Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / xsp / System / Web / UI / WebControls / AccessDataSource.cs / 1 / AccessDataSource.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Data; using System.Data.Common; using System.Data.OleDb; using System.Diagnostics; using System.Drawing; using System.Drawing.Design; using System.IO; using System.Security.Permissions; using System.Text; using System.Web.Caching; using System.Web.UI; ////// Allows a user to create a declarative connection to an Access database in a .aspx page. /// [ Designer("System.Web.UI.Design.WebControls.AccessDataSourceDesigner, " + AssemblyRef.SystemDesign), ToolboxBitmap(typeof(AccessDataSource)), WebSysDescription(SR.AccessDataSource_Description), WebSysDisplayName(SR.AccessDataSource_DisplayName) ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class AccessDataSource : SqlDataSource { private const string OleDbProviderName = "System.Data.OleDb"; private FileDataSourceCache _cache; private string _connectionString; private string _dataFile; private string _physicalDataFile; ////// Creates a new instance of AccessDataSource. /// public AccessDataSource() : base() { } ////// Creates a new instance of AccessDataSource with a specified connection string and select command. /// public AccessDataSource(string dataFile, string selectCommand) : base() { if (String.IsNullOrEmpty(dataFile)) { throw new ArgumentNullException("dataFile"); } DataFile = dataFile; SelectCommand = selectCommand; } ////// Specifies the cache settings for this data source. For the cache to /// work, the DataSourceMode must be set to DataSet. /// internal override DataSourceCache Cache { get { if (_cache == null) { _cache = new FileDataSourceCache(); } return _cache; } } ////// Gets the connection string for the AccessDataSource. This property is auto-generated and cannot be set. /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ] public override string ConnectionString { get { if (_connectionString == null) { _connectionString = CreateConnectionString(); } return _connectionString; } set { throw new InvalidOperationException(SR.GetString(SR.AccessDataSource_CannotSetConnectionString)); } } ////// The name of an Access database file. /// This property is not stored in ViewState. /// [ DefaultValue(""), Editor("System.Web.UI.Design.MdbDataFileEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)), UrlProperty(), WebCategory("Data"), WebSysDescription(SR.AccessDataSource_DataFile), ] public string DataFile { get { return (_dataFile == null) ? String.Empty : _dataFile; } set { if (DataFile != value) { _dataFile = value; _connectionString = null; _physicalDataFile = null; RaiseDataSourceChangedEvent(EventArgs.Empty); } } } ////// Gets the file data source cache object. /// private FileDataSourceCache FileDataSourceCache { get { FileDataSourceCache fileCache = Cache as FileDataSourceCache; Debug.Assert(fileCache != null, "Cache object should be a FileDataSourceCache"); return fileCache; } } ////// Gets the Physical path of the data file. /// private string PhysicalDataFile { get { if (_physicalDataFile == null) { _physicalDataFile = GetPhysicalDataFilePath(); } return _physicalDataFile; } } ////// Gets/sets the ADO.net managed provider name. This property is restricted to the OLE DB provider and cannot be set. /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ] public override string ProviderName { get { return OleDbProviderName; } set { throw new InvalidOperationException(SR.GetString(SR.AccessDataSource_CannotSetProvider, ID)); } } ////// A semi-colon delimited string indicating which databases to use for the dependency in the format "database1:table1;database2:table2". /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ] public override string SqlCacheDependency { get { throw new NotSupportedException(SR.GetString(SR.AccessDataSource_SqlCacheDependencyNotSupported, ID)); } set { throw new NotSupportedException(SR.GetString(SR.AccessDataSource_SqlCacheDependencyNotSupported, ID)); } } private void AddCacheFileDependency() { FileDataSourceCache.FileDependencies.Clear(); string filename = PhysicalDataFile; if (filename.Length > 0) { FileDataSourceCache.FileDependencies.Add(filename); } } ////// Creates a connection string for an Access database connection. /// The JET provider is automatically used, and the filename, username, password, and /// share mode are are set. /// private string CreateConnectionString() { return "Provider=Microsoft.Jet.OLEDB.4.0; Data Source=" + PhysicalDataFile; } ////// Creates a new AccessDataSourceView. /// protected override SqlDataSourceView CreateDataSourceView(string viewName) { return new AccessDataSourceView(this, viewName, Context); } protected override DbProviderFactory GetDbProviderFactory() { return OleDbFactory.Instance; } ////// Gets the appropriate mapped path for the DataFile. /// private string GetPhysicalDataFilePath() { string filename = DataFile; if (filename.Length == 0) { return null; } if (!System.Web.Util.UrlPath.IsAbsolutePhysicalPath(filename)) { // Root relative path if (DesignMode) { // This exception should never be thrown - the designer always maps paths // before using the runtime control. throw new NotSupportedException(SR.GetString(SR.AccessDataSource_DesignTimeRelativePathsNotSupported, ID)); } filename = Context.Request.MapPath(filename, AppRelativeTemplateSourceDirectory, true); } HttpRuntime.CheckFilePermission(filename, true); // We also need to check for path discovery permissions for the // file since the page developer will be able to see the physical // path in the ConnectionString property. if (!HttpRuntime.HasPathDiscoveryPermission(filename)) { throw new HttpException(SR.GetString(SR.AccessDataSource_NoPathDiscoveryPermission, HttpRuntime.GetSafePath(filename), ID)); } return filename; } ////// Saves data to the cache. /// internal override void SaveDataToCache(int startRowIndex, int maximumRows, object data, CacheDependency dependency) { AddCacheFileDependency(); base.SaveDataToCache(startRowIndex, maximumRows, data, dependency); } /*internal override void SaveTotalRowCountToCache(int totalRowCount) { AddCacheFileDependency(); base.SaveTotalRowCountToCache(totalRowCount); }*/ } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Data; using System.Data.Common; using System.Data.OleDb; using System.Diagnostics; using System.Drawing; using System.Drawing.Design; using System.IO; using System.Security.Permissions; using System.Text; using System.Web.Caching; using System.Web.UI; ////// Allows a user to create a declarative connection to an Access database in a .aspx page. /// [ Designer("System.Web.UI.Design.WebControls.AccessDataSourceDesigner, " + AssemblyRef.SystemDesign), ToolboxBitmap(typeof(AccessDataSource)), WebSysDescription(SR.AccessDataSource_Description), WebSysDisplayName(SR.AccessDataSource_DisplayName) ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class AccessDataSource : SqlDataSource { private const string OleDbProviderName = "System.Data.OleDb"; private FileDataSourceCache _cache; private string _connectionString; private string _dataFile; private string _physicalDataFile; ////// Creates a new instance of AccessDataSource. /// public AccessDataSource() : base() { } ////// Creates a new instance of AccessDataSource with a specified connection string and select command. /// public AccessDataSource(string dataFile, string selectCommand) : base() { if (String.IsNullOrEmpty(dataFile)) { throw new ArgumentNullException("dataFile"); } DataFile = dataFile; SelectCommand = selectCommand; } ////// Specifies the cache settings for this data source. For the cache to /// work, the DataSourceMode must be set to DataSet. /// internal override DataSourceCache Cache { get { if (_cache == null) { _cache = new FileDataSourceCache(); } return _cache; } } ////// Gets the connection string for the AccessDataSource. This property is auto-generated and cannot be set. /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ] public override string ConnectionString { get { if (_connectionString == null) { _connectionString = CreateConnectionString(); } return _connectionString; } set { throw new InvalidOperationException(SR.GetString(SR.AccessDataSource_CannotSetConnectionString)); } } ////// The name of an Access database file. /// This property is not stored in ViewState. /// [ DefaultValue(""), Editor("System.Web.UI.Design.MdbDataFileEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)), UrlProperty(), WebCategory("Data"), WebSysDescription(SR.AccessDataSource_DataFile), ] public string DataFile { get { return (_dataFile == null) ? String.Empty : _dataFile; } set { if (DataFile != value) { _dataFile = value; _connectionString = null; _physicalDataFile = null; RaiseDataSourceChangedEvent(EventArgs.Empty); } } } ////// Gets the file data source cache object. /// private FileDataSourceCache FileDataSourceCache { get { FileDataSourceCache fileCache = Cache as FileDataSourceCache; Debug.Assert(fileCache != null, "Cache object should be a FileDataSourceCache"); return fileCache; } } ////// Gets the Physical path of the data file. /// private string PhysicalDataFile { get { if (_physicalDataFile == null) { _physicalDataFile = GetPhysicalDataFilePath(); } return _physicalDataFile; } } ////// Gets/sets the ADO.net managed provider name. This property is restricted to the OLE DB provider and cannot be set. /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ] public override string ProviderName { get { return OleDbProviderName; } set { throw new InvalidOperationException(SR.GetString(SR.AccessDataSource_CannotSetProvider, ID)); } } ////// A semi-colon delimited string indicating which databases to use for the dependency in the format "database1:table1;database2:table2". /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ] public override string SqlCacheDependency { get { throw new NotSupportedException(SR.GetString(SR.AccessDataSource_SqlCacheDependencyNotSupported, ID)); } set { throw new NotSupportedException(SR.GetString(SR.AccessDataSource_SqlCacheDependencyNotSupported, ID)); } } private void AddCacheFileDependency() { FileDataSourceCache.FileDependencies.Clear(); string filename = PhysicalDataFile; if (filename.Length > 0) { FileDataSourceCache.FileDependencies.Add(filename); } } ////// Creates a connection string for an Access database connection. /// The JET provider is automatically used, and the filename, username, password, and /// share mode are are set. /// private string CreateConnectionString() { return "Provider=Microsoft.Jet.OLEDB.4.0; Data Source=" + PhysicalDataFile; } ////// Creates a new AccessDataSourceView. /// protected override SqlDataSourceView CreateDataSourceView(string viewName) { return new AccessDataSourceView(this, viewName, Context); } protected override DbProviderFactory GetDbProviderFactory() { return OleDbFactory.Instance; } ////// Gets the appropriate mapped path for the DataFile. /// private string GetPhysicalDataFilePath() { string filename = DataFile; if (filename.Length == 0) { return null; } if (!System.Web.Util.UrlPath.IsAbsolutePhysicalPath(filename)) { // Root relative path if (DesignMode) { // This exception should never be thrown - the designer always maps paths // before using the runtime control. throw new NotSupportedException(SR.GetString(SR.AccessDataSource_DesignTimeRelativePathsNotSupported, ID)); } filename = Context.Request.MapPath(filename, AppRelativeTemplateSourceDirectory, true); } HttpRuntime.CheckFilePermission(filename, true); // We also need to check for path discovery permissions for the // file since the page developer will be able to see the physical // path in the ConnectionString property. if (!HttpRuntime.HasPathDiscoveryPermission(filename)) { throw new HttpException(SR.GetString(SR.AccessDataSource_NoPathDiscoveryPermission, HttpRuntime.GetSafePath(filename), ID)); } return filename; } ////// Saves data to the cache. /// internal override void SaveDataToCache(int startRowIndex, int maximumRows, object data, CacheDependency dependency) { AddCacheFileDependency(); base.SaveDataToCache(startRowIndex, maximumRows, data, dependency); } /*internal override void SaveTotalRowCountToCache(int totalRowCount) { AddCacheFileDependency(); base.SaveTotalRowCountToCache(totalRowCount); }*/ } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
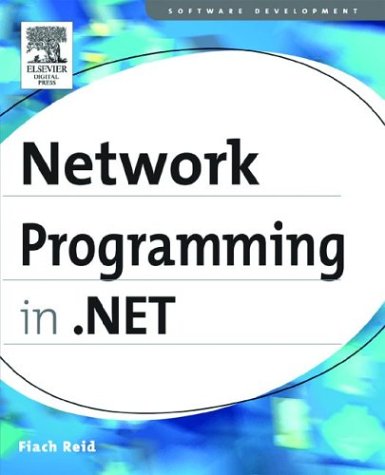
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StylusPointPropertyUnit.cs
- EmissiveMaterial.cs
- XslVisitor.cs
- DockProviderWrapper.cs
- XMLSyntaxException.cs
- MenuTracker.cs
- HttpResponseInternalBase.cs
- Decoder.cs
- ToolStripDropDownItemDesigner.cs
- Reference.cs
- _HelperAsyncResults.cs
- DesignerTransaction.cs
- ComplexBindingPropertiesAttribute.cs
- MetadataSource.cs
- TimestampInformation.cs
- TextEmbeddedObject.cs
- EncryptedData.cs
- ExtractedStateEntry.cs
- Parameter.cs
- XmlILStorageConverter.cs
- HtmlEmptyTagControlBuilder.cs
- Operators.cs
- GlyphRun.cs
- SqlCacheDependencySection.cs
- QueryStringParameter.cs
- BitArray.cs
- PhysicalAddress.cs
- QilReplaceVisitor.cs
- ConfigurationValidatorAttribute.cs
- ChannelServices.cs
- AssemblyNameProxy.cs
- Int32AnimationUsingKeyFrames.cs
- DataRelation.cs
- FieldBuilder.cs
- ProgressBarBrushConverter.cs
- ControlPager.cs
- SqlTypeSystemProvider.cs
- XmlComplianceUtil.cs
- DocumentViewerAutomationPeer.cs
- MethodBuilderInstantiation.cs
- SharedStatics.cs
- ArrayMergeHelper.cs
- ResourceLoader.cs
- IMembershipProvider.cs
- SizeConverter.cs
- DragCompletedEventArgs.cs
- SharedPersonalizationStateInfo.cs
- SafeLocalMemHandle.cs
- ButtonChrome.cs
- ScriptManagerProxy.cs
- MDIWindowDialog.cs
- CollectionBase.cs
- KeyedHashAlgorithm.cs
- ModelPropertyDescriptor.cs
- MessageSmuggler.cs
- CodeCastExpression.cs
- QueryCacheKey.cs
- MouseButtonEventArgs.cs
- DataBindingExpressionBuilder.cs
- CodeAccessSecurityEngine.cs
- NamespaceCollection.cs
- FtpRequestCacheValidator.cs
- PenContexts.cs
- ServiceModelSecurityTokenRequirement.cs
- XmlEventCache.cs
- PlatformCulture.cs
- Setter.cs
- NonClientArea.cs
- ValidationPropertyAttribute.cs
- WebBrowser.cs
- ElementNotEnabledException.cs
- DbDeleteCommandTree.cs
- WindowsFont.cs
- DSACryptoServiceProvider.cs
- CompiledQuery.cs
- Point3D.cs
- ProcessHost.cs
- WindowsListBox.cs
- InertiaTranslationBehavior.cs
- SmiMetaDataProperty.cs
- EnumValAlphaComparer.cs
- DbSourceCommand.cs
- WindowsFormsSynchronizationContext.cs
- DataGridViewTopLeftHeaderCell.cs
- ItemTypeToolStripMenuItem.cs
- ChildDocumentBlock.cs
- SingleObjectCollection.cs
- ElementNotEnabledException.cs
- shaperfactory.cs
- CollectionViewGroupRoot.cs
- MonikerBuilder.cs
- TextReader.cs
- WebPartEditorApplyVerb.cs
- Models.cs
- TextElementCollectionHelper.cs
- SHA256Cng.cs
- AxisAngleRotation3D.cs
- ImageListStreamer.cs
- XPathSelectionIterator.cs
- XMLUtil.cs