Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / xsp / System / Web / Configuration / ProfileSection.cs / 1 / ProfileSection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.IO; using System.Text; using System.Web.Util; using System.Security.Permissions; /**/ [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class ProfileSection : ConfigurationSection { private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propEnabled = new ConfigurationProperty("enabled", typeof(bool), true, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propDefaultProvider = new ConfigurationProperty("defaultProvider", typeof(string), "AspNetSqlProfileProvider", null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propProviders = new ConfigurationProperty("providers", typeof(ProviderSettingsCollection), null, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propProfile = new ConfigurationProperty("properties", typeof(RootProfilePropertySettingsCollection), null, ConfigurationPropertyOptions.IsDefaultCollection); private static readonly ConfigurationProperty _propInherits = new ConfigurationProperty("inherits", typeof(string), String.Empty, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propAutomaticSaveEnabled = new ConfigurationProperty("automaticSaveEnabled", typeof(bool), true, ConfigurationPropertyOptions.None); static ProfileSection() { // Property initialization _properties = new ConfigurationPropertyCollection(); _properties.Add(_propEnabled); _properties.Add(_propDefaultProvider); _properties.Add(_propProviders); _properties.Add(_propProfile); _properties.Add(_propInherits); _properties.Add(_propAutomaticSaveEnabled); } private long _recompilationHash; private bool _recompilationHashCached; internal long RecompilationHash { get { if (!_recompilationHashCached) { _recompilationHash = CalculateHash(); _recompilationHashCached = true; } return _recompilationHash; } } private long CalculateHash() { HashCodeCombiner hashCombiner = new HashCodeCombiner(); CalculateProfilePropertySettingsHash(PropertySettings, hashCombiner); if (PropertySettings != null) { foreach (ProfileGroupSettings pgs in PropertySettings.GroupSettings) { hashCombiner.AddObject(pgs.Name); CalculateProfilePropertySettingsHash(pgs.PropertySettings, hashCombiner); } } return hashCombiner.CombinedHash; } private void CalculateProfilePropertySettingsHash( ProfilePropertySettingsCollection settings, HashCodeCombiner hashCombiner) { foreach (ProfilePropertySettings pps in settings) { hashCombiner.AddObject(pps.Name); hashCombiner.AddObject(pps.Type); } } public ProfileSection() { } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } [ConfigurationProperty("automaticSaveEnabled", DefaultValue = true)] public bool AutomaticSaveEnabled { get { return (bool)base[_propAutomaticSaveEnabled]; } set { base[_propAutomaticSaveEnabled] = value; } } [ConfigurationProperty("enabled", DefaultValue = true)] public bool Enabled { get { return (bool)base[_propEnabled]; } set { base[_propEnabled] = value; } } [ConfigurationProperty("defaultProvider", DefaultValue = "AspNetSqlProfileProvider")] [StringValidator(MinLength = 1)] public string DefaultProvider { get { return (string)base[_propDefaultProvider]; } set { base[_propDefaultProvider] = value; } } [ConfigurationProperty("inherits", DefaultValue = "")] public string Inherits { get { return (string)base[_propInherits]; } set { base[_propInherits] = value; } } [ConfigurationProperty("providers")] public ProviderSettingsCollection Providers { get { return (ProviderSettingsCollection)base[_propProviders]; } } // not exposed to the API [ConfigurationProperty("properties")] public RootProfilePropertySettingsCollection PropertySettings { get { return (RootProfilePropertySettingsCollection)base[_propProfile]; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.IO; using System.Text; using System.Web.Util; using System.Security.Permissions; /**/ [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class ProfileSection : ConfigurationSection { private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propEnabled = new ConfigurationProperty("enabled", typeof(bool), true, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propDefaultProvider = new ConfigurationProperty("defaultProvider", typeof(string), "AspNetSqlProfileProvider", null, StdValidatorsAndConverters.NonEmptyStringValidator, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propProviders = new ConfigurationProperty("providers", typeof(ProviderSettingsCollection), null, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propProfile = new ConfigurationProperty("properties", typeof(RootProfilePropertySettingsCollection), null, ConfigurationPropertyOptions.IsDefaultCollection); private static readonly ConfigurationProperty _propInherits = new ConfigurationProperty("inherits", typeof(string), String.Empty, ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propAutomaticSaveEnabled = new ConfigurationProperty("automaticSaveEnabled", typeof(bool), true, ConfigurationPropertyOptions.None); static ProfileSection() { // Property initialization _properties = new ConfigurationPropertyCollection(); _properties.Add(_propEnabled); _properties.Add(_propDefaultProvider); _properties.Add(_propProviders); _properties.Add(_propProfile); _properties.Add(_propInherits); _properties.Add(_propAutomaticSaveEnabled); } private long _recompilationHash; private bool _recompilationHashCached; internal long RecompilationHash { get { if (!_recompilationHashCached) { _recompilationHash = CalculateHash(); _recompilationHashCached = true; } return _recompilationHash; } } private long CalculateHash() { HashCodeCombiner hashCombiner = new HashCodeCombiner(); CalculateProfilePropertySettingsHash(PropertySettings, hashCombiner); if (PropertySettings != null) { foreach (ProfileGroupSettings pgs in PropertySettings.GroupSettings) { hashCombiner.AddObject(pgs.Name); CalculateProfilePropertySettingsHash(pgs.PropertySettings, hashCombiner); } } return hashCombiner.CombinedHash; } private void CalculateProfilePropertySettingsHash( ProfilePropertySettingsCollection settings, HashCodeCombiner hashCombiner) { foreach (ProfilePropertySettings pps in settings) { hashCombiner.AddObject(pps.Name); hashCombiner.AddObject(pps.Type); } } public ProfileSection() { } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } [ConfigurationProperty("automaticSaveEnabled", DefaultValue = true)] public bool AutomaticSaveEnabled { get { return (bool)base[_propAutomaticSaveEnabled]; } set { base[_propAutomaticSaveEnabled] = value; } } [ConfigurationProperty("enabled", DefaultValue = true)] public bool Enabled { get { return (bool)base[_propEnabled]; } set { base[_propEnabled] = value; } } [ConfigurationProperty("defaultProvider", DefaultValue = "AspNetSqlProfileProvider")] [StringValidator(MinLength = 1)] public string DefaultProvider { get { return (string)base[_propDefaultProvider]; } set { base[_propDefaultProvider] = value; } } [ConfigurationProperty("inherits", DefaultValue = "")] public string Inherits { get { return (string)base[_propInherits]; } set { base[_propInherits] = value; } } [ConfigurationProperty("providers")] public ProviderSettingsCollection Providers { get { return (ProviderSettingsCollection)base[_propProviders]; } } // not exposed to the API [ConfigurationProperty("properties")] public RootProfilePropertySettingsCollection PropertySettings { get { return (RootProfilePropertySettingsCollection)base[_propProfile]; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
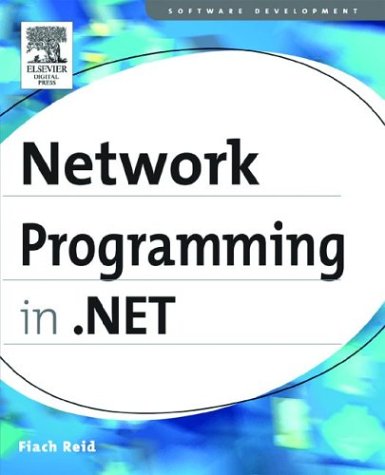
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ApplicationInfo.cs
- NamedPipeConnectionPool.cs
- CodeAttributeArgument.cs
- ExecutionEngineException.cs
- DataGridViewRowsRemovedEventArgs.cs
- InertiaTranslationBehavior.cs
- DeflateEmulationStream.cs
- control.ime.cs
- SoundPlayer.cs
- JoinGraph.cs
- TreeViewCancelEvent.cs
- DesignerSerializationOptionsAttribute.cs
- BitmapFrame.cs
- ReplyChannelAcceptor.cs
- NavigationWindow.cs
- EntityDataSourceDesignerHelper.cs
- WindowsListView.cs
- PropertyOverridesDialog.cs
- recordstatefactory.cs
- CharacterMetrics.cs
- HtmlForm.cs
- VerificationException.cs
- HtmlInputSubmit.cs
- SqlDataSourceEnumerator.cs
- DataSourceView.cs
- UserPersonalizationStateInfo.cs
- ReadOnlyCollectionBase.cs
- OdbcDataAdapter.cs
- DataBindEngine.cs
- ResourceManagerWrapper.cs
- MsmqIntegrationProcessProtocolHandler.cs
- XslTransformFileEditor.cs
- Guid.cs
- DataRelationCollection.cs
- ValidationErrorCollection.cs
- ExpressionBuilder.cs
- HttpDigestClientCredential.cs
- AbstractExpressions.cs
- SqlDataReader.cs
- OutputBuffer.cs
- ImmComposition.cs
- bidPrivateBase.cs
- ControlPropertyNameConverter.cs
- Fonts.cs
- CapabilitiesAssignment.cs
- SubqueryRules.cs
- ListControlStringCollectionEditor.cs
- SafeRightsManagementPubHandle.cs
- SafeNativeMethods.cs
- DataGridViewCellPaintingEventArgs.cs
- PreservationFileWriter.cs
- SchemaMapping.cs
- StatusStrip.cs
- Geometry.cs
- DesignTimeDataBinding.cs
- SharedDp.cs
- TypeContext.cs
- MinMaxParagraphWidth.cs
- MenuBase.cs
- GatewayDefinition.cs
- EmptyReadOnlyDictionaryInternal.cs
- Win32Exception.cs
- OrderablePartitioner.cs
- RadioButtonRenderer.cs
- SqlGenericUtil.cs
- IssuedTokenClientBehaviorsElement.cs
- ObjectStorage.cs
- sortedlist.cs
- EdmToObjectNamespaceMap.cs
- CollectionBuilder.cs
- ProviderSettings.cs
- DataGridViewAdvancedBorderStyle.cs
- ParameterSubsegment.cs
- CodeNamespaceImport.cs
- TypeExtensionConverter.cs
- MimeMapping.cs
- SerialErrors.cs
- SvcMapFileSerializer.cs
- NavigationPropertyEmitter.cs
- CodeExporter.cs
- ReadOnlyState.cs
- DependencyPropertyAttribute.cs
- SafeSystemMetrics.cs
- GraphicsPath.cs
- QueryMath.cs
- PageParser.cs
- IndividualDeviceConfig.cs
- Parser.cs
- StateBag.cs
- SelectionBorderGlyph.cs
- StickyNoteHelper.cs
- DefaultAsyncDataDispatcher.cs
- LineServicesRun.cs
- GacUtil.cs
- HwndHostAutomationPeer.cs
- PlanCompilerUtil.cs
- StrokeCollection.cs
- SignatureHelper.cs
- WaitHandle.cs
- NameValueSectionHandler.cs