Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / clr / src / BCL / System / Globalization / CalendarTable.cs / 1 / CalendarTable.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== namespace System.Globalization { using System.Runtime.Remoting; using System; using System.Runtime.CompilerServices; using System.Threading; using System.Runtime.Versioning; using System.Runtime.InteropServices; /*============================================================================== * * Calendar Data table for DateTimeFormatInfo classes. Used by System.Globalization.DateTimeFormatInfo. * ==============================================================================*/ internal class CalendarTable : BaseInfoTable { // The default instance of calendar table. We should only create one instance per app-domain. private static CalendarTable m_defaultInstance; unsafe CalendarTableData* m_calendars; [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] static CalendarTable() { m_defaultInstance = new CalendarTable("culture.nlp", true); } [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] internal CalendarTable(String fileName, bool fromAssembly) : base(fileName, fromAssembly) { // Do nothing here. } //////////////////////////////////////////////////////////////////////// // // Set Data Item Pointers that are unique to calendar table // //////////////////////////////////////////////////////////////////////// internal override unsafe void SetDataItemPointers() { m_itemSize = m_pCultureHeader->sizeCalendarItem; m_numItem = m_pCultureHeader->numCalendarItems; m_pDataPool = (ushort*)(m_pDataFileStart + m_pCultureHeader->offsetToDataPool); // We subtract item size because calender indices start at 1 but our table doesn't have an entry for 0 m_pItemData = m_pDataFileStart + m_pCultureHeader->offsetToCalendarItemData - m_itemSize; // Get calendar list. We subtract 1 because calender indices start at 1 but our table doesn't have an entry for 0 m_calendars = (CalendarTableData*)(m_pDataFileStart + m_pCultureHeader->offsetToCalendarItemData) - 1; } internal static CalendarTable Default { get { BCLDebug.Assert(m_defaultInstance != null, "CalendarTable.Default: default instance is not created."); return (m_defaultInstance); } } internal unsafe int ICURRENTERA(int id) { BCLDebug.Assert(id > 0 && id <= m_numItem, "[CalendarTable.ICURRENTERA]id out of calendar range"); return m_calendars[id].iCurrentEra; } internal unsafe int IFORMATFLAGS(int id) { BCLDebug.Assert(id > 0 && id <= m_numItem, "[CalendarTable.IFORMATFLAGS]id out of calendar range"); return m_calendars[id].iFormatFlags; } internal unsafe String[] SDAYNAMES(int id) { BCLDebug.Assert(id > 0 && id <= m_numItem, "[CalendarTable.SDAYNAMES]id out of calendar range"); return GetStringArray(m_calendars[id].saDayNames); } internal unsafe String[] SABBREVDAYNAMES(int id) { BCLDebug.Assert(id > 0 && id <= m_numItem, "[CalendarTable.SABBREVDAYNAMES]id out of calendar range"); return GetStringArray(m_calendars[id].saAbbrevDayNames); } internal unsafe String[] SSUPERSHORTDAYNAMES(int id) { BCLDebug.Assert(id > 0 && id <= m_numItem, "[CalendarTable.SSUPERSHORTDAYNAMES]id out of calendar range"); return GetStringArray(m_calendars[id].saSuperShortDayNames); } internal unsafe String[] SMONTHNAMES(int id) { BCLDebug.Assert(id > 0 && id <= m_numItem, "[CalendarTable.SMONTHNAMES]id out of calendar range"); return GetStringArray(m_calendars[id].saMonthNames); } internal unsafe String[] SABBREVMONTHNAMES(int id) { BCLDebug.Assert(id > 0 && id <= m_numItem, "[CalendarTable.SABBREVMONTHNAMES]id out of calendar range"); return GetStringArray(m_calendars[id].saAbbrevMonthNames); } internal unsafe String[] SLEAPYEARMONTHNAMES(int id) { BCLDebug.Assert(id > 0 && id <= m_numItem, "[CalendarTable.SLEAPYEARMONTHNAMES]id out of calendar range"); return GetStringArray(m_calendars[id].saLeapYearMonthNames); } internal unsafe String[] SSHORTDATE(int id) { BCLDebug.Assert(id > 0 && id <= m_numItem, "[CalendarTable.SSHORTDATE]id out of calendar range"); return GetStringArray(m_calendars[id].saShortDate); } internal unsafe String[] SLONGDATE(int id) { BCLDebug.Assert(id > 0 && id <= m_numItem, "[CalendarTable.SLONGDATE]id out of calendar range"); return GetStringArray(m_calendars[id].saLongDate); } internal unsafe String[] SYEARMONTH(int id) { BCLDebug.Assert(id > 0 && id <= m_numItem, "[CalendarTable.SYEARMONTH]id out of calendar range"); return GetStringArray(m_calendars[id].saYearMonth); } internal unsafe String SMONTHDAY(int id) { BCLDebug.Assert(id > 0 && id <= m_numItem, "[CalendarTable.SMONTHDAY]id out of calendar range"); return GetStringPoolString(m_calendars[id].sMonthDay); } internal unsafe int[][] SERARANGES(int id) { BCLDebug.Assert(id > 0 && id <= m_numItem, "[CalendarTable.SERARANGES]id out of calendar range"); return GetWordArrayArray(m_calendars[id].waaEraRanges); } internal unsafe String[] SERANAMES(int id) { BCLDebug.Assert(id > 0 && id <= m_numItem, "[CalendarTable.SERANAMES]id out of calendar range"); return GetStringArray(m_calendars[id].saEraNames); } internal unsafe String[] SABBREVERANAMES(int id) { BCLDebug.Assert(id > 0 && id <= m_numItem, "[CalendarTable.SABBREVERANAMES]id out of calendar range"); return GetStringArray(m_calendars[id].saAbbrevEraNames); } internal unsafe String[] SABBREVENGERANAMES(int id) { BCLDebug.Assert(id > 0 && id <= m_numItem, "[CalendarTable.SABBREVENGERANAMES]id out of calendar range"); return GetStringArray(m_calendars[id].saAbbrevEnglishEraNames); } [MethodImplAttribute(MethodImplOptions.InternalCall)] internal static extern String nativeGetEraName(int culture, int calID); } }
Link Menu
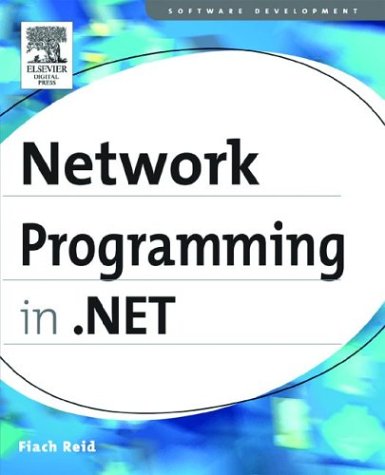
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GACMembershipCondition.cs
- EntityClientCacheKey.cs
- TreeBuilderBamlTranslator.cs
- SafeBitVector32.cs
- PropertyMappingExceptionEventArgs.cs
- TableCellsCollectionEditor.cs
- Grid.cs
- DBConnection.cs
- GeneralTransform2DTo3DTo2D.cs
- SchemaDeclBase.cs
- SHA256.cs
- RefreshInfo.cs
- SqlColumnizer.cs
- SerialErrors.cs
- VerificationException.cs
- StyleConverter.cs
- SqlBooleanizer.cs
- JapaneseCalendar.cs
- SocketPermission.cs
- DbBuffer.cs
- SoapEnumAttribute.cs
- HandleRef.cs
- WebPartDisplayMode.cs
- GroupBox.cs
- TreeViewImageKeyConverter.cs
- DataControlPagerLinkButton.cs
- AdCreatedEventArgs.cs
- FuncTypeConverter.cs
- DataGridTablesFactory.cs
- DrawTreeNodeEventArgs.cs
- PropertyStore.cs
- GraphicsPathIterator.cs
- AnimationClock.cs
- AssemblyCollection.cs
- DateBoldEvent.cs
- AnimatedTypeHelpers.cs
- ExitEventArgs.cs
- HtmlProps.cs
- MainMenu.cs
- SizeFConverter.cs
- TripleDES.cs
- ExpressionBuilder.cs
- BrowserCapabilitiesCodeGenerator.cs
- BaseCodeDomTreeGenerator.cs
- ContentPathSegment.cs
- DeclaredTypeElement.cs
- XPathCompileException.cs
- ToolStripSystemRenderer.cs
- HttpPostProtocolReflector.cs
- _AutoWebProxyScriptWrapper.cs
- PasswordTextContainer.cs
- SqlDependencyListener.cs
- HtmlValidatorAdapter.cs
- PropertyInfoSet.cs
- TreeViewBindingsEditorForm.cs
- BitmapImage.cs
- WebExceptionStatus.cs
- RandomNumberGenerator.cs
- UTF7Encoding.cs
- StreamProxy.cs
- SqlXmlStorage.cs
- XmlSchemaType.cs
- _UriSyntax.cs
- SoundPlayerAction.cs
- OciEnlistContext.cs
- Panel.cs
- DataGridViewCellConverter.cs
- SevenBitStream.cs
- ModulesEntry.cs
- NamedPipeAppDomainProtocolHandler.cs
- securitycriticaldataClass.cs
- Effect.cs
- ObjectDataSourceView.cs
- XmlDictionary.cs
- CodeDirectionExpression.cs
- SoapHeaders.cs
- AttributeProviderAttribute.cs
- SoapUnknownHeader.cs
- NavigationPropertyEmitter.cs
- EntityClientCacheEntry.cs
- EndpointInstanceProvider.cs
- SqlTrackingQuery.cs
- LeafCellTreeNode.cs
- NavigationFailedEventArgs.cs
- SortedDictionary.cs
- PeerUnsafeNativeCryptMethods.cs
- EntityDataSourceColumn.cs
- XamlParser.cs
- PasswordDeriveBytes.cs
- _Win32.cs
- Point4DConverter.cs
- DocumentSequence.cs
- UmAlQuraCalendar.cs
- HtmlTernaryTree.cs
- TabItemAutomationPeer.cs
- BufferBuilder.cs
- DataControlPagerLinkButton.cs
- XmlnsPrefixAttribute.cs
- HostingPreferredMapPath.cs
- CompareValidator.cs