Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / clr / src / BCL / System / Globalization / SortKey.cs / 1 / SortKey.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //////////////////////////////////////////////////////////////////////////// // // Class: SortKey // // Purpose: This class implements a set of methods for retrieving // sort key information. // // Date: August 12, 1998 // //////////////////////////////////////////////////////////////////////////// namespace System.Globalization { using System; using System.Runtime.CompilerServices; [System.Runtime.InteropServices.ComVisible(true)] [Serializable] public class SortKey { //--------------------------------------------------------------------// // Internal Information // //-------------------------------------------------------------------// // // Variables. // internal int win32LCID; // 32-bit Win32 locale ID. It may contain a 4-bit sort ID. internal CompareOptions options; // options internal String m_String; // original string internal byte[] m_KeyData; // sortkey data // Mask used to check if the c'tor has the right flags. private const CompareOptions ValidSortkeyCtorMaskOffFlags = ~(CompareOptions.IgnoreCase | CompareOptions.IgnoreSymbols | CompareOptions.IgnoreNonSpace | CompareOptions.IgnoreWidth | CompareOptions.IgnoreKanaType | CompareOptions.StringSort); //////////////////////////////////////////////////////////////////////// // // SortKey Constructor // // Implements CultureInfo.CompareInfo.GetSortKey(). // Package access only. // //////////////////////////////////////////////////////////////////////// unsafe internal SortKey(void* pSortingFile, int win32LCID, String str, CompareOptions options) { if (str==null) { throw new ArgumentNullException("str"); } // // WARNING! WARNING! WARNING! WARNING! WARNING! WARNING! WARNING! WARNING! WARNING! // // This parameter check was added in Whidbey prior to Beta 2. We are keeping this // change in mind in case we hear of any issues with it. // // WARNING! WARNING! WARNING! WARNING! WARNING! WARNING! WARNING! WARNING! WARNING! // if ((options & ValidSortkeyCtorMaskOffFlags) != 0) { throw new ArgumentException(Environment.GetResourceString("Argument_InvalidFlag"), "options"); } //This takes the sort id from CompareInfo, so we should be able to skip initializing it. this.win32LCID = win32LCID; this.options = options; m_String = str; //We need an initialized SortTable here, but since we're getting this as an instance //method off of a CompareInfo, we're guaranteed that that already happened. m_KeyData = CompareInfo.nativeCreateSortKey(pSortingFile, str, (int)options, win32LCID); } #if !FEATURE_PAL // // The following constructor is designed to be called from CompareInfo to get the // the sort key of specific string for synthetic culture // //internal SortKey(int win32LCID, String str, CompareOptions options) { if (str==null) { throw new ArgumentNullException("str"); } if ((options & ValidSortkeyCtorMaskOffFlags) != 0) { throw new ArgumentException(Environment.GetResourceString("Argument_InvalidFlag"), "options"); } if (CultureInfo.GetNativeSortKey(win32LCID, CompareInfo.GetNativeCompareFlags(options), str, str.Length, out m_KeyData) <= 0) { throw new ArgumentException(Environment.GetResourceString("Argument_InvalidFlag"), "str"); } this.win32LCID = win32LCID; this.options = options; this.m_String = str; } #endif // !FEATURE_PAL //////////////////////////////////////////////////////////////////////// // // GetOriginalString // // Returns the original string used to create the current instance // of SortKey. // //////////////////////////////////////////////////////////////////////// public virtual String OriginalString { get { return (m_String); } } //////////////////////////////////////////////////////////////////////// // // GetKeyData // // Returns a byte array representing the current instance of the // sort key. // //////////////////////////////////////////////////////////////////////// public virtual byte[] KeyData { get { return (byte[])(m_KeyData.Clone()); } } //////////////////////////////////////////////////////////////////////// // // Compare // // Compares the two sort keys. Returns 0 if the two sort keys are // equal, a number less than 0 if sortkey1 is less than sortkey2, // and a number greater than 0 if sortkey1 is greater than sortkey2. // //////////////////////////////////////////////////////////////////////// public static int Compare(SortKey sortkey1, SortKey sortkey2) { if (sortkey1==null || sortkey2==null) { throw new ArgumentNullException((sortkey1==null ? "sortkey1": "sortkey2")); } byte[] key1Data = sortkey1.m_KeyData; byte[] key2Data = sortkey2.m_KeyData; BCLDebug.Assert(key1Data!=null, "key1Data!=null"); BCLDebug.Assert(key2Data!=null, "key2Data!=null"); if (key1Data.Length == 0) { if (key2Data.Length == 0) { return (0); } return (-1); } if (key2Data.Length == 0) { return (1); } int compLen = (key1Data.Length key2Data[i]) { return (1); } if (key1Data[i]
Link Menu
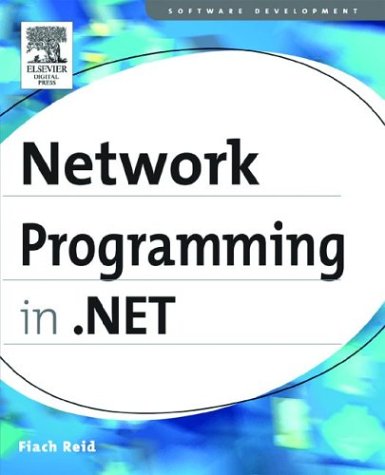
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NamespaceInfo.cs
- PeerNameRecordCollection.cs
- RuleEngine.cs
- XmlArrayItemAttribute.cs
- ChameleonKey.cs
- PipeConnection.cs
- GridLength.cs
- CurrentTimeZone.cs
- AttachedPropertyInfo.cs
- ProfileSettingsCollection.cs
- DropShadowBitmapEffect.cs
- SqlTypeSystemProvider.cs
- DefaultClaimSet.cs
- DesignerActionUIService.cs
- ImageSourceConverter.cs
- GZipDecoder.cs
- StringResourceManager.cs
- OleDbConnection.cs
- ImageUrlEditor.cs
- SafeMILHandle.cs
- AQNBuilder.cs
- DocumentPageView.cs
- IIS7UserPrincipal.cs
- DataGridViewCellStyleConverter.cs
- TextEditorCharacters.cs
- AutomationPatternInfo.cs
- newinstructionaction.cs
- AtlasWeb.Designer.cs
- MissingFieldException.cs
- InstanceHandleConflictException.cs
- BaseWebProxyFinder.cs
- WebPartZoneDesigner.cs
- WebBrowser.cs
- _TLSstream.cs
- SizeAnimationUsingKeyFrames.cs
- ComponentRenameEvent.cs
- WinFormsComponentEditor.cs
- ButtonColumn.cs
- SchemaDeclBase.cs
- StylusPointPropertyInfoDefaults.cs
- SetterBaseCollection.cs
- GridViewRowPresenter.cs
- RouteParser.cs
- UniqueConstraint.cs
- TextBox.cs
- SQLSingleStorage.cs
- TextEditorLists.cs
- AssertFilter.cs
- PathFigureCollection.cs
- KeyInterop.cs
- ServiceDocumentFormatter.cs
- FastPropertyAccessor.cs
- EncoderParameters.cs
- CapabilitiesPattern.cs
- ArgumentDesigner.xaml.cs
- XmlSchemaParticle.cs
- XmlSchemaComplexContentExtension.cs
- Underline.cs
- Enum.cs
- DataServiceRequest.cs
- SecurityRequiresReviewAttribute.cs
- WebPartAddingEventArgs.cs
- DataListCommandEventArgs.cs
- VisualBrush.cs
- CreateUserWizardStep.cs
- WebPartDisplayModeCancelEventArgs.cs
- VisualStyleRenderer.cs
- lengthconverter.cs
- XPathAncestorIterator.cs
- XslNumber.cs
- BitmapDecoder.cs
- CqlParser.cs
- updateconfighost.cs
- FacetValueContainer.cs
- StylesEditorDialog.cs
- WasHostedComPlusFactory.cs
- ReaderWriterLock.cs
- WindowAutomationPeer.cs
- CodeNamespaceImportCollection.cs
- DesignerDataParameter.cs
- DbTypeMap.cs
- login.cs
- LinearGradientBrush.cs
- IListConverters.cs
- Selection.cs
- TextSerializer.cs
- RegexBoyerMoore.cs
- Subtree.cs
- CompilerLocalReference.cs
- XPathAncestorQuery.cs
- LassoSelectionBehavior.cs
- ClickablePoint.cs
- HandlerWithFactory.cs
- DataBoundControlHelper.cs
- WebPartRestoreVerb.cs
- securitycriticaldataformultiplegetandset.cs
- DecoderReplacementFallback.cs
- FlatButtonAppearance.cs
- WebPartChrome.cs
- WebPartCatalogCloseVerb.cs