Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / clr / src / BCL / System / Security / Policy / ZoneMembershipCondition.cs / 1 / ZoneMembershipCondition.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // ZoneMembershipCondition.cs // // Implementation of membership condition for zones // namespace System.Security.Policy { using System; using SecurityManager = System.Security.SecurityManager; using PermissionSet = System.Security.PermissionSet; using SecurityElement = System.Security.SecurityElement; using System.Collections; using System.Globalization; [Serializable] [System.Runtime.InteropServices.ComVisible(true)] sealed public class ZoneMembershipCondition : IMembershipCondition, IConstantMembershipCondition, IReportMatchMembershipCondition { //------------------------------------------------------ // // PRIVATE CONSTANTS // //----------------------------------------------------- private static readonly String[] s_names = {"MyComputer", "Intranet", "Trusted", "Internet", "Untrusted"}; //----------------------------------------------------- // // PRIVATE STATE DATA // //----------------------------------------------------- private SecurityZone m_zone; private SecurityElement m_element; //------------------------------------------------------ // // PUBLIC CONSTRUCTORS // //----------------------------------------------------- internal ZoneMembershipCondition() { m_zone = SecurityZone.NoZone; } public ZoneMembershipCondition( SecurityZone zone ) { VerifyZone( zone ); this.SecurityZone = zone; } //------------------------------------------------------ // // PUBLIC ACCESSOR METHODS // //------------------------------------------------------ public SecurityZone SecurityZone { set { VerifyZone( value ); m_zone = value; } get { if (m_zone == SecurityZone.NoZone && m_element != null) ParseZone(); return m_zone; } } //----------------------------------------------------- // // PRIVATE AND PROTECTED HELPERS FOR ACCESSORS AND CONSTRUCTORS // //------------------------------------------------------ private static void VerifyZone( SecurityZone zone ) { if (zone < SecurityZone.MyComputer || zone > SecurityZone.Untrusted) { throw new ArgumentException( Environment.GetResourceString( "Argument_IllegalZone" ) ); } } //----------------------------------------------------- // // IMEMBERSHIPCONDITION IMPLEMENTATION // //----------------------------------------------------- public bool Check( Evidence evidence ) { object usedEvidence = null; return (this as IReportMatchMembershipCondition).Check(evidence, out usedEvidence); } bool IReportMatchMembershipCondition.Check(Evidence evidence, out object usedEvidence) { usedEvidence = null; if (evidence == null) return false; IEnumerator enumerator = evidence.GetHostEnumerator(); while (enumerator.MoveNext()) { Zone zone = enumerator.Current as Zone; if (zone != null) { if (m_zone == SecurityZone.NoZone && m_element != null) ParseZone(); if (zone.SecurityZone == m_zone) { usedEvidence = zone; return true; } } } return false; } public IMembershipCondition Copy() { if (m_zone == SecurityZone.NoZone && m_element != null) ParseZone(); return new ZoneMembershipCondition( m_zone ); } public SecurityElement ToXml() { return ToXml( null ); } public void FromXml( SecurityElement e ) { FromXml( e, null ); } public SecurityElement ToXml( PolicyLevel level ) { if (m_zone == SecurityZone.NoZone && m_element != null) ParseZone(); SecurityElement root = new SecurityElement( "IMembershipCondition" ); System.Security.Util.XMLUtil.AddClassAttribute( root, this.GetType(), "System.Security.Policy.ZoneMembershipCondition" ); // If you hit this assert then most likely you are trying to change the name of this class. // This is ok as long as you change the hard coded string above and change the assert below. BCLDebug.Assert( this.GetType().FullName.Equals( "System.Security.Policy.ZoneMembershipCondition" ), "Class name changed!" ); root.AddAttribute( "version", "1" ); if (m_zone != SecurityZone.NoZone) root.AddAttribute( "Zone", Enum.GetName( typeof( SecurityZone ), m_zone ) ); return root; } public void FromXml( SecurityElement e, PolicyLevel level ) { if (e == null) throw new ArgumentNullException("e"); if (!e.Tag.Equals( "IMembershipCondition" )) { throw new ArgumentException( Environment.GetResourceString( "Argument_MembershipConditionElement" ) ); } lock (this) { m_zone = SecurityZone.NoZone; m_element = e; } } private void ParseZone() { lock (this) { if (m_element == null) return; String eZone = m_element.Attribute( "Zone" ); m_zone = SecurityZone.NoZone; if (eZone != null) { m_zone = (SecurityZone)Enum.Parse( typeof( SecurityZone ), eZone ); } else { throw new ArgumentException( Environment.GetResourceString( "Argument_ZoneCannotBeNull" ) ); } VerifyZone(m_zone); m_element = null; } } public override bool Equals( Object o ) { ZoneMembershipCondition that = (o as ZoneMembershipCondition); if (that != null) { if (this.m_zone == SecurityZone.NoZone && this.m_element != null) this.ParseZone(); if (that.m_zone == SecurityZone.NoZone && that.m_element != null) that.ParseZone(); if(this.m_zone == that.m_zone) { return true; } } return false; } public override int GetHashCode() { if (this.m_zone == SecurityZone.NoZone && this.m_element != null) this.ParseZone(); return (int)m_zone; } public override String ToString() { if (m_zone == SecurityZone.NoZone && m_element != null) ParseZone(); return String.Format( CultureInfo.CurrentCulture, Environment.GetResourceString( "Zone_ToString" ), s_names[(int)m_zone] ); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // ZoneMembershipCondition.cs // // Implementation of membership condition for zones // namespace System.Security.Policy { using System; using SecurityManager = System.Security.SecurityManager; using PermissionSet = System.Security.PermissionSet; using SecurityElement = System.Security.SecurityElement; using System.Collections; using System.Globalization; [Serializable] [System.Runtime.InteropServices.ComVisible(true)] sealed public class ZoneMembershipCondition : IMembershipCondition, IConstantMembershipCondition, IReportMatchMembershipCondition { //------------------------------------------------------ // // PRIVATE CONSTANTS // //----------------------------------------------------- private static readonly String[] s_names = {"MyComputer", "Intranet", "Trusted", "Internet", "Untrusted"}; //----------------------------------------------------- // // PRIVATE STATE DATA // //----------------------------------------------------- private SecurityZone m_zone; private SecurityElement m_element; //------------------------------------------------------ // // PUBLIC CONSTRUCTORS // //----------------------------------------------------- internal ZoneMembershipCondition() { m_zone = SecurityZone.NoZone; } public ZoneMembershipCondition( SecurityZone zone ) { VerifyZone( zone ); this.SecurityZone = zone; } //------------------------------------------------------ // // PUBLIC ACCESSOR METHODS // //------------------------------------------------------ public SecurityZone SecurityZone { set { VerifyZone( value ); m_zone = value; } get { if (m_zone == SecurityZone.NoZone && m_element != null) ParseZone(); return m_zone; } } //----------------------------------------------------- // // PRIVATE AND PROTECTED HELPERS FOR ACCESSORS AND CONSTRUCTORS // //------------------------------------------------------ private static void VerifyZone( SecurityZone zone ) { if (zone < SecurityZone.MyComputer || zone > SecurityZone.Untrusted) { throw new ArgumentException( Environment.GetResourceString( "Argument_IllegalZone" ) ); } } //----------------------------------------------------- // // IMEMBERSHIPCONDITION IMPLEMENTATION // //----------------------------------------------------- public bool Check( Evidence evidence ) { object usedEvidence = null; return (this as IReportMatchMembershipCondition).Check(evidence, out usedEvidence); } bool IReportMatchMembershipCondition.Check(Evidence evidence, out object usedEvidence) { usedEvidence = null; if (evidence == null) return false; IEnumerator enumerator = evidence.GetHostEnumerator(); while (enumerator.MoveNext()) { Zone zone = enumerator.Current as Zone; if (zone != null) { if (m_zone == SecurityZone.NoZone && m_element != null) ParseZone(); if (zone.SecurityZone == m_zone) { usedEvidence = zone; return true; } } } return false; } public IMembershipCondition Copy() { if (m_zone == SecurityZone.NoZone && m_element != null) ParseZone(); return new ZoneMembershipCondition( m_zone ); } public SecurityElement ToXml() { return ToXml( null ); } public void FromXml( SecurityElement e ) { FromXml( e, null ); } public SecurityElement ToXml( PolicyLevel level ) { if (m_zone == SecurityZone.NoZone && m_element != null) ParseZone(); SecurityElement root = new SecurityElement( "IMembershipCondition" ); System.Security.Util.XMLUtil.AddClassAttribute( root, this.GetType(), "System.Security.Policy.ZoneMembershipCondition" ); // If you hit this assert then most likely you are trying to change the name of this class. // This is ok as long as you change the hard coded string above and change the assert below. BCLDebug.Assert( this.GetType().FullName.Equals( "System.Security.Policy.ZoneMembershipCondition" ), "Class name changed!" ); root.AddAttribute( "version", "1" ); if (m_zone != SecurityZone.NoZone) root.AddAttribute( "Zone", Enum.GetName( typeof( SecurityZone ), m_zone ) ); return root; } public void FromXml( SecurityElement e, PolicyLevel level ) { if (e == null) throw new ArgumentNullException("e"); if (!e.Tag.Equals( "IMembershipCondition" )) { throw new ArgumentException( Environment.GetResourceString( "Argument_MembershipConditionElement" ) ); } lock (this) { m_zone = SecurityZone.NoZone; m_element = e; } } private void ParseZone() { lock (this) { if (m_element == null) return; String eZone = m_element.Attribute( "Zone" ); m_zone = SecurityZone.NoZone; if (eZone != null) { m_zone = (SecurityZone)Enum.Parse( typeof( SecurityZone ), eZone ); } else { throw new ArgumentException( Environment.GetResourceString( "Argument_ZoneCannotBeNull" ) ); } VerifyZone(m_zone); m_element = null; } } public override bool Equals( Object o ) { ZoneMembershipCondition that = (o as ZoneMembershipCondition); if (that != null) { if (this.m_zone == SecurityZone.NoZone && this.m_element != null) this.ParseZone(); if (that.m_zone == SecurityZone.NoZone && that.m_element != null) that.ParseZone(); if(this.m_zone == that.m_zone) { return true; } } return false; } public override int GetHashCode() { if (this.m_zone == SecurityZone.NoZone && this.m_element != null) this.ParseZone(); return (int)m_zone; } public override String ToString() { if (m_zone == SecurityZone.NoZone && m_element != null) ParseZone(); return String.Format( CultureInfo.CurrentCulture, Environment.GetResourceString( "Zone_ToString" ), s_names[(int)m_zone] ); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
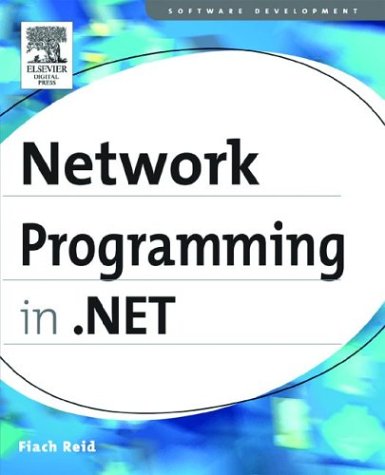
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GridItemCollection.cs
- ConfigXmlComment.cs
- OrderingQueryOperator.cs
- ObjectStateManagerMetadata.cs
- EntityRecordInfo.cs
- PolyBezierSegmentFigureLogic.cs
- FunctionQuery.cs
- JapaneseCalendar.cs
- PreProcessInputEventArgs.cs
- DragEvent.cs
- BitStream.cs
- ExpressionBindingsDialog.cs
- newinstructionaction.cs
- XPathBinder.cs
- CompilationAssemblyInstallComponent.cs
- RegexTypeEditor.cs
- _NTAuthentication.cs
- DataQuery.cs
- TablePattern.cs
- LoginStatusDesigner.cs
- AuthStoreRoleProvider.cs
- MissingMemberException.cs
- CodeGroup.cs
- odbcmetadatafactory.cs
- TextViewDesigner.cs
- Bidi.cs
- MemoryFailPoint.cs
- AggregationMinMaxHelpers.cs
- ApplicationProxyInternal.cs
- LocatorPartList.cs
- AspProxy.cs
- TimeZone.cs
- HtmlElementEventArgs.cs
- ThaiBuddhistCalendar.cs
- TTSEngineTypes.cs
- GenericTypeParameterBuilder.cs
- Section.cs
- MarginCollapsingState.cs
- Translator.cs
- GraphicsContext.cs
- MemoryFailPoint.cs
- PerCallInstanceContextProvider.cs
- SubMenuStyle.cs
- LeafCellTreeNode.cs
- RoleGroupCollection.cs
- HandleCollector.cs
- Underline.cs
- Marshal.cs
- UnsafeNativeMethods.cs
- LinqDataSourceDisposeEventArgs.cs
- IWorkflowDebuggerService.cs
- BuildProviderCollection.cs
- FilterableData.cs
- DataGridLength.cs
- KnownBoxes.cs
- XmlToDatasetMap.cs
- SortQuery.cs
- loginstatus.cs
- HttpCookieCollection.cs
- TemplateControl.cs
- TextDecoration.cs
- AspCompat.cs
- CompareInfo.cs
- PropertyManager.cs
- Context.cs
- PriorityQueue.cs
- HostVisual.cs
- DocumentSequenceHighlightLayer.cs
- NullableBoolConverter.cs
- SqlRewriteScalarSubqueries.cs
- WebPartConnectionsEventArgs.cs
- Sequence.cs
- DecoderExceptionFallback.cs
- DataSourceProvider.cs
- TextEndOfLine.cs
- NoneExcludedImageIndexConverter.cs
- Pen.cs
- ADMembershipUser.cs
- TimeoutValidationAttribute.cs
- EntityDataSource.cs
- EdmItemCollection.cs
- OneOf.cs
- CallbackException.cs
- DESCryptoServiceProvider.cs
- XmlElementAttributes.cs
- ContentPropertyAttribute.cs
- TypeConverterBase.cs
- PatternMatcher.cs
- InfoCardRSAOAEPKeyExchangeDeformatter.cs
- TypeResolvingOptionsAttribute.cs
- SizeIndependentAnimationStorage.cs
- CollectionContainer.cs
- ThemeInfoAttribute.cs
- InstanceContextManager.cs
- DataServiceHostWrapper.cs
- CancellationTokenSource.cs
- Point4D.cs
- DataControlFieldCollection.cs
- SQLBytes.cs
- ImageDrawing.cs