Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Markup / XamlWriter.cs / 1 / XamlWriter.cs
//---------------------------------------------------------------------------- // // File: XamlWriter.cs // // Description: // base Parser class that parses XML markup into an Avalon Element Tree // // // History: // 6/06/01: rogerg Created as Parser.cs // 5/29/03: peterost Ported to wcp as Parser.cs // 8/04/05: a-neabbu Split Parser into XamlReader and XamlWriter // // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Xml; using System.IO; using System.IO.Packaging; using System.Windows; using System.Collections; using System.Diagnostics; using System.Reflection; using System.Windows.Threading; using MS.Utility; using System.Security; using System.Security.Permissions; using System.Security.Policy; using System.Text; using System.ComponentModel.Design.Serialization; using System.Globalization; using System.Windows.Markup.Primitives; using MS.Internal.IO.Packaging; using MS.Internal.PresentationFramework; namespace System.Windows.Markup { ////// Parsing class used to create an Windows Presentation Platform Tree /// public static class XamlWriter { #region Public Methods ////// Save gets the xml respresentation /// for the given object instance /// /// /// Object instance /// ////// XAML string representing object instance /// ////// We only allow Serialization in partial trust. Although we would throw an exception later anyways, /// we throw one here so we know where to expect the exception. ( public static string Save(object obj) { // Must be in full trust SecurityHelper.DemandUnmanagedCode(); // Validate input arguments if (obj == null) { throw new ArgumentNullException("obj"); } // Create TextWriter StringBuilder sb = new StringBuilder(); TextWriter writer = new StringWriter(sb, XamlSerializerUtil.EnglishUSCulture); try { Save(obj, writer); } finally { // Close writer writer.Close(); } return sb.ToString(); } /// /// Save writes the xml respresentation /// for the given object instance using the given writer /// /// /// Object instance /// /// /// Text Writer /// ////// We only allow Serialization in partial trust. Although we would throw an exception later anyways, /// we throw one here so we know where to expect the exception. ( public static void Save(object obj, TextWriter writer) { // Must be in full trust SecurityHelper.DemandUnmanagedCode(); // Validate input arguments if (obj == null) { throw new ArgumentNullException("obj"); } if (writer == null) { throw new ArgumentNullException("writer"); } // Create XmlTextWriter XmlTextWriter xmlWriter = new XmlTextWriter(writer); MarkupWriter.SaveAsXml(xmlWriter, obj); } /// /// Save writes the xml respresentation /// for the given object instance to the given stream /// /// /// Object instance /// /// /// Stream /// ////// We only allow Serialization in partial trust. Although we would throw an exception later anyways, /// we throw one here so we know where to expect the exception. ( public static void Save(object obj, Stream stream) { // Must be in full trust SecurityHelper.DemandUnmanagedCode(); // Validate input arguments if (obj == null) { throw new ArgumentNullException("obj"); } if (stream == null) { throw new ArgumentNullException("stream"); } // Create XmlTextWriter XmlTextWriter xmlWriter = new XmlTextWriter(stream, null); MarkupWriter.SaveAsXml(xmlWriter, obj); } /// /// Save writes the xml respresentation /// for the given object instance using the given /// writer. In addition it also allows the designer /// to participate in this conversion. /// /// /// Object instance /// /// /// XmlWriter /// ////// We only allow Serialization in partial trust. Although we would throw an exception later anyways, /// we throw one here so we know where to expect the exception. ( public static void Save(object obj, XmlWriter xmlWriter) { // Must be in full trust SecurityHelper.DemandUnmanagedCode(); // Validate input arguments if (obj == null) { throw new ArgumentNullException("obj"); } if (xmlWriter == null) { throw new ArgumentNullException("xmlWriter"); } try { MarkupWriter.SaveAsXml(xmlWriter, obj); } finally { xmlWriter.Flush(); } } /// /// Save writes the xml respresentation /// for the given object instance using the /// given XmlTextWriter embedded in the manager. /// /// /// Object instance /// /// /// Serialization Manager /// ////// We only allow Serialization in partial trust. Although we would throw an exception later anyways, /// we throw one here so we know where to expect the exception. ( public static void Save(object obj, XamlDesignerSerializationManager manager) { // Must be in full trust SecurityHelper.DemandUnmanagedCode(); // Validate input arguments if (obj == null) { throw new ArgumentNullException("obj"); } if (manager == null) { throw new ArgumentNullException("manager"); } MarkupWriter.SaveAsXml(manager.XmlWriter, obj, manager); } #endregion Public Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: XamlWriter.cs // // Description: // base Parser class that parses XML markup into an Avalon Element Tree // // // History: // 6/06/01: rogerg Created as Parser.cs // 5/29/03: peterost Ported to wcp as Parser.cs // 8/04/05: a-neabbu Split Parser into XamlReader and XamlWriter // // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Xml; using System.IO; using System.IO.Packaging; using System.Windows; using System.Collections; using System.Diagnostics; using System.Reflection; using System.Windows.Threading; using MS.Utility; using System.Security; using System.Security.Permissions; using System.Security.Policy; using System.Text; using System.ComponentModel.Design.Serialization; using System.Globalization; using System.Windows.Markup.Primitives; using MS.Internal.IO.Packaging; using MS.Internal.PresentationFramework; namespace System.Windows.Markup { /// /// Parsing class used to create an Windows Presentation Platform Tree /// public static class XamlWriter { #region Public Methods ////// Save gets the xml respresentation /// for the given object instance /// /// /// Object instance /// ////// XAML string representing object instance /// ////// We only allow Serialization in partial trust. Although we would throw an exception later anyways, /// we throw one here so we know where to expect the exception. ( public static string Save(object obj) { // Must be in full trust SecurityHelper.DemandUnmanagedCode(); // Validate input arguments if (obj == null) { throw new ArgumentNullException("obj"); } // Create TextWriter StringBuilder sb = new StringBuilder(); TextWriter writer = new StringWriter(sb, XamlSerializerUtil.EnglishUSCulture); try { Save(obj, writer); } finally { // Close writer writer.Close(); } return sb.ToString(); } /// /// Save writes the xml respresentation /// for the given object instance using the given writer /// /// /// Object instance /// /// /// Text Writer /// ////// We only allow Serialization in partial trust. Although we would throw an exception later anyways, /// we throw one here so we know where to expect the exception. ( public static void Save(object obj, TextWriter writer) { // Must be in full trust SecurityHelper.DemandUnmanagedCode(); // Validate input arguments if (obj == null) { throw new ArgumentNullException("obj"); } if (writer == null) { throw new ArgumentNullException("writer"); } // Create XmlTextWriter XmlTextWriter xmlWriter = new XmlTextWriter(writer); MarkupWriter.SaveAsXml(xmlWriter, obj); } /// /// Save writes the xml respresentation /// for the given object instance to the given stream /// /// /// Object instance /// /// /// Stream /// ////// We only allow Serialization in partial trust. Although we would throw an exception later anyways, /// we throw one here so we know where to expect the exception. ( public static void Save(object obj, Stream stream) { // Must be in full trust SecurityHelper.DemandUnmanagedCode(); // Validate input arguments if (obj == null) { throw new ArgumentNullException("obj"); } if (stream == null) { throw new ArgumentNullException("stream"); } // Create XmlTextWriter XmlTextWriter xmlWriter = new XmlTextWriter(stream, null); MarkupWriter.SaveAsXml(xmlWriter, obj); } /// /// Save writes the xml respresentation /// for the given object instance using the given /// writer. In addition it also allows the designer /// to participate in this conversion. /// /// /// Object instance /// /// /// XmlWriter /// ////// We only allow Serialization in partial trust. Although we would throw an exception later anyways, /// we throw one here so we know where to expect the exception. ( public static void Save(object obj, XmlWriter xmlWriter) { // Must be in full trust SecurityHelper.DemandUnmanagedCode(); // Validate input arguments if (obj == null) { throw new ArgumentNullException("obj"); } if (xmlWriter == null) { throw new ArgumentNullException("xmlWriter"); } try { MarkupWriter.SaveAsXml(xmlWriter, obj); } finally { xmlWriter.Flush(); } } /// /// Save writes the xml respresentation /// for the given object instance using the /// given XmlTextWriter embedded in the manager. /// /// /// Object instance /// /// /// Serialization Manager /// ////// We only allow Serialization in partial trust. Although we would throw an exception later anyways, /// we throw one here so we know where to expect the exception. ( public static void Save(object obj, XamlDesignerSerializationManager manager) { // Must be in full trust SecurityHelper.DemandUnmanagedCode(); // Validate input arguments if (obj == null) { throw new ArgumentNullException("obj"); } if (manager == null) { throw new ArgumentNullException("manager"); } MarkupWriter.SaveAsXml(manager.XmlWriter, obj, manager); } #endregion Public Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
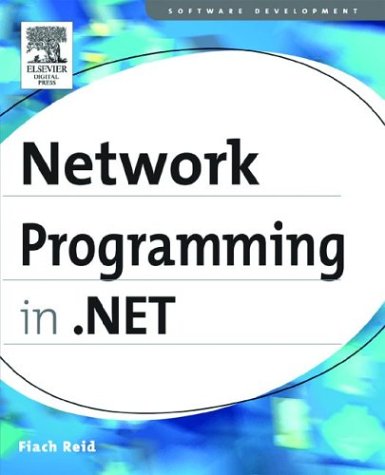
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ModuleConfigurationInfo.cs
- ReachSerializationCacheItems.cs
- DrawingAttributesDefaultValueFactory.cs
- SqlServices.cs
- SqlTransaction.cs
- HttpContextServiceHost.cs
- CallInfo.cs
- ItemDragEvent.cs
- RecommendedAsConfigurableAttribute.cs
- StackSpiller.Temps.cs
- StrongName.cs
- ServiceDocumentFormatter.cs
- AllMembershipCondition.cs
- DocumentPageViewAutomationPeer.cs
- XPathQilFactory.cs
- ToolboxItemAttribute.cs
- SafeThemeHandle.cs
- ResourceProperty.cs
- ChameleonKey.cs
- DbParameterCollection.cs
- IdentityNotMappedException.cs
- ExpressionBuilderCollection.cs
- Timer.cs
- BaseCodeDomTreeGenerator.cs
- SortQuery.cs
- FileNotFoundException.cs
- WebResponse.cs
- DataGridLength.cs
- PersistenceContextEnlistment.cs
- Query.cs
- SelectionListDesigner.cs
- AspNetHostingPermission.cs
- EditorZoneBase.cs
- XmlException.cs
- Verify.cs
- ResourceSetExpression.cs
- WindowsProgressbar.cs
- BaseUriHelper.cs
- TrustManager.cs
- WebControlsSection.cs
- SignatureDescription.cs
- ServiceParser.cs
- WebEventCodes.cs
- ContainsRowNumberChecker.cs
- SslStream.cs
- ConsumerConnectionPointCollection.cs
- XmlSchemaExporter.cs
- InvalidProgramException.cs
- ExceptionHandlers.cs
- MappingModelBuildProvider.cs
- InvalidAsynchronousStateException.cs
- TimeSpanStorage.cs
- CurrentTimeZone.cs
- DataTableMappingCollection.cs
- SectionVisual.cs
- EventHandlerService.cs
- SystemIcons.cs
- SamlDelegatingWriter.cs
- CollectionBuilder.cs
- CSharpCodeProvider.cs
- XmlSchemaExternal.cs
- CodeChecksumPragma.cs
- smtppermission.cs
- XsltException.cs
- HtmlSelect.cs
- BitSet.cs
- PointHitTestResult.cs
- TerminateWorkflow.cs
- SecurityValidationBehavior.cs
- RepeaterItemCollection.cs
- ManagementClass.cs
- NetMsmqBindingElement.cs
- EntityDataSourceChangingEventArgs.cs
- HierarchicalDataBoundControlAdapter.cs
- SendMailErrorEventArgs.cs
- XmlNodeChangedEventArgs.cs
- ProfilePropertySettings.cs
- DataPagerFieldCollection.cs
- InputDevice.cs
- ContentValidator.cs
- storagemappingitemcollection.viewdictionary.cs
- DrawingVisual.cs
- DictionaryCustomTypeDescriptor.cs
- ProcessHostConfigUtils.cs
- InstanceKeyCompleteException.cs
- CompositeTypefaceMetrics.cs
- AuthenticationServiceManager.cs
- WebPartsSection.cs
- ScrollEventArgs.cs
- TextRangeEditTables.cs
- TransactionFormatter.cs
- RichTextBoxDesigner.cs
- DataColumnMapping.cs
- WindowsProgressbar.cs
- SymLanguageType.cs
- TimeIntervalCollection.cs
- EditorPartCollection.cs
- XMLUtil.cs
- DPAPIProtectedConfigurationProvider.cs
- DeploymentSection.cs