Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / Data / System / Data / SQLTypes / SQLGuid.cs / 1 / SQLGuid.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //junfang //[....] //[....] //----------------------------------------------------------------------------- //************************************************************************* // @File: SqlGuid.cs // // Create by: JunFang // // Purpose: Implementation of SqlGuid which is equivalent to // data type "uniqueidentifier" in SQL Server // // Notes: // // History: // // 11/1/99 JunFang Created. // // @EndHeader@ //************************************************************************* using System; using System.Data.Common; using System.Xml; using System.Xml.Schema; using System.Xml.Serialization; namespace System.Data.SqlTypes { ////// [Serializable] [XmlSchemaProvider("GetXsdType")] public struct SqlGuid : INullable, IComparable, IXmlSerializable { private static readonly int SizeOfGuid = 16; // Comparison orders. private static readonly int[] x_rgiGuidOrder = new int[16] {10, 11, 12, 13, 14, 15, 8, 9, 6, 7, 4, 5, 0, 1, 2, 3}; private byte[] m_value; // the SqlGuid is null if m_value is null // constructor // construct a SqlGuid.Null private SqlGuid(bool fNull) { m_value = null; } ////// Represents a globally unique identifier to be stored in /// or retrieved from a database. /// ////// public SqlGuid(byte[] value) { if (value == null || value.Length != SizeOfGuid) throw new ArgumentException(SQLResource.InvalidArraySizeMessage); m_value = new byte[SizeOfGuid]; value.CopyTo(m_value, 0); } ///[To be supplied.] ////// internal SqlGuid(byte[] value, bool ignored) { if (value == null || value.Length != SizeOfGuid) throw new ArgumentException(SQLResource.InvalidArraySizeMessage); m_value = value; } ///[To be supplied.] ////// public SqlGuid(String s) { m_value = (new Guid(s)).ToByteArray(); } ///[To be supplied.] ////// public SqlGuid(Guid g) { m_value = g.ToByteArray(); } public SqlGuid(int a, short b, short c, byte d, byte e, byte f, byte g, byte h, byte i, byte j, byte k) : this(new Guid(a, b, c, d, e, f, g, h, i, j, k)) { } // INullable ///[To be supplied.] ////// public bool IsNull { get { return(m_value == null);} } // property: Value ///[To be supplied.] ////// public Guid Value { get { if (IsNull) throw new SqlNullValueException(); else return new Guid(m_value); } } // Implicit conversion from Guid to SqlGuid ///[To be supplied.] ////// public static implicit operator SqlGuid(Guid x) { return new SqlGuid(x); } // Explicit conversion from SqlGuid to Guid. Throw exception if x is Null. ///[To be supplied.] ////// public static explicit operator Guid(SqlGuid x) { return x.Value; } ///[To be supplied.] ////// public byte[] ToByteArray() { byte[] ret = new byte[SizeOfGuid]; m_value.CopyTo(ret, 0); return ret; } ///[To be supplied.] ////// public override String ToString() { if (IsNull) return SQLResource.NullString; Guid g = new Guid(m_value); return g.ToString(); } ///[To be supplied.] ////// public static SqlGuid Parse(String s) { if (s == SQLResource.NullString) return SqlGuid.Null; else return new SqlGuid(s); } // Comparison operators private static EComparison Compare(SqlGuid x, SqlGuid y) { //Swap to the correct order to be compared for (int i = 0; i < SizeOfGuid; i++) { byte b1, b2; b1 = x.m_value [x_rgiGuidOrder[i]]; b2 = y.m_value [x_rgiGuidOrder[i]]; if (b1 != b2) return(b1 < b2) ? EComparison.LT : EComparison.GT; } return EComparison.EQ; } // Implicit conversions // Explicit conversions // Explicit conversion from SqlString to SqlGuid ///[To be supplied.] ////// public static explicit operator SqlGuid(SqlString x) { return x.IsNull ? Null : new SqlGuid(x.Value); } // Explicit conversion from SqlBinary to SqlGuid ///[To be supplied.] ////// public static explicit operator SqlGuid(SqlBinary x) { return x.IsNull ? Null : new SqlGuid(x.Value); } // Overloading comparison operators ///[To be supplied.] ////// public static SqlBoolean operator==(SqlGuid x, SqlGuid y) { return(x.IsNull || y.IsNull) ? SqlBoolean.Null : new SqlBoolean(Compare(x, y) == EComparison.EQ); } ///[To be supplied.] ////// public static SqlBoolean operator!=(SqlGuid x, SqlGuid y) { return ! (x == y); } ///[To be supplied.] ////// public static SqlBoolean operator<(SqlGuid x, SqlGuid y) { return(x.IsNull || y.IsNull) ? SqlBoolean.Null : new SqlBoolean(Compare(x, y) == EComparison.LT); } ///[To be supplied.] ////// public static SqlBoolean operator>(SqlGuid x, SqlGuid y) { return(x.IsNull || y.IsNull) ? SqlBoolean.Null : new SqlBoolean(Compare(x, y) == EComparison.GT); } ///[To be supplied.] ////// public static SqlBoolean operator<=(SqlGuid x, SqlGuid y) { if (x.IsNull || y.IsNull) return SqlBoolean.Null; EComparison cmp = Compare(x, y); return new SqlBoolean(cmp == EComparison.LT || cmp == EComparison.EQ); } ///[To be supplied.] ////// public static SqlBoolean operator>=(SqlGuid x, SqlGuid y) { if (x.IsNull || y.IsNull) return SqlBoolean.Null; EComparison cmp = Compare(x, y); return new SqlBoolean(cmp == EComparison.GT || cmp == EComparison.EQ); } //-------------------------------------------------- // Alternative methods for overloaded operators //-------------------------------------------------- // Alternative method for operator == public static SqlBoolean Equals(SqlGuid x, SqlGuid y) { return (x == y); } // Alternative method for operator != public static SqlBoolean NotEquals(SqlGuid x, SqlGuid y) { return (x != y); } // Alternative method for operator < public static SqlBoolean LessThan(SqlGuid x, SqlGuid y) { return (x < y); } // Alternative method for operator > public static SqlBoolean GreaterThan(SqlGuid x, SqlGuid y) { return (x > y); } // Alternative method for operator <= public static SqlBoolean LessThanOrEqual(SqlGuid x, SqlGuid y) { return (x <= y); } // Alternative method for operator >= public static SqlBoolean GreaterThanOrEqual(SqlGuid x, SqlGuid y) { return (x >= y); } // Alternative method for conversions. public SqlString ToSqlString() { return (SqlString)this; } public SqlBinary ToSqlBinary() { return (SqlBinary)this; } // IComparable // Compares this object to another object, returning an integer that // indicates the relationship. // Returns a value less than zero if this < object, zero if this = object, // or a value greater than zero if this > object. // null is considered to be less than any instance. // If object is not of same type, this method throws an ArgumentException. ///[To be supplied.] ////// public int CompareTo(Object value) { if (value is SqlGuid) { SqlGuid i = (SqlGuid)value; return CompareTo(i); } throw ADP.WrongType(value.GetType(), typeof(SqlGuid)); } public int CompareTo(SqlGuid value) { // If both Null, consider them equal. // Otherwise, Null is less than anything. if (IsNull) return value.IsNull ? 0 : -1; else if (value.IsNull) return 1; if (this < value) return -1; if (this > value) return 1; return 0; } // Compares this instance with a specified object ///[To be supplied.] ////// public override bool Equals(Object value) { if (!(value is SqlGuid)) { return false; } SqlGuid i = (SqlGuid)value; if (i.IsNull || IsNull) return (i.IsNull && IsNull); else return (this == i).Value; } // For hashing purpose ///[To be supplied.] ////// public override int GetHashCode() { return IsNull ? 0 : Value.GetHashCode(); } ///[To be supplied.] ////// XmlSchema IXmlSerializable.GetSchema() { return null; } ///[To be supplied.] ////// void IXmlSerializable.ReadXml(XmlReader reader) { string isNull = reader.GetAttribute("nil", XmlSchema.InstanceNamespace); if (isNull != null && XmlConvert.ToBoolean(isNull)) { m_value = null; } else { m_value = new Guid(reader.ReadElementString()).ToByteArray(); } } ///[To be supplied.] ////// void IXmlSerializable.WriteXml(XmlWriter writer) { if (IsNull) { writer.WriteAttributeString("xsi", "nil", XmlSchema.InstanceNamespace, "true"); } else { writer.WriteString(XmlConvert.ToString(new Guid(m_value))); } } ///[To be supplied.] ////// public static XmlQualifiedName GetXsdType(XmlSchemaSet schemaSet) { return new XmlQualifiedName("string", XmlSchema.Namespace); } ///[To be supplied.] ////// public static readonly SqlGuid Null = new SqlGuid(true); } // SqlGuid } // namespace System.Data.SqlTypes // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //junfang //[....] //[....] //----------------------------------------------------------------------------- //************************************************************************* // @File: SqlGuid.cs // // Create by: JunFang // // Purpose: Implementation of SqlGuid which is equivalent to // data type "uniqueidentifier" in SQL Server // // Notes: // // History: // // 11/1/99 JunFang Created. // // @EndHeader@ //************************************************************************* using System; using System.Data.Common; using System.Xml; using System.Xml.Schema; using System.Xml.Serialization; namespace System.Data.SqlTypes { ////// [Serializable] [XmlSchemaProvider("GetXsdType")] public struct SqlGuid : INullable, IComparable, IXmlSerializable { private static readonly int SizeOfGuid = 16; // Comparison orders. private static readonly int[] x_rgiGuidOrder = new int[16] {10, 11, 12, 13, 14, 15, 8, 9, 6, 7, 4, 5, 0, 1, 2, 3}; private byte[] m_value; // the SqlGuid is null if m_value is null // constructor // construct a SqlGuid.Null private SqlGuid(bool fNull) { m_value = null; } ////// Represents a globally unique identifier to be stored in /// or retrieved from a database. /// ////// public SqlGuid(byte[] value) { if (value == null || value.Length != SizeOfGuid) throw new ArgumentException(SQLResource.InvalidArraySizeMessage); m_value = new byte[SizeOfGuid]; value.CopyTo(m_value, 0); } ///[To be supplied.] ////// internal SqlGuid(byte[] value, bool ignored) { if (value == null || value.Length != SizeOfGuid) throw new ArgumentException(SQLResource.InvalidArraySizeMessage); m_value = value; } ///[To be supplied.] ////// public SqlGuid(String s) { m_value = (new Guid(s)).ToByteArray(); } ///[To be supplied.] ////// public SqlGuid(Guid g) { m_value = g.ToByteArray(); } public SqlGuid(int a, short b, short c, byte d, byte e, byte f, byte g, byte h, byte i, byte j, byte k) : this(new Guid(a, b, c, d, e, f, g, h, i, j, k)) { } // INullable ///[To be supplied.] ////// public bool IsNull { get { return(m_value == null);} } // property: Value ///[To be supplied.] ////// public Guid Value { get { if (IsNull) throw new SqlNullValueException(); else return new Guid(m_value); } } // Implicit conversion from Guid to SqlGuid ///[To be supplied.] ////// public static implicit operator SqlGuid(Guid x) { return new SqlGuid(x); } // Explicit conversion from SqlGuid to Guid. Throw exception if x is Null. ///[To be supplied.] ////// public static explicit operator Guid(SqlGuid x) { return x.Value; } ///[To be supplied.] ////// public byte[] ToByteArray() { byte[] ret = new byte[SizeOfGuid]; m_value.CopyTo(ret, 0); return ret; } ///[To be supplied.] ////// public override String ToString() { if (IsNull) return SQLResource.NullString; Guid g = new Guid(m_value); return g.ToString(); } ///[To be supplied.] ////// public static SqlGuid Parse(String s) { if (s == SQLResource.NullString) return SqlGuid.Null; else return new SqlGuid(s); } // Comparison operators private static EComparison Compare(SqlGuid x, SqlGuid y) { //Swap to the correct order to be compared for (int i = 0; i < SizeOfGuid; i++) { byte b1, b2; b1 = x.m_value [x_rgiGuidOrder[i]]; b2 = y.m_value [x_rgiGuidOrder[i]]; if (b1 != b2) return(b1 < b2) ? EComparison.LT : EComparison.GT; } return EComparison.EQ; } // Implicit conversions // Explicit conversions // Explicit conversion from SqlString to SqlGuid ///[To be supplied.] ////// public static explicit operator SqlGuid(SqlString x) { return x.IsNull ? Null : new SqlGuid(x.Value); } // Explicit conversion from SqlBinary to SqlGuid ///[To be supplied.] ////// public static explicit operator SqlGuid(SqlBinary x) { return x.IsNull ? Null : new SqlGuid(x.Value); } // Overloading comparison operators ///[To be supplied.] ////// public static SqlBoolean operator==(SqlGuid x, SqlGuid y) { return(x.IsNull || y.IsNull) ? SqlBoolean.Null : new SqlBoolean(Compare(x, y) == EComparison.EQ); } ///[To be supplied.] ////// public static SqlBoolean operator!=(SqlGuid x, SqlGuid y) { return ! (x == y); } ///[To be supplied.] ////// public static SqlBoolean operator<(SqlGuid x, SqlGuid y) { return(x.IsNull || y.IsNull) ? SqlBoolean.Null : new SqlBoolean(Compare(x, y) == EComparison.LT); } ///[To be supplied.] ////// public static SqlBoolean operator>(SqlGuid x, SqlGuid y) { return(x.IsNull || y.IsNull) ? SqlBoolean.Null : new SqlBoolean(Compare(x, y) == EComparison.GT); } ///[To be supplied.] ////// public static SqlBoolean operator<=(SqlGuid x, SqlGuid y) { if (x.IsNull || y.IsNull) return SqlBoolean.Null; EComparison cmp = Compare(x, y); return new SqlBoolean(cmp == EComparison.LT || cmp == EComparison.EQ); } ///[To be supplied.] ////// public static SqlBoolean operator>=(SqlGuid x, SqlGuid y) { if (x.IsNull || y.IsNull) return SqlBoolean.Null; EComparison cmp = Compare(x, y); return new SqlBoolean(cmp == EComparison.GT || cmp == EComparison.EQ); } //-------------------------------------------------- // Alternative methods for overloaded operators //-------------------------------------------------- // Alternative method for operator == public static SqlBoolean Equals(SqlGuid x, SqlGuid y) { return (x == y); } // Alternative method for operator != public static SqlBoolean NotEquals(SqlGuid x, SqlGuid y) { return (x != y); } // Alternative method for operator < public static SqlBoolean LessThan(SqlGuid x, SqlGuid y) { return (x < y); } // Alternative method for operator > public static SqlBoolean GreaterThan(SqlGuid x, SqlGuid y) { return (x > y); } // Alternative method for operator <= public static SqlBoolean LessThanOrEqual(SqlGuid x, SqlGuid y) { return (x <= y); } // Alternative method for operator >= public static SqlBoolean GreaterThanOrEqual(SqlGuid x, SqlGuid y) { return (x >= y); } // Alternative method for conversions. public SqlString ToSqlString() { return (SqlString)this; } public SqlBinary ToSqlBinary() { return (SqlBinary)this; } // IComparable // Compares this object to another object, returning an integer that // indicates the relationship. // Returns a value less than zero if this < object, zero if this = object, // or a value greater than zero if this > object. // null is considered to be less than any instance. // If object is not of same type, this method throws an ArgumentException. ///[To be supplied.] ////// public int CompareTo(Object value) { if (value is SqlGuid) { SqlGuid i = (SqlGuid)value; return CompareTo(i); } throw ADP.WrongType(value.GetType(), typeof(SqlGuid)); } public int CompareTo(SqlGuid value) { // If both Null, consider them equal. // Otherwise, Null is less than anything. if (IsNull) return value.IsNull ? 0 : -1; else if (value.IsNull) return 1; if (this < value) return -1; if (this > value) return 1; return 0; } // Compares this instance with a specified object ///[To be supplied.] ////// public override bool Equals(Object value) { if (!(value is SqlGuid)) { return false; } SqlGuid i = (SqlGuid)value; if (i.IsNull || IsNull) return (i.IsNull && IsNull); else return (this == i).Value; } // For hashing purpose ///[To be supplied.] ////// public override int GetHashCode() { return IsNull ? 0 : Value.GetHashCode(); } ///[To be supplied.] ////// XmlSchema IXmlSerializable.GetSchema() { return null; } ///[To be supplied.] ////// void IXmlSerializable.ReadXml(XmlReader reader) { string isNull = reader.GetAttribute("nil", XmlSchema.InstanceNamespace); if (isNull != null && XmlConvert.ToBoolean(isNull)) { m_value = null; } else { m_value = new Guid(reader.ReadElementString()).ToByteArray(); } } ///[To be supplied.] ////// void IXmlSerializable.WriteXml(XmlWriter writer) { if (IsNull) { writer.WriteAttributeString("xsi", "nil", XmlSchema.InstanceNamespace, "true"); } else { writer.WriteString(XmlConvert.ToString(new Guid(m_value))); } } ///[To be supplied.] ////// public static XmlQualifiedName GetXsdType(XmlSchemaSet schemaSet) { return new XmlQualifiedName("string", XmlSchema.Namespace); } ///[To be supplied.] ////// public static readonly SqlGuid Null = new SqlGuid(true); } // SqlGuid } // namespace System.Data.SqlTypes // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
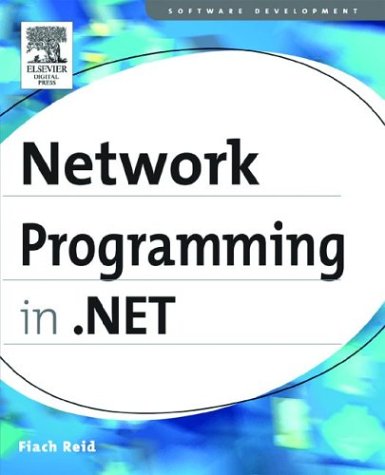
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ADMembershipProvider.cs
- LinkLabelLinkClickedEvent.cs
- BamlBinaryReader.cs
- TraceContext.cs
- PropertyChange.cs
- WriteFileContext.cs
- WindowsHyperlink.cs
- FloaterParaClient.cs
- XPathSelectionIterator.cs
- StringOutput.cs
- UIElement.cs
- IResourceProvider.cs
- DataSourceListEditor.cs
- BitStack.cs
- HttpRequestWrapper.cs
- Page.cs
- LoginCancelEventArgs.cs
- CheckBoxDesigner.cs
- SystemIPGlobalStatistics.cs
- DataGridViewRowHeaderCell.cs
- Filter.cs
- MarkupCompiler.cs
- ComPlusDiagnosticTraceSchemas.cs
- XmlObjectSerializer.cs
- Cursors.cs
- TextBox.cs
- SmiContext.cs
- NetMsmqSecurity.cs
- GuidTagList.cs
- ReliabilityContractAttribute.cs
- control.ime.cs
- DetailsViewPageEventArgs.cs
- Int32RectValueSerializer.cs
- XmlBinaryWriter.cs
- DataViewListener.cs
- IRCollection.cs
- ApplicationManager.cs
- FileDialogCustomPlace.cs
- XmlWrappingWriter.cs
- NoneExcludedImageIndexConverter.cs
- BooleanAnimationBase.cs
- Globals.cs
- Main.cs
- CellNormalizer.cs
- ParameterModifier.cs
- StatusCommandUI.cs
- SerializerWriterEventHandlers.cs
- ProvidersHelper.cs
- EmptyEnumerator.cs
- TreeNodeCollection.cs
- WebPartConnectionsCancelVerb.cs
- DataListItem.cs
- Symbol.cs
- XmlSchemaAppInfo.cs
- GcSettings.cs
- DocumentViewerAutomationPeer.cs
- ObjectDataSourceChooseTypePanel.cs
- HtmlTableCell.cs
- ParameterSubsegment.cs
- TagNameToTypeMapper.cs
- ManualResetEvent.cs
- PixelShader.cs
- NetMsmqSecurityElement.cs
- HttpEncoderUtility.cs
- RestHandler.cs
- FixedDocumentPaginator.cs
- PropertyItem.cs
- TextServicesHost.cs
- ServicePrincipalNameElement.cs
- Enumerable.cs
- RawMouseInputReport.cs
- Crc32Helper.cs
- XmlSchema.cs
- GridViewCancelEditEventArgs.cs
- XmlMemberMapping.cs
- PathGradientBrush.cs
- PropVariant.cs
- ClientUrlResolverWrapper.cs
- WmlTextBoxAdapter.cs
- LineInfo.cs
- FlowDocumentPageViewerAutomationPeer.cs
- AsynchronousChannelMergeEnumerator.cs
- SmtpException.cs
- MeshGeometry3D.cs
- SignatureDescription.cs
- ToolStripSettings.cs
- RootBrowserWindowProxy.cs
- ObjectListItemCollection.cs
- DrawingState.cs
- FileRecordSequence.cs
- WindowsRebar.cs
- ImageAnimator.cs
- ReadingWritingEntityEventArgs.cs
- SerializationEventsCache.cs
- RelationshipSet.cs
- FieldNameLookup.cs
- StreamFormatter.cs
- ZipIOExtraFieldZip64Element.cs
- FixedSOMLineCollection.cs
- keycontainerpermission.cs