Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / WinForms / Managed / System / WinForms / Layout / ArrangedElementCollection.cs / 1 / ArrangedElementCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms.Layout { using System.Collections; using System.Security.Permissions; ///public class ArrangedElementCollection : IList { internal static ArrangedElementCollection Empty = new ArrangedElementCollection(0); // We wrap an ArrayList rather than inherit from CollectionBase because we // do not want to break binary compatibility with ControlCollection. private ArrayList _innerList; // Internal constructor prevents externals from getting a hold of one of these. // We'll open this up in Orcas. internal ArrangedElementCollection() { _innerList = new ArrayList(4); } internal ArrangedElementCollection(ArrayList innerList) { _innerList = innerList; } private ArrangedElementCollection(int size) { _innerList = new ArrayList(size); } internal ArrayList InnerList { get { return _innerList; } } internal virtual IArrangedElement this[int index] { get { return (IArrangedElement) InnerList[index]; } } /// public override bool Equals(object obj) { ArrangedElementCollection other = obj as ArrangedElementCollection; if (other == null || Count != other.Count) { return false; } for (int i = 0; i < Count; i++) { if (this.InnerList[i] != other.InnerList[i]) { return false; } } return true; } // Required if you override Equals. /// public override int GetHashCode() { return base.GetHashCode(); } // Repositions a element in this list. internal void MoveElement(IArrangedElement element, int fromIndex, int toIndex) { int delta = toIndex - fromIndex; switch (delta) { case -1: case 1: // simple swap this.InnerList[fromIndex] = this.InnerList[toIndex]; break; default: int start = 0; int dest = 0; // which direction are we moving? if (delta > 0) { // shift down by the delta to open the new spot start = fromIndex + 1; dest = fromIndex; } else { // shift up by the delta to open the new spot start = toIndex; dest = toIndex + 1; // make it positive delta = -delta; } Copy(this, start, this, dest, delta); break; } this.InnerList[toIndex] = element; } private static void Copy (ArrangedElementCollection sourceList, int sourceIndex, ArrangedElementCollection destinationList, int destinationIndex, int length) { if(sourceIndex < destinationIndex) { // We need to copy from the back forward to prevent overwrite if source and // destination lists are the same, so we need to flip the source/dest indices // to point at the end of the spans to be copied. sourceIndex = sourceIndex + length; destinationIndex = destinationIndex + length; for(;length > 0; length--) { destinationList.InnerList[--destinationIndex] = sourceList.InnerList[--sourceIndex]; } } else { for(;length > 0; length--) { destinationList.InnerList[destinationIndex++] = sourceList.InnerList[sourceIndex++]; } } } #region IList Members void IList.Clear() { InnerList.Clear(); } bool IList.IsFixedSize { get { return InnerList.IsFixedSize; }} bool IList.Contains(object value) { return InnerList.Contains(value); } /// public virtual bool IsReadOnly { get { return InnerList.IsReadOnly; }} void IList.RemoveAt(int index) { InnerList.RemoveAt(index); } void IList.Remove(object value) { InnerList.Remove(value); } int IList.Add(object value) { return InnerList.Add(value); } int IList.IndexOf(object value) { return InnerList.IndexOf(value); } void IList.Insert(int index, object value) { throw new NotSupportedException(); /* InnerList.Insert(index, value); */ } /// /// object IList.this[int index] { get { return InnerList[index]; } set { throw new NotSupportedException(); } } #endregion #region ICollection Members /// public virtual int Count { get { return InnerList.Count; }} object ICollection.SyncRoot { get { return InnerList.SyncRoot; }} /// public void CopyTo(Array array, int index) { InnerList.CopyTo(array, index); } bool ICollection.IsSynchronized { get { return InnerList.IsSynchronized; }} #endregion #region IEnumerable Members /// public virtual IEnumerator GetEnumerator() { return InnerList.GetEnumerator(); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms.Layout { using System.Collections; using System.Security.Permissions; ///public class ArrangedElementCollection : IList { internal static ArrangedElementCollection Empty = new ArrangedElementCollection(0); // We wrap an ArrayList rather than inherit from CollectionBase because we // do not want to break binary compatibility with ControlCollection. private ArrayList _innerList; // Internal constructor prevents externals from getting a hold of one of these. // We'll open this up in Orcas. internal ArrangedElementCollection() { _innerList = new ArrayList(4); } internal ArrangedElementCollection(ArrayList innerList) { _innerList = innerList; } private ArrangedElementCollection(int size) { _innerList = new ArrayList(size); } internal ArrayList InnerList { get { return _innerList; } } internal virtual IArrangedElement this[int index] { get { return (IArrangedElement) InnerList[index]; } } /// public override bool Equals(object obj) { ArrangedElementCollection other = obj as ArrangedElementCollection; if (other == null || Count != other.Count) { return false; } for (int i = 0; i < Count; i++) { if (this.InnerList[i] != other.InnerList[i]) { return false; } } return true; } // Required if you override Equals. /// public override int GetHashCode() { return base.GetHashCode(); } // Repositions a element in this list. internal void MoveElement(IArrangedElement element, int fromIndex, int toIndex) { int delta = toIndex - fromIndex; switch (delta) { case -1: case 1: // simple swap this.InnerList[fromIndex] = this.InnerList[toIndex]; break; default: int start = 0; int dest = 0; // which direction are we moving? if (delta > 0) { // shift down by the delta to open the new spot start = fromIndex + 1; dest = fromIndex; } else { // shift up by the delta to open the new spot start = toIndex; dest = toIndex + 1; // make it positive delta = -delta; } Copy(this, start, this, dest, delta); break; } this.InnerList[toIndex] = element; } private static void Copy (ArrangedElementCollection sourceList, int sourceIndex, ArrangedElementCollection destinationList, int destinationIndex, int length) { if(sourceIndex < destinationIndex) { // We need to copy from the back forward to prevent overwrite if source and // destination lists are the same, so we need to flip the source/dest indices // to point at the end of the spans to be copied. sourceIndex = sourceIndex + length; destinationIndex = destinationIndex + length; for(;length > 0; length--) { destinationList.InnerList[--destinationIndex] = sourceList.InnerList[--sourceIndex]; } } else { for(;length > 0; length--) { destinationList.InnerList[destinationIndex++] = sourceList.InnerList[sourceIndex++]; } } } #region IList Members void IList.Clear() { InnerList.Clear(); } bool IList.IsFixedSize { get { return InnerList.IsFixedSize; }} bool IList.Contains(object value) { return InnerList.Contains(value); } /// public virtual bool IsReadOnly { get { return InnerList.IsReadOnly; }} void IList.RemoveAt(int index) { InnerList.RemoveAt(index); } void IList.Remove(object value) { InnerList.Remove(value); } int IList.Add(object value) { return InnerList.Add(value); } int IList.IndexOf(object value) { return InnerList.IndexOf(value); } void IList.Insert(int index, object value) { throw new NotSupportedException(); /* InnerList.Insert(index, value); */ } /// /// object IList.this[int index] { get { return InnerList[index]; } set { throw new NotSupportedException(); } } #endregion #region ICollection Members /// public virtual int Count { get { return InnerList.Count; }} object ICollection.SyncRoot { get { return InnerList.SyncRoot; }} /// public void CopyTo(Array array, int index) { InnerList.CopyTo(array, index); } bool ICollection.IsSynchronized { get { return InnerList.IsSynchronized; }} #endregion #region IEnumerable Members /// public virtual IEnumerator GetEnumerator() { return InnerList.GetEnumerator(); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
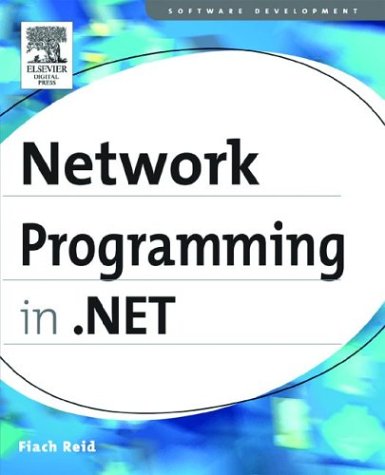
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Visual3D.cs
- FusionWrap.cs
- EventWaitHandle.cs
- EntryIndex.cs
- GcHandle.cs
- AspNetSynchronizationContext.cs
- DocumentApplicationState.cs
- ScrollProperties.cs
- Rijndael.cs
- LocalizationComments.cs
- ContextQuery.cs
- PropertyManager.cs
- VirtualPathUtility.cs
- RoleManagerEventArgs.cs
- DispatcherProcessingDisabled.cs
- StyleTypedPropertyAttribute.cs
- EventRouteFactory.cs
- LexicalChunk.cs
- FileFormatException.cs
- Visual.cs
- SoapEnumAttribute.cs
- ValidationEventArgs.cs
- EpmContentDeSerializerBase.cs
- JavaScriptObjectDeserializer.cs
- ToolStripDesignerAvailabilityAttribute.cs
- GestureRecognitionResult.cs
- TextProperties.cs
- CodeNamespaceCollection.cs
- SmtpReplyReader.cs
- DataGridViewCell.cs
- GroupBox.cs
- Root.cs
- MasterPage.cs
- DisplayInformation.cs
- CellParaClient.cs
- EdmComplexPropertyAttribute.cs
- UdpConstants.cs
- QueryStringParameter.cs
- Hyperlink.cs
- GlyphRunDrawing.cs
- CompiledXpathExpr.cs
- AlignmentYValidation.cs
- ExtenderProvidedPropertyAttribute.cs
- ToolbarAUtomationPeer.cs
- Operand.cs
- JoinCqlBlock.cs
- URLIdentityPermission.cs
- RankException.cs
- AmbientValueAttribute.cs
- QueryCursorEventArgs.cs
- XslCompiledTransform.cs
- ReleaseInstanceMode.cs
- Page.cs
- AssemblyAttributesGoHere.cs
- HtmlInputFile.cs
- CacheChildrenQuery.cs
- AsyncCompletedEventArgs.cs
- DocumentPaginator.cs
- RelationshipSet.cs
- XamlPoint3DCollectionSerializer.cs
- AddInServer.cs
- PropertyEmitter.cs
- ClientFormsIdentity.cs
- GroupItem.cs
- _MultipleConnectAsync.cs
- CompilerState.cs
- RequestQueue.cs
- DocumentPageView.cs
- BuildManagerHost.cs
- CodeAttributeArgument.cs
- TemplateControlCodeDomTreeGenerator.cs
- PipeException.cs
- TextBoxBase.cs
- InstanceCreationEditor.cs
- DataGridClipboardCellContent.cs
- NetworkInformationException.cs
- CustomErrorsSection.cs
- XmlNodeComparer.cs
- ContractBase.cs
- DocumentReferenceCollection.cs
- SpecialFolderEnumConverter.cs
- ResetableIterator.cs
- SafeEventLogWriteHandle.cs
- WhereQueryOperator.cs
- IdleTimeoutMonitor.cs
- PackageDigitalSignature.cs
- LinkedList.cs
- ResourceReader.cs
- DataServiceExpressionVisitor.cs
- WmlSelectionListAdapter.cs
- HttpListenerResponse.cs
- MasterPage.cs
- parserscommon.cs
- URLMembershipCondition.cs
- WebPartConnectionsConfigureVerb.cs
- StringStorage.cs
- FileDialog.cs
- XmlSchemaRedefine.cs
- ReferenceConverter.cs
- DateBoldEvent.cs