Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / WinForms / Managed / System / WinForms / DataStreamFromComStream.cs / 1 / DataStreamFromComStream.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Runtime.InteropServices; using System.Diagnostics; using System; using System.IO; ////// /// /// internal class DataStreamFromComStream : Stream { private UnsafeNativeMethods.IStream comStream; public DataStreamFromComStream(UnsafeNativeMethods.IStream comStream) : base() { this.comStream = comStream; } public override long Position { get { return Seek(0, SeekOrigin.Current); } set { Seek(value, SeekOrigin.Begin); } } public override bool CanWrite { get { return true; } } public override bool CanSeek { get { return true; } } public override bool CanRead { get { return true; } } public override long Length { get { long curPos = this.Position; long endPos = Seek(0, SeekOrigin.End); this.Position = curPos; return endPos - curPos; } } /* private void _NotImpl(string message) { NotSupportedException ex = new NotSupportedException(message, new ExternalException(SR.GetString(SR.ExternalException), NativeMethods.E_NOTIMPL)); throw ex; } */ private unsafe int _Read(void* handle, int bytes) { return comStream.Read((IntPtr)handle, bytes); } private unsafe int _Write(void* handle, int bytes) { return comStream.Write((IntPtr)handle, bytes); } public override void Flush() { } public unsafe override int Read(byte[] buffer, int index, int count) { int bytesRead = 0; if (count > 0 && index >= 0 && (count + index) <= buffer.Length) { fixed (byte* ch = buffer) { bytesRead = _Read((void*)(ch + index), count); } } return bytesRead; } public override void SetLength(long value) { comStream.SetSize(value); } public override long Seek(long offset, SeekOrigin origin) { return comStream.Seek(offset, (int)origin); } public unsafe override void Write(byte[] buffer, int index, int count) { int bytesWritten = 0; if (count > 0 && index >= 0 && (count + index) <= buffer.Length) { try { fixed (byte* b = buffer) { bytesWritten = _Write((void*)(b + index), count); } } catch { } } if (bytesWritten < count) { throw new IOException(SR.GetString(SR.DataStreamWrite)); } } protected override void Dispose(bool disposing) { try { if (disposing && comStream != null) { try { comStream.Commit(NativeMethods.STGC_DEFAULT); } catch(Exception) { } } // Can't release a COM stream from the finalizer thread. comStream = null; } finally { base.Dispose(disposing); } } ~DataStreamFromComStream() { Dispose(false); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Runtime.InteropServices; using System.Diagnostics; using System; using System.IO; ////// /// /// internal class DataStreamFromComStream : Stream { private UnsafeNativeMethods.IStream comStream; public DataStreamFromComStream(UnsafeNativeMethods.IStream comStream) : base() { this.comStream = comStream; } public override long Position { get { return Seek(0, SeekOrigin.Current); } set { Seek(value, SeekOrigin.Begin); } } public override bool CanWrite { get { return true; } } public override bool CanSeek { get { return true; } } public override bool CanRead { get { return true; } } public override long Length { get { long curPos = this.Position; long endPos = Seek(0, SeekOrigin.End); this.Position = curPos; return endPos - curPos; } } /* private void _NotImpl(string message) { NotSupportedException ex = new NotSupportedException(message, new ExternalException(SR.GetString(SR.ExternalException), NativeMethods.E_NOTIMPL)); throw ex; } */ private unsafe int _Read(void* handle, int bytes) { return comStream.Read((IntPtr)handle, bytes); } private unsafe int _Write(void* handle, int bytes) { return comStream.Write((IntPtr)handle, bytes); } public override void Flush() { } public unsafe override int Read(byte[] buffer, int index, int count) { int bytesRead = 0; if (count > 0 && index >= 0 && (count + index) <= buffer.Length) { fixed (byte* ch = buffer) { bytesRead = _Read((void*)(ch + index), count); } } return bytesRead; } public override void SetLength(long value) { comStream.SetSize(value); } public override long Seek(long offset, SeekOrigin origin) { return comStream.Seek(offset, (int)origin); } public unsafe override void Write(byte[] buffer, int index, int count) { int bytesWritten = 0; if (count > 0 && index >= 0 && (count + index) <= buffer.Length) { try { fixed (byte* b = buffer) { bytesWritten = _Write((void*)(b + index), count); } } catch { } } if (bytesWritten < count) { throw new IOException(SR.GetString(SR.DataStreamWrite)); } } protected override void Dispose(bool disposing) { try { if (disposing && comStream != null) { try { comStream.Commit(NativeMethods.STGC_DEFAULT); } catch(Exception) { } } // Can't release a COM stream from the finalizer thread. comStream = null; } finally { base.Dispose(disposing); } } ~DataStreamFromComStream() { Dispose(false); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
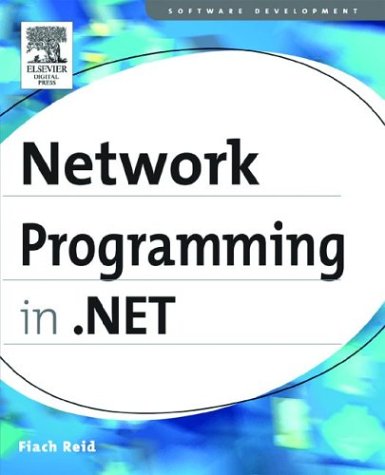
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ManagementExtension.cs
- SQLStringStorage.cs
- RelationshipManager.cs
- SocketElement.cs
- DoubleCollection.cs
- DocumentViewer.cs
- IncrementalReadDecoders.cs
- DragAssistanceManager.cs
- TreeNodeStyleCollection.cs
- SoapHttpTransportImporter.cs
- CloseCollectionAsyncResult.cs
- SecurityKeyIdentifier.cs
- PeerReferralPolicy.cs
- XmlSchemas.cs
- DataBinding.cs
- WindowsListViewItemStartMenu.cs
- WindowsTooltip.cs
- SetIterators.cs
- DSASignatureDeformatter.cs
- ConnectionInterfaceCollection.cs
- BinaryReader.cs
- SqlRetyper.cs
- Int16AnimationBase.cs
- TypeDelegator.cs
- BindingExpressionUncommonField.cs
- TextOnlyOutput.cs
- Perspective.cs
- RSACryptoServiceProvider.cs
- WebPartDisplayModeCollection.cs
- XmlAttributeProperties.cs
- InputLanguageProfileNotifySink.cs
- DateTimeOffset.cs
- CodePropertyReferenceExpression.cs
- LinqToSqlWrapper.cs
- AVElementHelper.cs
- ProfileParameter.cs
- _SecureChannel.cs
- EasingKeyFrames.cs
- DockingAttribute.cs
- BasicSecurityProfileVersion.cs
- ServiceHttpModule.cs
- CommandDevice.cs
- ApplicationSettingsBase.cs
- MemoryMappedView.cs
- WebPartDescription.cs
- ChangeNode.cs
- ContourSegment.cs
- BasicExpandProvider.cs
- SmiXetterAccessMap.cs
- DataBoundControl.cs
- ApplicationInfo.cs
- AnimatedTypeHelpers.cs
- XmlUnspecifiedAttribute.cs
- X509CertificateEndpointIdentity.cs
- ExtendedPropertyCollection.cs
- SessionEndingCancelEventArgs.cs
- XmlRawWriter.cs
- OrderedDictionary.cs
- jithelpers.cs
- FindCriteria11.cs
- RectValueSerializer.cs
- ForwardPositionQuery.cs
- FormatStringEditor.cs
- KeyboardEventArgs.cs
- ScriptManager.cs
- WebPartConnection.cs
- XmlComment.cs
- XmlSerializationWriter.cs
- EncodingNLS.cs
- DataList.cs
- TypeInfo.cs
- ResourceKey.cs
- ContextQuery.cs
- RequestStatusBarUpdateEventArgs.cs
- DisableDpiAwarenessAttribute.cs
- CallbackException.cs
- SerialPinChanges.cs
- RowTypePropertyElement.cs
- Interop.cs
- DataGridViewHitTestInfo.cs
- DataGridItemEventArgs.cs
- XmlDictionaryString.cs
- MobileControlDesigner.cs
- PasswordTextContainer.cs
- ExportOptions.cs
- webeventbuffer.cs
- BitmapEffectCollection.cs
- ItemsControl.cs
- SubpageParaClient.cs
- SevenBitStream.cs
- DbConnectionPoolIdentity.cs
- DefaultValidator.cs
- ActionFrame.cs
- GeneralTransform3DGroup.cs
- HttpModuleCollection.cs
- BamlLocalizableResourceKey.cs
- Window.cs
- LOSFormatter.cs
- DataSourceCacheDurationConverter.cs
- WindowInteractionStateTracker.cs