Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / CommonUI / System / Drawing / Advanced / PointF.cs / 1 / PointF.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing { using System.Diagnostics; using System.Drawing; using System.ComponentModel; using System; using System.Diagnostics.CodeAnalysis; using System.Globalization; /** * Represents a point in 2D coordinate space * (float precision floating-point coordinates) */ ////// /// Represents an ordered pair of x and y coordinates that /// define a point in a two-dimensional plane. /// [Serializable] [System.Runtime.InteropServices.ComVisible(true)] public struct PointF { ////// /// public static readonly PointF Empty = new PointF(); private float x; private float y; /** * Create a new Point object at the given location */ ////// Creates a new instance of the ///class /// with member data left uninitialized. /// /// /// public PointF(float x, float y) { this.x = x; this.y = y; } ////// Initializes a new instance of the ///class /// with the specified coordinates. /// /// /// [Browsable(false)] public bool IsEmpty { get { return x == 0f && y == 0f; } } ////// Gets a value indicating whether this ///is empty. /// /// /// public float X { get { return x; } set { x = value; } } ////// Gets the x-coordinate of this ///. /// /// /// public float Y { get { return y; } set { y = value; } } ////// Gets the y-coordinate of this ///. /// /// /// public static PointF operator +(PointF pt, Size sz) { return Add(pt, sz); } ////// Translates a ///by a given . /// /// /// public static PointF operator -(PointF pt, Size sz) { return Subtract(pt, sz); } ////// Translates a ///by the negative of a given . /// /// public static PointF operator +(PointF pt, SizeF sz) { return Add(pt, sz); } ////// Translates a ///by a given . /// /// public static PointF operator -(PointF pt, SizeF sz) { return Subtract(pt, sz); } ////// Translates a ///by the negative of a given . /// /// /// public static bool operator ==(PointF left, PointF right) { return left.X == right.X && left.Y == right.Y; } ////// Compares two ///objects. The result specifies /// whether the values of the and properties of the two /// objects are equal. /// /// /// public static bool operator !=(PointF left, PointF right) { return !(left == right); } ////// Compares two ///objects. The result specifies whether the values /// of the or properties of the two /// /// objects are unequal. /// /// public static PointF Add(PointF pt, Size sz) { return new PointF(pt.X + sz.Width, pt.Y + sz.Height); } ////// Translates a ///by a given . /// /// public static PointF Subtract(PointF pt, Size sz) { return new PointF(pt.X - sz.Width, pt.Y - sz.Height); } ////// Translates a ///by the negative of a given . /// /// public static PointF Add(PointF pt, SizeF sz){ return new PointF(pt.X + sz.Width, pt.Y + sz.Height); } ////// Translates a ///by a given . /// /// public static PointF Subtract(PointF pt, SizeF sz){ return new PointF(pt.X - sz.Width, pt.Y - sz.Height); } ////// Translates a ///by the negative of a given . /// /// /// public override bool Equals(object obj) { if (!(obj is PointF)) return false; PointF comp = (PointF)obj; return comp.X == this.X && comp.Y == this.Y && comp.GetType().Equals(this.GetType()); } ///[To be supplied.] ////// /// public override int GetHashCode() { return base.GetHashCode(); } ///[To be supplied.] ///public override string ToString() { return string.Format(CultureInfo.CurrentCulture, "{{X={0}, Y={1}}}", x, y); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing { using System.Diagnostics; using System.Drawing; using System.ComponentModel; using System; using System.Diagnostics.CodeAnalysis; using System.Globalization; /** * Represents a point in 2D coordinate space * (float precision floating-point coordinates) */ ////// /// Represents an ordered pair of x and y coordinates that /// define a point in a two-dimensional plane. /// [Serializable] [System.Runtime.InteropServices.ComVisible(true)] public struct PointF { ////// /// public static readonly PointF Empty = new PointF(); private float x; private float y; /** * Create a new Point object at the given location */ ////// Creates a new instance of the ///class /// with member data left uninitialized. /// /// /// public PointF(float x, float y) { this.x = x; this.y = y; } ////// Initializes a new instance of the ///class /// with the specified coordinates. /// /// /// [Browsable(false)] public bool IsEmpty { get { return x == 0f && y == 0f; } } ////// Gets a value indicating whether this ///is empty. /// /// /// public float X { get { return x; } set { x = value; } } ////// Gets the x-coordinate of this ///. /// /// /// public float Y { get { return y; } set { y = value; } } ////// Gets the y-coordinate of this ///. /// /// /// public static PointF operator +(PointF pt, Size sz) { return Add(pt, sz); } ////// Translates a ///by a given . /// /// /// public static PointF operator -(PointF pt, Size sz) { return Subtract(pt, sz); } ////// Translates a ///by the negative of a given . /// /// public static PointF operator +(PointF pt, SizeF sz) { return Add(pt, sz); } ////// Translates a ///by a given . /// /// public static PointF operator -(PointF pt, SizeF sz) { return Subtract(pt, sz); } ////// Translates a ///by the negative of a given . /// /// /// public static bool operator ==(PointF left, PointF right) { return left.X == right.X && left.Y == right.Y; } ////// Compares two ///objects. The result specifies /// whether the values of the and properties of the two /// objects are equal. /// /// /// public static bool operator !=(PointF left, PointF right) { return !(left == right); } ////// Compares two ///objects. The result specifies whether the values /// of the or properties of the two /// /// objects are unequal. /// /// public static PointF Add(PointF pt, Size sz) { return new PointF(pt.X + sz.Width, pt.Y + sz.Height); } ////// Translates a ///by a given . /// /// public static PointF Subtract(PointF pt, Size sz) { return new PointF(pt.X - sz.Width, pt.Y - sz.Height); } ////// Translates a ///by the negative of a given . /// /// public static PointF Add(PointF pt, SizeF sz){ return new PointF(pt.X + sz.Width, pt.Y + sz.Height); } ////// Translates a ///by a given . /// /// public static PointF Subtract(PointF pt, SizeF sz){ return new PointF(pt.X - sz.Width, pt.Y - sz.Height); } ////// Translates a ///by the negative of a given . /// /// /// public override bool Equals(object obj) { if (!(obj is PointF)) return false; PointF comp = (PointF)obj; return comp.X == this.X && comp.Y == this.Y && comp.GetType().Equals(this.GetType()); } ///[To be supplied.] ////// /// public override int GetHashCode() { return base.GetHashCode(); } ///[To be supplied.] ///public override string ToString() { return string.Format(CultureInfo.CurrentCulture, "{{X={0}, Y={1}}}", x, y); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
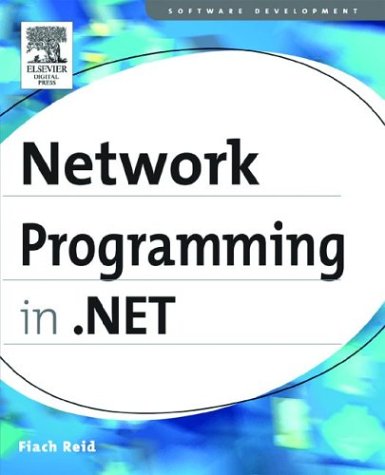
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FieldNameLookup.cs
- MarkupCompilePass1.cs
- GenericsInstances.cs
- SafeNativeMethodsMilCoreApi.cs
- wgx_exports.cs
- CodeRemoveEventStatement.cs
- FormatterServicesNoSerializableCheck.cs
- AmbiguousMatchException.cs
- AppDomainGrammarProxy.cs
- ControlCachePolicy.cs
- ActiveDocumentEvent.cs
- DataGridLinkButton.cs
- BamlTreeNode.cs
- HMACSHA384.cs
- BitmapEffectState.cs
- FrameSecurityDescriptor.cs
- ServiceModelInstallComponent.cs
- ProjectionCamera.cs
- DeferredElementTreeState.cs
- CheckBoxField.cs
- WmiEventSink.cs
- ConfigurationSectionCollection.cs
- RowToFieldTransformer.cs
- MessageSecurityTokenVersion.cs
- PopupEventArgs.cs
- EntityTransaction.cs
- DbSetClause.cs
- AuthorizationContext.cs
- DocumentGridContextMenu.cs
- OleServicesContext.cs
- BuildManagerHost.cs
- DefaultTextStoreTextComposition.cs
- XmlRawWriterWrapper.cs
- EncryptedPackage.cs
- ListViewItemMouseHoverEvent.cs
- DocumentReference.cs
- DesignerActionKeyboardBehavior.cs
- CTreeGenerator.cs
- HTMLTagNameToTypeMapper.cs
- RegexWriter.cs
- CodeTryCatchFinallyStatement.cs
- CharConverter.cs
- InputMethodStateTypeInfo.cs
- TabRenderer.cs
- TypeListConverter.cs
- TraceContext.cs
- DoubleLinkList.cs
- MsmqTransportSecurity.cs
- AllMembershipCondition.cs
- PerformanceCounterCategory.cs
- IfJoinedCondition.cs
- Page.cs
- ManualResetEvent.cs
- DataGridViewTextBoxCell.cs
- LinearGradientBrush.cs
- ToolStripPanel.cs
- LineUtil.cs
- XmlArrayAttribute.cs
- MetadataProperty.cs
- HostProtectionException.cs
- BuildProvidersCompiler.cs
- BinaryVersion.cs
- RegisterInfo.cs
- FullTrustAssemblyCollection.cs
- ScriptingSectionGroup.cs
- ListItemCollection.cs
- PrimitiveXmlSerializers.cs
- DbDataAdapter.cs
- MorphHelpers.cs
- ProviderUtil.cs
- XmlWellformedWriter.cs
- GenericAuthenticationEventArgs.cs
- PopupRoot.cs
- DeclarativeConditionsCollection.cs
- GlyphingCache.cs
- StyleHelper.cs
- PersistNameAttribute.cs
- QueryOpcode.cs
- StandardToolWindows.cs
- RegisteredArrayDeclaration.cs
- OneOfTypeConst.cs
- XmlName.cs
- ServiceProviders.cs
- CryptoApi.cs
- RemotingServices.cs
- StateItem.cs
- DbMetaDataFactory.cs
- RequestTimeoutManager.cs
- _AutoWebProxyScriptHelper.cs
- DataGridViewCheckBoxCell.cs
- NumericUpDown.cs
- EntityDataSourceSelectingEventArgs.cs
- AddInAttribute.cs
- MimeAnyImporter.cs
- XPathNode.cs
- IsolatedStorageException.cs
- FlowNode.cs
- UpdatePanelTrigger.cs
- UInt32Storage.cs
- AnimationException.cs