Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / Animation / Generated / ObjectKeyFrameCollection.cs / 1 / ObjectKeyFrameCollection.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Windows.Media.Animation; using System.Windows.Media.Media3D; namespace System.Windows.Media.Animation { ////// This collection is used in conjunction with a KeyFrameObjectAnimation /// to animate a Object property value along a set of key frames. /// public class ObjectKeyFrameCollection : Freezable, IList { #region Data private List_keyFrames; private static ObjectKeyFrameCollection s_emptyCollection; #endregion #region Constructors /// /// Creates a new ObjectKeyFrameCollection. /// public ObjectKeyFrameCollection() : base() { _keyFrames = new List< ObjectKeyFrame>(2); } #endregion #region Static Methods ////// An empty ObjectKeyFrameCollection. /// public static ObjectKeyFrameCollection Empty { get { if (s_emptyCollection == null) { ObjectKeyFrameCollection emptyCollection = new ObjectKeyFrameCollection(); emptyCollection._keyFrames = new List< ObjectKeyFrame>(0); emptyCollection.Freeze(); s_emptyCollection = emptyCollection; } return s_emptyCollection; } } #endregion #region Freezable ////// Creates a freezable copy of this ObjectKeyFrameCollection. /// ///The copy public new ObjectKeyFrameCollection Clone() { return (ObjectKeyFrameCollection)base.Clone(); } ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new ObjectKeyFrameCollection(); } ////// Implementation of protected override void CloneCore(Freezable sourceFreezable) { ObjectKeyFrameCollection sourceCollection = (ObjectKeyFrameCollection) sourceFreezable; base.CloneCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< ObjectKeyFrame>(count); for (int i = 0; i < count; i++) { ObjectKeyFrame keyFrame = (ObjectKeyFrame)sourceCollection._keyFrames[i].Clone(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.CloneCore . ////// Implementation of protected override void CloneCurrentValueCore(Freezable sourceFreezable) { ObjectKeyFrameCollection sourceCollection = (ObjectKeyFrameCollection) sourceFreezable; base.CloneCurrentValueCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< ObjectKeyFrame>(count); for (int i = 0; i < count; i++) { ObjectKeyFrame keyFrame = (ObjectKeyFrame)sourceCollection._keyFrames[i].CloneCurrentValue(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.CloneCurrentValueCore . ////// Implementation of protected override void GetAsFrozenCore(Freezable sourceFreezable) { ObjectKeyFrameCollection sourceCollection = (ObjectKeyFrameCollection) sourceFreezable; base.GetAsFrozenCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< ObjectKeyFrame>(count); for (int i = 0; i < count; i++) { ObjectKeyFrame keyFrame = (ObjectKeyFrame)sourceCollection._keyFrames[i].GetAsFrozen(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.GetAsFrozenCore . ////// Implementation of protected override void GetCurrentValueAsFrozenCore(Freezable sourceFreezable) { ObjectKeyFrameCollection sourceCollection = (ObjectKeyFrameCollection) sourceFreezable; base.GetCurrentValueAsFrozenCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< ObjectKeyFrame>(count); for (int i = 0; i < count; i++) { ObjectKeyFrame keyFrame = (ObjectKeyFrame)sourceCollection._keyFrames[i].GetCurrentValueAsFrozen(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.GetCurrentValueAsFrozenCore . ////// /// protected override bool FreezeCore(bool isChecking) { bool canFreeze = base.FreezeCore(isChecking); for (int i = 0; i < _keyFrames.Count && canFreeze; i++) { canFreeze &= Freezable.Freeze(_keyFrames[i], isChecking); } return canFreeze; } #endregion #region IEnumerable ////// Returns an enumerator of the ObjectKeyFrames in the collection. /// public IEnumerator GetEnumerator() { ReadPreamble(); return _keyFrames.GetEnumerator(); } #endregion #region ICollection ////// Returns the number of ObjectKeyFrames in the collection. /// public int Count { get { ReadPreamble(); return _keyFrames.Count; } } ////// See public bool IsSynchronized { get { ReadPreamble(); return (IsFrozen || Dispatcher != null); } } ///ICollection.IsSynchronized . ////// See public object SyncRoot { get { ReadPreamble(); return ((ICollection)_keyFrames).SyncRoot; } } ///ICollection.SyncRoot . ////// Copies all of the ObjectKeyFrames in the collection to an /// array. /// void ICollection.CopyTo(Array array, int index) { ReadPreamble(); ((ICollection)_keyFrames).CopyTo(array, index); } ////// Copies all of the ObjectKeyFrames in the collection to an /// array of ObjectKeyFrames. /// public void CopyTo(ObjectKeyFrame[] array, int index) { ReadPreamble(); _keyFrames.CopyTo(array, index); } #endregion #region IList ////// Adds a ObjectKeyFrame to the collection. /// int IList.Add(object keyFrame) { return Add((ObjectKeyFrame)keyFrame); } ////// Adds a ObjectKeyFrame to the collection. /// public int Add(ObjectKeyFrame keyFrame) { if (keyFrame == null) { throw new ArgumentNullException("keyFrame"); } WritePreamble(); OnFreezablePropertyChanged(null, keyFrame); _keyFrames.Add(keyFrame); WritePostscript(); return _keyFrames.Count - 1; } ////// Removes all ObjectKeyFrames from the collection. /// public void Clear() { WritePreamble(); if (_keyFrames.Count > 0) { for (int i = 0; i < _keyFrames.Count; i++) { OnFreezablePropertyChanged(_keyFrames[i], null); } _keyFrames.Clear(); WritePostscript(); } } ////// Returns true of the collection contains the given ObjectKeyFrame. /// bool IList.Contains(object keyFrame) { return Contains((ObjectKeyFrame)keyFrame); } ////// Returns true of the collection contains the given ObjectKeyFrame. /// public bool Contains(ObjectKeyFrame keyFrame) { ReadPreamble(); return _keyFrames.Contains(keyFrame); } ////// Returns the index of a given ObjectKeyFrame in the collection. /// int IList.IndexOf(object keyFrame) { return IndexOf((ObjectKeyFrame)keyFrame); } ////// Returns the index of a given ObjectKeyFrame in the collection. /// public int IndexOf(ObjectKeyFrame keyFrame) { ReadPreamble(); return _keyFrames.IndexOf(keyFrame); } ////// Inserts a ObjectKeyFrame into a specific location in the collection. /// void IList.Insert(int index, object keyFrame) { Insert(index, (ObjectKeyFrame)keyFrame); } ////// Inserts a ObjectKeyFrame into a specific location in the collection. /// public void Insert(int index, ObjectKeyFrame keyFrame) { if (keyFrame == null) { throw new ArgumentNullException("keyFrame"); } WritePreamble(); OnFreezablePropertyChanged(null, keyFrame); _keyFrames.Insert(index, keyFrame); WritePostscript(); } ////// Returns true if the collection is frozen. /// public bool IsFixedSize { get { ReadPreamble(); return IsFrozen; } } ////// Returns true if the collection is frozen. /// public bool IsReadOnly { get { ReadPreamble(); return IsFrozen; } } ////// Removes a ObjectKeyFrame from the collection. /// void IList.Remove(object keyFrame) { Remove((ObjectKeyFrame)keyFrame); } ////// Removes a ObjectKeyFrame from the collection. /// public void Remove(ObjectKeyFrame keyFrame) { WritePreamble(); if (_keyFrames.Contains(keyFrame)) { OnFreezablePropertyChanged(keyFrame, null); _keyFrames.Remove(keyFrame); WritePostscript(); } } ////// Removes the ObjectKeyFrame at the specified index from the collection. /// public void RemoveAt(int index) { WritePreamble(); OnFreezablePropertyChanged(_keyFrames[index], null); _keyFrames.RemoveAt(index); WritePostscript(); } ////// Gets or sets the ObjectKeyFrame at a given index. /// object IList.this[int index] { get { return this[index]; } set { this[index] = (ObjectKeyFrame)value; } } ////// Gets or sets the ObjectKeyFrame at a given index. /// public ObjectKeyFrame this[int index] { get { ReadPreamble(); return _keyFrames[index]; } set { if (value == null) { throw new ArgumentNullException(String.Format(CultureInfo.InvariantCulture, "ObjectKeyFrameCollection[{0}]", index)); } WritePreamble(); if (value != _keyFrames[index]) { OnFreezablePropertyChanged(_keyFrames[index], value); _keyFrames[index] = value; Debug.Assert(_keyFrames[index] != null); WritePostscript(); } } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Windows.Media.Animation; using System.Windows.Media.Media3D; namespace System.Windows.Media.Animation { ////// This collection is used in conjunction with a KeyFrameObjectAnimation /// to animate a Object property value along a set of key frames. /// public class ObjectKeyFrameCollection : Freezable, IList { #region Data private List_keyFrames; private static ObjectKeyFrameCollection s_emptyCollection; #endregion #region Constructors /// /// Creates a new ObjectKeyFrameCollection. /// public ObjectKeyFrameCollection() : base() { _keyFrames = new List< ObjectKeyFrame>(2); } #endregion #region Static Methods ////// An empty ObjectKeyFrameCollection. /// public static ObjectKeyFrameCollection Empty { get { if (s_emptyCollection == null) { ObjectKeyFrameCollection emptyCollection = new ObjectKeyFrameCollection(); emptyCollection._keyFrames = new List< ObjectKeyFrame>(0); emptyCollection.Freeze(); s_emptyCollection = emptyCollection; } return s_emptyCollection; } } #endregion #region Freezable ////// Creates a freezable copy of this ObjectKeyFrameCollection. /// ///The copy public new ObjectKeyFrameCollection Clone() { return (ObjectKeyFrameCollection)base.Clone(); } ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new ObjectKeyFrameCollection(); } ////// Implementation of protected override void CloneCore(Freezable sourceFreezable) { ObjectKeyFrameCollection sourceCollection = (ObjectKeyFrameCollection) sourceFreezable; base.CloneCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< ObjectKeyFrame>(count); for (int i = 0; i < count; i++) { ObjectKeyFrame keyFrame = (ObjectKeyFrame)sourceCollection._keyFrames[i].Clone(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.CloneCore . ////// Implementation of protected override void CloneCurrentValueCore(Freezable sourceFreezable) { ObjectKeyFrameCollection sourceCollection = (ObjectKeyFrameCollection) sourceFreezable; base.CloneCurrentValueCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< ObjectKeyFrame>(count); for (int i = 0; i < count; i++) { ObjectKeyFrame keyFrame = (ObjectKeyFrame)sourceCollection._keyFrames[i].CloneCurrentValue(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.CloneCurrentValueCore . ////// Implementation of protected override void GetAsFrozenCore(Freezable sourceFreezable) { ObjectKeyFrameCollection sourceCollection = (ObjectKeyFrameCollection) sourceFreezable; base.GetAsFrozenCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< ObjectKeyFrame>(count); for (int i = 0; i < count; i++) { ObjectKeyFrame keyFrame = (ObjectKeyFrame)sourceCollection._keyFrames[i].GetAsFrozen(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.GetAsFrozenCore . ////// Implementation of protected override void GetCurrentValueAsFrozenCore(Freezable sourceFreezable) { ObjectKeyFrameCollection sourceCollection = (ObjectKeyFrameCollection) sourceFreezable; base.GetCurrentValueAsFrozenCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< ObjectKeyFrame>(count); for (int i = 0; i < count; i++) { ObjectKeyFrame keyFrame = (ObjectKeyFrame)sourceCollection._keyFrames[i].GetCurrentValueAsFrozen(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.GetCurrentValueAsFrozenCore . ////// /// protected override bool FreezeCore(bool isChecking) { bool canFreeze = base.FreezeCore(isChecking); for (int i = 0; i < _keyFrames.Count && canFreeze; i++) { canFreeze &= Freezable.Freeze(_keyFrames[i], isChecking); } return canFreeze; } #endregion #region IEnumerable ////// Returns an enumerator of the ObjectKeyFrames in the collection. /// public IEnumerator GetEnumerator() { ReadPreamble(); return _keyFrames.GetEnumerator(); } #endregion #region ICollection ////// Returns the number of ObjectKeyFrames in the collection. /// public int Count { get { ReadPreamble(); return _keyFrames.Count; } } ////// See public bool IsSynchronized { get { ReadPreamble(); return (IsFrozen || Dispatcher != null); } } ///ICollection.IsSynchronized . ////// See public object SyncRoot { get { ReadPreamble(); return ((ICollection)_keyFrames).SyncRoot; } } ///ICollection.SyncRoot . ////// Copies all of the ObjectKeyFrames in the collection to an /// array. /// void ICollection.CopyTo(Array array, int index) { ReadPreamble(); ((ICollection)_keyFrames).CopyTo(array, index); } ////// Copies all of the ObjectKeyFrames in the collection to an /// array of ObjectKeyFrames. /// public void CopyTo(ObjectKeyFrame[] array, int index) { ReadPreamble(); _keyFrames.CopyTo(array, index); } #endregion #region IList ////// Adds a ObjectKeyFrame to the collection. /// int IList.Add(object keyFrame) { return Add((ObjectKeyFrame)keyFrame); } ////// Adds a ObjectKeyFrame to the collection. /// public int Add(ObjectKeyFrame keyFrame) { if (keyFrame == null) { throw new ArgumentNullException("keyFrame"); } WritePreamble(); OnFreezablePropertyChanged(null, keyFrame); _keyFrames.Add(keyFrame); WritePostscript(); return _keyFrames.Count - 1; } ////// Removes all ObjectKeyFrames from the collection. /// public void Clear() { WritePreamble(); if (_keyFrames.Count > 0) { for (int i = 0; i < _keyFrames.Count; i++) { OnFreezablePropertyChanged(_keyFrames[i], null); } _keyFrames.Clear(); WritePostscript(); } } ////// Returns true of the collection contains the given ObjectKeyFrame. /// bool IList.Contains(object keyFrame) { return Contains((ObjectKeyFrame)keyFrame); } ////// Returns true of the collection contains the given ObjectKeyFrame. /// public bool Contains(ObjectKeyFrame keyFrame) { ReadPreamble(); return _keyFrames.Contains(keyFrame); } ////// Returns the index of a given ObjectKeyFrame in the collection. /// int IList.IndexOf(object keyFrame) { return IndexOf((ObjectKeyFrame)keyFrame); } ////// Returns the index of a given ObjectKeyFrame in the collection. /// public int IndexOf(ObjectKeyFrame keyFrame) { ReadPreamble(); return _keyFrames.IndexOf(keyFrame); } ////// Inserts a ObjectKeyFrame into a specific location in the collection. /// void IList.Insert(int index, object keyFrame) { Insert(index, (ObjectKeyFrame)keyFrame); } ////// Inserts a ObjectKeyFrame into a specific location in the collection. /// public void Insert(int index, ObjectKeyFrame keyFrame) { if (keyFrame == null) { throw new ArgumentNullException("keyFrame"); } WritePreamble(); OnFreezablePropertyChanged(null, keyFrame); _keyFrames.Insert(index, keyFrame); WritePostscript(); } ////// Returns true if the collection is frozen. /// public bool IsFixedSize { get { ReadPreamble(); return IsFrozen; } } ////// Returns true if the collection is frozen. /// public bool IsReadOnly { get { ReadPreamble(); return IsFrozen; } } ////// Removes a ObjectKeyFrame from the collection. /// void IList.Remove(object keyFrame) { Remove((ObjectKeyFrame)keyFrame); } ////// Removes a ObjectKeyFrame from the collection. /// public void Remove(ObjectKeyFrame keyFrame) { WritePreamble(); if (_keyFrames.Contains(keyFrame)) { OnFreezablePropertyChanged(keyFrame, null); _keyFrames.Remove(keyFrame); WritePostscript(); } } ////// Removes the ObjectKeyFrame at the specified index from the collection. /// public void RemoveAt(int index) { WritePreamble(); OnFreezablePropertyChanged(_keyFrames[index], null); _keyFrames.RemoveAt(index); WritePostscript(); } ////// Gets or sets the ObjectKeyFrame at a given index. /// object IList.this[int index] { get { return this[index]; } set { this[index] = (ObjectKeyFrame)value; } } ////// Gets or sets the ObjectKeyFrame at a given index. /// public ObjectKeyFrame this[int index] { get { ReadPreamble(); return _keyFrames[index]; } set { if (value == null) { throw new ArgumentNullException(String.Format(CultureInfo.InvariantCulture, "ObjectKeyFrameCollection[{0}]", index)); } WritePreamble(); if (value != _keyFrames[index]) { OnFreezablePropertyChanged(_keyFrames[index], value); _keyFrames[index] = value; Debug.Assert(_keyFrames[index] != null); WritePostscript(); } } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
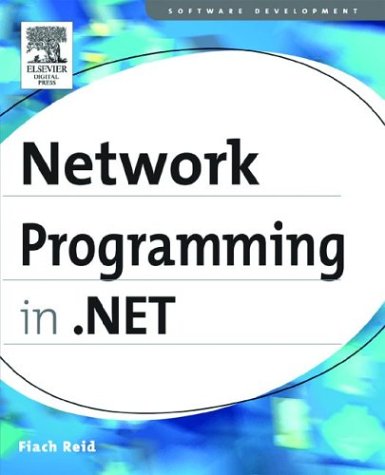
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IpcServerChannel.cs
- LinkClickEvent.cs
- LocalizableResourceBuilder.cs
- RowUpdatedEventArgs.cs
- Configuration.cs
- FrameworkRichTextComposition.cs
- DataTablePropertyDescriptor.cs
- XmlCodeExporter.cs
- Globals.cs
- XLinq.cs
- RelationshipNavigation.cs
- SystemNetworkInterface.cs
- Pen.cs
- HtmlTextArea.cs
- XomlSerializationHelpers.cs
- SingleResultAttribute.cs
- WebPartEditVerb.cs
- DocumentEventArgs.cs
- TimeSpanOrInfiniteConverter.cs
- InertiaTranslationBehavior.cs
- DetailsView.cs
- HWStack.cs
- FontFamily.cs
- XmlValidatingReaderImpl.cs
- TargetConverter.cs
- HttpHandler.cs
- SelectionRangeConverter.cs
- XmlValueConverter.cs
- mda.cs
- ZipIOLocalFileDataDescriptor.cs
- ResolvedKeyFrameEntry.cs
- MaskedTextProvider.cs
- FilePrompt.cs
- RegexGroupCollection.cs
- HtmlElementErrorEventArgs.cs
- ModelItemDictionaryImpl.cs
- DataExpression.cs
- DataSourceGeneratorException.cs
- CapabilitiesUse.cs
- ThreadStaticAttribute.cs
- RoutedEventHandlerInfo.cs
- VSWCFServiceContractGenerator.cs
- DesignOnlyAttribute.cs
- ConnectionInterfaceCollection.cs
- HasCopySemanticsAttribute.cs
- Misc.cs
- UniqueEventHelper.cs
- MemberRelationshipService.cs
- WebPartsPersonalization.cs
- SlotInfo.cs
- HtmlShim.cs
- BinaryMessageEncodingBindingElement.cs
- EncryptedData.cs
- DbTransaction.cs
- Int64Converter.cs
- QueueAccessMode.cs
- ResourceDictionary.cs
- TransactionScopeDesigner.cs
- MsmqIntegrationMessageProperty.cs
- XamlSerializerUtil.cs
- AlphabeticalEnumConverter.cs
- PeerInvitationResponse.cs
- SBCSCodePageEncoding.cs
- CodeChecksumPragma.cs
- ExceptionWrapper.cs
- ModuleBuilderData.cs
- RoutingConfiguration.cs
- errorpatternmatcher.cs
- ParamArrayAttribute.cs
- TransactionChannelListener.cs
- HttpCapabilitiesEvaluator.cs
- FusionWrap.cs
- FileSystemWatcher.cs
- QueryContinueDragEvent.cs
- HyperLinkField.cs
- JsonDeserializer.cs
- ActiveXContainer.cs
- XmlResolver.cs
- CharConverter.cs
- HtmlTableRowCollection.cs
- XmlEncoding.cs
- ListViewItemSelectionChangedEvent.cs
- ControlParameter.cs
- StreamReader.cs
- HideDisabledControlAdapter.cs
- ZipIOBlockManager.cs
- StorageEntityContainerMapping.cs
- _SafeNetHandles.cs
- SpotLight.cs
- ViewBase.cs
- TextCollapsingProperties.cs
- SeparatorAutomationPeer.cs
- Dictionary.cs
- Propagator.Evaluator.cs
- DataGrid.cs
- _ConnectStream.cs
- translator.cs
- METAHEADER.cs
- ThemeInfoAttribute.cs
- TemplatedAdorner.cs