Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Metadata / Edm / ComplexType.cs / 1 / ComplexType.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System.Data.Common; using System.Threading; using System.Diagnostics; namespace System.Data.Metadata.Edm { ////// Represent the Edm Complex Type /// public class ComplexType : StructuralType { #region Constructors ////// Initializes a new instance of Complex Type with the given properties /// /// The name of the complex type /// The namespace name of the type /// The version of this type /// dataSpace in which this ComplexType belongs to ///If either name, namespace or version arguments are null internal ComplexType(string name, string namespaceName, DataSpace dataSpace) : base(name, namespaceName, dataSpace) { } ////// Initializes a new instance of Complex Type - required for bootstraping code /// internal ComplexType() { // No initialization of item attributes in here, it's used as a pass thru in the case for delay population // of item attributes } #endregion #region Fields private ReadOnlyMetadataCollection_properties; #endregion #region Properties /// /// Returns the kind of the type /// public override BuiltInTypeKind BuiltInTypeKind { get { return BuiltInTypeKind.ComplexType; } } ////// Returns just the properties from the collection /// of members on this type /// public ReadOnlyMetadataCollectionProperties { get { Debug.Assert(IsReadOnly, "this is a wrapper around this.Members, don't call it during metadata loading, only call it after the metadata is set to readonly"); if (null == _properties) { Interlocked.CompareExchange(ref _properties, new FilteredReadOnlyMetadataCollection ( this.Members, Helper.IsEdmProperty), null); } return _properties; } } #endregion #region Methods /// /// Validates a EdmMember object to determine if it can be added to this type's /// Members collection. If this method returns without throwing, it is assumed /// the member is valid. /// /// The member to validate ///Thrown if the member is not a EdmProperty internal override void ValidateMemberForAdd(EdmMember member) { if (!Helper.IsEdmProperty(member) && !Helper.IsNavigationProperty(member)) { throw EntityUtil.ComplexTypeInvalidMembers(); } } #endregion } internal sealed class ClrComplexType : ComplexType { ///cached CLR type handle, allowing the Type reference to be GC'd private readonly System.RuntimeTypeHandle _type; ///cached dynamic method to construct a CLR instance private Delegate _constructor; private readonly string _cspaceTypeName; ////// Initializes a new instance of Complex Type with properties from the type. /// /// The CLR type to construct from internal ClrComplexType(Type clrType, string cspaceNamespaceName, string cspaceTypeName) : base(EntityUtil.GenericCheckArgumentNull(clrType, "clrType").Name, clrType.Namespace ?? string.Empty, DataSpace.OSpace) { System.Diagnostics.Debug.Assert(!String.IsNullOrEmpty(cspaceNamespaceName) && !String.IsNullOrEmpty(cspaceTypeName), "Mapping information must never be null"); _type = clrType.TypeHandle; _cspaceTypeName = cspaceNamespaceName + "." + cspaceTypeName; this.Abstract = clrType.IsAbstract; } internal static ClrComplexType CreateReadonlyClrComplexType(Type clrType, string cspaceNamespaceName, string cspaceTypeName) { ClrComplexType type = new ClrComplexType(clrType, cspaceNamespaceName, cspaceTypeName); type.SetReadOnly(); return type; } ///cached dynamic method to construct a CLR instance internal Delegate Constructor { get { return _constructor; } set { // It doesn't matter which delegate wins, but only one should be jitted Interlocked.CompareExchange(ref _constructor, value, null); } } ////// internal override System.Type ClrType { get { return Type.GetTypeFromHandle(_type); } } internal string CSpaceTypeName { get { return _cspaceTypeName; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System.Data.Common; using System.Threading; using System.Diagnostics; namespace System.Data.Metadata.Edm { ////// Represent the Edm Complex Type /// public class ComplexType : StructuralType { #region Constructors ////// Initializes a new instance of Complex Type with the given properties /// /// The name of the complex type /// The namespace name of the type /// The version of this type /// dataSpace in which this ComplexType belongs to ///If either name, namespace or version arguments are null internal ComplexType(string name, string namespaceName, DataSpace dataSpace) : base(name, namespaceName, dataSpace) { } ////// Initializes a new instance of Complex Type - required for bootstraping code /// internal ComplexType() { // No initialization of item attributes in here, it's used as a pass thru in the case for delay population // of item attributes } #endregion #region Fields private ReadOnlyMetadataCollection_properties; #endregion #region Properties /// /// Returns the kind of the type /// public override BuiltInTypeKind BuiltInTypeKind { get { return BuiltInTypeKind.ComplexType; } } ////// Returns just the properties from the collection /// of members on this type /// public ReadOnlyMetadataCollectionProperties { get { Debug.Assert(IsReadOnly, "this is a wrapper around this.Members, don't call it during metadata loading, only call it after the metadata is set to readonly"); if (null == _properties) { Interlocked.CompareExchange(ref _properties, new FilteredReadOnlyMetadataCollection ( this.Members, Helper.IsEdmProperty), null); } return _properties; } } #endregion #region Methods /// /// Validates a EdmMember object to determine if it can be added to this type's /// Members collection. If this method returns without throwing, it is assumed /// the member is valid. /// /// The member to validate ///Thrown if the member is not a EdmProperty internal override void ValidateMemberForAdd(EdmMember member) { if (!Helper.IsEdmProperty(member) && !Helper.IsNavigationProperty(member)) { throw EntityUtil.ComplexTypeInvalidMembers(); } } #endregion } internal sealed class ClrComplexType : ComplexType { ///cached CLR type handle, allowing the Type reference to be GC'd private readonly System.RuntimeTypeHandle _type; ///cached dynamic method to construct a CLR instance private Delegate _constructor; private readonly string _cspaceTypeName; ////// Initializes a new instance of Complex Type with properties from the type. /// /// The CLR type to construct from internal ClrComplexType(Type clrType, string cspaceNamespaceName, string cspaceTypeName) : base(EntityUtil.GenericCheckArgumentNull(clrType, "clrType").Name, clrType.Namespace ?? string.Empty, DataSpace.OSpace) { System.Diagnostics.Debug.Assert(!String.IsNullOrEmpty(cspaceNamespaceName) && !String.IsNullOrEmpty(cspaceTypeName), "Mapping information must never be null"); _type = clrType.TypeHandle; _cspaceTypeName = cspaceNamespaceName + "." + cspaceTypeName; this.Abstract = clrType.IsAbstract; } internal static ClrComplexType CreateReadonlyClrComplexType(Type clrType, string cspaceNamespaceName, string cspaceTypeName) { ClrComplexType type = new ClrComplexType(clrType, cspaceNamespaceName, cspaceTypeName); type.SetReadOnly(); return type; } ///cached dynamic method to construct a CLR instance internal Delegate Constructor { get { return _constructor; } set { // It doesn't matter which delegate wins, but only one should be jitted Interlocked.CompareExchange(ref _constructor, value, null); } } ////// internal override System.Type ClrType { get { return Type.GetTypeFromHandle(_type); } } internal string CSpaceTypeName { get { return _cspaceTypeName; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
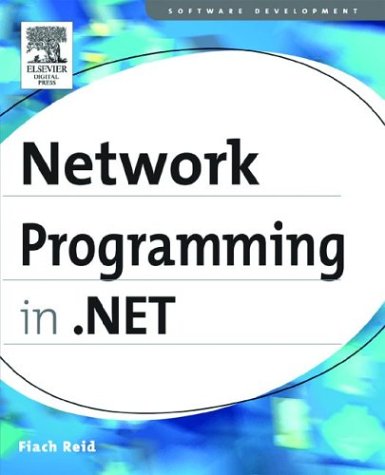
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataTableReader.cs
- OleDbDataAdapter.cs
- ViewValidator.cs
- XmlnsDictionary.cs
- PathFigureCollectionValueSerializer.cs
- AppDomainManager.cs
- QilLiteral.cs
- DataGrid.cs
- StylusSystemGestureEventArgs.cs
- GetRecipientListRequest.cs
- MethodRental.cs
- MutableAssemblyCacheEntry.cs
- HiddenFieldPageStatePersister.cs
- RouteItem.cs
- ColumnHeader.cs
- SqlCacheDependency.cs
- DrawingAttributesDefaultValueFactory.cs
- BamlTreeNode.cs
- DockPattern.cs
- ProfileSection.cs
- EncodingDataItem.cs
- OutputScope.cs
- Attribute.cs
- ConsumerConnectionPoint.cs
- __Filters.cs
- FigureParagraph.cs
- SecureStringHasher.cs
- SessionStateContainer.cs
- CacheDependency.cs
- MediaPlayer.cs
- HtmlTableRowCollection.cs
- SupportsEventValidationAttribute.cs
- BufferModesCollection.cs
- System.Data.OracleClient_BID.cs
- RequestQueue.cs
- EventLogReader.cs
- AssemblyAssociatedContentFileAttribute.cs
- ObjectAnimationUsingKeyFrames.cs
- ChangePassword.cs
- PartitionResolver.cs
- StoragePropertyMapping.cs
- DataGridColumn.cs
- metrodevice.cs
- XmlCountingReader.cs
- XmlRawWriter.cs
- WebPartActionVerb.cs
- DataSourceSerializationException.cs
- XPathCompileException.cs
- Attributes.cs
- CacheEntry.cs
- ToolStripSeparatorRenderEventArgs.cs
- VirtualPathUtility.cs
- PreviousTrackingServiceAttribute.cs
- HostingEnvironment.cs
- Stack.cs
- SqlClientWrapperSmiStreamChars.cs
- EventWaitHandle.cs
- TextWriter.cs
- JournalNavigationScope.cs
- ClientSideProviderDescription.cs
- StateChangeEvent.cs
- util.cs
- ButtonColumn.cs
- TextBreakpoint.cs
- ContainerTracking.cs
- PolyLineSegment.cs
- ContainerParaClient.cs
- XmlSchemaChoice.cs
- PrimarySelectionAdorner.cs
- Regex.cs
- CodeExpressionStatement.cs
- TdsParserSafeHandles.cs
- QuaternionValueSerializer.cs
- HebrewNumber.cs
- BamlLocalizableResource.cs
- WindowsMenu.cs
- XmlHierarchyData.cs
- DeflateStream.cs
- XmlAttribute.cs
- SynchronizedMessageSource.cs
- SecureStringHasher.cs
- WizardStepBase.cs
- ToolBar.cs
- mansign.cs
- NativeDirectoryServicesQueryAPIs.cs
- UnitySerializationHolder.cs
- FlowDocumentScrollViewer.cs
- Int32Rect.cs
- OdbcStatementHandle.cs
- AffineTransform3D.cs
- ProgressBarBrushConverter.cs
- SafeNativeMethods.cs
- WindowsContainer.cs
- MetadataFile.cs
- EncryptedPackageFilter.cs
- Quaternion.cs
- CodeDirectoryCompiler.cs
- DocumentOrderComparer.cs
- CodeTypeConstructor.cs
- KeyGesture.cs