Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / UIAutomation / UIAutomationClient / System / Windows / Automation / ValuePattern.cs / 1 / ValuePattern.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Client-side wrapper for Value Pattern // // History: // 06/23/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Windows.Automation.Provider; using MS.Internal.Automation; namespace System.Windows.Automation { ////// Represents UI elements that are expressing a value /// #if (INTERNAL_COMPILE) internal class ValuePattern: BasePattern #else public class ValuePattern: BasePattern #endif { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors // internal so that RangeValue can derive from this internal ValuePattern(AutomationElement el, SafePatternHandle hPattern, bool cached) : base(el, hPattern) { _hPattern = hPattern; _cached = cached; } #endregion Constructors //------------------------------------------------------ // // Public Constants / Readonly Fields // //----------------------------------------------------- #region Public Constants and Readonly Fields ///Value pattern public static readonly AutomationPattern Pattern = ValuePatternIdentifiers.Pattern; ///Property ID: Value - Value of a value control, as a human-readable string public static readonly AutomationProperty ValueProperty = ValuePatternIdentifiers.ValueProperty; ///Property ID: IsReadOnly - Indicates that the value can only be read, not modified. public static readonly AutomationProperty IsReadOnlyProperty = ValuePatternIdentifiers.IsReadOnlyProperty; #endregion Public Constants and Readonly Fields //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public Methods ////// Request to set the value that this UI element is representing /// /// Value to set the UI to, the provider is responsible for converting from a string into the appropriate data type /// ////// This API does not work inside the secure execution environment. /// public void SetValue( string value ) { Misc.ValidateArgumentNonNull(value, "value"); // Test the Enabled state prior to the more general Read-Only state. object enabled = _el.GetCurrentPropertyValue(AutomationElementIdentifiers.IsEnabledProperty); if (enabled is bool && !(bool)enabled) { throw new ElementNotEnabledException(); } // Test the Read-Only state after the more specific Enabled state. object readOnly = _el.GetCurrentPropertyValue(IsReadOnlyProperty); if (readOnly is bool && (bool)readOnly) { throw new InvalidOperationException(SR.Get(SRID.ValueReadonly)); } UiaCoreApi.ValuePattern_SetValue(_hPattern, value); } #endregion Public Methods //----------------------------------------------------- // // Public Properties // //------------------------------------------------------ #region Public Properties ////// /// This member allows access to previously requested /// cached properties for this element. The returned object /// has accessors for each property defined for this pattern. /// ////// Cached property values must have been previously requested /// using a CacheRequest. If you try to access a cached /// property that was not previously requested, an InvalidOperation /// Exception will be thrown. /// /// To get the value of a property at the current point in time, /// access the property via the Current accessor instead of /// Cached. /// public ValuePatternInformation Cached { get { Misc.ValidateCached(_cached); return new ValuePatternInformation(_el, true); } } ////// This member allows access to current property values /// for this element. The returned object has accessors for /// each property defined for this pattern. /// ////// This pattern must be from an AutomationElement with a /// Full reference in order to get current values. If the /// AutomationElement was obtained using AutomationElementMode.None, /// then it contains only cached data, and attempting to get /// the current value of any property will throw an InvalidOperationException. /// /// To get the cached value of a property that was previously /// specified using a CacheRequest, access the property via the /// Cached accessor instead of Current. /// public ValuePatternInformation Current { get { Misc.ValidateCurrent(_hPattern); return new ValuePatternInformation(_el, false); } } #endregion Public Properties //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods static internal object Wrap(AutomationElement el, SafePatternHandle hPattern, bool cached) { return new ValuePattern(el, hPattern, cached); } #endregion Internal Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private SafePatternHandle _hPattern; private bool _cached; #endregion Private Fields //----------------------------------------------------- // // Nested Classes // //------------------------------------------------------ #region Nested Classes ////// This class provides access to either Cached or Current /// properties on a pattern via the pattern's .Cached or /// .Current accessors. /// public struct ValuePatternInformation { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- #region Constructors internal ValuePatternInformation(AutomationElement el, bool useCache) { _el = el; _useCache = useCache; } #endregion Constructors //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ///Value of a value control, as a a string. /// ////// This API does not work inside the secure execution environment. /// public string Value { get { // object temp = _el.GetPatternPropertyValue(ValueProperty, _useCache); return temp.ToString(); } } ////// Indicates that the value can only be read, not modified. ///returns True if the control is read-only /// ////// This API does not work inside the secure execution environment. /// public bool IsReadOnly { get { return (bool)_el.GetPatternPropertyValue(IsReadOnlyProperty, _useCache); } } #endregion Public Properties //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private AutomationElement _el; // AutomationElement that contains the cache or live reference private bool _useCache; // true to use cache, false to use live reference to get current values #endregion Private Fields } #endregion Nested Classes } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // ///// // Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Client-side wrapper for Value Pattern // // History: // 06/23/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Windows.Automation.Provider; using MS.Internal.Automation; namespace System.Windows.Automation { ////// Represents UI elements that are expressing a value /// #if (INTERNAL_COMPILE) internal class ValuePattern: BasePattern #else public class ValuePattern: BasePattern #endif { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors // internal so that RangeValue can derive from this internal ValuePattern(AutomationElement el, SafePatternHandle hPattern, bool cached) : base(el, hPattern) { _hPattern = hPattern; _cached = cached; } #endregion Constructors //------------------------------------------------------ // // Public Constants / Readonly Fields // //----------------------------------------------------- #region Public Constants and Readonly Fields ///Value pattern public static readonly AutomationPattern Pattern = ValuePatternIdentifiers.Pattern; ///Property ID: Value - Value of a value control, as a human-readable string public static readonly AutomationProperty ValueProperty = ValuePatternIdentifiers.ValueProperty; ///Property ID: IsReadOnly - Indicates that the value can only be read, not modified. public static readonly AutomationProperty IsReadOnlyProperty = ValuePatternIdentifiers.IsReadOnlyProperty; #endregion Public Constants and Readonly Fields //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public Methods ////// Request to set the value that this UI element is representing /// /// Value to set the UI to, the provider is responsible for converting from a string into the appropriate data type /// ////// This API does not work inside the secure execution environment. /// public void SetValue( string value ) { Misc.ValidateArgumentNonNull(value, "value"); // Test the Enabled state prior to the more general Read-Only state. object enabled = _el.GetCurrentPropertyValue(AutomationElementIdentifiers.IsEnabledProperty); if (enabled is bool && !(bool)enabled) { throw new ElementNotEnabledException(); } // Test the Read-Only state after the more specific Enabled state. object readOnly = _el.GetCurrentPropertyValue(IsReadOnlyProperty); if (readOnly is bool && (bool)readOnly) { throw new InvalidOperationException(SR.Get(SRID.ValueReadonly)); } UiaCoreApi.ValuePattern_SetValue(_hPattern, value); } #endregion Public Methods //----------------------------------------------------- // // Public Properties // //------------------------------------------------------ #region Public Properties ////// /// This member allows access to previously requested /// cached properties for this element. The returned object /// has accessors for each property defined for this pattern. /// ////// Cached property values must have been previously requested /// using a CacheRequest. If you try to access a cached /// property that was not previously requested, an InvalidOperation /// Exception will be thrown. /// /// To get the value of a property at the current point in time, /// access the property via the Current accessor instead of /// Cached. /// public ValuePatternInformation Cached { get { Misc.ValidateCached(_cached); return new ValuePatternInformation(_el, true); } } ////// This member allows access to current property values /// for this element. The returned object has accessors for /// each property defined for this pattern. /// ////// This pattern must be from an AutomationElement with a /// Full reference in order to get current values. If the /// AutomationElement was obtained using AutomationElementMode.None, /// then it contains only cached data, and attempting to get /// the current value of any property will throw an InvalidOperationException. /// /// To get the cached value of a property that was previously /// specified using a CacheRequest, access the property via the /// Cached accessor instead of Current. /// public ValuePatternInformation Current { get { Misc.ValidateCurrent(_hPattern); return new ValuePatternInformation(_el, false); } } #endregion Public Properties //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods static internal object Wrap(AutomationElement el, SafePatternHandle hPattern, bool cached) { return new ValuePattern(el, hPattern, cached); } #endregion Internal Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private SafePatternHandle _hPattern; private bool _cached; #endregion Private Fields //----------------------------------------------------- // // Nested Classes // //------------------------------------------------------ #region Nested Classes ////// This class provides access to either Cached or Current /// properties on a pattern via the pattern's .Cached or /// .Current accessors. /// public struct ValuePatternInformation { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- #region Constructors internal ValuePatternInformation(AutomationElement el, bool useCache) { _el = el; _useCache = useCache; } #endregion Constructors //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ///Value of a value control, as a a string. /// ////// This API does not work inside the secure execution environment. /// public string Value { get { // object temp = _el.GetPatternPropertyValue(ValueProperty, _useCache); return temp.ToString(); } } ////// Indicates that the value can only be read, not modified. ///returns True if the control is read-only /// ////// This API does not work inside the secure execution environment. /// public bool IsReadOnly { get { return (bool)_el.GetPatternPropertyValue(IsReadOnlyProperty, _useCache); } } #endregion Public Properties //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private AutomationElement _el; // AutomationElement that contains the cache or live reference private bool _useCache; // true to use cache, false to use live reference to get current values #endregion Private Fields } #endregion Nested Classes } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.///
Link Menu
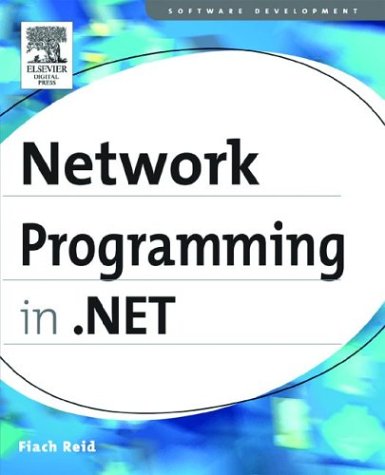
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PseudoWebRequest.cs
- NativeWrapper.cs
- InitiatorSessionSymmetricMessageSecurityProtocol.cs
- MarkupCompilePass1.cs
- XmlComment.cs
- MediaCommands.cs
- BlobPersonalizationState.cs
- MultipleViewPatternIdentifiers.cs
- TargetException.cs
- SrgsOneOf.cs
- MobileControlsSectionHandler.cs
- PenLineCapValidation.cs
- BamlRecordReader.cs
- EUCJPEncoding.cs
- RecognitionResult.cs
- ResourceBinder.cs
- DeclarativeCatalogPart.cs
- DataGridViewRowConverter.cs
- SystemGatewayIPAddressInformation.cs
- ExtensionQuery.cs
- DataServiceResponse.cs
- DataSetViewSchema.cs
- ModelTreeManager.cs
- Size3DConverter.cs
- EditorAttributeInfo.cs
- ToolTipService.cs
- HtmlTable.cs
- PieceNameHelper.cs
- PolicyStatement.cs
- IriParsingElement.cs
- Math.cs
- SystemResourceHost.cs
- ExpressionVisitorHelpers.cs
- State.cs
- NavigationWindow.cs
- XPathNodeIterator.cs
- RichTextBoxConstants.cs
- DataShape.cs
- RawStylusSystemGestureInputReport.cs
- ObjectDataSourceView.cs
- CodeGen.cs
- CmsUtils.cs
- ParameterCollection.cs
- BitmapMetadataBlob.cs
- SHA256Managed.cs
- XmlDomTextWriter.cs
- LayoutTable.cs
- PixelFormats.cs
- Debugger.cs
- GridItemPatternIdentifiers.cs
- GatewayDefinition.cs
- DesignerOptionService.cs
- HelpKeywordAttribute.cs
- DeclarationUpdate.cs
- LocalizabilityAttribute.cs
- Internal.cs
- UniqueID.cs
- DocumentPageView.cs
- MultiSelectRootGridEntry.cs
- ClientData.cs
- objectresult_tresulttype.cs
- FrameworkContentElement.cs
- FormattedTextSymbols.cs
- XmlWriterSettings.cs
- Pkcs7Signer.cs
- RotateTransform3D.cs
- DragEvent.cs
- _TransmitFileOverlappedAsyncResult.cs
- WebServiceErrorEvent.cs
- SafeArrayTypeMismatchException.cs
- AsyncPostBackErrorEventArgs.cs
- QilGenerator.cs
- AssemblyInfo.cs
- MultiSelectRootGridEntry.cs
- SplashScreenNativeMethods.cs
- CounterCreationData.cs
- DesignerTransactionCloseEvent.cs
- SendKeys.cs
- FileDialog_Vista.cs
- BitmapEffectGeneralTransform.cs
- UnicodeEncoding.cs
- MessageQueuePermissionEntry.cs
- RtfNavigator.cs
- DataGridViewColumnDesignTimeVisibleAttribute.cs
- ProxyGenerationError.cs
- InputElement.cs
- FileDialogCustomPlace.cs
- WebPartActionVerb.cs
- SecurityMode.cs
- BuildManager.cs
- SqlClientFactory.cs
- ResourceProperty.cs
- PerformanceCounterPermissionEntry.cs
- ForEachAction.cs
- ExpressionBuilderCollection.cs
- ImageListImage.cs
- SpellerInterop.cs
- Comparer.cs
- MenuItemStyle.cs
- DiscreteKeyFrames.cs