Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / MS / Internal / IO / Packaging / xmlfixedPageInfo.cs / 1 / xmlfixedPageInfo.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Implements a DOM-based subclass of the FixedPageInfo abstract class. // The class functions as an array of XmlGlyphRunInfo's in markup order. // // History: // 05/06/2004: JohnLarc: Initial implementation //--------------------------------------------------------------------------- using System; using System.Windows; // For ExceptionStringTable using System.Xml; // For DOM objects using System.Diagnostics; // For Assert using System.Globalization; // For CultureInfo namespace MS.Internal.IO.Packaging { internal class XmlFixedPageInfo : MS.Internal.FixedPageInfo { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Initialize object from DOM node. /// ////// The DOM node is assumed to be a XAML FixedPage element. Its namespace URI /// is subsequently used to look for its nested Glyphs elements (see private property NodeList). /// internal XmlFixedPageInfo(XmlNode fixedPageNode) { _pageNode = fixedPageNode; Debug.Assert(_pageNode != null); if (_pageNode.LocalName != _fixedPageName || _pageNode.NamespaceURI != ElementTableKey.FixedMarkupNamespace) { throw new ArgumentException(SR.Get(SRID.UnexpectedXmlNodeInXmlFixedPageInfoConstructor, _pageNode.NamespaceURI, _pageNode.LocalName, ElementTableKey.FixedMarkupNamespace, _fixedPageName)); } } #endregion Constructors //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- #region Internal Methods ////// Get the glyph run at zero-based position 'position'. /// ////// Returns null for a nonexistent position. No exception raised. /// internal override GlyphRunInfo GlyphRunAtPosition(int position) { if (position < 0 || position >= GlyphRunList.Length) { return null; } if (GlyphRunList[position] == null) { GlyphRunList[position] = new XmlGlyphRunInfo(NodeList[position]); } return GlyphRunList[position]; } #endregion Internal Methods //------------------------------------------------------ // // Internal Properties // //------------------------------------------------------ #region Internal Properties ////// Indicates the number of glyph runs on the page. /// internal override int GlyphRunCount { get { return GlyphRunList.Length; } } #endregion Internal Properties //----------------------------------------------------- // // Private Properties // //------------------------------------------------------ #region Private Properties ////// Lazily initialize _glyphRunList, an array of XmlGlyphInfo objects, /// using the NodeList private property. /// ////// When using Visual Studio to step through code using this property, make sure the option /// "Allow property evaluation in variables windows" is unchecked. /// private XmlGlyphRunInfo[] GlyphRunList { get { if (_glyphRunList == null) { _glyphRunList = new XmlGlyphRunInfo[NodeList.Count]; } return _glyphRunList; } } ////// Lazily initialize the list of Glyphs elements on the page using XPath. /// ////// When using Visual Studio to step through code using this property, make sure the option /// "Allow property evaluation in variables windows" is unchecked. /// private XmlNodeList NodeList { get { if (_nodeList == null) { string glyphRunQuery = String.Format(CultureInfo.InvariantCulture, ".//*[namespace-uri()='{0}' and local-name()='{1}']", ElementTableKey.FixedMarkupNamespace, _glyphRunName); _nodeList = _pageNode.SelectNodes(glyphRunQuery); } return _nodeList; } } #endregion Private Properties //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields #region Constants private const string _fixedPageName = "FixedPage"; private const string _glyphRunName = "Glyphs"; #endregion Constants private XmlNode _pageNode; private XmlNodeList _nodeList = null; private XmlGlyphRunInfo[] _glyphRunList = null; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Implements a DOM-based subclass of the FixedPageInfo abstract class. // The class functions as an array of XmlGlyphRunInfo's in markup order. // // History: // 05/06/2004: JohnLarc: Initial implementation //--------------------------------------------------------------------------- using System; using System.Windows; // For ExceptionStringTable using System.Xml; // For DOM objects using System.Diagnostics; // For Assert using System.Globalization; // For CultureInfo namespace MS.Internal.IO.Packaging { internal class XmlFixedPageInfo : MS.Internal.FixedPageInfo { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Initialize object from DOM node. /// ////// The DOM node is assumed to be a XAML FixedPage element. Its namespace URI /// is subsequently used to look for its nested Glyphs elements (see private property NodeList). /// internal XmlFixedPageInfo(XmlNode fixedPageNode) { _pageNode = fixedPageNode; Debug.Assert(_pageNode != null); if (_pageNode.LocalName != _fixedPageName || _pageNode.NamespaceURI != ElementTableKey.FixedMarkupNamespace) { throw new ArgumentException(SR.Get(SRID.UnexpectedXmlNodeInXmlFixedPageInfoConstructor, _pageNode.NamespaceURI, _pageNode.LocalName, ElementTableKey.FixedMarkupNamespace, _fixedPageName)); } } #endregion Constructors //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- #region Internal Methods ////// Get the glyph run at zero-based position 'position'. /// ////// Returns null for a nonexistent position. No exception raised. /// internal override GlyphRunInfo GlyphRunAtPosition(int position) { if (position < 0 || position >= GlyphRunList.Length) { return null; } if (GlyphRunList[position] == null) { GlyphRunList[position] = new XmlGlyphRunInfo(NodeList[position]); } return GlyphRunList[position]; } #endregion Internal Methods //------------------------------------------------------ // // Internal Properties // //------------------------------------------------------ #region Internal Properties ////// Indicates the number of glyph runs on the page. /// internal override int GlyphRunCount { get { return GlyphRunList.Length; } } #endregion Internal Properties //----------------------------------------------------- // // Private Properties // //------------------------------------------------------ #region Private Properties ////// Lazily initialize _glyphRunList, an array of XmlGlyphInfo objects, /// using the NodeList private property. /// ////// When using Visual Studio to step through code using this property, make sure the option /// "Allow property evaluation in variables windows" is unchecked. /// private XmlGlyphRunInfo[] GlyphRunList { get { if (_glyphRunList == null) { _glyphRunList = new XmlGlyphRunInfo[NodeList.Count]; } return _glyphRunList; } } ////// Lazily initialize the list of Glyphs elements on the page using XPath. /// ////// When using Visual Studio to step through code using this property, make sure the option /// "Allow property evaluation in variables windows" is unchecked. /// private XmlNodeList NodeList { get { if (_nodeList == null) { string glyphRunQuery = String.Format(CultureInfo.InvariantCulture, ".//*[namespace-uri()='{0}' and local-name()='{1}']", ElementTableKey.FixedMarkupNamespace, _glyphRunName); _nodeList = _pageNode.SelectNodes(glyphRunQuery); } return _nodeList; } } #endregion Private Properties //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields #region Constants private const string _fixedPageName = "FixedPage"; private const string _glyphRunName = "Glyphs"; #endregion Constants private XmlNode _pageNode; private XmlNodeList _nodeList = null; private XmlGlyphRunInfo[] _glyphRunList = null; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
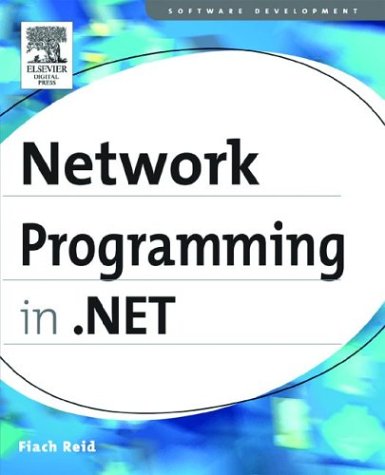
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SetStoryboardSpeedRatio.cs
- Merger.cs
- GraphicsContainer.cs
- HttpCookie.cs
- DesignerTextViewAdapter.cs
- SynchronizedDispatch.cs
- TabPage.cs
- DescendentsWalkerBase.cs
- WhileDesigner.xaml.cs
- FileSystemInfo.cs
- AppDomainResourcePerfCounters.cs
- ThreadStateException.cs
- TextTreeInsertElementUndoUnit.cs
- storepermission.cs
- TrackBar.cs
- WSTrustDec2005.cs
- SystemMulticastIPAddressInformation.cs
- SafeArrayRankMismatchException.cs
- VolatileEnlistmentState.cs
- IntegerFacetDescriptionElement.cs
- RectangleGeometry.cs
- ToolStripTextBox.cs
- RevocationPoint.cs
- RulePatternOps.cs
- ReplyChannelBinder.cs
- BindingExpressionUncommonField.cs
- DataListCommandEventArgs.cs
- DateTimeValueSerializerContext.cs
- DSASignatureFormatter.cs
- XamlRtfConverter.cs
- CancellationState.cs
- AttributeProviderAttribute.cs
- NegationPusher.cs
- StorageInfo.cs
- Parameter.cs
- ObjectComplexPropertyMapping.cs
- ActivityTrace.cs
- InputReport.cs
- EntityDesignerUtils.cs
- EntityObject.cs
- PaperSource.cs
- TraceHelpers.cs
- BuildManager.cs
- HttpInputStream.cs
- WebPartEditorCancelVerb.cs
- BitmapEffectDrawing.cs
- CreateUserWizard.cs
- PermissionRequestEvidence.cs
- Executor.cs
- ConnectorRouter.cs
- HttpContext.cs
- RepeatBehavior.cs
- VisualBrush.cs
- Stream.cs
- XmlIlVisitor.cs
- DiscardableAttribute.cs
- XMLSyntaxException.cs
- OutputCacheProfile.cs
- DropTarget.cs
- GridViewSortEventArgs.cs
- CheckBox.cs
- Utility.cs
- MetaDataInfo.cs
- MetadataConversionError.cs
- nulltextcontainer.cs
- RSAProtectedConfigurationProvider.cs
- XmlSchemaException.cs
- FindCriteriaElement.cs
- ValueUnavailableException.cs
- TextTreeUndo.cs
- CallTemplateAction.cs
- PostBackTrigger.cs
- ErrorWebPart.cs
- MsmqEncryptionAlgorithm.cs
- HttpDebugHandler.cs
- TraceSection.cs
- DoubleLink.cs
- ToolStripCodeDomSerializer.cs
- ResponseBodyWriter.cs
- Highlights.cs
- RadioButton.cs
- ToolStripPanelRenderEventArgs.cs
- ArrangedElementCollection.cs
- SecurityVersion.cs
- SQLRoleProvider.cs
- NetworkInterface.cs
- NamedObject.cs
- ServiceNameElementCollection.cs
- EncryptedHeaderXml.cs
- VirtualPathProvider.cs
- Convert.cs
- ImageKeyConverter.cs
- SizeConverter.cs
- StylusDownEventArgs.cs
- AuthenticationModuleElementCollection.cs
- Transform.cs
- ConfigXmlComment.cs
- Message.cs
- TaskFactory.cs
- ScrollViewer.cs