Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Media3D / Size3D.cs / 1 / Size3D.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: 3D size implementation. // // // // History: // 06/26/2003 : t-gregr - Created // //--------------------------------------------------------------------------- using System.Windows; using System.Windows.Media.Media3D; using System; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Media3D { ////// Size3D - A value type which defined a size in terms of non-negative width, /// length, and height. /// public partial struct Size3D { #region Constructors ////// Constructor which sets the size's initial values. Values must be non-negative. /// /// X dimension of the new size. /// Y dimension of the new size. /// Z dimension of the new size. public Size3D(double x, double y, double z) { if (x < 0 || y < 0 || z < 0) { throw new System.ArgumentException(SR.Get(SRID.Size3D_DimensionCannotBeNegative)); } _x = x; _y = y; _z = z; } #endregion Constructors #region Statics ////// Empty - a static property which provides an Empty size. X, Y, and Z are /// negative-infinity. This is the only situation /// where size can be negative. /// public static Size3D Empty { get { return s_empty; } } #endregion Statics #region Public Methods and Properties ////// IsEmpty - this returns true if this size is the Empty size. /// Note: If size is 0 this Size3D still contains a 0, 1, or 2 dimensional set /// of points, so this method should not be used to check for 0 volume. /// public bool IsEmpty { get { return _x < 0; } } ////// Size in X dimension. Default is 0, must be non-negative. /// public double X { get { return _x; } set { if (IsEmpty) { throw new System.InvalidOperationException(SR.Get(SRID.Size3D_CannotModifyEmptySize)); } if (value < 0) { throw new System.ArgumentException(SR.Get(SRID.Size3D_DimensionCannotBeNegative)); } _x = value; } } ////// Size in Y dimension. Default is 0, must be non-negative. /// public double Y { get { return _y; } set { if (IsEmpty) { throw new System.InvalidOperationException(SR.Get(SRID.Size3D_CannotModifyEmptySize)); } if (value < 0) { throw new System.ArgumentException(SR.Get(SRID.Size3D_DimensionCannotBeNegative)); } _y = value; } } ////// Size in Z dimension. Default is 0, must be non-negative. /// public double Z { get { return _z; } set { if (IsEmpty) { throw new System.InvalidOperationException(SR.Get(SRID.Size3D_CannotModifyEmptySize)); } if (value < 0) { throw new System.ArgumentException(SR.Get(SRID.Size3D_DimensionCannotBeNegative)); } _z = value; } } #endregion Public Methods #region Public Operators ////// Explicit conversion to Vector. /// /// The size to convert to a vector. ///A vector equal to this size. public static explicit operator Vector3D(Size3D size) { return new Vector3D(size._x, size._y, size._z); } ////// Explicit conversion to point. /// /// The size to convert to a point. ///A point equal to this size. public static explicit operator Point3D(Size3D size) { return new Point3D(size._x, size._y, size._z); } #endregion Public Operators #region Private Methods private static Size3D CreateEmptySize3D() { Size3D empty = new Size3D(); // Can't use setters because they throw on negative values empty._x = Double.NegativeInfinity; empty._y = Double.NegativeInfinity; empty._z = Double.NegativeInfinity; return empty; } #endregion Private Methods #region Private Fields private readonly static Size3D s_empty = CreateEmptySize3D(); #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: 3D size implementation. // // // // History: // 06/26/2003 : t-gregr - Created // //--------------------------------------------------------------------------- using System.Windows; using System.Windows.Media.Media3D; using System; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Media3D { ////// Size3D - A value type which defined a size in terms of non-negative width, /// length, and height. /// public partial struct Size3D { #region Constructors ////// Constructor which sets the size's initial values. Values must be non-negative. /// /// X dimension of the new size. /// Y dimension of the new size. /// Z dimension of the new size. public Size3D(double x, double y, double z) { if (x < 0 || y < 0 || z < 0) { throw new System.ArgumentException(SR.Get(SRID.Size3D_DimensionCannotBeNegative)); } _x = x; _y = y; _z = z; } #endregion Constructors #region Statics ////// Empty - a static property which provides an Empty size. X, Y, and Z are /// negative-infinity. This is the only situation /// where size can be negative. /// public static Size3D Empty { get { return s_empty; } } #endregion Statics #region Public Methods and Properties ////// IsEmpty - this returns true if this size is the Empty size. /// Note: If size is 0 this Size3D still contains a 0, 1, or 2 dimensional set /// of points, so this method should not be used to check for 0 volume. /// public bool IsEmpty { get { return _x < 0; } } ////// Size in X dimension. Default is 0, must be non-negative. /// public double X { get { return _x; } set { if (IsEmpty) { throw new System.InvalidOperationException(SR.Get(SRID.Size3D_CannotModifyEmptySize)); } if (value < 0) { throw new System.ArgumentException(SR.Get(SRID.Size3D_DimensionCannotBeNegative)); } _x = value; } } ////// Size in Y dimension. Default is 0, must be non-negative. /// public double Y { get { return _y; } set { if (IsEmpty) { throw new System.InvalidOperationException(SR.Get(SRID.Size3D_CannotModifyEmptySize)); } if (value < 0) { throw new System.ArgumentException(SR.Get(SRID.Size3D_DimensionCannotBeNegative)); } _y = value; } } ////// Size in Z dimension. Default is 0, must be non-negative. /// public double Z { get { return _z; } set { if (IsEmpty) { throw new System.InvalidOperationException(SR.Get(SRID.Size3D_CannotModifyEmptySize)); } if (value < 0) { throw new System.ArgumentException(SR.Get(SRID.Size3D_DimensionCannotBeNegative)); } _z = value; } } #endregion Public Methods #region Public Operators ////// Explicit conversion to Vector. /// /// The size to convert to a vector. ///A vector equal to this size. public static explicit operator Vector3D(Size3D size) { return new Vector3D(size._x, size._y, size._z); } ////// Explicit conversion to point. /// /// The size to convert to a point. ///A point equal to this size. public static explicit operator Point3D(Size3D size) { return new Point3D(size._x, size._y, size._z); } #endregion Public Operators #region Private Methods private static Size3D CreateEmptySize3D() { Size3D empty = new Size3D(); // Can't use setters because they throw on negative values empty._x = Double.NegativeInfinity; empty._y = Double.NegativeInfinity; empty._z = Double.NegativeInfinity; return empty; } #endregion Private Methods #region Private Fields private readonly static Size3D s_empty = CreateEmptySize3D(); #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
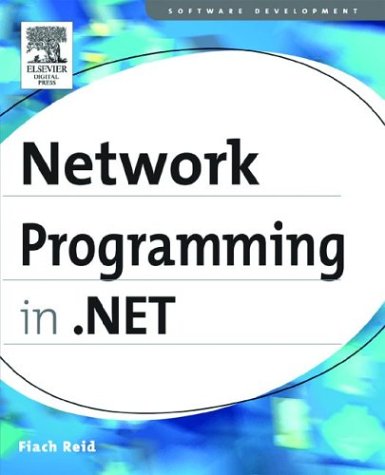
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FunctionDetailsReader.cs
- odbcmetadatacollectionnames.cs
- Canonicalizers.cs
- ChtmlImageAdapter.cs
- BoundsDrawingContextWalker.cs
- ManagedWndProcTracker.cs
- ListViewItem.cs
- ComboBoxRenderer.cs
- Currency.cs
- Rfc2898DeriveBytes.cs
- HttpException.cs
- NestPullup.cs
- TemplatePartAttribute.cs
- SQLString.cs
- Gdiplus.cs
- AuthenticationException.cs
- WebConvert.cs
- UInt16.cs
- CodeSnippetTypeMember.cs
- Setter.cs
- Stack.cs
- BamlCollectionHolder.cs
- DataSourceControlBuilder.cs
- ConstantSlot.cs
- MarkupExtensionParser.cs
- FunctionOverloadResolver.cs
- PassportIdentity.cs
- Material.cs
- FamilyTypefaceCollection.cs
- ValidatingCollection.cs
- ResourceDisplayNameAttribute.cs
- ColorContext.cs
- AutomationIdentifier.cs
- XmlDataSourceNodeDescriptor.cs
- PairComparer.cs
- TextDecorationLocationValidation.cs
- StylusPointDescription.cs
- NCryptNative.cs
- CharacterBufferReference.cs
- _TransmitFileOverlappedAsyncResult.cs
- StylusPointPropertyId.cs
- TypedTableHandler.cs
- SQLMembershipProvider.cs
- ClientOperation.cs
- FactoryMaker.cs
- EntitySet.cs
- Version.cs
- TextViewSelectionProcessor.cs
- Types.cs
- ConstructorExpr.cs
- DataSetFieldSchema.cs
- wgx_render.cs
- TimerEventSubscription.cs
- LinqDataSourceContextEventArgs.cs
- TableCell.cs
- Int64KeyFrameCollection.cs
- RegexEditorDialog.cs
- DescendentsWalker.cs
- HandlerWithFactory.cs
- FileIOPermission.cs
- EdmSchemaAttribute.cs
- ScrollProviderWrapper.cs
- XmlWrappingReader.cs
- SmtpSpecifiedPickupDirectoryElement.cs
- HostingPreferredMapPath.cs
- ProfileEventArgs.cs
- SmtpClient.cs
- BatchParser.cs
- __Filters.cs
- RSAOAEPKeyExchangeFormatter.cs
- ComboBoxItem.cs
- StringSource.cs
- GeneratedView.cs
- XPathDocument.cs
- VariableModifiersHelper.cs
- ColorAnimationBase.cs
- Activity.cs
- COM2ExtendedTypeConverter.cs
- UrlPath.cs
- AuthenticationSection.cs
- LinqDataSourceEditData.cs
- XmlCustomFormatter.cs
- HttpCacheVary.cs
- WsatTransactionHeader.cs
- HtmlUtf8RawTextWriter.cs
- SecurityPermission.cs
- Point3D.cs
- RootDesignerSerializerAttribute.cs
- ComponentSerializationService.cs
- TextRunTypographyProperties.cs
- ControlDesignerState.cs
- CheckBoxRenderer.cs
- StylusDownEventArgs.cs
- NumberSubstitution.cs
- GroupByQueryOperator.cs
- PrintDocument.cs
- IndentedTextWriter.cs
- AssociationEndMember.cs
- RootProjectionNode.cs
- CalendarTable.cs