Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Documents / FixedSOMElement.cs / 1 / FixedSOMElement.cs
/*++ File: FixedSOMElement.cs Copyright (C) 2005 Microsoft Corporation. All rights reserved. Description: Abstract class that provides a common base class for all non-container semantic elements. These elements have a fixed node and start and end symbol indices associated with them. History: 05/17/2005: agurcan - Created --*/ namespace System.Windows.Documents { using System.Collections.Generic; using System.Diagnostics; using System.Windows.Media; using System.Windows.Media.Imaging; using System.Windows.Shapes; using System.Windows.Controls; internal abstract class FixedSOMElement : FixedSOMSemanticBox { //-------------------------------------------------------------------- // // Constructors // //--------------------------------------------------------------------- #region Constructors protected FixedSOMElement(FixedNode fixedNode, int startIndex, int endIndex, GeneralTransform transform) { _fixedNode = fixedNode; _startIndex = startIndex; _endIndex = endIndex; Transform trans = transform.AffineTransform; if (trans != null) { _mat = trans.Value; } else { _mat = Transform.Identity.Value; } } protected FixedSOMElement(FixedNode fixedNode, GeneralTransform transform) { _fixedNode = fixedNode; Transform trans = transform.AffineTransform; if (trans != null) { _mat = trans.Value; } else { _mat = Transform.Identity.Value; } } #endregion Constructors //------------------------------------------------------------------- // // Public Properties // //--------------------------------------------------------------------- #region Static methods public static FixedSOMElement CreateFixedSOMElement(FixedPage page, UIElement uiElement, FixedNode fixedNode, int startIndex, int endIndex) { FixedSOMElement element = null; if (uiElement is Glyphs) { Glyphs glyphs = uiElement as Glyphs; if (glyphs.UnicodeString.Length > 0) { GlyphRun glyphRun = glyphs.ToGlyphRun(); Rect alignmentBox = glyphRun.ComputeAlignmentBox(); alignmentBox.Offset(glyphs.OriginX, glyphs.OriginY); GeneralTransform transform = glyphs.TransformToAncestor(page); if (startIndex < 0) { startIndex = 0; } if (endIndex < 0) { endIndex = glyphRun.Characters == null ? 0 : glyphRun.Characters.Count; } element = FixedSOMTextRun.Create(alignmentBox, transform, glyphs, fixedNode, startIndex, endIndex, false); } } else if (uiElement is Image) { element = FixedSOMImage.Create(page, uiElement as Image, fixedNode); } else if (uiElement is Path) { element = FixedSOMImage.Create(page, uiElement as Path, fixedNode); } return element; } #endregion Static methods //-------------------------------------------------------------------- // // Public Properties // //--------------------------------------------------------------------- #region Public Properties public FixedNode FixedNode { get { return _fixedNode; } } public int StartIndex { get { return _startIndex; } } public int EndIndex { get { return _endIndex; } } #endregion Public Properties //-------------------------------------------------------------------- // // Internal Properties // //---------------------------------------------------------------------- #region Internal Properties internal FlowNode FlowNode { get { return _flowNode; } set { _flowNode = value; } } internal int OffsetInFlowNode { get { return _offsetInFlowNode; } set { _offsetInFlowNode = value; } } internal Matrix Matrix { get { return _mat; } } #endregion Internal Properties //------------------------------------------------------------------- // // Protected Fields // //---------------------------------------------------------------------- #region Protected Fields protected FixedNode _fixedNode ; protected int _startIndex; protected int _endIndex; protected Matrix _mat; #endregion Protected Fields #region Private Fields private FlowNode _flowNode; private int _offsetInFlowNode; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /*++ File: FixedSOMElement.cs Copyright (C) 2005 Microsoft Corporation. All rights reserved. Description: Abstract class that provides a common base class for all non-container semantic elements. These elements have a fixed node and start and end symbol indices associated with them. History: 05/17/2005: agurcan - Created --*/ namespace System.Windows.Documents { using System.Collections.Generic; using System.Diagnostics; using System.Windows.Media; using System.Windows.Media.Imaging; using System.Windows.Shapes; using System.Windows.Controls; internal abstract class FixedSOMElement : FixedSOMSemanticBox { //-------------------------------------------------------------------- // // Constructors // //--------------------------------------------------------------------- #region Constructors protected FixedSOMElement(FixedNode fixedNode, int startIndex, int endIndex, GeneralTransform transform) { _fixedNode = fixedNode; _startIndex = startIndex; _endIndex = endIndex; Transform trans = transform.AffineTransform; if (trans != null) { _mat = trans.Value; } else { _mat = Transform.Identity.Value; } } protected FixedSOMElement(FixedNode fixedNode, GeneralTransform transform) { _fixedNode = fixedNode; Transform trans = transform.AffineTransform; if (trans != null) { _mat = trans.Value; } else { _mat = Transform.Identity.Value; } } #endregion Constructors //------------------------------------------------------------------- // // Public Properties // //--------------------------------------------------------------------- #region Static methods public static FixedSOMElement CreateFixedSOMElement(FixedPage page, UIElement uiElement, FixedNode fixedNode, int startIndex, int endIndex) { FixedSOMElement element = null; if (uiElement is Glyphs) { Glyphs glyphs = uiElement as Glyphs; if (glyphs.UnicodeString.Length > 0) { GlyphRun glyphRun = glyphs.ToGlyphRun(); Rect alignmentBox = glyphRun.ComputeAlignmentBox(); alignmentBox.Offset(glyphs.OriginX, glyphs.OriginY); GeneralTransform transform = glyphs.TransformToAncestor(page); if (startIndex < 0) { startIndex = 0; } if (endIndex < 0) { endIndex = glyphRun.Characters == null ? 0 : glyphRun.Characters.Count; } element = FixedSOMTextRun.Create(alignmentBox, transform, glyphs, fixedNode, startIndex, endIndex, false); } } else if (uiElement is Image) { element = FixedSOMImage.Create(page, uiElement as Image, fixedNode); } else if (uiElement is Path) { element = FixedSOMImage.Create(page, uiElement as Path, fixedNode); } return element; } #endregion Static methods //-------------------------------------------------------------------- // // Public Properties // //--------------------------------------------------------------------- #region Public Properties public FixedNode FixedNode { get { return _fixedNode; } } public int StartIndex { get { return _startIndex; } } public int EndIndex { get { return _endIndex; } } #endregion Public Properties //-------------------------------------------------------------------- // // Internal Properties // //---------------------------------------------------------------------- #region Internal Properties internal FlowNode FlowNode { get { return _flowNode; } set { _flowNode = value; } } internal int OffsetInFlowNode { get { return _offsetInFlowNode; } set { _offsetInFlowNode = value; } } internal Matrix Matrix { get { return _mat; } } #endregion Internal Properties //------------------------------------------------------------------- // // Protected Fields // //---------------------------------------------------------------------- #region Protected Fields protected FixedNode _fixedNode ; protected int _startIndex; protected int _endIndex; protected Matrix _mat; #endregion Protected Fields #region Private Fields private FlowNode _flowNode; private int _offsetInFlowNode; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
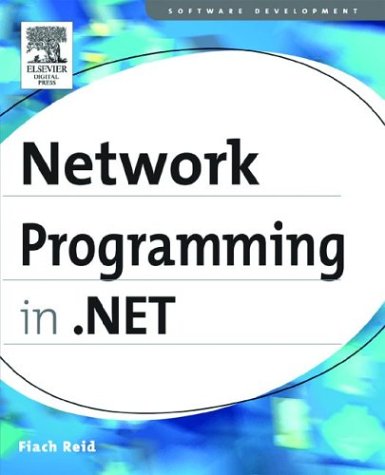
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PropertyDescriptors.cs
- ProbeMatchesApril2005.cs
- BrushMappingModeValidation.cs
- CryptoApi.cs
- XComponentModel.cs
- GenericIdentity.cs
- TreeNodeEventArgs.cs
- XmlDocumentFragment.cs
- ApplicationId.cs
- Validator.cs
- BeginStoryboard.cs
- HwndSourceKeyboardInputSite.cs
- ButtonFlatAdapter.cs
- TextFormatterContext.cs
- MimeParameters.cs
- Scripts.cs
- MsmqIntegrationProcessProtocolHandler.cs
- ExternalException.cs
- SamlAuthorizationDecisionStatement.cs
- OdbcCommand.cs
- ZoneButton.cs
- SnapLine.cs
- AsyncWaitHandle.cs
- ClientTargetSection.cs
- PointAnimationBase.cs
- TabPage.cs
- XmlTypeMapping.cs
- validation.cs
- OrderedDictionary.cs
- SerializerProvider.cs
- RtfToXamlLexer.cs
- ConnectivityStatus.cs
- StreamReader.cs
- DynamicRenderer.cs
- MethodBuilder.cs
- DrawingGroupDrawingContext.cs
- WsatProxy.cs
- OleDbParameterCollection.cs
- TdsEnums.cs
- InvalidOperationException.cs
- ConfigUtil.cs
- DataPagerCommandEventArgs.cs
- DBConnectionString.cs
- ReadOnlyHierarchicalDataSource.cs
- VectorConverter.cs
- ScopelessEnumAttribute.cs
- StreamResourceInfo.cs
- ToolstripProfessionalRenderer.cs
- ExtensionsSection.cs
- CommandBinding.cs
- TextRunTypographyProperties.cs
- WindowProviderWrapper.cs
- ExpressionBuilderContext.cs
- SelfIssuedAuthRSAPKCS1SignatureFormatter.cs
- ConnectorDragDropGlyph.cs
- MULTI_QI.cs
- GridViewSelectEventArgs.cs
- Registry.cs
- SequenceQuery.cs
- WorkflowInstanceExtensionCollection.cs
- TreeNodeStyle.cs
- Vector3DCollection.cs
- IResourceProvider.cs
- SocketElement.cs
- DesignerActionMethodItem.cs
- SoapAttributeAttribute.cs
- AccessibleObject.cs
- ThreadPool.cs
- processwaithandle.cs
- WebPartConnectVerb.cs
- BaseTemplatedMobileComponentEditor.cs
- ZipIORawDataFileBlock.cs
- CurrencyManager.cs
- MarkupWriter.cs
- Control.cs
- LockCookie.cs
- PackageRelationshipSelector.cs
- MLangCodePageEncoding.cs
- BooleanFunctions.cs
- AuthenticationManager.cs
- ProxyAssemblyNotLoadedException.cs
- CancelAsyncOperationRequest.cs
- XmlLanguageConverter.cs
- WmlPhoneCallAdapter.cs
- EntitySetDataBindingList.cs
- Application.cs
- CroppedBitmap.cs
- FreezableOperations.cs
- WsiProfilesElement.cs
- XmlSchemaInclude.cs
- Vector3DCollectionValueSerializer.cs
- StorageEntityContainerMapping.cs
- DLinqAssociationProvider.cs
- RNGCryptoServiceProvider.cs
- DropDownButton.cs
- TrustSection.cs
- SkewTransform.cs
- CategoryGridEntry.cs
- HybridObjectCache.cs
- XPathSelectionIterator.cs