Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / CommonUI / System / Drawing / RectangleConverter.cs / 1 / RectangleConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Drawing { using System.Runtime.Serialization.Formatters; using System.Runtime.InteropServices; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using Microsoft.Win32; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Globalization; using System.Reflection; ////// /// RectangleConverter is a class that can be used to convert /// rectangles from one data type to another. Access this /// class through the TypeDescriptor. /// public class RectangleConverter : TypeConverter { ////// /// Determines if this converter can convert an object in the given source /// type to the native type of the converter. /// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return base.CanConvertFrom(context, sourceType); } ////// /// public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(InstanceDescriptor)) { return true; } return base.CanConvertTo(context, destinationType); } ///Gets a value indicating whether this converter can /// convert an object to the given destination type using the context. ////// /// Converts the given object to the converter's native type. /// [SuppressMessage("Microsoft.Performance", "CA1808:AvoidCallsThatBoxValueTypes")] public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { string strValue = value as string; if (strValue != null) { string text = strValue.Trim(); if (text.Length == 0) { return null; } else { // Parse 4 integer values. // if (culture == null) { culture = CultureInfo.CurrentCulture; } char sep = culture.TextInfo.ListSeparator[0]; string[] tokens = text.Split(new char[] {sep}); int[] values = new int[tokens.Length]; TypeConverter intConverter = TypeDescriptor.GetConverter(typeof(int)); for (int i = 0; i < values.Length; i++) { // Note: ConvertFromString will raise exception if value cannot be converted. values[i] = (int)intConverter.ConvertFromString(context, culture, tokens[i]); } if (values.Length == 4) { return new Rectangle(values[0], values[1], values[2], values[3]); } else { throw new ArgumentException(SR.GetString(SR.TextParseFailedFormat, "text", text, "x, y, width, height")); } } } return base.ConvertFrom(context, culture, value); } ////// /// Converts the given object to another type. The most common types to convert /// are to and from a string object. The default implementation will make a call /// to ToString on the object if the object is valid and if the destination /// type is string. If this cannot convert to the desitnation type, this will /// throw a NotSupportedException. /// public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } if( value is Rectangle ){ if (destinationType == typeof(string)) { Rectangle rect = (Rectangle)value; if (culture == null) { culture = CultureInfo.CurrentCulture; } string sep = culture.TextInfo.ListSeparator + " "; TypeConverter intConverter = TypeDescriptor.GetConverter(typeof(int)); string[] args = new string[4]; int nArg = 0; // Note: ConvertToString will raise exception if value cannot be converted. args[nArg++] = intConverter.ConvertToString(context, culture, rect.X); args[nArg++] = intConverter.ConvertToString(context, culture, rect.Y); args[nArg++] = intConverter.ConvertToString(context, culture, rect.Width); args[nArg++] = intConverter.ConvertToString(context, culture, rect.Height); return string.Join(sep, args); } if (destinationType == typeof(InstanceDescriptor)) { Rectangle rect = (Rectangle)value; ConstructorInfo ctor = typeof(Rectangle).GetConstructor(new Type[] { typeof(int), typeof(int), typeof(int), typeof(int)}); if (ctor != null) { return new InstanceDescriptor(ctor, new object[] { rect.X, rect.Y, rect.Width, rect.Height}); } } } return base.ConvertTo(context, culture, value, destinationType); } ////// /// Creates an instance of this type given a set of property values /// for the object. This is useful for objects that are immutable, but still /// want to provide changable properties. /// [SuppressMessage("Microsoft.Performance", "CA1808:AvoidCallsThatBoxValueTypes")] [SuppressMessage("Microsoft.Security", "CA2102:CatchNonClsCompliantExceptionsInGeneralHandlers")] public override object CreateInstance(ITypeDescriptorContext context, IDictionary propertyValues) { if( propertyValues == null ){ throw new ArgumentNullException( "propertyValues" ); } object x = propertyValues["X"]; object y = propertyValues["Y"]; object width = propertyValues["Width"]; object height = propertyValues["Height"]; if(x == null || y == null || width == null || height == null || !(x is int) || !(y is int) || !(width is int) || !(height is int) ) { throw new ArgumentException(SR.GetString(SR.PropertyValueInvalidEntry)); } return new Rectangle((int)x, (int)y, (int)width, (int)height); } ////// /// Determines if changing a value on this object should require a call to /// CreateInstance to create a new value. /// public override bool GetCreateInstanceSupported(ITypeDescriptorContext context) { return true; } ////// /// Retrieves the set of properties for this type. By default, a type has /// does not return any properties. An easy implementation of this method /// can just call TypeDescriptor.GetProperties for the correct data type. /// public override PropertyDescriptorCollection GetProperties(ITypeDescriptorContext context, object value, Attribute[] attributes) { PropertyDescriptorCollection props = TypeDescriptor.GetProperties(typeof(Rectangle), attributes); return props.Sort(new string[] {"X", "Y", "Width", "Height"}); } ////// /// Determines if this object supports properties. By default, this /// is false. /// public override bool GetPropertiesSupported(ITypeDescriptorContext context) { return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
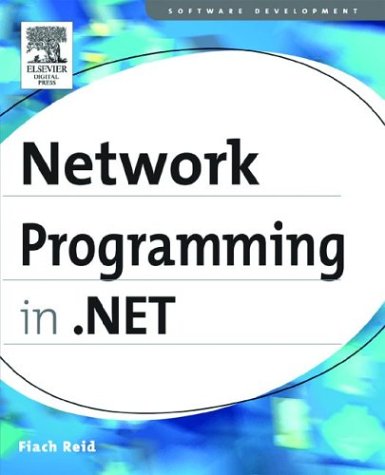
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CacheMode.cs
- WarningException.cs
- TreeNodeSelectionProcessor.cs
- DetailsViewPageEventArgs.cs
- EntityDataSourceEntityTypeFilterConverter.cs
- ITreeGenerator.cs
- Int16Animation.cs
- SessionStateItemCollection.cs
- SqlBooleanizer.cs
- PerspectiveCamera.cs
- XmlSchemas.cs
- CriticalExceptions.cs
- SchemaTableColumn.cs
- ListView.cs
- SecureUICommand.cs
- WebPartConnectionsCancelVerb.cs
- ConstantSlot.cs
- ChangePasswordAutoFormat.cs
- UnmanagedMemoryStream.cs
- HelpEvent.cs
- XmlnsCompatibleWithAttribute.cs
- CodeObjectCreateExpression.cs
- RepeatInfo.cs
- ReadWriteControlDesigner.cs
- NetworkStream.cs
- processwaithandle.cs
- HtmlInputSubmit.cs
- Material.cs
- CapiNative.cs
- WebControlParameterProxy.cs
- TextRange.cs
- ServiceContractAttribute.cs
- HttpBufferlessInputStream.cs
- PolicyLevel.cs
- xmlglyphRunInfo.cs
- HandlerBase.cs
- DateTimeConverter2.cs
- MexHttpBindingElement.cs
- MediaTimeline.cs
- SafeReversePInvokeHandle.cs
- WebServiceData.cs
- SystemColors.cs
- StatusCommandUI.cs
- BinaryObjectReader.cs
- DataSpaceManager.cs
- QuaternionConverter.cs
- RolePrincipal.cs
- SqlCommandBuilder.cs
- Underline.cs
- SystemWebSectionGroup.cs
- RoleManagerSection.cs
- SqlFactory.cs
- WhitespaceRuleReader.cs
- SafeFileMappingHandle.cs
- XmlSchemaSimpleTypeList.cs
- ServiceOperationParameter.cs
- DiscardableAttribute.cs
- PropertySet.cs
- ConfigUtil.cs
- StylusTip.cs
- HtmlValidatorAdapter.cs
- BitmapSource.cs
- GraphicsContainer.cs
- DependencyObjectPropertyDescriptor.cs
- WebPartsPersonalization.cs
- BrowserDefinitionCollection.cs
- PTManager.cs
- ReplacementText.cs
- X509RecipientCertificateClientElement.cs
- SqlNode.cs
- PlanCompilerUtil.cs
- VirtualDirectoryMappingCollection.cs
- DiscardableAttribute.cs
- ProjectedSlot.cs
- TextEffect.cs
- CalendarAutoFormatDialog.cs
- ProjectionPlan.cs
- EdmComplexTypeAttribute.cs
- DynamicDataRoute.cs
- WindowsListBox.cs
- ClientSettingsStore.cs
- EntityTypeEmitter.cs
- JapaneseLunisolarCalendar.cs
- StreamWithDictionary.cs
- ForceCopyBuildProvider.cs
- CommandField.cs
- SqlInternalConnectionSmi.cs
- FontDifferentiator.cs
- SqlDesignerDataSourceView.cs
- UnaryQueryOperator.cs
- IsolationInterop.cs
- HostingEnvironmentWrapper.cs
- PageCatalogPart.cs
- DataGridRowClipboardEventArgs.cs
- ClientScriptManagerWrapper.cs
- AutomationPeer.cs
- ExpressionBuilderCollection.cs
- HttpBrowserCapabilitiesWrapper.cs
- PhonemeEventArgs.cs
- CompiledELinqQueryState.cs