Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / UI / WebParts / PersonalizationProvider.cs / 1 / PersonalizationProvider.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls.WebParts { using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Configuration; using System.Configuration.Provider; using System.Security.Permissions; using System.Security.Principal; using System.Web; using System.Web.Configuration; using System.Web.Hosting; using System.Web.Util; ////// The provider used to access the personalization store for WebPart pages. /// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public abstract class PersonalizationProvider : ProviderBase { private const string scopeFieldName = "__WPPS"; private const string sharedScopeFieldValue = "s"; private const string userScopeFieldValue = "u"; private ICollection _supportedUserCapabilities; ////// Initializes an instance of PersonalizationProvider. /// protected PersonalizationProvider() { } ////// The name of the application that this provider should use to store /// and retrieve personalization data from. /// public abstract string ApplicationName { get; set; } ////// protected virtual IList CreateSupportedUserCapabilities() { ArrayList list = new ArrayList(); list.Add(WebPartPersonalization.EnterSharedScopeUserCapability); list.Add(WebPartPersonalization.ModifyStateUserCapability); return list; } ////// public virtual PersonalizationScope DetermineInitialScope(WebPartManager webPartManager, PersonalizationState loadedState) { if (webPartManager == null) { throw new ArgumentNullException("webPartManager"); } Page page = webPartManager.Page; if (page == null) { throw new ArgumentException(SR.GetString(SR.PropertyCannotBeNull, "Page"), "webPartManager"); } HttpRequest request = page.RequestInternal; if (request == null) { throw new ArgumentException(SR.GetString(SR.PropertyCannotBeNull, "Page.Request"), "webPartManager"); } PersonalizationScope scope = webPartManager.Personalization.InitialScope; IPrincipal user = null; if (request.IsAuthenticated) { user = page.User; } if (user == null) { // if no user has been authenticated, then just load all user data scope = PersonalizationScope.Shared; } else { if (page.IsPostBack) { string postedMode = page.Request[scopeFieldName]; if (postedMode == sharedScopeFieldValue) { scope = PersonalizationScope.Shared; } else if (postedMode == userScopeFieldValue) { scope = PersonalizationScope.User; } } else if ((page.PreviousPage != null) && (page.PreviousPage.IsCrossPagePostBack == false)) { WebPartManager previousWebPartManager = WebPartManager.GetCurrentWebPartManager(page.PreviousPage); if (previousWebPartManager != null) { // Note that we check the types of the page, so we don't // look the at the PreviousPage in a cross-page posting scenario scope = previousWebPartManager.Personalization.Scope; } } // Special-case Web Part Export so it executes in the same security context as the page itself (VSWhidbey 426574) // Setting the initial scope from what's been asked for in the export parameters else if (page.IsExportingWebPart) { scope = (page.IsExportingWebPartShared ? PersonalizationScope.Shared : PersonalizationScope.User); } if ((scope == PersonalizationScope.Shared) && (webPartManager.Personalization.CanEnterSharedScope == false)) { scope = PersonalizationScope.User; } } string fieldValue = (scope == PersonalizationScope.Shared) ? sharedScopeFieldValue : userScopeFieldValue; page.ClientScript.RegisterHiddenField(scopeFieldName, fieldValue); return scope; } ////// public virtual IDictionary DetermineUserCapabilities(WebPartManager webPartManager) { if (webPartManager == null) { throw new ArgumentNullException("webPartManager"); } Page page = webPartManager.Page; if (page == null) { throw new ArgumentException(SR.GetString(SR.PropertyCannotBeNull, "Page"), "webPartManager"); } HttpRequest request = page.RequestInternal; if (request == null) { throw new ArgumentException(SR.GetString(SR.PropertyCannotBeNull, "Page.Request"), "webPartManager"); } IPrincipal user = null; if (request.IsAuthenticated) { user = page.User; } if (user != null) { if (_supportedUserCapabilities == null) { _supportedUserCapabilities = CreateSupportedUserCapabilities(); } if ((_supportedUserCapabilities != null) && (_supportedUserCapabilities.Count != 0)) { WebPartsSection configSection = RuntimeConfig.GetConfig().WebParts; if (configSection != null) { WebPartsPersonalizationAuthorization authConfig = configSection.Personalization.Authorization; if (authConfig != null) { IDictionary capabilities = new HybridDictionary(); foreach (WebPartUserCapability capability in _supportedUserCapabilities) { if (authConfig.IsUserAllowed(user, capability.Name)) { capabilities[capability] = capability; } } return capabilities; } } } } return new HybridDictionary(); } public abstract PersonalizationStateInfoCollection FindState(PersonalizationScope scope, PersonalizationStateQuery query, int pageIndex, int pageSize, out int totalRecords); public abstract int GetCountOfState(PersonalizationScope scope, PersonalizationStateQuery query); ////// private void GetParameters(WebPartManager webPartManager, out string path, out string userName) { if (webPartManager == null) { throw new ArgumentNullException("webPartManager"); } Page page = webPartManager.Page; if (page == null) { throw new ArgumentException(SR.GetString(SR.PropertyCannotBeNull, "Page"), "webPartManager"); } HttpRequest request = page.RequestInternal; if (request == null) { throw new ArgumentException(SR.GetString(SR.PropertyCannotBeNull, "Page.Request"), "webPartManager"); } path = request.AppRelativeCurrentExecutionFilePath; userName = null; if ((webPartManager.Personalization.Scope == PersonalizationScope.User) && page.Request.IsAuthenticated) { userName = page.User.Identity.Name; } } ////// Loads the data from the data store for the specified path and user. /// If only shared data is to be loaded, userName is null or empty. /// protected abstract void LoadPersonalizationBlobs(WebPartManager webPartManager, string path, string userName, ref byte[] sharedDataBlob, ref byte[] userDataBlob); ////// Allows the provider to load personalization data. The specified /// WebPartManager is used to access the current page, which can be used /// to retrieve the path and user information. /// public virtual PersonalizationState LoadPersonalizationState(WebPartManager webPartManager, bool ignoreCurrentUser) { if (webPartManager == null) { throw new ArgumentNullException("webPartManager"); } string path; string userName; GetParameters(webPartManager, out path, out userName); if (ignoreCurrentUser) { userName = null; } byte[] sharedDataBlob = null; byte[] userDataBlob = null; LoadPersonalizationBlobs(webPartManager, path, userName, ref sharedDataBlob, ref userDataBlob); BlobPersonalizationState blobState = new BlobPersonalizationState(webPartManager); blobState.LoadDataBlobs(sharedDataBlob, userDataBlob); return blobState; } ////// Removes the data from the data store for the specified path and user. /// If userName is null or empty, the shared data is to be reset. /// protected abstract void ResetPersonalizationBlob(WebPartManager webPartManager, string path, string userName); ////// Allows the provider to reset personalization data. The specified /// WebPartManager is used to access the current page, which can be used /// to retrieve the path and user information. /// public virtual void ResetPersonalizationState(WebPartManager webPartManager) { if (webPartManager == null) { throw new ArgumentNullException("webPartManager"); } string path; string userName; GetParameters(webPartManager, out path, out userName); ResetPersonalizationBlob(webPartManager, path, userName); } public abstract int ResetState(PersonalizationScope scope, string[] paths, string[] usernames); public abstract int ResetUserState(string path, DateTime userInactiveSinceDate); ////// Saves the data into the data store for the specified path and user. /// If only shared data is to be saved, userName is null or empty. /// protected abstract void SavePersonalizationBlob(WebPartManager webPartManager, string path, string userName, byte[] dataBlob); ////// Allows the provider to save personalization data. The specified information /// contains a reference to the WebPartManager, which is used to access the /// current Page, and its path and user information. /// public virtual void SavePersonalizationState(PersonalizationState state) { if (state == null) { throw new ArgumentNullException("state"); } BlobPersonalizationState blobState = state as BlobPersonalizationState; if (blobState == null) { throw new ArgumentException(SR.GetString(SR.PersonalizationProvider_WrongType), "state"); } WebPartManager webPartManager = blobState.WebPartManager; string path; string userName; GetParameters(webPartManager, out path, out userName); byte[] dataBlob = null; bool reset = blobState.IsEmpty; if (reset == false) { dataBlob = blobState.SaveDataBlob(); reset = (dataBlob == null) || (dataBlob.Length == 0); } if (reset) { ResetPersonalizationBlob(webPartManager, path, userName); } else { SavePersonalizationBlob(webPartManager, path, userName, dataBlob); } } } }
Link Menu
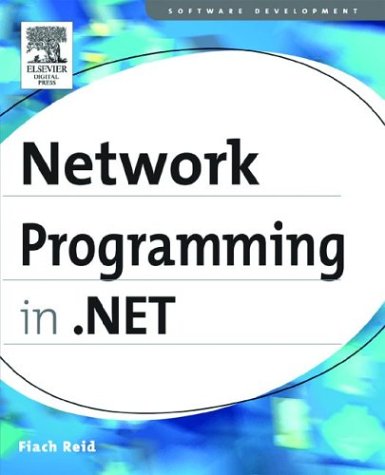
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SQLDateTimeStorage.cs
- Stylus.cs
- User.cs
- UrlPath.cs
- TypeSystem.cs
- WindowsListViewItemStartMenu.cs
- Color.cs
- UserPreferenceChangedEventArgs.cs
- CommonXSendMessage.cs
- DetailsViewInsertEventArgs.cs
- HtmlControlPersistable.cs
- AttachedProperty.cs
- DataPagerFieldCollection.cs
- ClientBuildManagerCallback.cs
- ImageButton.cs
- PagerSettings.cs
- Globals.cs
- ManipulationInertiaStartingEventArgs.cs
- ConversionContext.cs
- QueryResults.cs
- ContractCodeDomInfo.cs
- DiscriminatorMap.cs
- KnownAssembliesSet.cs
- MonikerSyntaxException.cs
- CodeGeneratorOptions.cs
- Vector3DCollectionValueSerializer.cs
- AxImporter.cs
- WebServiceResponseDesigner.cs
- SubstitutionList.cs
- Mapping.cs
- NonceToken.cs
- ThemeDictionaryExtension.cs
- XmlSchemaAll.cs
- Dictionary.cs
- WebService.cs
- SqlSelectStatement.cs
- ConfigurationFileMap.cs
- ProvideValueServiceProvider.cs
- XamlReaderConstants.cs
- WebPart.cs
- EventLogRecord.cs
- unsafenativemethodstextservices.cs
- InstancePersistenceCommandException.cs
- DrawingContextDrawingContextWalker.cs
- CngKeyBlobFormat.cs
- DesignerActionList.cs
- PeerNameResolver.cs
- DynamicDataExtensions.cs
- MetaData.cs
- HostingEnvironment.cs
- DataGridViewHitTestInfo.cs
- RuntimeResourceSet.cs
- SerTrace.cs
- XamlLoadErrorInfo.cs
- OrthographicCamera.cs
- GridEntryCollection.cs
- XmlLoader.cs
- IISUnsafeMethods.cs
- JsonEncodingStreamWrapper.cs
- BamlVersionHeader.cs
- CalendarAutoFormatDialog.cs
- StylusPlugInCollection.cs
- X509Certificate2.cs
- Select.cs
- _ChunkParse.cs
- PageThemeParser.cs
- UInt32Storage.cs
- _ConnectOverlappedAsyncResult.cs
- Predicate.cs
- PlaceHolder.cs
- DoubleAverageAggregationOperator.cs
- TabItemAutomationPeer.cs
- DataKeyArray.cs
- HtmlElementEventArgs.cs
- IncomingWebRequestContext.cs
- FormViewInsertedEventArgs.cs
- KeyedCollection.cs
- TabControl.cs
- CharUnicodeInfo.cs
- ReadOnlyDataSource.cs
- CompilerGlobalScopeAttribute.cs
- NominalTypeEliminator.cs
- ZipArchive.cs
- ISAPIRuntime.cs
- DataGridViewHitTestInfo.cs
- OleDbParameter.cs
- HtmlPageAdapter.cs
- RelationshipEndMember.cs
- CodeDefaultValueExpression.cs
- HtmlForm.cs
- ProviderBase.cs
- QueryExpr.cs
- SiteMapNodeItem.cs
- RefExpr.cs
- SettingsPropertyCollection.cs
- BeginEvent.cs
- NodeFunctions.cs
- BuildManagerHost.cs
- SafeProcessHandle.cs
- WindowShowOrOpenTracker.cs