Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / Data / System / Data / SqlClient / SqlReferenceCollection.cs / 1 / SqlReferenceCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- using System; using System.Data; using System.Data.Common; using System.Diagnostics; using System.Data.ProviderBase; namespace System.Data.SqlClient { sealed internal class SqlReferenceCollection : DbReferenceCollection { internal const int DataReaderTag = 1; private int _dataReaderCount; internal bool MayHaveDataReader { get { return (0 != _dataReaderCount); } } override public void Add(object value, int tag) { Debug.Assert(DataReaderTag == tag, "unexpected tag?"); Debug.Assert(value is SqlDataReader, "tag doesn't match object type: SqlDataReader"); _dataReaderCount++; base.AddItem(value, tag); } internal void Deactivate() { if (MayHaveDataReader) { base.Notify(0); _dataReaderCount = 0; } Purge(); } internal SqlDataReader FindLiveReader(SqlCommand command) { // if null == command, will find first live datareader // else will find live datareader assocated with the command if (MayHaveDataReader) { foreach (SqlDataReader dataReader in Filter(DataReaderTag)) { if ((null != dataReader) && !dataReader.IsClosed && ((null == command) || (command == dataReader.Command))) { return dataReader; } } } return null; } override protected bool NotifyItem(int message, int tag, object value) { Debug.Assert(0 == message, "unexpected message?"); Debug.Assert(DataReaderTag == tag, "unexpected tag?"); SqlDataReader rdr = (SqlDataReader)value; if (!rdr.IsClosed) { rdr.CloseReaderFromConnection (); } return false; // remove it from the collection } override public void Remove(object value) { Debug.Assert(value is SqlDataReader, "SqlReferenceCollection.Remove expected a SqlDataReader"); _dataReaderCount--; base.RemoveItem(value); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- using System; using System.Data; using System.Data.Common; using System.Diagnostics; using System.Data.ProviderBase; namespace System.Data.SqlClient { sealed internal class SqlReferenceCollection : DbReferenceCollection { internal const int DataReaderTag = 1; private int _dataReaderCount; internal bool MayHaveDataReader { get { return (0 != _dataReaderCount); } } override public void Add(object value, int tag) { Debug.Assert(DataReaderTag == tag, "unexpected tag?"); Debug.Assert(value is SqlDataReader, "tag doesn't match object type: SqlDataReader"); _dataReaderCount++; base.AddItem(value, tag); } internal void Deactivate() { if (MayHaveDataReader) { base.Notify(0); _dataReaderCount = 0; } Purge(); } internal SqlDataReader FindLiveReader(SqlCommand command) { // if null == command, will find first live datareader // else will find live datareader assocated with the command if (MayHaveDataReader) { foreach (SqlDataReader dataReader in Filter(DataReaderTag)) { if ((null != dataReader) && !dataReader.IsClosed && ((null == command) || (command == dataReader.Command))) { return dataReader; } } } return null; } override protected bool NotifyItem(int message, int tag, object value) { Debug.Assert(0 == message, "unexpected message?"); Debug.Assert(DataReaderTag == tag, "unexpected tag?"); SqlDataReader rdr = (SqlDataReader)value; if (!rdr.IsClosed) { rdr.CloseReaderFromConnection (); } return false; // remove it from the collection } override public void Remove(object value) { Debug.Assert(value is SqlDataReader, "SqlReferenceCollection.Remove expected a SqlDataReader"); _dataReaderCount--; base.RemoveItem(value); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
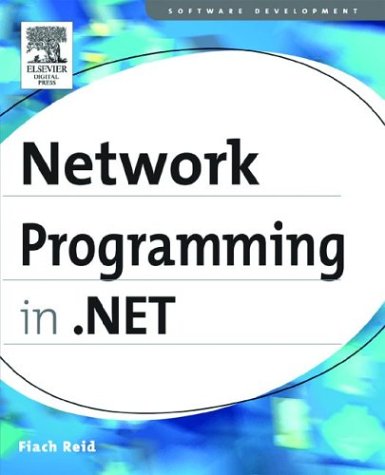
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SharedUtils.cs
- WizardPanel.cs
- GcHandle.cs
- PieceDirectory.cs
- RoleManagerSection.cs
- TableItemPattern.cs
- NullNotAllowedCollection.cs
- BulletChrome.cs
- TimeEnumHelper.cs
- StreamWriter.cs
- PathSegment.cs
- SamlAdvice.cs
- OdbcReferenceCollection.cs
- DynamicDataExtensions.cs
- MultipleViewPattern.cs
- PasswordBoxAutomationPeer.cs
- ResourceDisplayNameAttribute.cs
- TableHeaderCell.cs
- ExceptionValidationRule.cs
- HyperlinkAutomationPeer.cs
- TextFormatterHost.cs
- NeutralResourcesLanguageAttribute.cs
- OleStrCAMarshaler.cs
- HMACMD5.cs
- FloaterParagraph.cs
- COAUTHINFO.cs
- DataGridViewCellValidatingEventArgs.cs
- EventLogException.cs
- IProvider.cs
- RequestCacheEntry.cs
- Menu.cs
- HttpHandlersSection.cs
- PerformanceCounterManager.cs
- OdbcInfoMessageEvent.cs
- ViewCellRelation.cs
- SafeLibraryHandle.cs
- ThrowHelper.cs
- ConfigsHelper.cs
- ComplexObject.cs
- MobileControlsSection.cs
- TransactionScope.cs
- EnumValAlphaComparer.cs
- ContainerParagraph.cs
- ObjectDisposedException.cs
- altserialization.cs
- NaturalLanguageHyphenator.cs
- StringArrayConverter.cs
- JpegBitmapEncoder.cs
- MaskInputRejectedEventArgs.cs
- ControllableStoryboardAction.cs
- HwndStylusInputProvider.cs
- MimeMapping.cs
- DoubleLink.cs
- ZoneLinkButton.cs
- CssClassPropertyAttribute.cs
- UpdatableGenericsFeature.cs
- DataTemplateSelector.cs
- PageCatalogPart.cs
- MetadataArtifactLoaderComposite.cs
- DataGridRowDetailsEventArgs.cs
- XslTransformFileEditor.cs
- DocumentSequence.cs
- TypeHelpers.cs
- ExtendedPropertyInfo.cs
- NotifyIcon.cs
- PositiveTimeSpanValidatorAttribute.cs
- PropertyChangingEventArgs.cs
- TreeNodeMouseHoverEvent.cs
- PagesSection.cs
- Globals.cs
- DataGridViewRowStateChangedEventArgs.cs
- DocumentViewer.cs
- WebEventCodes.cs
- EventLogPropertySelector.cs
- ZipIOZip64EndOfCentralDirectoryLocatorBlock.cs
- ZipIORawDataFileBlock.cs
- Header.cs
- Buffer.cs
- CheckBoxStandardAdapter.cs
- CacheMemory.cs
- Margins.cs
- FileSystemInfo.cs
- streamingZipPartStream.cs
- PolyLineSegment.cs
- PowerModeChangedEventArgs.cs
- X509WindowsSecurityToken.cs
- X509CertificateStore.cs
- EntityCollection.cs
- WebPartCancelEventArgs.cs
- XmlLanguage.cs
- GridViewEditEventArgs.cs
- ReflectTypeDescriptionProvider.cs
- ZipIOExtraField.cs
- EDesignUtil.cs
- ExtenderControl.cs
- ChtmlMobileTextWriter.cs
- SpeechRecognitionEngine.cs
- TextTreeNode.cs
- ProfileParameter.cs
- entityreference_tresulttype.cs