Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / IdentityModel / System / IdentityModel / Tokens / SamlAction.cs / 1 / SamlAction.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.IdentityModel.Tokens { using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.IdentityModel.Tokens; using System.IdentityModel.Selectors; using System.Xml; using System.Xml.Serialization; using System.Runtime.Serialization; public class SamlAction { string ns; string action; bool isReadOnly = false; public SamlAction(string action) : this(action, null) { } public SamlAction(string action, string ns) { if (String.IsNullOrEmpty(action)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument("action", SR.GetString(SR.SAMLActionNameRequired)); this.action = action; this.ns = ns; } public SamlAction() { } public string Action { get {return this.action; } set { if (isReadOnly) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.ObjectIsReadOnly))); if (String.IsNullOrEmpty(value)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument("value", SR.GetString(SR.SAMLActionNameRequired)); this.action = value; } } public string Namespace { get { return this.ns; } set { if (isReadOnly) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.ObjectIsReadOnly))); this.ns = value; } } public bool IsReadOnly { get { return this.isReadOnly; } } public void MakeReadOnly() { this.isReadOnly = true; } void CheckObjectValidity() { if (String.IsNullOrEmpty(action)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLActionNameRequired))); } public virtual void ReadXml(XmlDictionaryReader reader, SamlSerializer samlSerializer, SecurityTokenSerializer keyInfoSerializer, SecurityTokenResolver outOfBandTokenResolver) { if (reader == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("reader")); if (samlSerializer == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("samlSerializer")); #pragma warning suppress 56506 // samlSerializer.DictionaryManager is never null. SamlDictionary dictionary = samlSerializer.DictionaryManager.SamlDictionary; if (reader.IsStartElement(dictionary.Action, dictionary.Namespace)) { // The Namespace attribute is optional. this.ns = reader.GetAttribute(dictionary.ActionNamespaceAttribute, null); reader.MoveToContent(); this.action = reader.ReadString(); if (string.IsNullOrEmpty(this.action)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLActionNameRequiredOnRead))); reader.MoveToContent(); reader.ReadEndElement(); } } public virtual void WriteXml(XmlDictionaryWriter writer, SamlSerializer samlSerializer, SecurityTokenSerializer keyInfoSerializer) { CheckObjectValidity(); if (writer == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("writer")); if (samlSerializer == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("samlSerializer")); #pragma warning suppress 56506 // samlSerializer.DictionaryManager is never null. SamlDictionary dictionary = samlSerializer.DictionaryManager.SamlDictionary; writer.WriteStartElement(dictionary.PreferredPrefix.Value, dictionary.Action, dictionary.Namespace); if (this.ns != null) { writer.WriteStartAttribute(dictionary.ActionNamespaceAttribute, null); writer.WriteString(this.ns); writer.WriteEndAttribute(); } writer.WriteString(this.action); writer.WriteEndElement(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
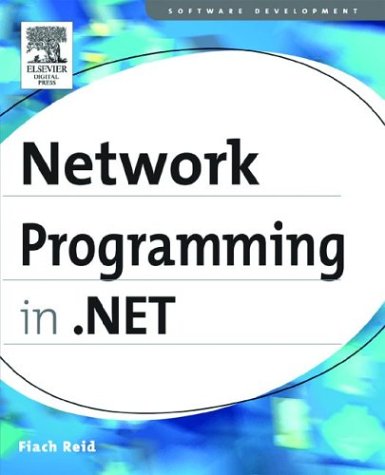
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ConfigurationPropertyCollection.cs
- WbemProvider.cs
- DataViewListener.cs
- JumpPath.cs
- JoinTreeSlot.cs
- UnknownWrapper.cs
- HttpProcessUtility.cs
- FunctionParameter.cs
- DoubleAnimationClockResource.cs
- bidPrivateBase.cs
- WebHttpEndpointElement.cs
- Application.cs
- DataGridItemEventArgs.cs
- BitmapPalettes.cs
- RemotingSurrogateSelector.cs
- PopupEventArgs.cs
- ImportOptions.cs
- SatelliteContractVersionAttribute.cs
- SqlCacheDependencyDatabase.cs
- NetworkInformationPermission.cs
- FontStyle.cs
- ChangeNode.cs
- EntityProviderFactory.cs
- ToolStripMenuItem.cs
- ContextQuery.cs
- Exception.cs
- ProcessHostFactoryHelper.cs
- GridViewUpdateEventArgs.cs
- XmlRawWriter.cs
- SpeechRecognizer.cs
- PropertySourceInfo.cs
- Decimal.cs
- Stream.cs
- ControlBindingsConverter.cs
- EndpointBehaviorElementCollection.cs
- HtmlInputImage.cs
- TextParaClient.cs
- ObjectStateManager.cs
- EtwTrace.cs
- TearOffProxy.cs
- Parallel.cs
- PropertyCondition.cs
- ColorContext.cs
- Camera.cs
- ComponentRenameEvent.cs
- WpfGeneratedKnownTypes.cs
- OrderedDictionary.cs
- GridViewRow.cs
- OperationAbortedException.cs
- StoreUtilities.cs
- DesignerCategoryAttribute.cs
- MobileListItemCollection.cs
- TracingConnectionListener.cs
- StringDictionary.cs
- DesignerView.cs
- AppDomainShutdownMonitor.cs
- CaseStatementSlot.cs
- XmlName.cs
- WebPartActionVerb.cs
- CalendarDay.cs
- XmlSchemaParticle.cs
- JpegBitmapEncoder.cs
- DiscreteKeyFrames.cs
- MailWebEventProvider.cs
- XmlSchemaChoice.cs
- SubqueryRules.cs
- ProcessHostConfigUtils.cs
- QueryExecutionOption.cs
- WebPartCatalogAddVerb.cs
- Funcletizer.cs
- CheckableControlBaseAdapter.cs
- SafeNativeMethodsCLR.cs
- HTTP_SERVICE_CONFIG_URLACL_PARAM.cs
- Semaphore.cs
- NumberFormatter.cs
- OleDbPropertySetGuid.cs
- CompletedAsyncResult.cs
- PeerResolverMode.cs
- CoordinationService.cs
- PointHitTestResult.cs
- FileRecordSequenceCompletedAsyncResult.cs
- CommandField.cs
- RedBlackList.cs
- ToolConsole.cs
- Utility.cs
- SelectionListComponentEditor.cs
- GroupItemAutomationPeer.cs
- VisualTarget.cs
- SourceFileBuildProvider.cs
- ReadingWritingEntityEventArgs.cs
- InvalidOperationException.cs
- x509utils.cs
- StylusPointCollection.cs
- FormViewPagerRow.cs
- EnumConverter.cs
- BasePropertyDescriptor.cs
- InfoCardRSACryptoProvider.cs
- ObjectItemCollection.cs
- SynchronizedInputProviderWrapper.cs
- ToolStripSettings.cs