Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Input / TextServicesCompartment.cs / 1 / TextServicesCompartment.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Manages Text Services Compartment. // // History: // 07/30/2003 : yutakas - Ported from .net tree. // //--------------------------------------------------------------------------- using System; using System.Runtime.InteropServices; using System.Windows.Threading; using System.Security; using System.Security.Permissions; using System.Diagnostics; using System.Collections; using MS.Utility; using MS.Win32; using MS.Internal; namespace System.Windows.Input { //----------------------------------------------------- // // TextServicesCompartment class // //----------------------------------------------------- internal class TextServicesCompartment { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- ////// Critical - directly calls unmanaged code based on guid /// [SecurityCritical] internal TextServicesCompartment(Guid guid, UnsafeNativeMethods.ITfCompartmentMgr compartmentmgr) { _guid = guid; _compartmentmgr = new SecurityCriticalData(compartmentmgr); _cookie = UnsafeNativeMethods.TF_INVALID_COOKIE; } //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public Methods #endregion Public Methods //----------------------------------------------------- // // Public Operators // //------------------------------------------------------ //----------------------------------------------------- // // Public Properties // //----------------------------------------------------- //----------------------------------------------------- // // Public Events // //------------------------------------------------------ //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- #region Internal Methods /// /// Advise the notify sink of the compartment update. /// ////// Critical - wires up multiple unmanaged objects together /// [SecurityCritical] internal void AdviseNotifySink(UnsafeNativeMethods.ITfCompartmentEventSink sink) { Debug.Assert(_cookie == UnsafeNativeMethods.TF_INVALID_COOKIE, "cookie is already set."); UnsafeNativeMethods.ITfCompartment compartment = GetITfCompartment(); if (compartment == null) return; UnsafeNativeMethods.ITfSource source = compartment as UnsafeNativeMethods.ITfSource; // workaround because I can't pass a ref to a readonly constant Guid guid = UnsafeNativeMethods.IID_ITfCompartmentEventSink; source.AdviseSink(ref guid, sink, out _cookie); Marshal.ReleaseComObject(compartment); Marshal.ReleaseComObject(source); } ////// Unadvise the notify sink of the compartment update. /// ////// Critical - wires up multiple unmanaged objects together /// [SecurityCritical] internal void UnadviseNotifySink() { Debug.Assert(_cookie != UnsafeNativeMethods.TF_INVALID_COOKIE, "cookie is not set."); UnsafeNativeMethods.ITfCompartment compartment = GetITfCompartment(); if (compartment == null) return; UnsafeNativeMethods.ITfSource source = compartment as UnsafeNativeMethods.ITfSource; source.UnadviseSink(_cookie); _cookie = UnsafeNativeMethods.TF_INVALID_COOKIE; Marshal.ReleaseComObject(compartment); Marshal.ReleaseComObject(source); } ////// Retrieve ITfCompartment /// ////// Critical - returns critical resource /// [SecurityCritical] internal UnsafeNativeMethods.ITfCompartment GetITfCompartment() { UnsafeNativeMethods.ITfCompartment itfcompartment; _compartmentmgr.Value.GetCompartment(ref _guid, out itfcompartment); return itfcompartment; } #endregion Internal methods //------------------------------------------------------ // // Internal Properties // //----------------------------------------------------- #region Internal Properties ////// Cast the compartment variant to bool. /// internal bool BooleanValue { get { object obj = Value; if (obj == null) return false; if ((int)obj != 0) return true; return false; } set { Value = value ? 1 : 0; } } ////// Cast the compartment variant to int. /// internal int IntValue { get { object obj = Value; if (obj == null) return 0; return (int)obj; } set { Value = value; } } ////// Get the compartment variant. /// ////// Critical - access unmanaged code /// TreatAsSafe - returns "safe" variant based value from the store /// internal object Value { [SecurityCritical, SecurityTreatAsSafe] get { UnsafeNativeMethods.ITfCompartment compartment = GetITfCompartment(); if (compartment == null) return null; object obj; compartment.GetValue(out obj); Marshal.ReleaseComObject(compartment); return obj; } [SecurityCritical, SecurityTreatAsSafe] set { UnsafeNativeMethods.ITfCompartment compartment = GetITfCompartment(); if (compartment == null) return; compartment.SetValue(0 /* clientid */, ref value); Marshal.ReleaseComObject(compartment); } } #endregion Internal Properties //----------------------------------------------------- // // Private Methods // //----------------------------------------------------- //------------------------------------------------------ // // Private Fields // //----------------------------------------------------- #region Private Fields ////// Critical: UnsafeNativeMethods.ITfCompartmentMgr has methods with SuppressUnmanagedCodeSecurity. /// private readonly SecurityCriticalData_compartmentmgr; private Guid _guid; private int _cookie; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Manages Text Services Compartment. // // History: // 07/30/2003 : yutakas - Ported from .net tree. // //--------------------------------------------------------------------------- using System; using System.Runtime.InteropServices; using System.Windows.Threading; using System.Security; using System.Security.Permissions; using System.Diagnostics; using System.Collections; using MS.Utility; using MS.Win32; using MS.Internal; namespace System.Windows.Input { //----------------------------------------------------- // // TextServicesCompartment class // //----------------------------------------------------- internal class TextServicesCompartment { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- ////// Critical - directly calls unmanaged code based on guid /// [SecurityCritical] internal TextServicesCompartment(Guid guid, UnsafeNativeMethods.ITfCompartmentMgr compartmentmgr) { _guid = guid; _compartmentmgr = new SecurityCriticalData(compartmentmgr); _cookie = UnsafeNativeMethods.TF_INVALID_COOKIE; } //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public Methods #endregion Public Methods //----------------------------------------------------- // // Public Operators // //------------------------------------------------------ //----------------------------------------------------- // // Public Properties // //----------------------------------------------------- //----------------------------------------------------- // // Public Events // //------------------------------------------------------ //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- #region Internal Methods /// /// Advise the notify sink of the compartment update. /// ////// Critical - wires up multiple unmanaged objects together /// [SecurityCritical] internal void AdviseNotifySink(UnsafeNativeMethods.ITfCompartmentEventSink sink) { Debug.Assert(_cookie == UnsafeNativeMethods.TF_INVALID_COOKIE, "cookie is already set."); UnsafeNativeMethods.ITfCompartment compartment = GetITfCompartment(); if (compartment == null) return; UnsafeNativeMethods.ITfSource source = compartment as UnsafeNativeMethods.ITfSource; // workaround because I can't pass a ref to a readonly constant Guid guid = UnsafeNativeMethods.IID_ITfCompartmentEventSink; source.AdviseSink(ref guid, sink, out _cookie); Marshal.ReleaseComObject(compartment); Marshal.ReleaseComObject(source); } ////// Unadvise the notify sink of the compartment update. /// ////// Critical - wires up multiple unmanaged objects together /// [SecurityCritical] internal void UnadviseNotifySink() { Debug.Assert(_cookie != UnsafeNativeMethods.TF_INVALID_COOKIE, "cookie is not set."); UnsafeNativeMethods.ITfCompartment compartment = GetITfCompartment(); if (compartment == null) return; UnsafeNativeMethods.ITfSource source = compartment as UnsafeNativeMethods.ITfSource; source.UnadviseSink(_cookie); _cookie = UnsafeNativeMethods.TF_INVALID_COOKIE; Marshal.ReleaseComObject(compartment); Marshal.ReleaseComObject(source); } ////// Retrieve ITfCompartment /// ////// Critical - returns critical resource /// [SecurityCritical] internal UnsafeNativeMethods.ITfCompartment GetITfCompartment() { UnsafeNativeMethods.ITfCompartment itfcompartment; _compartmentmgr.Value.GetCompartment(ref _guid, out itfcompartment); return itfcompartment; } #endregion Internal methods //------------------------------------------------------ // // Internal Properties // //----------------------------------------------------- #region Internal Properties ////// Cast the compartment variant to bool. /// internal bool BooleanValue { get { object obj = Value; if (obj == null) return false; if ((int)obj != 0) return true; return false; } set { Value = value ? 1 : 0; } } ////// Cast the compartment variant to int. /// internal int IntValue { get { object obj = Value; if (obj == null) return 0; return (int)obj; } set { Value = value; } } ////// Get the compartment variant. /// ////// Critical - access unmanaged code /// TreatAsSafe - returns "safe" variant based value from the store /// internal object Value { [SecurityCritical, SecurityTreatAsSafe] get { UnsafeNativeMethods.ITfCompartment compartment = GetITfCompartment(); if (compartment == null) return null; object obj; compartment.GetValue(out obj); Marshal.ReleaseComObject(compartment); return obj; } [SecurityCritical, SecurityTreatAsSafe] set { UnsafeNativeMethods.ITfCompartment compartment = GetITfCompartment(); if (compartment == null) return; compartment.SetValue(0 /* clientid */, ref value); Marshal.ReleaseComObject(compartment); } } #endregion Internal Properties //----------------------------------------------------- // // Private Methods // //----------------------------------------------------- //------------------------------------------------------ // // Private Fields // //----------------------------------------------------- #region Private Fields ////// Critical: UnsafeNativeMethods.ITfCompartmentMgr has methods with SuppressUnmanagedCodeSecurity. /// private readonly SecurityCriticalData_compartmentmgr; private Guid _guid; private int _cookie; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
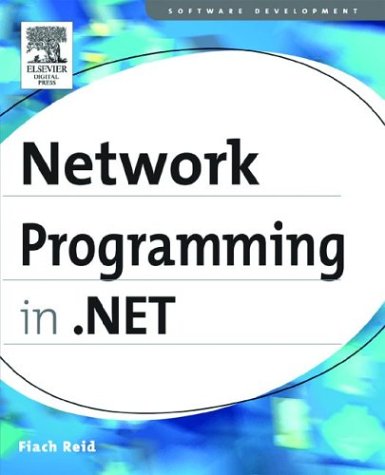
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Authorization.cs
- ApplicationException.cs
- WebSysDisplayNameAttribute.cs
- WmlListAdapter.cs
- TagMapCollection.cs
- TraceContextRecord.cs
- TextParaClient.cs
- ProcessingInstructionAction.cs
- ProfileInfo.cs
- smtpconnection.cs
- BeginGetFileNameFromUserRequest.cs
- SqlExpressionNullability.cs
- Comparer.cs
- MemberDomainMap.cs
- MdiWindowListStrip.cs
- DeploymentSectionCache.cs
- Size3DValueSerializer.cs
- CapiHashAlgorithm.cs
- ServiceDebugBehavior.cs
- WindowsRichEdit.cs
- InstanceLockedException.cs
- InvokeHandlers.cs
- HWStack.cs
- FragmentQueryKB.cs
- XmlSchemaNotation.cs
- XmlReader.cs
- SiteMapDesignerDataSourceView.cs
- DiagnosticStrings.cs
- WindowsMenu.cs
- SelectedDatesCollection.cs
- CreateUserWizard.cs
- ReadOnlyCollectionBase.cs
- BasePattern.cs
- JsonEncodingStreamWrapper.cs
- XDRSchema.cs
- xdrvalidator.cs
- ControlValuePropertyAttribute.cs
- COM2IVsPerPropertyBrowsingHandler.cs
- Oid.cs
- DataGridViewButtonColumn.cs
- PageHandlerFactory.cs
- Wildcard.cs
- HostingPreferredMapPath.cs
- TableLayoutPanel.cs
- ExpressionDumper.cs
- TrustManager.cs
- OutputCacheSection.cs
- ExpressionEditorAttribute.cs
- Cursors.cs
- CodeGen.cs
- TextServicesPropertyRanges.cs
- CellLabel.cs
- RenderDataDrawingContext.cs
- SecurityState.cs
- DataContractSerializerOperationBehavior.cs
- PingOptions.cs
- HtmlInputFile.cs
- ComponentDispatcher.cs
- FormCollection.cs
- HandlerBase.cs
- WindowsStartMenu.cs
- TypeGeneratedEventArgs.cs
- MsdtcClusterUtils.cs
- TextChange.cs
- ContainerAction.cs
- HostingEnvironmentSection.cs
- XmlAnyAttributeAttribute.cs
- RestHandler.cs
- RangeBase.cs
- ProcessProtocolHandler.cs
- SQLUtility.cs
- SuppressMergeCheckAttribute.cs
- HttpDictionary.cs
- _NTAuthentication.cs
- FontInfo.cs
- FileSecurity.cs
- PersonalizationDictionary.cs
- _SslStream.cs
- SamlAction.cs
- EdmProviderManifest.cs
- DataBoundControlAdapter.cs
- StatusBarPanelClickEvent.cs
- BamlBinaryReader.cs
- IdentityManager.cs
- PrivateFontCollection.cs
- LinearGradientBrush.cs
- WorkflowServiceBuildProvider.cs
- HttpConfigurationSystem.cs
- MessageTransmitTraceRecord.cs
- COM2IProvidePropertyBuilderHandler.cs
- ManagedCodeMarkers.cs
- FixedStringLookup.cs
- PointCollection.cs
- Exception.cs
- ExternalDataExchangeService.cs
- SignatureToken.cs
- CompositeDesignerAccessibleObject.cs
- TextBlockAutomationPeer.cs
- BooleanKeyFrameCollection.cs
- DataGridViewCellEventArgs.cs