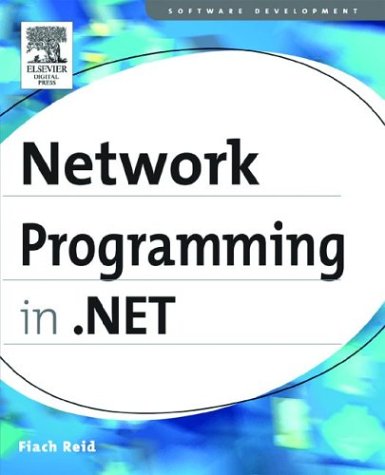
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GeneralTransform3DCollection.cs
- SafeProcessHandle.cs
- SqlColumnizer.cs
- OleDbParameter.cs
- WindowsPen.cs
- IFormattable.cs
- ToolStripArrowRenderEventArgs.cs
- Model3DCollection.cs
- BindingMAnagerBase.cs
- SrgsNameValueTag.cs
- Blend.cs
- ModuleBuilder.cs
- ContextActivityUtils.cs
- AutomationPatternInfo.cs
- EncodingNLS.cs
- XsltCompileContext.cs
- EntitySqlQueryCacheKey.cs
- ComponentCollection.cs
- ObjectStateEntryOriginalDbUpdatableDataRecord.cs
- OrthographicCamera.cs
- DataProviderNameConverter.cs
- cache.cs
- ComponentGuaranteesAttribute.cs
- Win32KeyboardDevice.cs
- ResourceFallbackManager.cs
- MimeFormReflector.cs
- MediaSystem.cs
- TextLineBreak.cs
- GridViewCommandEventArgs.cs
- IdnElement.cs
- precedingquery.cs
- NetTcpBindingCollectionElement.cs
- CultureTable.cs
- CachedTypeface.cs
- ConfigurationStrings.cs
- AuthenticationManager.cs
- ParserExtension.cs
- PrinterSettings.cs
- WebPartsSection.cs
- TraceInternal.cs
- ScrollChrome.cs
- HtmlControlDesigner.cs
- AsymmetricAlgorithm.cs
- ToolStripContentPanel.cs
- GlyphRunDrawing.cs
- DeflateStreamAsyncResult.cs
- DataGridViewLinkColumn.cs
- PerformanceCounterPermission.cs
- ButtonFlatAdapter.cs
- ExpressionNode.cs
- PkcsUtils.cs
- SqlTriggerContext.cs
- MtomMessageEncodingElement.cs
- AuthenticationService.cs
- FillBehavior.cs
- PauseStoryboard.cs
- DictionaryGlobals.cs
- SecurityPolicySection.cs
- StylusPlugInCollection.cs
- ChineseLunisolarCalendar.cs
- JoinTreeSlot.cs
- DataGridDetailsPresenter.cs
- DBNull.cs
- connectionpool.cs
- UIElementPropertyUndoUnit.cs
- NullExtension.cs
- UriTemplateTrieNode.cs
- PackWebRequest.cs
- RangeBase.cs
- XmlTypeAttribute.cs
- SqlDataSourceEnumerator.cs
- FormatConvertedBitmap.cs
- SerializationException.cs
- CustomWebEventKey.cs
- DrawingImage.cs
- DataGridPreparingCellForEditEventArgs.cs
- XmlAttributeHolder.cs
- PropertyInfoSet.cs
- RemotingConfiguration.cs
- RedirectionProxy.cs
- XmlStringTable.cs
- PersonalizationStateInfoCollection.cs
- StringComparer.cs
- TextWriter.cs
- WebPartDeleteVerb.cs
- CommonDialog.cs
- JsonFormatWriterGenerator.cs
- CodeSubDirectoriesCollection.cs
- ReadOnlyDataSource.cs
- PreApplicationStartMethodAttribute.cs
- ConfigurationElementProperty.cs
- AutoScrollHelper.cs
- WebWorkflowRole.cs
- DataGridViewSelectedCellsAccessibleObject.cs
- DesigntimeLicenseContext.cs
- AnnotationResourceChangedEventArgs.cs
- SignedXml.cs
- LogicalExpressionTypeConverter.cs
- WebBrowserNavigatingEventHandler.cs
- NativeMethods.cs