Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Documents / Block.cs / 1 / Block.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Block element. // Block element - an abstract class, a base for elements allowed // as siblings of Paragraphs. // //--------------------------------------------------------------------------- using System.ComponentModel; // TypeConverter using System.Windows.Controls; // Border using System.Windows.Media; // Brush using MS.Internal; // DoubleUtil using MS.Internal.Text; // Text DPI restrictions using MS.Internal.PtsHost.UnsafeNativeMethods; // PTS restrictions namespace System.Windows.Documents { ////// Block element - an abstract class, a base for elements allowed /// as siblings of Paragraphs. /// Only Blocks are allowed on top level of FlowDocument /// and other structural text elements like Section, ListItem, TableCell. /// public abstract class Block : TextElement { //------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- #region Public Properties ////// A collection of Blocks containing this Block in its sequential tree. /// May return null if this Block is not inserted into any tree. /// public BlockCollection SiblingBlocks { get { if (this.Parent == null) { return null; } return new BlockCollection(this, /*isOwnerParent*/false); } } ////// Returns a Block immediately following this one /// on the same level of siblings /// public Block NextBlock { get { return this.NextElement as Block; } } ////// Returns a block immediately preceding this one /// on the same level of siblings /// public Block PreviousBlock { get { return this.PreviousElement as Block; } } ////// DependencyProperty for hyphenation property. /// public static readonly DependencyProperty IsHyphenationEnabledProperty = DependencyProperty.RegisterAttached( "IsHyphenationEnabled", typeof(bool), typeof(Block), new FrameworkPropertyMetadata( false, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ////// CLR property for hyphenation /// public bool IsHyphenationEnabled { get { return (bool)GetValue(IsHyphenationEnabledProperty); } set { SetValue(IsHyphenationEnabledProperty, value); } } ////// DependencyProperty setter for /// The element to which to write the attached property. /// The property value to set public static void SetIsHyphenationEnabled(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(IsHyphenationEnabledProperty, value); } ///property. /// /// DependencyProperty getter for /// The element from which to read the attached property. public static bool GetIsHyphenationEnabled(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(IsHyphenationEnabledProperty); } ///property. /// /// DependencyProperty for public static readonly DependencyProperty MarginProperty = DependencyProperty.Register( "Margin", typeof(Thickness), typeof(Block), new FrameworkPropertyMetadata( new Thickness(), FrameworkPropertyMetadataOptions.AffectsMeasure), new ValidateValueCallback(IsValidMargin)); ///property. /// /// The Margin property specifies the margin of the element. /// public Thickness Margin { get { return (Thickness)GetValue(MarginProperty); } set { SetValue(MarginProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty PaddingProperty = DependencyProperty.Register( "Padding", typeof(Thickness), typeof(Block), new FrameworkPropertyMetadata( new Thickness(), FrameworkPropertyMetadataOptions.AffectsMeasure), new ValidateValueCallback(IsValidPadding)); ///property. /// /// The Padding property specifies the padding of the element. /// public Thickness Padding { get { return (Thickness)GetValue(PaddingProperty); } set { SetValue(PaddingProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty BorderThicknessProperty = DependencyProperty.Register( "BorderThickness", typeof(Thickness), typeof(Block), new FrameworkPropertyMetadata( new Thickness(), FrameworkPropertyMetadataOptions.AffectsMeasure), new ValidateValueCallback(IsValidBorderThickness)); ///property. /// /// The BorderThickness property specifies the border of the element. /// public Thickness BorderThickness { get { return (Thickness)GetValue(BorderThicknessProperty); } set { SetValue(BorderThicknessProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty BorderBrushProperty = DependencyProperty.Register( "BorderBrush", typeof(Brush), typeof(Block), new FrameworkPropertyMetadata( null, FrameworkPropertyMetadataOptions.AffectsRender)); ///property. /// /// The BorderBrush property specifies the brush of the border. /// public Brush BorderBrush { get { return (Brush)GetValue(BorderBrushProperty); } set { SetValue(BorderBrushProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty TextAlignmentProperty = DependencyProperty.RegisterAttached( "TextAlignment", typeof(TextAlignment), typeof(Block), new FrameworkPropertyMetadata( TextAlignment.Left, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits), new ValidateValueCallback(IsValidTextAlignment)); ///property. /// /// /// public TextAlignment TextAlignment { get { return (TextAlignment)GetValue(TextAlignmentProperty); } set { SetValue(TextAlignmentProperty, value); } } ////// DependencyProperty setter for /// The element to which to write the attached property. /// The property value to set public static void SetTextAlignment(DependencyObject element, TextAlignment value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(TextAlignmentProperty, value); } ///property. /// /// DependencyProperty getter for /// The element from which to read the attached property. public static TextAlignment GetTextAlignment(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (TextAlignment)element.GetValue(TextAlignmentProperty); } ///property. /// /// DependencyProperty for public static readonly DependencyProperty FlowDirectionProperty = FrameworkElement.FlowDirectionProperty.AddOwner(typeof(Block)); ///property. /// /// The FlowDirection property specifies the flow direction of the element. /// public FlowDirection FlowDirection { get { return (FlowDirection)GetValue(FlowDirectionProperty); } set { SetValue(FlowDirectionProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty LineHeightProperty = DependencyProperty.RegisterAttached( "LineHeight", typeof(double), typeof(Block), new FrameworkPropertyMetadata( double.NaN, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits), new ValidateValueCallback(IsValidLineHeight)); ///property. /// /// The LineHeight property specifies the height of each generated line box. /// [TypeConverter(typeof(LengthConverter))] public double LineHeight { get { return (double)GetValue(LineHeightProperty); } set { SetValue(LineHeightProperty, value); } } ////// DependencyProperty setter for /// The element to which to write the attached property. /// The property value to set public static void SetLineHeight(DependencyObject element, double value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(LineHeightProperty, value); } ///property. /// /// DependencyProperty getter for /// The element from which to read the attached property. [TypeConverter(typeof(LengthConverter))] public static double GetLineHeight(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (double)element.GetValue(LineHeightProperty); } ///property. /// /// DependencyProperty for public static readonly DependencyProperty LineStackingStrategyProperty = DependencyProperty.RegisterAttached( "LineStackingStrategy", typeof(LineStackingStrategy), typeof(Block), new FrameworkPropertyMetadata( LineStackingStrategy.MaxHeight, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits), new ValidateValueCallback(IsValidLineStackingStrategy)); ///property. /// /// The LineStackingStrategy property specifies how lines are placed /// public LineStackingStrategy LineStackingStrategy { get { return (LineStackingStrategy)GetValue(LineStackingStrategyProperty); } set { SetValue(LineStackingStrategyProperty, value); } } ////// DependencyProperty setter for /// The element to which to write the attached property. /// The property value to set public static void SetLineStackingStrategy(DependencyObject element, LineStackingStrategy value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(LineStackingStrategyProperty, value); } ///property. /// /// DependencyProperty getter for /// The element from which to read the attached property. public static LineStackingStrategy GetLineStackingStrategy(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (LineStackingStrategy)element.GetValue(LineStackingStrategyProperty); } ///property. /// /// Page break property, replaces PageBreak element. Indicates that a break should occur before this page. /// public static readonly DependencyProperty BreakPageBeforeProperty = DependencyProperty.Register( "BreakPageBefore", typeof(bool), typeof(Block), new FrameworkPropertyMetadata( false, FrameworkPropertyMetadataOptions.AffectsParentMeasure)); ////// The BreakPageBefore property indicates that a break should occur before this page /// public bool BreakPageBefore { get { return (bool)GetValue(BreakPageBeforeProperty); } set { SetValue(BreakPageBeforeProperty, value); } } ////// Column break property, replaces ColumnBreak element. Indicates that a break should occur before this column. /// public static readonly DependencyProperty BreakColumnBeforeProperty = DependencyProperty.Register( "BreakColumnBefore", typeof(bool), typeof(Block), new FrameworkPropertyMetadata( false, FrameworkPropertyMetadataOptions.AffectsParentMeasure)); ////// The BreakColumnBefore property indicates that a break should occur before this column /// public bool BreakColumnBefore { get { return (bool)GetValue(BreakColumnBeforeProperty); } set { SetValue(BreakColumnBeforeProperty, value); } } ////// ClearFloaters property, replaces FloaterClear element. Clears floater in specified WrapDirection /// public static readonly DependencyProperty ClearFloatersProperty = DependencyProperty.Register( "ClearFloaters", typeof(WrapDirection), typeof(Block), new FrameworkPropertyMetadata( WrapDirection.None, FrameworkPropertyMetadataOptions.AffectsParentMeasure), new ValidateValueCallback(IsValidWrapDirection)); ////// ClearFloaters property, replaces FloaterClear element. Clears floater in specified WrapDirection /// public WrapDirection ClearFloaters { get { return (WrapDirection)GetValue(ClearFloatersProperty); } set { SetValue(ClearFloatersProperty, value); } } #endregion Public Properties //-------------------------------------------------------------------- // // Internal Methods // //------------------------------------------------------------------- #region Internal Methods internal static bool IsValidMargin(object o) { Thickness t = (Thickness)o; return IsValidThickness(t, /*allow NaN*/true); } internal static bool IsValidPadding(object o) { Thickness t = (Thickness)o; return IsValidThickness(t, /*allow NaN*/true); } internal static bool IsValidBorderThickness(object o) { Thickness t = (Thickness)o; return IsValidThickness(t, /*allow NaN*/false); } #endregion Internal Methods //------------------------------------------------------ // // Internal Properties // //------------------------------------------------------ #region Internal Properties ////// Marks this element's left edge as visible to IMEs. /// This means element boundaries will act as word breaks. /// internal override bool IsIMEStructuralElement { get { return true; } } #endregion Internal Properties //------------------------------------------------------------------- // // Private Methods // //-------------------------------------------------------------------- #region Private Methods private static bool IsValidLineHeight(object o) { double lineHeight = (double)o; double minLineHeight = TextDpi.MinWidth; double maxLineHeight = Math.Min(1000000, PTS.MaxFontSize); if (Double.IsNaN(lineHeight)) { return true; } if (lineHeight < minLineHeight) { return false; } if (lineHeight > maxLineHeight) { return false; } return true; } private static bool IsValidLineStackingStrategy(object o) { LineStackingStrategy value = (LineStackingStrategy)o; return (value == LineStackingStrategy.MaxHeight || value == LineStackingStrategy.BlockLineHeight); } private static bool IsValidTextAlignment(object o) { TextAlignment value = (TextAlignment)o; return value == TextAlignment.Center || value == TextAlignment.Justify || value == TextAlignment.Left || value == TextAlignment.Right; } private static bool IsValidWrapDirection(object o) { WrapDirection value = (WrapDirection)o; return value == WrapDirection.None || value == WrapDirection.Left || value == WrapDirection.Right || value == WrapDirection.Both; } internal static bool IsValidThickness(Thickness t, bool allowNaN) { double maxThickness = Math.Min(1000000, PTS.MaxPageSize); if (!allowNaN) { if (Double.IsNaN(t.Left) || Double.IsNaN(t.Right) || Double.IsNaN(t.Top) || Double.IsNaN(t.Bottom)) { return false; } } if (!Double.IsNaN(t.Left) && (t.Left < 0 || t.Left > maxThickness)) { return false; } if (!Double.IsNaN(t.Right) && (t.Right < 0 || t.Right > maxThickness)) { return false; } if (!Double.IsNaN(t.Top) && (t.Top < 0 || t.Top > maxThickness)) { return false; } if (!Double.IsNaN(t.Bottom) && (t.Bottom < 0 || t.Bottom > maxThickness)) { return false; } return true; } #endregion Private Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Block element. // Block element - an abstract class, a base for elements allowed // as siblings of Paragraphs. // //--------------------------------------------------------------------------- using System.ComponentModel; // TypeConverter using System.Windows.Controls; // Border using System.Windows.Media; // Brush using MS.Internal; // DoubleUtil using MS.Internal.Text; // Text DPI restrictions using MS.Internal.PtsHost.UnsafeNativeMethods; // PTS restrictions namespace System.Windows.Documents { ////// Block element - an abstract class, a base for elements allowed /// as siblings of Paragraphs. /// Only Blocks are allowed on top level of FlowDocument /// and other structural text elements like Section, ListItem, TableCell. /// public abstract class Block : TextElement { //------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- #region Public Properties ////// A collection of Blocks containing this Block in its sequential tree. /// May return null if this Block is not inserted into any tree. /// public BlockCollection SiblingBlocks { get { if (this.Parent == null) { return null; } return new BlockCollection(this, /*isOwnerParent*/false); } } ////// Returns a Block immediately following this one /// on the same level of siblings /// public Block NextBlock { get { return this.NextElement as Block; } } ////// Returns a block immediately preceding this one /// on the same level of siblings /// public Block PreviousBlock { get { return this.PreviousElement as Block; } } ////// DependencyProperty for hyphenation property. /// public static readonly DependencyProperty IsHyphenationEnabledProperty = DependencyProperty.RegisterAttached( "IsHyphenationEnabled", typeof(bool), typeof(Block), new FrameworkPropertyMetadata( false, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits)); ////// CLR property for hyphenation /// public bool IsHyphenationEnabled { get { return (bool)GetValue(IsHyphenationEnabledProperty); } set { SetValue(IsHyphenationEnabledProperty, value); } } ////// DependencyProperty setter for /// The element to which to write the attached property. /// The property value to set public static void SetIsHyphenationEnabled(DependencyObject element, bool value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(IsHyphenationEnabledProperty, value); } ///property. /// /// DependencyProperty getter for /// The element from which to read the attached property. public static bool GetIsHyphenationEnabled(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (bool)element.GetValue(IsHyphenationEnabledProperty); } ///property. /// /// DependencyProperty for public static readonly DependencyProperty MarginProperty = DependencyProperty.Register( "Margin", typeof(Thickness), typeof(Block), new FrameworkPropertyMetadata( new Thickness(), FrameworkPropertyMetadataOptions.AffectsMeasure), new ValidateValueCallback(IsValidMargin)); ///property. /// /// The Margin property specifies the margin of the element. /// public Thickness Margin { get { return (Thickness)GetValue(MarginProperty); } set { SetValue(MarginProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty PaddingProperty = DependencyProperty.Register( "Padding", typeof(Thickness), typeof(Block), new FrameworkPropertyMetadata( new Thickness(), FrameworkPropertyMetadataOptions.AffectsMeasure), new ValidateValueCallback(IsValidPadding)); ///property. /// /// The Padding property specifies the padding of the element. /// public Thickness Padding { get { return (Thickness)GetValue(PaddingProperty); } set { SetValue(PaddingProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty BorderThicknessProperty = DependencyProperty.Register( "BorderThickness", typeof(Thickness), typeof(Block), new FrameworkPropertyMetadata( new Thickness(), FrameworkPropertyMetadataOptions.AffectsMeasure), new ValidateValueCallback(IsValidBorderThickness)); ///property. /// /// The BorderThickness property specifies the border of the element. /// public Thickness BorderThickness { get { return (Thickness)GetValue(BorderThicknessProperty); } set { SetValue(BorderThicknessProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty BorderBrushProperty = DependencyProperty.Register( "BorderBrush", typeof(Brush), typeof(Block), new FrameworkPropertyMetadata( null, FrameworkPropertyMetadataOptions.AffectsRender)); ///property. /// /// The BorderBrush property specifies the brush of the border. /// public Brush BorderBrush { get { return (Brush)GetValue(BorderBrushProperty); } set { SetValue(BorderBrushProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty TextAlignmentProperty = DependencyProperty.RegisterAttached( "TextAlignment", typeof(TextAlignment), typeof(Block), new FrameworkPropertyMetadata( TextAlignment.Left, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits), new ValidateValueCallback(IsValidTextAlignment)); ///property. /// /// /// public TextAlignment TextAlignment { get { return (TextAlignment)GetValue(TextAlignmentProperty); } set { SetValue(TextAlignmentProperty, value); } } ////// DependencyProperty setter for /// The element to which to write the attached property. /// The property value to set public static void SetTextAlignment(DependencyObject element, TextAlignment value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(TextAlignmentProperty, value); } ///property. /// /// DependencyProperty getter for /// The element from which to read the attached property. public static TextAlignment GetTextAlignment(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (TextAlignment)element.GetValue(TextAlignmentProperty); } ///property. /// /// DependencyProperty for public static readonly DependencyProperty FlowDirectionProperty = FrameworkElement.FlowDirectionProperty.AddOwner(typeof(Block)); ///property. /// /// The FlowDirection property specifies the flow direction of the element. /// public FlowDirection FlowDirection { get { return (FlowDirection)GetValue(FlowDirectionProperty); } set { SetValue(FlowDirectionProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty LineHeightProperty = DependencyProperty.RegisterAttached( "LineHeight", typeof(double), typeof(Block), new FrameworkPropertyMetadata( double.NaN, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits), new ValidateValueCallback(IsValidLineHeight)); ///property. /// /// The LineHeight property specifies the height of each generated line box. /// [TypeConverter(typeof(LengthConverter))] public double LineHeight { get { return (double)GetValue(LineHeightProperty); } set { SetValue(LineHeightProperty, value); } } ////// DependencyProperty setter for /// The element to which to write the attached property. /// The property value to set public static void SetLineHeight(DependencyObject element, double value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(LineHeightProperty, value); } ///property. /// /// DependencyProperty getter for /// The element from which to read the attached property. [TypeConverter(typeof(LengthConverter))] public static double GetLineHeight(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (double)element.GetValue(LineHeightProperty); } ///property. /// /// DependencyProperty for public static readonly DependencyProperty LineStackingStrategyProperty = DependencyProperty.RegisterAttached( "LineStackingStrategy", typeof(LineStackingStrategy), typeof(Block), new FrameworkPropertyMetadata( LineStackingStrategy.MaxHeight, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender | FrameworkPropertyMetadataOptions.Inherits), new ValidateValueCallback(IsValidLineStackingStrategy)); ///property. /// /// The LineStackingStrategy property specifies how lines are placed /// public LineStackingStrategy LineStackingStrategy { get { return (LineStackingStrategy)GetValue(LineStackingStrategyProperty); } set { SetValue(LineStackingStrategyProperty, value); } } ////// DependencyProperty setter for /// The element to which to write the attached property. /// The property value to set public static void SetLineStackingStrategy(DependencyObject element, LineStackingStrategy value) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(LineStackingStrategyProperty, value); } ///property. /// /// DependencyProperty getter for /// The element from which to read the attached property. public static LineStackingStrategy GetLineStackingStrategy(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return (LineStackingStrategy)element.GetValue(LineStackingStrategyProperty); } ///property. /// /// Page break property, replaces PageBreak element. Indicates that a break should occur before this page. /// public static readonly DependencyProperty BreakPageBeforeProperty = DependencyProperty.Register( "BreakPageBefore", typeof(bool), typeof(Block), new FrameworkPropertyMetadata( false, FrameworkPropertyMetadataOptions.AffectsParentMeasure)); ////// The BreakPageBefore property indicates that a break should occur before this page /// public bool BreakPageBefore { get { return (bool)GetValue(BreakPageBeforeProperty); } set { SetValue(BreakPageBeforeProperty, value); } } ////// Column break property, replaces ColumnBreak element. Indicates that a break should occur before this column. /// public static readonly DependencyProperty BreakColumnBeforeProperty = DependencyProperty.Register( "BreakColumnBefore", typeof(bool), typeof(Block), new FrameworkPropertyMetadata( false, FrameworkPropertyMetadataOptions.AffectsParentMeasure)); ////// The BreakColumnBefore property indicates that a break should occur before this column /// public bool BreakColumnBefore { get { return (bool)GetValue(BreakColumnBeforeProperty); } set { SetValue(BreakColumnBeforeProperty, value); } } ////// ClearFloaters property, replaces FloaterClear element. Clears floater in specified WrapDirection /// public static readonly DependencyProperty ClearFloatersProperty = DependencyProperty.Register( "ClearFloaters", typeof(WrapDirection), typeof(Block), new FrameworkPropertyMetadata( WrapDirection.None, FrameworkPropertyMetadataOptions.AffectsParentMeasure), new ValidateValueCallback(IsValidWrapDirection)); ////// ClearFloaters property, replaces FloaterClear element. Clears floater in specified WrapDirection /// public WrapDirection ClearFloaters { get { return (WrapDirection)GetValue(ClearFloatersProperty); } set { SetValue(ClearFloatersProperty, value); } } #endregion Public Properties //-------------------------------------------------------------------- // // Internal Methods // //------------------------------------------------------------------- #region Internal Methods internal static bool IsValidMargin(object o) { Thickness t = (Thickness)o; return IsValidThickness(t, /*allow NaN*/true); } internal static bool IsValidPadding(object o) { Thickness t = (Thickness)o; return IsValidThickness(t, /*allow NaN*/true); } internal static bool IsValidBorderThickness(object o) { Thickness t = (Thickness)o; return IsValidThickness(t, /*allow NaN*/false); } #endregion Internal Methods //------------------------------------------------------ // // Internal Properties // //------------------------------------------------------ #region Internal Properties ////// Marks this element's left edge as visible to IMEs. /// This means element boundaries will act as word breaks. /// internal override bool IsIMEStructuralElement { get { return true; } } #endregion Internal Properties //------------------------------------------------------------------- // // Private Methods // //-------------------------------------------------------------------- #region Private Methods private static bool IsValidLineHeight(object o) { double lineHeight = (double)o; double minLineHeight = TextDpi.MinWidth; double maxLineHeight = Math.Min(1000000, PTS.MaxFontSize); if (Double.IsNaN(lineHeight)) { return true; } if (lineHeight < minLineHeight) { return false; } if (lineHeight > maxLineHeight) { return false; } return true; } private static bool IsValidLineStackingStrategy(object o) { LineStackingStrategy value = (LineStackingStrategy)o; return (value == LineStackingStrategy.MaxHeight || value == LineStackingStrategy.BlockLineHeight); } private static bool IsValidTextAlignment(object o) { TextAlignment value = (TextAlignment)o; return value == TextAlignment.Center || value == TextAlignment.Justify || value == TextAlignment.Left || value == TextAlignment.Right; } private static bool IsValidWrapDirection(object o) { WrapDirection value = (WrapDirection)o; return value == WrapDirection.None || value == WrapDirection.Left || value == WrapDirection.Right || value == WrapDirection.Both; } internal static bool IsValidThickness(Thickness t, bool allowNaN) { double maxThickness = Math.Min(1000000, PTS.MaxPageSize); if (!allowNaN) { if (Double.IsNaN(t.Left) || Double.IsNaN(t.Right) || Double.IsNaN(t.Top) || Double.IsNaN(t.Bottom)) { return false; } } if (!Double.IsNaN(t.Left) && (t.Left < 0 || t.Left > maxThickness)) { return false; } if (!Double.IsNaN(t.Right) && (t.Right < 0 || t.Right > maxThickness)) { return false; } if (!Double.IsNaN(t.Top) && (t.Top < 0 || t.Top > maxThickness)) { return false; } if (!Double.IsNaN(t.Bottom) && (t.Bottom < 0 || t.Bottom > maxThickness)) { return false; } return true; } #endregion Private Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
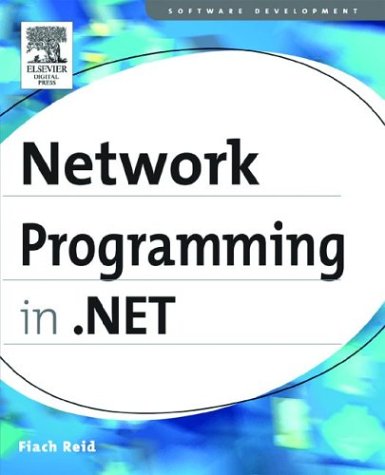
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HTMLTagNameToTypeMapper.cs
- QueryStringParameter.cs
- HttpRuntimeSection.cs
- BehaviorEditorPart.cs
- RootBrowserWindow.cs
- XmlConverter.cs
- SqlBulkCopyColumnMappingCollection.cs
- VectorValueSerializer.cs
- ItemCollection.cs
- TextRangeBase.cs
- ItemChangedEventArgs.cs
- QueueSurrogate.cs
- WorkflowInstanceUnhandledExceptionRecord.cs
- RowsCopiedEventArgs.cs
- FileChangesMonitor.cs
- ResizeGrip.cs
- WebPartConnectionsCancelVerb.cs
- EncryptedKeyIdentifierClause.cs
- ReflectEventDescriptor.cs
- CallSiteOps.cs
- Object.cs
- PropertyItem.cs
- MemberInitExpression.cs
- SqlGenericUtil.cs
- TypeDescriptionProviderAttribute.cs
- TextLineResult.cs
- StyleSheetComponentEditor.cs
- OuterGlowBitmapEffect.cs
- WaitForChangedResult.cs
- SkewTransform.cs
- Token.cs
- LoginUtil.cs
- StreamReader.cs
- PopupControlService.cs
- LayoutExceptionEventArgs.cs
- TextPatternIdentifiers.cs
- RIPEMD160Managed.cs
- printdlgexmarshaler.cs
- SecurityMode.cs
- ClientScriptManager.cs
- OlePropertyStructs.cs
- ConvertEvent.cs
- KeyConverter.cs
- OperationResponse.cs
- TimeSpanMinutesConverter.cs
- TripleDESCryptoServiceProvider.cs
- SmiRequestExecutor.cs
- OleDbMetaDataFactory.cs
- EmissiveMaterial.cs
- ControlValuePropertyAttribute.cs
- DescendantQuery.cs
- Menu.cs
- SrgsRuleRef.cs
- InternalConfigSettingsFactory.cs
- EdmRelationshipRoleAttribute.cs
- DesignerExtenders.cs
- NetworkInformationException.cs
- DataGridRowClipboardEventArgs.cs
- ItemContainerGenerator.cs
- RewritingValidator.cs
- prompt.cs
- SoapAttributes.cs
- Helper.cs
- UserControlAutomationPeer.cs
- XmlSchemaException.cs
- XmlDomTextWriter.cs
- MessageQueue.cs
- ValidatorCompatibilityHelper.cs
- FontUnit.cs
- CacheRequest.cs
- ProfessionalColors.cs
- DrawingContextDrawingContextWalker.cs
- SemanticBasicElement.cs
- DataServiceKeyAttribute.cs
- ListMarkerSourceInfo.cs
- SecurityPolicySection.cs
- BitConverter.cs
- DatatypeImplementation.cs
- CodeAttributeDeclarationCollection.cs
- WindowsProgressbar.cs
- VectorAnimationBase.cs
- ExpressionEvaluator.cs
- BitmapSizeOptions.cs
- DebugController.cs
- RepeatButtonAutomationPeer.cs
- Helper.cs
- Viewport3DAutomationPeer.cs
- XsltLibrary.cs
- CopyAttributesAction.cs
- XmlUtil.cs
- XsltOutput.cs
- BinaryConverter.cs
- FixedBufferAttribute.cs
- ErrorFormatterPage.cs
- ProcessProtocolHandler.cs
- PreservationFileReader.cs
- MemberHolder.cs
- SecureStringHasher.cs
- COM2IProvidePropertyBuilderHandler.cs
- EncoderFallback.cs