Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / DataGridViewAccessibleObject.cs / 1 / DataGridViewAccessibleObject.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System.Security.Permissions; using System.Diagnostics.CodeAnalysis; using System.Drawing; namespace System.Windows.Forms { public partial class DataGridView { [ System.Runtime.InteropServices.ComVisible(true) ] ///protected class DataGridViewAccessibleObject : ControlAccessibleObject { DataGridView owner; DataGridViewTopRowAccessibleObject topRowAccessibilityObject = null; DataGridViewSelectedCellsAccessibleObject selectedCellsAccessibilityObject = null; /// public DataGridViewAccessibleObject(DataGridView owner) : base(owner) { this.owner = owner; } /// public override string Name { [SuppressMessage("Microsoft.Globalization", "CA1303:DoNotPassLiteralsAsLocalizedParameters")] // Don't localize string "DataGridView". get { string name = this.Owner.AccessibleName; if (!String.IsNullOrEmpty(name)) { return name; } else { // The default name should not be localized. return "DataGridView"; } } } /// public override AccessibleRole Role { get { AccessibleRole role = owner.AccessibleRole; if (role != AccessibleRole.Default) { return role; } // the Default AccessibleRole is Table return AccessibleRole.Table; } } private AccessibleObject TopRowAccessibilityObject { get { if (this.topRowAccessibilityObject == null) { this.topRowAccessibilityObject = new DataGridViewTopRowAccessibleObject(this.owner); } return this.topRowAccessibilityObject; } } private AccessibleObject SelectedCellsAccessibilityObject { get { if (this.selectedCellsAccessibilityObject == null) { this.selectedCellsAccessibilityObject = new DataGridViewSelectedCellsAccessibleObject(this.owner); } return this.selectedCellsAccessibilityObject; } } /// public override AccessibleObject GetChild(int index) { if (this.owner.Columns.Count == 0) { System.Diagnostics.Debug.Assert(this.GetChildCount() == 0); return null; } if (index < 1 && this.owner.ColumnHeadersVisible) { return this.TopRowAccessibilityObject; } if (this.owner.ColumnHeadersVisible) { index--; } if (index < this.owner.Rows.GetRowCount(DataGridViewElementStates.Visible)) { int actualRowIndex = this.owner.Rows.DisplayIndexToRowIndex(index); return this.owner.Rows[actualRowIndex].AccessibilityObject; } index -= this.owner.Rows.GetRowCount(DataGridViewElementStates.Visible); if (this.owner.horizScrollBar.Visible) { if (index == 0) { return this.owner.horizScrollBar.AccessibilityObject; } else { index--; } } if (this.owner.vertScrollBar.Visible) { if (index == 0) { return this.owner.vertScrollBar.AccessibilityObject; } } return null; } /// public override int GetChildCount() { if (this.owner.Columns.Count == 0) { return 0; } int childCount = this.owner.Rows.GetRowCount(DataGridViewElementStates.Visible); // the column header collection Accessible Object if (this.owner.ColumnHeadersVisible) { childCount++; } if (this.owner.horizScrollBar.Visible) { childCount++; } if (this.owner.vertScrollBar.Visible) { childCount++; } return childCount; } /// public override AccessibleObject GetFocused() { if (this.owner.Focused && this.owner.CurrentCell != null) { return this.owner.CurrentCell.AccessibilityObject; } else { return null; } } /// public override AccessibleObject GetSelected() { return this.SelectedCellsAccessibilityObject; } /// public override AccessibleObject HitTest(int x, int y) { Point pt = this.owner.PointToClient(new Point(x, y)); HitTestInfo hti = this.owner.HitTest(pt.X, pt.Y); switch (hti.Type) { case DataGridViewHitTestType.Cell: return this.owner.Rows[hti.RowIndex].Cells[hti.ColumnIndex].AccessibilityObject; case DataGridViewHitTestType.ColumnHeader: // map the column index to the actual display index int actualDisplayIndex = this.owner.Columns.ColumnIndexToActualDisplayIndex(hti.ColumnIndex, DataGridViewElementStates.Visible); if (this.owner.RowHeadersVisible) { // increment the childIndex because the first child in the TopRowAccessibleObject is the TopLeftHeaderCellAccObj return this.TopRowAccessibilityObject.GetChild(actualDisplayIndex + 1); } else { return this.TopRowAccessibilityObject.GetChild(actualDisplayIndex); } case DataGridViewHitTestType.RowHeader: return this.owner.Rows[hti.RowIndex].AccessibilityObject; case DataGridViewHitTestType.TopLeftHeader: return this.owner.TopLeftHeaderCell.AccessibilityObject; case DataGridViewHitTestType.VerticalScrollBar: return this.owner.VerticalScrollBar.AccessibilityObject; case DataGridViewHitTestType.HorizontalScrollBar: return this.owner.HorizontalScrollBar.AccessibilityObject; default: return null; } } /// [SecurityPermission(SecurityAction.Demand, Flags = SecurityPermissionFlag.UnmanagedCode)] public override AccessibleObject Navigate(AccessibleNavigation navigationDirection) { switch (navigationDirection) { case AccessibleNavigation.FirstChild: return this.GetChild(0); case AccessibleNavigation.LastChild: return this.GetChild(this.GetChildCount() - 1); default: return null; } } /* [....]: why is this method defined and not used? // this method is called when the accessible object needs to be reset // Example: when the user changes the display index on a column or when the user modifies the column collection internal void Reset() { this.NotifyClients(AccessibleEvents.Reorder); } */ } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
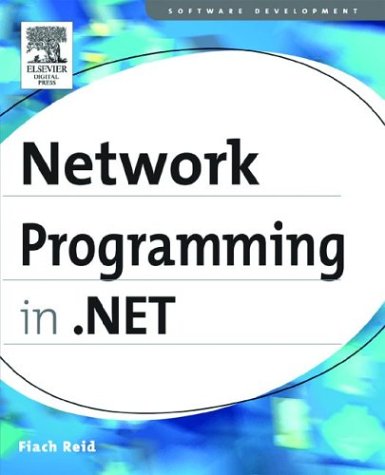
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FaultBookmark.cs
- CompatibleIComparer.cs
- CodeStatement.cs
- WorkflowViewService.cs
- NodeInfo.cs
- translator.cs
- ContractUtils.cs
- UserNameSecurityTokenProvider.cs
- AxHostDesigner.cs
- RawStylusInputReport.cs
- DynamicResourceExtension.cs
- SqlServer2KCompatibilityAnnotation.cs
- InlineCollection.cs
- SqlEnums.cs
- TableItemStyle.cs
- PolicyDesigner.cs
- SelectedDatesCollection.cs
- SecurityRuntime.cs
- ButtonFlatAdapter.cs
- ColumnCollection.cs
- SchemaObjectWriter.cs
- Color.cs
- GridErrorDlg.cs
- PointLightBase.cs
- AssertUtility.cs
- SrgsGrammarCompiler.cs
- LocalizableResourceBuilder.cs
- HandlerWithFactory.cs
- LinkUtilities.cs
- CategoryGridEntry.cs
- WhitespaceRuleLookup.cs
- Renderer.cs
- ProcessThread.cs
- SqlCacheDependency.cs
- DataGridColumnFloatingHeader.cs
- TypeUsage.cs
- RijndaelCryptoServiceProvider.cs
- ImageClickEventArgs.cs
- XmlCharCheckingWriter.cs
- WindowsListViewGroupSubsetLink.cs
- DockingAttribute.cs
- OdbcConnection.cs
- SQLInt16Storage.cs
- StorageMappingItemCollection.cs
- RSAPKCS1SignatureDeformatter.cs
- LambdaCompiler.Lambda.cs
- WebPartConnectionCollection.cs
- InheritanceAttribute.cs
- DataAdapter.cs
- dsa.cs
- SafeHandles.cs
- InteropBitmapSource.cs
- TreeView.cs
- GB18030Encoding.cs
- Mutex.cs
- XmlExtensionFunction.cs
- RuleSettingsCollection.cs
- SqlFactory.cs
- TraceContext.cs
- ProcessInputEventArgs.cs
- EntityTemplateFactory.cs
- ContentFileHelper.cs
- VariableQuery.cs
- TextEvent.cs
- OdbcUtils.cs
- BasicSecurityProfileVersion.cs
- CompiledAction.cs
- SpeechRecognizer.cs
- PropertyInfoSet.cs
- FileDialogCustomPlaces.cs
- FastPropertyAccessor.cs
- SerialErrors.cs
- CompiledIdentityConstraint.cs
- HandlerWithFactory.cs
- DbQueryCommandTree.cs
- DataSourceSelectArguments.cs
- CanonicalXml.cs
- IPCCacheManager.cs
- HtmlHistory.cs
- ProfilePropertyNameValidator.cs
- InputGestureCollection.cs
- CollectionEditorDialog.cs
- CharacterMetrics.cs
- ThreadNeutralSemaphore.cs
- StylusShape.cs
- ResourcePool.cs
- SendKeys.cs
- BindingMemberInfo.cs
- CleanUpVirtualizedItemEventArgs.cs
- CompiledQuery.cs
- IssuanceLicense.cs
- SoapExtensionTypeElement.cs
- XhtmlTextWriter.cs
- DefaultParameterValueAttribute.cs
- PermissionListSet.cs
- CallbackValidator.cs
- HtmlElementCollection.cs
- ConfigurationStrings.cs
- TextRangeEditTables.cs
- DataBindEngine.cs