Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / UI / WebControls / CompareValidator.cs / 1 / CompareValidator.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System.ComponentModel; using System.Web; using System.Globalization; using System.Security.Permissions; using System.Web.Util; ////// [ ToolboxData("<{0}:CompareValidator runat=\"server\" ErrorMessage=\"CompareValidator\">{0}:CompareValidator>") ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class CompareValidator : BaseCompareValidator { ///Compares the value of an input control to another input control or /// a constant value using a variety of operators and types. ////// [ WebCategory("Behavior"), Themeable(false), DefaultValue(""), WebSysDescription(SR.CompareValidator_ControlToCompare), TypeConverter(typeof(ValidatedControlConverter)) ] public string ControlToCompare { get { object o = ViewState["ControlToCompare"]; return((o == null) ? String.Empty : (string)o); } set { ViewState["ControlToCompare"] = value; } } ///Gets or sets the ID of the input control to compare with. ////// [ WebCategory("Behavior"), Themeable(false), DefaultValue(ValidationCompareOperator.Equal), WebSysDescription(SR.CompareValidator_Operator) ] public ValidationCompareOperator Operator { get { object o = ViewState["Operator"]; return((o == null) ? ValidationCompareOperator.Equal : (ValidationCompareOperator)o); } set { if (value < ValidationCompareOperator.Equal || value > ValidationCompareOperator.DataTypeCheck) { throw new ArgumentOutOfRangeException("value"); } ViewState["Operator"] = value; } } ///Gets or sets the comparison operation to perform. ////// [ WebCategory("Behavior"), Themeable(false), DefaultValue(""), WebSysDescription(SR.CompareValidator_ValueToCompare) ] public string ValueToCompare { get { object o = ViewState["ValueToCompare"]; return((o == null) ? String.Empty : (string)o); } set { ViewState["ValueToCompare"] = value; } } //Gets or sets the specific value to compare with. ///// AddAttributesToRender method // ////// /// protected override void AddAttributesToRender(HtmlTextWriter writer) { base.AddAttributesToRender(writer); if (RenderUplevel) { string id = ClientID; HtmlTextWriter expandoAttributeWriter = (EnableLegacyRendering) ? writer : null; AddExpandoAttribute(expandoAttributeWriter, id, "evaluationfunction", "CompareValidatorEvaluateIsValid", false); if (ControlToCompare.Length > 0) { string controlToCompareID = GetControlRenderID(ControlToCompare); AddExpandoAttribute(expandoAttributeWriter, id, "controltocompare", controlToCompareID); AddExpandoAttribute(expandoAttributeWriter, id, "controlhookup", controlToCompareID); } if (ValueToCompare.Length > 0) { string valueToCompareString = ValueToCompare; if (CultureInvariantValues) { valueToCompareString = ConvertCultureInvariantToCurrentCultureFormat(valueToCompareString, Type); } AddExpandoAttribute(expandoAttributeWriter, id, "valuetocompare", valueToCompareString); } if (Operator != ValidationCompareOperator.Equal) { AddExpandoAttribute(expandoAttributeWriter, id, "operator", PropertyConverter.EnumToString(typeof(ValidationCompareOperator), Operator), false); } } } ///Adds the attributes of this control to the output stream for rendering on the /// client. ////// /// protected override bool ControlPropertiesValid() { // Check the control id references if (ControlToCompare.Length > 0) { CheckControlValidationProperty(ControlToCompare, "ControlToCompare"); if (StringUtil.EqualsIgnoreCase(ControlToValidate, ControlToCompare)) { throw new HttpException(SR.GetString(SR.Validator_bad_compare_control, ID, ControlToCompare)); } } else { // Check Values if (Operator != ValidationCompareOperator.DataTypeCheck && !CanConvert(ValueToCompare, Type, CultureInvariantValues)) { throw new HttpException( SR.GetString( SR.Validator_value_bad_type, new string [] { ValueToCompare, "ValueToCompare", ID, PropertyConverter.EnumToString(typeof(ValidationDataType), Type), })); } } return base.ControlPropertiesValid(); } ///Checks the properties of a the control for valid values. ////// /// EvaluateIsValid method /// protected override bool EvaluateIsValid() { Debug.Assert(PropertiesValid, "Properties should have already been checked"); // Get the peices of text from the control. string leftText = GetControlValidationValue(ControlToValidate); Debug.Assert(leftText != null, "Should have already caught this!"); // Special case: if the string is blank, we don't try to validate it. The input should be // trimmed for coordination with the RequiredFieldValidator. if (leftText.Trim().Length == 0) { return true; } // VSWhidbey 83168 bool convertDate = (Type == ValidationDataType.Date && !DetermineRenderUplevel()); if (convertDate && !IsInStandardDateFormat(leftText)) { leftText = ConvertToShortDateString(leftText); } // The control has precedence over the fixed value bool isCultureInvariantValue = false; string rightText = string.Empty; if (ControlToCompare.Length > 0) { rightText = GetControlValidationValue(ControlToCompare); Debug.Assert(rightText != null, "Should have already caught this!"); // VSWhidbey 83089 if (convertDate && !IsInStandardDateFormat(rightText)) { rightText = ConvertToShortDateString(rightText); } } else { rightText = ValueToCompare; isCultureInvariantValue = CultureInvariantValues; } return Compare(leftText, false, rightText, isCultureInvariantValue, Operator, Type); } } }
Link Menu
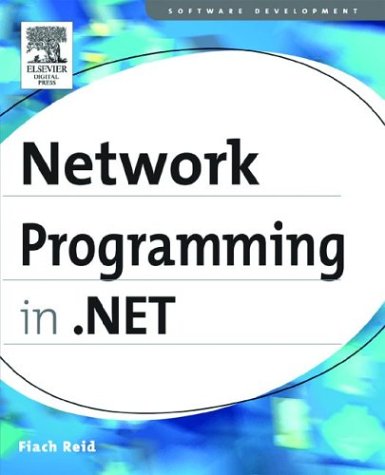
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PrintPageEvent.cs
- SectionXmlInfo.cs
- COM2Enum.cs
- AsnEncodedData.cs
- NetworkInterface.cs
- MouseGestureValueSerializer.cs
- RuleAction.cs
- CapabilitiesState.cs
- DependencyProperty.cs
- PropertyNames.cs
- IriParsingElement.cs
- CodeLinePragma.cs
- WhitespaceRuleReader.cs
- TextBoxLine.cs
- RightsManagementInformation.cs
- HttpListenerContext.cs
- ZipIORawDataFileBlock.cs
- SubstitutionResponseElement.cs
- RowUpdatingEventArgs.cs
- SqlBinder.cs
- XmlSerializer.cs
- DetailsView.cs
- SchemaCollectionPreprocessor.cs
- TriState.cs
- InboundActivityHelper.cs
- ToolStripItemImageRenderEventArgs.cs
- SingleBodyParameterMessageFormatter.cs
- DetailsViewDeleteEventArgs.cs
- ITextView.cs
- AdornedElementPlaceholder.cs
- ClientBase.cs
- SyndicationFeed.cs
- ModifyActivitiesPropertyDescriptor.cs
- ProfileEventArgs.cs
- MailWebEventProvider.cs
- SoapMessage.cs
- FtpWebRequest.cs
- RandomNumberGenerator.cs
- TransportSecurityProtocolFactory.cs
- WebBrowserContainer.cs
- HtmlTableCellCollection.cs
- IntSecurity.cs
- _SSPIWrapper.cs
- AttachedPropertyBrowsableForChildrenAttribute.cs
- SamlSecurityToken.cs
- BitmapCodecInfo.cs
- Hex.cs
- MimeReflector.cs
- ArrayTypeMismatchException.cs
- ExpressionBuilderCollection.cs
- TextParagraphCache.cs
- DocumentPageViewAutomationPeer.cs
- XmlIterators.cs
- RuntimeConfigLKG.cs
- PenThreadPool.cs
- InheritedPropertyChangedEventArgs.cs
- GPRECTF.cs
- XmlSchemaAnnotated.cs
- StreamingContext.cs
- ElementUtil.cs
- DocumentPaginator.cs
- Boolean.cs
- HMACRIPEMD160.cs
- WindowsSysHeader.cs
- ObjectDataSourceChooseTypePanel.cs
- OciLobLocator.cs
- Matrix.cs
- DataBindingHandlerAttribute.cs
- FlowDocumentPageViewerAutomationPeer.cs
- DataGridViewDataErrorEventArgs.cs
- PathNode.cs
- ProfileModule.cs
- storepermission.cs
- DbReferenceCollection.cs
- Block.cs
- ParallelLoopState.cs
- XPathDocumentBuilder.cs
- SecurityContext.cs
- StubHelpers.cs
- CreationContext.cs
- WindowsFormsHost.cs
- EditorBrowsableAttribute.cs
- XXXInfos.cs
- ChtmlTextBoxAdapter.cs
- TextEditorLists.cs
- MarkupCompilePass2.cs
- BinaryFormatter.cs
- DataGridViewImageCell.cs
- ThicknessAnimationUsingKeyFrames.cs
- ParallelTimeline.cs
- RectAnimationClockResource.cs
- securitycriticaldataClass.cs
- StaticResourceExtension.cs
- ADMembershipUser.cs
- entityreference_tresulttype.cs
- SID.cs
- TokenCreationException.cs
- WebPartCatalogCloseVerb.cs
- EmulateRecognizeCompletedEventArgs.cs
- TextBox.cs