Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / FontSizeConverter.cs / 1 / FontSizeConverter.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: FontSizeConverter.cs // // Description: Converter for FontSize to Double // // History: // 01/12/05 : GHermann - Ported from FontSize // //--------------------------------------------------------------------------- using System; using System.IO; using System.Diagnostics; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; // for ConstructorInfo using System.Globalization; // for CultureInfo using MS.Utility; // For ExceptionStringTable using System.Windows.Media; // for Typeface using System.Windows.Controls; // for ReadingPanel using System.Windows.Documents; using System.Windows.Markup; using MS.Internal; using MS.Internal.Text; using MS.Internal.Documents; namespace System.Windows { ////// TypeConverter to convert FontSize to/from other types. /// Currently: string, int, float, and double are supported. /// // Used by Parser to parse BoxUnits from markup. public class FontSizeConverter : TypeConverter { ////// TypeConverter method override. /// /// ITypeDescriptorContext /// Type to convert from ///true if conversion is possible public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { return (sourceType == typeof(string) || sourceType == typeof(int) || sourceType == typeof(float) || sourceType == typeof(double)); } ////// TypeConverter method override. /// /// ITypeDescriptorContext /// Type to convert to ///true if conversion is possible public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(InstanceDescriptor)) { return true; } return base.CanConvertTo(context, destinationType); } ////// TypeConverter method implementation. /// /// ITypeDescriptorContext /// Current culture (see CLR specs) /// value to convert from ///value that is result of conversion public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value == null) { throw GetConvertFromException(value); } // NB: The culture passed from Avalon parser should always be the invariant culture. // Do we care about usage in other circumstances? string text = value as string; if (text != null) { double amount; FromString(text, culture, out amount); return amount; } if (value is System.Int32 || value is System.Single || value is System.Double) { return (double)value; } // Can't convert, wrong type return null; } ////// TypeConverter method implementation. /// /// ITypeDescriptorContext /// current culture (see CLR specs) /// value to convert from /// Type to convert to ///converted value public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } double fs = (double)value; if (destinationType == typeof(string)) { return fs.ToString(culture); } if (destinationType == typeof(int)) { return (int)fs; } if (destinationType == typeof(float)) { return (float)fs; } if (destinationType == typeof(double)) { return fs; } return base.ConvertTo(context, culture, value, destinationType); } ////// Convert a string into an amount and a font size type that can be used to create /// and instance of a Fontsize, or to serialize in a more compact representation to /// a baml stream. /// internal static void FromString( string text, CultureInfo culture, out double amount) { amount = LengthConverter.FromString(text, culture); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: FontSizeConverter.cs // // Description: Converter for FontSize to Double // // History: // 01/12/05 : GHermann - Ported from FontSize // //--------------------------------------------------------------------------- using System; using System.IO; using System.Diagnostics; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; // for ConstructorInfo using System.Globalization; // for CultureInfo using MS.Utility; // For ExceptionStringTable using System.Windows.Media; // for Typeface using System.Windows.Controls; // for ReadingPanel using System.Windows.Documents; using System.Windows.Markup; using MS.Internal; using MS.Internal.Text; using MS.Internal.Documents; namespace System.Windows { ////// TypeConverter to convert FontSize to/from other types. /// Currently: string, int, float, and double are supported. /// // Used by Parser to parse BoxUnits from markup. public class FontSizeConverter : TypeConverter { ////// TypeConverter method override. /// /// ITypeDescriptorContext /// Type to convert from ///true if conversion is possible public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { return (sourceType == typeof(string) || sourceType == typeof(int) || sourceType == typeof(float) || sourceType == typeof(double)); } ////// TypeConverter method override. /// /// ITypeDescriptorContext /// Type to convert to ///true if conversion is possible public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(InstanceDescriptor)) { return true; } return base.CanConvertTo(context, destinationType); } ////// TypeConverter method implementation. /// /// ITypeDescriptorContext /// Current culture (see CLR specs) /// value to convert from ///value that is result of conversion public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value == null) { throw GetConvertFromException(value); } // NB: The culture passed from Avalon parser should always be the invariant culture. // Do we care about usage in other circumstances? string text = value as string; if (text != null) { double amount; FromString(text, culture, out amount); return amount; } if (value is System.Int32 || value is System.Single || value is System.Double) { return (double)value; } // Can't convert, wrong type return null; } ////// TypeConverter method implementation. /// /// ITypeDescriptorContext /// current culture (see CLR specs) /// value to convert from /// Type to convert to ///converted value public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } double fs = (double)value; if (destinationType == typeof(string)) { return fs.ToString(culture); } if (destinationType == typeof(int)) { return (int)fs; } if (destinationType == typeof(float)) { return (float)fs; } if (destinationType == typeof(double)) { return fs; } return base.ConvertTo(context, culture, value, destinationType); } ////// Convert a string into an amount and a font size type that can be used to create /// and instance of a Fontsize, or to serialize in a more compact representation to /// a baml stream. /// internal static void FromString( string text, CultureInfo culture, out double amount) { amount = LengthConverter.FromString(text, culture); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
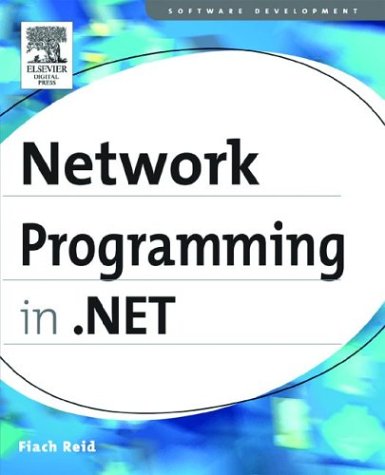
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlAnyAttributeAttribute.cs
- GetMemberBinder.cs
- UnsafeCollabNativeMethods.cs
- XmlLoader.cs
- XmlSchemaInferenceException.cs
- FontSizeConverter.cs
- HeaderUtility.cs
- StyleHelper.cs
- DeviceFiltersSection.cs
- DnsEndpointIdentity.cs
- OdbcConnectionPoolProviderInfo.cs
- WebPartDisplayModeCollection.cs
- PathGeometry.cs
- SecurityDocument.cs
- CompleteWizardStep.cs
- EntryPointNotFoundException.cs
- FlowDocumentScrollViewer.cs
- ServiceModelReg.cs
- SettingsProviderCollection.cs
- VersionedStream.cs
- HuffCodec.cs
- CallbackValidator.cs
- ButtonFlatAdapter.cs
- TimerElapsedEvenArgs.cs
- RequestQueue.cs
- FlowLayoutPanelDesigner.cs
- RepeatBehaviorConverter.cs
- ValidationError.cs
- NativeMethods.cs
- TrustSection.cs
- WSDualHttpSecurityElement.cs
- QilXmlWriter.cs
- VisualSerializer.cs
- StringAttributeCollection.cs
- SliderAutomationPeer.cs
- GroupItemAutomationPeer.cs
- ListenDesigner.cs
- FigureParaClient.cs
- SelectionItemProviderWrapper.cs
- CreateUserWizardStep.cs
- UIElement.cs
- AssemblyAssociatedContentFileAttribute.cs
- DataControlButton.cs
- DataBoundControl.cs
- TextBoxBase.cs
- StateDesigner.CommentLayoutGlyph.cs
- SafeNativeMemoryHandle.cs
- TimeoutValidationAttribute.cs
- DefaultEventAttribute.cs
- HeaderCollection.cs
- SqlMethodCallConverter.cs
- ContainerVisual.cs
- ProfileModule.cs
- SafeArrayRankMismatchException.cs
- InvokeBinder.cs
- ClipboardData.cs
- ApplicationHost.cs
- GeometryValueSerializer.cs
- SystemIPv4InterfaceProperties.cs
- WebBrowserPermission.cs
- RegexRunnerFactory.cs
- ToolStripPanel.cs
- RuntimeArgumentHandle.cs
- sqlser.cs
- CardSpaceSelector.cs
- StreamingContext.cs
- AudioFileOut.cs
- ErrorReporting.cs
- QueryStack.cs
- Mappings.cs
- TypeNameConverter.cs
- CryptoHandle.cs
- Query.cs
- ProcessHostConfigUtils.cs
- PropertyGridCommands.cs
- WsdlBuildProvider.cs
- IndexedSelectQueryOperator.cs
- AbsoluteQuery.cs
- PersistenceTypeAttribute.cs
- PlainXmlDeserializer.cs
- EmptyStringExpandableObjectConverter.cs
- RangeBase.cs
- EntitySetBase.cs
- ObjectNotFoundException.cs
- NullRuntimeConfig.cs
- Vector3dCollection.cs
- SqlMethodAttribute.cs
- Debug.cs
- Visual3D.cs
- XamlReaderHelper.cs
- Animatable.cs
- Comparer.cs
- AsyncOperationManager.cs
- BamlLocalizer.cs
- ToolStripProfessionalLowResolutionRenderer.cs
- ExceptionHandlersDesigner.cs
- BufferBuilder.cs
- ModelItemCollectionImpl.cs
- ProfileProvider.cs
- PropertyRef.cs