Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / VisualTarget.cs / 1305600 / VisualTarget.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // // History: // 01/06/2005 : ABaioura - Created. // //----------------------------------------------------------------------------- using System.Windows.Media; using System.Windows.Media.Composition; using System.Security; using System.Diagnostics; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// /// public class VisualTarget : CompositionTarget { //--------------------------------------------------------------------- // // Constructors // //--------------------------------------------------------------------- #region Constructors ////// VisualTarget /// public VisualTarget(HostVisual hostVisual) { if (hostVisual == null) { throw new ArgumentNullException("hostVisual"); } _hostVisual = hostVisual; _connected = false; MediaContext.RegisterICompositionTarget(Dispatcher, this); } ////// This function gets called when VisualTarget is created on the channel /// i.e. from CreateUCEResources. /// private void BeginHosting() { Debug.Assert(!_connected); try { // // Initiate hosting by the specified host visual. // _hostVisual.BeginHosting(this); _connected = true; } catch { // // If exception has occurred after we have registered with // MediaContext, we need to unregister to properly release // allocated resources. // NOTE: We need to properly unregister in a disconnected state // MediaContext.UnregisterICompositionTarget(Dispatcher, this); throw; } } internal override void CreateUCEResources(DUCE.Channel channel, DUCE.Channel outOfBandChannel) { Debug.Assert(channel != null); Debug.Assert(outOfBandChannel != null); _outOfBandChannel = outOfBandChannel; // create visual target resources base.CreateUCEResources(channel, outOfBandChannel); // Update state to propagate flags as necessary StateChangedCallback( new object[] { HostStateFlags.None }); // // Addref content node on the channel. We need extra reference // on that node so that it does not get immediately released // when Dispose is called. Actual release of the node needs // to be synchronized with node disconnect by the host. // bool resourceCreated = _contentRoot.CreateOrAddRefOnChannel(this, outOfBandChannel, s_contentRootType); Debug.Assert(!resourceCreated); _contentRoot.CreateOrAddRefOnChannel(this, channel, s_contentRootType); BeginHosting(); } #endregion Constructors //---------------------------------------------------------------------- // // Public Properties // //--------------------------------------------------------------------- #region Public Properties ////// Returns matrix that can be used to transform coordinates from this /// target to the rendering destination device. /// public override Matrix TransformToDevice { get { VerifyAPIReadOnly(); Matrix m = WorldTransform; m.Invert(); return m; } } ////// Returns matrix that can be used to transform coordinates from /// the rendering destination device to this target. /// public override Matrix TransformFromDevice { get { VerifyAPIReadOnly(); return WorldTransform; } } #endregion Public Properties //---------------------------------------------------------------------- // // Public Methods // //---------------------------------------------------------------------- #region Private Fields ////// Dispose cleans up the state associated with HwndTarget. /// ////// Critical: Sets RootVisual to null /// PublicOK: This code has the same affect as removing elements in a window /// [SecurityCritical] public override void Dispose() { try { VerifyAccess(); if (!IsDisposed) { if (_hostVisual != null && _connected) { RootVisual = null; // // Unregister this CompositionTarget from the MediaSystem. // // we need to properly unregister in a disconnected state MediaContext.UnregisterICompositionTarget(Dispatcher, this); } } } finally { base.Dispose(); } } ////// This function gets called when VisualTarget is removed on the channel /// i.e. from ReleaseUCEResources. /// private void EndHosting() { Debug.Assert(_connected); _hostVisual.EndHosting(); _connected = false; } ////// This method is used to release all uce resources either on Shutdown or session disconnect /// internal override void ReleaseUCEResources(DUCE.Channel channel, DUCE.Channel outOfBandChannel) { EndHosting(); _contentRoot.ReleaseOnChannel(channel); if (_contentRoot.IsOnChannel(outOfBandChannel)) { _contentRoot.ReleaseOnChannel(outOfBandChannel); } base.ReleaseUCEResources(channel, outOfBandChannel); } #endregion Public Methods #region Internal Properties ////// The out of band channel on our the MediaContext this /// resource was created. /// This is needed by HostVisual for handle duplication. /// internal DUCE.Channel OutOfBandChannel { get { return _outOfBandChannel; } } #endregion Internal Properties //--------------------------------------------------------------------- // // Private Fields // //---------------------------------------------------------------------- #region Private Fields DUCE.Channel _outOfBandChannel; private HostVisual _hostVisual; // Flag indicating whether VisualTarget-HostVisual connection exists. private bool _connected; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
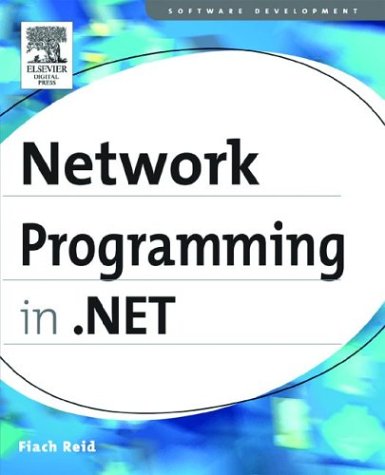
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RSAOAEPKeyExchangeFormatter.cs
- SmiTypedGetterSetter.cs
- SqlMethods.cs
- ClientSettingsStore.cs
- Source.cs
- ArrayConverter.cs
- EditorBrowsableAttribute.cs
- MetaType.cs
- ListViewUpdateEventArgs.cs
- ProjectionCamera.cs
- AttachedAnnotation.cs
- ControlUtil.cs
- GeneralTransformGroup.cs
- PageParserFilter.cs
- FixedSOMGroup.cs
- ObjectKeyFrameCollection.cs
- CodeCompiler.cs
- XmlChildNodes.cs
- MetafileHeader.cs
- SqlNode.cs
- Point3DKeyFrameCollection.cs
- MarkupExtensionParser.cs
- DbCommandTree.cs
- HttpModuleCollection.cs
- SocketConnection.cs
- SqlBooleanizer.cs
- OverflowException.cs
- WebPartDisplayModeEventArgs.cs
- SchemaNames.cs
- MimeBasePart.cs
- DataSourceConverter.cs
- Transform.cs
- ArrayExtension.cs
- LambdaCompiler.Logical.cs
- PartitionedStream.cs
- Clipboard.cs
- SafeArrayTypeMismatchException.cs
- LogicalExpressionTypeConverter.cs
- OrderByExpression.cs
- WebPartCatalogAddVerb.cs
- ValidationPropertyAttribute.cs
- AttributeCollection.cs
- DateTimeConstantAttribute.cs
- TypeConverterMarkupExtension.cs
- EncodingDataItem.cs
- FileIOPermission.cs
- DataGridViewLinkColumn.cs
- DataBinding.cs
- BindingBase.cs
- MemberHolder.cs
- ResizingMessageFilter.cs
- SchemaTypeEmitter.cs
- AuthenticationConfig.cs
- SeekStoryboard.cs
- WebBrowser.cs
- NavigationCommands.cs
- ParameterRetriever.cs
- XmlBinaryReader.cs
- ChangeConflicts.cs
- RichTextBoxConstants.cs
- CompressedStack.cs
- SwitchElementsCollection.cs
- baseaxisquery.cs
- ProgressBarHighlightConverter.cs
- ThreadStaticAttribute.cs
- ControlHelper.cs
- EntryWrittenEventArgs.cs
- ZipIOExtraFieldPaddingElement.cs
- MatrixCamera.cs
- DES.cs
- UnicastIPAddressInformationCollection.cs
- Char.cs
- ColumnMapTranslator.cs
- WorkItem.cs
- SQLByte.cs
- XmlElementCollection.cs
- XhtmlConformanceSection.cs
- MDIControlStrip.cs
- Size.cs
- EditorAttribute.cs
- ConstraintStruct.cs
- ExceptionRoutedEventArgs.cs
- PagePropertiesChangingEventArgs.cs
- SchemaConstraints.cs
- xsdvalidator.cs
- ChainOfResponsibility.cs
- TransferRequestHandler.cs
- WebPartDisplayMode.cs
- DesignerVerb.cs
- XsdBuildProvider.cs
- UIElementHelper.cs
- Literal.cs
- GroupBox.cs
- XamlSerializer.cs
- SqlCacheDependency.cs
- AssociationTypeEmitter.cs
- SerializationIncompleteException.cs
- FileRecordSequenceCompletedAsyncResult.cs
- SoapCodeExporter.cs
- ZipIORawDataFileBlock.cs