Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / fx / src / Xml / System / Xml / XmlQualifiedName.cs / 2 / XmlQualifiedName.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml { using System.Collections; using System.Diagnostics; ////// /// [Serializable] public class XmlQualifiedName { string name; string ns; [NonSerialized] Int32 hash; ///[To be supplied.] ////// /// public static readonly XmlQualifiedName Empty = new XmlQualifiedName(string.Empty); ///[To be supplied.] ////// /// public XmlQualifiedName() : this(string.Empty, string.Empty) {} ///[To be supplied.] ////// /// public XmlQualifiedName(string name) : this(name, string.Empty) {} ///[To be supplied.] ////// /// public XmlQualifiedName(string name, string ns) { this.ns = ns == null ? string.Empty : ns; this.name = name == null ? string.Empty : name; } ///[To be supplied.] ////// /// public string Namespace { get { return ns; } } ///[To be supplied.] ////// /// public string Name { get { return name; } } ///[To be supplied.] ////// /// public override int GetHashCode() { if(hash == 0) { hash = Name.GetHashCode() /*+ Namespace.GetHashCode()*/; // for perf reasons we are not taking ns's hashcode. } return hash; } ///[To be supplied.] ////// /// public bool IsEmpty { get { return Name.Length == 0 && Namespace.Length == 0; } } ///[To be supplied.] ////// /// public override string ToString() { return Namespace.Length == 0 ? Name : string.Concat(Namespace, ":" , Name); } ///[To be supplied.] ////// /// public override bool Equals(object other) { XmlQualifiedName qname; if ((object) this == other) { return true; } qname = other as XmlQualifiedName; if (qname != null) { return (Name == qname.Name && Namespace == qname.Namespace); } return false; } ///[To be supplied.] ////// /// public static bool operator ==(XmlQualifiedName a, XmlQualifiedName b) { if ((object) a == (object) b) return true; if ((object) a == null || (object) b == null) return false; return (a.Name == b.Name && a.Namespace == b.Namespace); } ///[To be supplied.] ////// /// public static bool operator !=(XmlQualifiedName a, XmlQualifiedName b) { return !(a == b); } ///[To be supplied.] ////// /// public static string ToString(string name, string ns) { return ns == null || ns.Length == 0 ? name : ns + ":" + name; } // --------- Some useful internal stuff ----------------- internal void Init(string name, string ns) { Debug.Assert(name != null && ns != null); this.name = name; this.ns = ns; this.hash = 0; } internal void SetNamespace(string ns) { Debug.Assert(ns != null); this.ns = ns; //Not changing hash since ns is not used to compute hashcode } internal void Verify() { XmlConvert.VerifyNCName(name); if (ns.Length != 0) { XmlConvert.ToUri(ns); } } internal void Atomize(XmlNameTable nameTable) { Debug.Assert(name != null); name = nameTable.Add(name); ns = nameTable.Add(ns); } internal static XmlQualifiedName Parse(string s, IXmlNamespaceResolver nsmgr, out string prefix) { string localName; ValidateNames.ParseQNameThrow(s, out prefix, out localName); string uri = nsmgr.LookupNamespace(prefix); if (uri == null) { if (prefix.Length != 0) { throw new XmlException(Res.Xml_UnknownNs, prefix); } else { //Re-map namespace of empty prefix to string.Empty when there is no default namespace declared uri = string.Empty; } } return new XmlQualifiedName(localName, uri); } internal XmlQualifiedName Clone() { return (XmlQualifiedName)MemberwiseClone(); } internal static int Compare(XmlQualifiedName a, XmlQualifiedName b) { if (null == a) { return (null == b) ? 0 : -1; } if (null == b) { return 1; } int i = String.CompareOrdinal(a.Namespace, b.Namespace); if (i == 0) { i = String.CompareOrdinal(a.Name, b.Name); } return i; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.[To be supplied.] ///
Link Menu
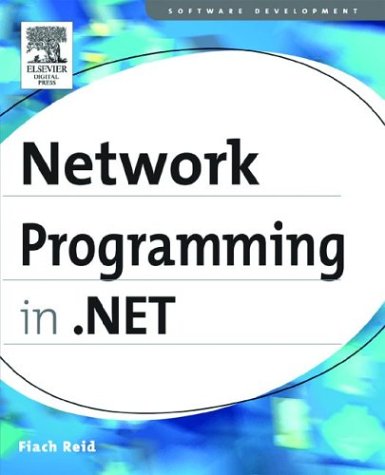
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Manipulation.cs
- FilterException.cs
- MimePart.cs
- RectKeyFrameCollection.cs
- querybuilder.cs
- AutoGeneratedField.cs
- Rectangle.cs
- UpWmlMobileTextWriter.cs
- SHA1CryptoServiceProvider.cs
- SaveFileDialog.cs
- XamlClipboardData.cs
- Attributes.cs
- ParallelLoopState.cs
- WebBrowserProgressChangedEventHandler.cs
- ListBindingConverter.cs
- DragEvent.cs
- XPathException.cs
- ValueChangedEventManager.cs
- CompilerWrapper.cs
- PointConverter.cs
- AssemblyAttributes.cs
- SmtpReplyReaderFactory.cs
- CommandID.cs
- ParameterBuilder.cs
- QuaternionRotation3D.cs
- SafeLocalAllocation.cs
- DeflateStream.cs
- NumberFunctions.cs
- TdsParameterSetter.cs
- NativeRecognizer.cs
- SqlDataReaderSmi.cs
- ZipIOExtraFieldPaddingElement.cs
- AssertSection.cs
- EmptyWithCancelationCheckWorkItem.cs
- AdCreatedEventArgs.cs
- StylusPlugin.cs
- Vector3DConverter.cs
- LingerOption.cs
- HttpSessionStateWrapper.cs
- TCEAdapterGenerator.cs
- OleDbConnectionPoolGroupProviderInfo.cs
- FileVersionInfo.cs
- TextBreakpoint.cs
- XPathExpr.cs
- ListViewItemSelectionChangedEvent.cs
- LocatorPart.cs
- CompiledQueryCacheEntry.cs
- LiteralText.cs
- TextContainer.cs
- X509SecurityTokenProvider.cs
- RelationshipManager.cs
- ObjectItemCachedAssemblyLoader.cs
- _HeaderInfoTable.cs
- InstanceLockedException.cs
- XmlSchemaAttributeGroup.cs
- WindowsEditBox.cs
- XmlIlTypeHelper.cs
- Ppl.cs
- ListSourceHelper.cs
- NamespaceImport.cs
- XmlSchemaType.cs
- AttachedPropertyBrowsableForTypeAttribute.cs
- SafeSystemMetrics.cs
- JsonQNameDataContract.cs
- SqlGatherProducedAliases.cs
- UnsafeNativeMethods.cs
- CollectionChange.cs
- XmlSerializer.cs
- CounterCreationDataCollection.cs
- HwndMouseInputProvider.cs
- EventRecord.cs
- ProgressBarRenderer.cs
- WizardStepBase.cs
- PointCollectionValueSerializer.cs
- DataGridViewSelectedCellsAccessibleObject.cs
- CompoundFileReference.cs
- XmlSchemaImport.cs
- Visitor.cs
- ExpressionPrefixAttribute.cs
- DataGridCellItemAutomationPeer.cs
- XmlBindingWorker.cs
- BeginEvent.cs
- typedescriptorpermissionattribute.cs
- SetStateEventArgs.cs
- ClientBase.cs
- SafeNativeMethodsOther.cs
- SetStateEventArgs.cs
- QilReplaceVisitor.cs
- Compensation.cs
- OdbcReferenceCollection.cs
- TemplateParser.cs
- CqlIdentifiers.cs
- ListControl.cs
- Dictionary.cs
- CodeCatchClauseCollection.cs
- ToolTip.cs
- NestPullup.cs
- StatusStrip.cs
- DataObject.cs
- BigInt.cs