Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / Effects / DropShadowBitmapEffect.cs / 3 / DropShadowBitmapEffect.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2005 // // File: BitmapEffectDropShadow.cs //----------------------------------------------------------------------------- #region Using directives using System; using System.Collections.Generic; using System.Text; using System.Runtime.InteropServices; using MS.Internal.PresentationCore; using System.Security; #endregion namespace System.Windows.Media.Effects { ////// The class definition for DropShadowBitmapEffect /// public partial class DropShadowBitmapEffect { ////// Constructor /// public DropShadowBitmapEffect() { } ////// Creates the unmanaged effect handle /// unsafe protected override SafeHandle CreateUnmanagedEffect() { return Create(new Guid(0x459a3fbe, 0xd8ac, 0x4692, 0x87, 0x4b, 0x7a, 0x26, 0x57, 0x15, 0xaa, 0x16)); } ////// /// Update (propagetes) properties to the unmanaged effect /// ////// This method demands permission because effects should not be run /// in partial trust. /// /// SecurityCritical - because SetValue has a link demand /// SecutiryTreatAsSafe - because it demans UIWindow permission /// [SecurityCritical, SecurityTreatAsSafe] protected override void UpdateUnmanagedPropertyState(SafeHandle unmanagedEffect) { SecurityHelper.DemandUIWindowPermission(); BitmapEffect.SetValue(unmanagedEffect, "Color", this.Color); BitmapEffect.SetValue(unmanagedEffect, "ShadowDepth", this.ShadowDepth); BitmapEffect.SetValue(unmanagedEffect, "Direction", this.Direction); BitmapEffect.SetValue(unmanagedEffect, "Noise", this.Noise); BitmapEffect.SetValue(unmanagedEffect, "Opacity", this.Opacity); BitmapEffect.SetValue(unmanagedEffect, "Softness", this.Softness); } ////// An ImageEffect can be used to emulate a DropShadowBitmapEffect with certain restrictions. This /// method returns true when it is possible to emulate the DropShadowBitmapEffect using an ImageEffect. /// internal override bool CanBeEmulatedUsingEffectPipeline() { return (Noise == 0.0); } ////// Returns a ImageEffect that emulates this DropShadowBitmapEffect. /// internal override Effect GetEmulatingEffect() { if (_imageEffectEmulation == null) { _imageEffectEmulation = new DropShadowEffect(); } if (_imageEffectEmulation.Color != Color) { _imageEffectEmulation.Color = Color; } // The limits on ShadowDepth preserve existing behavior. // A scale transform can scale the shadow depth to exceed 50.0, // and this behavior is also handled correctly in the unmanaged layer. if (_imageEffectEmulation.ShadowDepth != ShadowDepth) { if (ShadowDepth >= 50.0) { _imageEffectEmulation.ShadowDepth = 50.0; } else if (ShadowDepth < 0.0) { _imageEffectEmulation.ShadowDepth = 0.0; } else { _imageEffectEmulation.ShadowDepth = ShadowDepth; } } if (_imageEffectEmulation.Direction != Direction) { _imageEffectEmulation.Direction = Direction; } if (_imageEffectEmulation.Opacity != Opacity) { if (Opacity >= 1.0) { _imageEffectEmulation.Opacity = 1.0; } else if (Opacity <= 0.0) { _imageEffectEmulation.Opacity = 0.0; } else { _imageEffectEmulation.Opacity = Opacity; } } if (_imageEffectEmulation.BlurRadius / _MAX_EMULATED_BLUR_RADIUS != Softness) { if (Softness >= 1.0) { _imageEffectEmulation.BlurRadius = _MAX_EMULATED_BLUR_RADIUS; } else if (Softness <= 0.0) { _imageEffectEmulation.BlurRadius = 0.0; } else { _imageEffectEmulation.BlurRadius = _MAX_EMULATED_BLUR_RADIUS * Softness; } } _imageEffectEmulation.RenderingBias = RenderingBias.Performance; return _imageEffectEmulation; } DropShadowEffect _imageEffectEmulation; private const double _MAX_EMULATED_BLUR_RADIUS = 25.0; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2005 // // File: BitmapEffectDropShadow.cs //----------------------------------------------------------------------------- #region Using directives using System; using System.Collections.Generic; using System.Text; using System.Runtime.InteropServices; using MS.Internal.PresentationCore; using System.Security; #endregion namespace System.Windows.Media.Effects { ////// The class definition for DropShadowBitmapEffect /// public partial class DropShadowBitmapEffect { ////// Constructor /// public DropShadowBitmapEffect() { } ////// Creates the unmanaged effect handle /// unsafe protected override SafeHandle CreateUnmanagedEffect() { return Create(new Guid(0x459a3fbe, 0xd8ac, 0x4692, 0x87, 0x4b, 0x7a, 0x26, 0x57, 0x15, 0xaa, 0x16)); } ////// /// Update (propagetes) properties to the unmanaged effect /// ////// This method demands permission because effects should not be run /// in partial trust. /// /// SecurityCritical - because SetValue has a link demand /// SecutiryTreatAsSafe - because it demans UIWindow permission /// [SecurityCritical, SecurityTreatAsSafe] protected override void UpdateUnmanagedPropertyState(SafeHandle unmanagedEffect) { SecurityHelper.DemandUIWindowPermission(); BitmapEffect.SetValue(unmanagedEffect, "Color", this.Color); BitmapEffect.SetValue(unmanagedEffect, "ShadowDepth", this.ShadowDepth); BitmapEffect.SetValue(unmanagedEffect, "Direction", this.Direction); BitmapEffect.SetValue(unmanagedEffect, "Noise", this.Noise); BitmapEffect.SetValue(unmanagedEffect, "Opacity", this.Opacity); BitmapEffect.SetValue(unmanagedEffect, "Softness", this.Softness); } ////// An ImageEffect can be used to emulate a DropShadowBitmapEffect with certain restrictions. This /// method returns true when it is possible to emulate the DropShadowBitmapEffect using an ImageEffect. /// internal override bool CanBeEmulatedUsingEffectPipeline() { return (Noise == 0.0); } ////// Returns a ImageEffect that emulates this DropShadowBitmapEffect. /// internal override Effect GetEmulatingEffect() { if (_imageEffectEmulation == null) { _imageEffectEmulation = new DropShadowEffect(); } if (_imageEffectEmulation.Color != Color) { _imageEffectEmulation.Color = Color; } // The limits on ShadowDepth preserve existing behavior. // A scale transform can scale the shadow depth to exceed 50.0, // and this behavior is also handled correctly in the unmanaged layer. if (_imageEffectEmulation.ShadowDepth != ShadowDepth) { if (ShadowDepth >= 50.0) { _imageEffectEmulation.ShadowDepth = 50.0; } else if (ShadowDepth < 0.0) { _imageEffectEmulation.ShadowDepth = 0.0; } else { _imageEffectEmulation.ShadowDepth = ShadowDepth; } } if (_imageEffectEmulation.Direction != Direction) { _imageEffectEmulation.Direction = Direction; } if (_imageEffectEmulation.Opacity != Opacity) { if (Opacity >= 1.0) { _imageEffectEmulation.Opacity = 1.0; } else if (Opacity <= 0.0) { _imageEffectEmulation.Opacity = 0.0; } else { _imageEffectEmulation.Opacity = Opacity; } } if (_imageEffectEmulation.BlurRadius / _MAX_EMULATED_BLUR_RADIUS != Softness) { if (Softness >= 1.0) { _imageEffectEmulation.BlurRadius = _MAX_EMULATED_BLUR_RADIUS; } else if (Softness <= 0.0) { _imageEffectEmulation.BlurRadius = 0.0; } else { _imageEffectEmulation.BlurRadius = _MAX_EMULATED_BLUR_RADIUS * Softness; } } _imageEffectEmulation.RenderingBias = RenderingBias.Performance; return _imageEffectEmulation; } DropShadowEffect _imageEffectEmulation; private const double _MAX_EMULATED_BLUR_RADIUS = 25.0; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
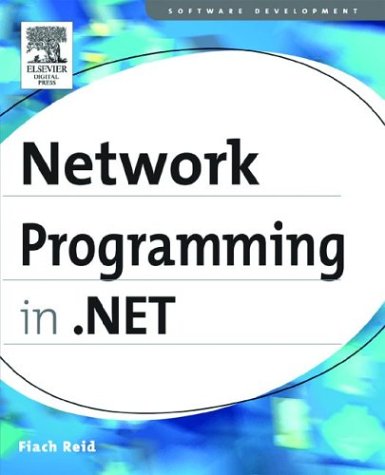
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CreateInstanceBinder.cs
- SqlDependencyUtils.cs
- DateTimeFormatInfoScanner.cs
- _AcceptOverlappedAsyncResult.cs
- UidManager.cs
- HasCopySemanticsAttribute.cs
- EdmType.cs
- PeerNeighborManager.cs
- RuleSettingsCollection.cs
- Padding.cs
- ModulesEntry.cs
- ImageMapEventArgs.cs
- ConfigurationSettings.cs
- Deflater.cs
- ControlEvent.cs
- ArrayList.cs
- XmlRawWriterWrapper.cs
- StreamWriter.cs
- TransformerInfo.cs
- EventDescriptor.cs
- Pair.cs
- xmlglyphRunInfo.cs
- InsufficientMemoryException.cs
- PointCollectionConverter.cs
- DBSqlParserColumn.cs
- OdbcRowUpdatingEvent.cs
- TextFragmentEngine.cs
- BitFlagsGenerator.cs
- SafeNativeMethods.cs
- ConnectionPoolManager.cs
- TransactionFlowElement.cs
- PerformanceCountersElement.cs
- PriorityRange.cs
- ITextView.cs
- SchemaAttDef.cs
- EpmAttributeNameBuilder.cs
- VideoDrawing.cs
- IPCCacheManager.cs
- ArrayHelper.cs
- DrawingVisualDrawingContext.cs
- AdRotator.cs
- PropertyValue.cs
- TextEncodedRawTextWriter.cs
- SqlParameter.cs
- Section.cs
- SiteMap.cs
- UriTemplateDispatchFormatter.cs
- ServiceModelEnumValidatorAttribute.cs
- SocketException.cs
- RIPEMD160Managed.cs
- WebControlParameterProxy.cs
- WeakEventTable.cs
- XXXInfos.cs
- SystemNetworkInterface.cs
- TagMapInfo.cs
- TypeConverterValueSerializer.cs
- ArgumentFixer.cs
- SecurityContextKeyIdentifierClause.cs
- XmlAttributeHolder.cs
- AsymmetricAlgorithm.cs
- SymbolType.cs
- EdmFunction.cs
- FastEncoderWindow.cs
- EditorResources.cs
- ContainerControlDesigner.cs
- CustomExpressionEventArgs.cs
- PerformanceCounterPermissionEntry.cs
- PropertyToken.cs
- Roles.cs
- ProviderConnectionPointCollection.cs
- DriveInfo.cs
- SqlFacetAttribute.cs
- TextStore.cs
- ToolboxDataAttribute.cs
- ProviderIncompatibleException.cs
- TableFieldsEditor.cs
- ApplicationId.cs
- EmbossBitmapEffect.cs
- Connector.cs
- ModelServiceImpl.cs
- DataKey.cs
- QilIterator.cs
- SqlDependencyUtils.cs
- DelayedRegex.cs
- StylusPointDescription.cs
- ListViewInsertionMark.cs
- TimelineClockCollection.cs
- Convert.cs
- DynamicUpdateCommand.cs
- PrimaryKeyTypeConverter.cs
- EncryptedReference.cs
- DataSourceHelper.cs
- ZipIOCentralDirectoryFileHeader.cs
- PenContexts.cs
- COM2TypeInfoProcessor.cs
- AssemblyInfo.cs
- PenCursorManager.cs
- TextSelectionHelper.cs
- WrapPanel.cs
- Animatable.cs