Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / xsp / System / Web / Compilation / CompilerTypeWithParams.cs / 1 / CompilerTypeWithParams.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Compilation { using System; using System.Security.Permissions; using System.IO; using System.Collections; using System.Globalization; using System.CodeDom; using System.CodeDom.Compiler; using System.Web.Hosting; using System.Web.Util; using System.Web.UI; using System.Web.Configuration; /* * This class describes a CodeDom compiler, along with the parameters that it uses. * The reason we need this class is that if two files both use the same language, * but ask for different command line options (e.g. debug vs retail), we will not * be able to compile them together. So effectively, we need to treat them as * different languages. */ [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class CompilerType { private Type _codeDomProviderType; public Type CodeDomProviderType { get { return _codeDomProviderType; } } private CompilerParameters _compilParams; public CompilerParameters CompilerParameters { get { return _compilParams; } } internal CompilerType(Type codeDomProviderType, CompilerParameters compilParams) { Debug.Assert(codeDomProviderType != null); _codeDomProviderType = codeDomProviderType; if (compilParams == null) _compilParams = new CompilerParameters(); else _compilParams = compilParams; } internal CompilerType Clone() { // Clone the CompilerParameters to make sure the original is untouched return new CompilerType(_codeDomProviderType, CloneCompilerParameters()); } private CompilerParameters CloneCompilerParameters() { CompilerParameters copy = new CompilerParameters(); copy.IncludeDebugInformation = _compilParams.IncludeDebugInformation; copy.TreatWarningsAsErrors = _compilParams.TreatWarningsAsErrors; copy.WarningLevel = _compilParams.WarningLevel; copy.CompilerOptions = _compilParams.CompilerOptions; return copy; } public override int GetHashCode() { return _codeDomProviderType.GetHashCode(); } public override bool Equals(Object o) { CompilerType other = o as CompilerType; if (o == null) return false; return _codeDomProviderType == other._codeDomProviderType && _compilParams.WarningLevel == other._compilParams.WarningLevel && _compilParams.IncludeDebugInformation == other._compilParams.IncludeDebugInformation && _compilParams.CompilerOptions == other._compilParams.CompilerOptions; } internal AssemblyBuilder CreateAssemblyBuilder(CompilationSection compConfig, ICollection referencedAssemblies) { return CreateAssemblyBuilder(compConfig, referencedAssemblies, null /*generatedFilesDir*/, null /*outputAssemblyName*/); } internal AssemblyBuilder CreateAssemblyBuilder(CompilationSection compConfig, ICollection referencedAssemblies, string generatedFilesDir, string outputAssemblyName) { // Create a special AssemblyBuilder when we're only supposed to generate // source files but not compile them (for ClientBuildManager.GetCodeDirectoryInformation) if (generatedFilesDir != null) { return new CbmCodeGeneratorBuildProviderHost(compConfig, referencedAssemblies, this, generatedFilesDir, outputAssemblyName); } return new AssemblyBuilder(compConfig, referencedAssemblies, this, outputAssemblyName); } private static CompilerType GetDefaultCompilerTypeWithParams( CompilationSection compConfig, VirtualPath configPath) { // By default, use C# when no provider is asking for a specific language return CompilationUtil.GetCSharpCompilerInfo(compConfig, configPath); } internal static AssemblyBuilder GetDefaultAssemblyBuilder(CompilationSection compConfig, ICollection referencedAssemblies, VirtualPath configPath, string outputAssemblyName) { return GetDefaultAssemblyBuilder(compConfig, referencedAssemblies, configPath, null /*generatedFilesDir*/, outputAssemblyName); } internal static AssemblyBuilder GetDefaultAssemblyBuilder(CompilationSection compConfig, ICollection referencedAssemblies, VirtualPath configPath, string generatedFilesDir, string outputAssemblyName) { CompilerType ctwp = GetDefaultCompilerTypeWithParams(compConfig, configPath); return ctwp.CreateAssemblyBuilder(compConfig, referencedAssemblies, generatedFilesDir, outputAssemblyName); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Compilation { using System; using System.Security.Permissions; using System.IO; using System.Collections; using System.Globalization; using System.CodeDom; using System.CodeDom.Compiler; using System.Web.Hosting; using System.Web.Util; using System.Web.UI; using System.Web.Configuration; /* * This class describes a CodeDom compiler, along with the parameters that it uses. * The reason we need this class is that if two files both use the same language, * but ask for different command line options (e.g. debug vs retail), we will not * be able to compile them together. So effectively, we need to treat them as * different languages. */ [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class CompilerType { private Type _codeDomProviderType; public Type CodeDomProviderType { get { return _codeDomProviderType; } } private CompilerParameters _compilParams; public CompilerParameters CompilerParameters { get { return _compilParams; } } internal CompilerType(Type codeDomProviderType, CompilerParameters compilParams) { Debug.Assert(codeDomProviderType != null); _codeDomProviderType = codeDomProviderType; if (compilParams == null) _compilParams = new CompilerParameters(); else _compilParams = compilParams; } internal CompilerType Clone() { // Clone the CompilerParameters to make sure the original is untouched return new CompilerType(_codeDomProviderType, CloneCompilerParameters()); } private CompilerParameters CloneCompilerParameters() { CompilerParameters copy = new CompilerParameters(); copy.IncludeDebugInformation = _compilParams.IncludeDebugInformation; copy.TreatWarningsAsErrors = _compilParams.TreatWarningsAsErrors; copy.WarningLevel = _compilParams.WarningLevel; copy.CompilerOptions = _compilParams.CompilerOptions; return copy; } public override int GetHashCode() { return _codeDomProviderType.GetHashCode(); } public override bool Equals(Object o) { CompilerType other = o as CompilerType; if (o == null) return false; return _codeDomProviderType == other._codeDomProviderType && _compilParams.WarningLevel == other._compilParams.WarningLevel && _compilParams.IncludeDebugInformation == other._compilParams.IncludeDebugInformation && _compilParams.CompilerOptions == other._compilParams.CompilerOptions; } internal AssemblyBuilder CreateAssemblyBuilder(CompilationSection compConfig, ICollection referencedAssemblies) { return CreateAssemblyBuilder(compConfig, referencedAssemblies, null /*generatedFilesDir*/, null /*outputAssemblyName*/); } internal AssemblyBuilder CreateAssemblyBuilder(CompilationSection compConfig, ICollection referencedAssemblies, string generatedFilesDir, string outputAssemblyName) { // Create a special AssemblyBuilder when we're only supposed to generate // source files but not compile them (for ClientBuildManager.GetCodeDirectoryInformation) if (generatedFilesDir != null) { return new CbmCodeGeneratorBuildProviderHost(compConfig, referencedAssemblies, this, generatedFilesDir, outputAssemblyName); } return new AssemblyBuilder(compConfig, referencedAssemblies, this, outputAssemblyName); } private static CompilerType GetDefaultCompilerTypeWithParams( CompilationSection compConfig, VirtualPath configPath) { // By default, use C# when no provider is asking for a specific language return CompilationUtil.GetCSharpCompilerInfo(compConfig, configPath); } internal static AssemblyBuilder GetDefaultAssemblyBuilder(CompilationSection compConfig, ICollection referencedAssemblies, VirtualPath configPath, string outputAssemblyName) { return GetDefaultAssemblyBuilder(compConfig, referencedAssemblies, configPath, null /*generatedFilesDir*/, outputAssemblyName); } internal static AssemblyBuilder GetDefaultAssemblyBuilder(CompilationSection compConfig, ICollection referencedAssemblies, VirtualPath configPath, string generatedFilesDir, string outputAssemblyName) { CompilerType ctwp = GetDefaultCompilerTypeWithParams(compConfig, configPath); return ctwp.CreateAssemblyBuilder(compConfig, referencedAssemblies, generatedFilesDir, outputAssemblyName); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
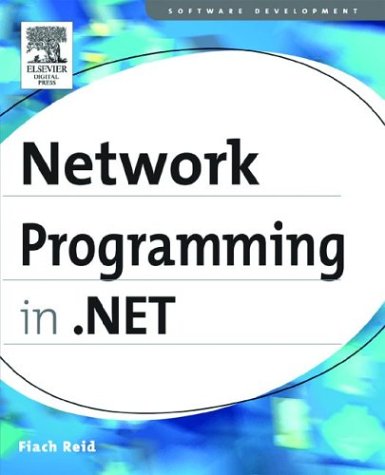
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AuthenticatedStream.cs
- DivideByZeroException.cs
- TextTreeUndo.cs
- SmtpSection.cs
- Encoding.cs
- RC2CryptoServiceProvider.cs
- DispatcherHookEventArgs.cs
- PrivilegedConfigurationManager.cs
- SamlAction.cs
- DirectoryLocalQuery.cs
- WmpBitmapEncoder.cs
- SmtpLoginAuthenticationModule.cs
- _SecureChannel.cs
- BamlWriter.cs
- SqlInfoMessageEvent.cs
- InheritedPropertyDescriptor.cs
- BuilderPropertyEntry.cs
- LazyTextWriterCreator.cs
- LogLogRecordEnumerator.cs
- SystemFonts.cs
- PointAnimationBase.cs
- AesCryptoServiceProvider.cs
- ToolStripControlHost.cs
- StringFormat.cs
- KeyValueConfigurationElement.cs
- SpotLight.cs
- CursorInteropHelper.cs
- TreeNodeCollection.cs
- UserControl.cs
- FieldAccessException.cs
- DataRelation.cs
- WebPartConnectionsCancelVerb.cs
- SqlBulkCopyColumnMapping.cs
- ImmutablePropertyDescriptorGridEntry.cs
- ActivityLocationReferenceEnvironment.cs
- GeneralTransformCollection.cs
- BmpBitmapEncoder.cs
- parserscommon.cs
- base64Transforms.cs
- RegexMatchCollection.cs
- TypeForwardedFromAttribute.cs
- SQLDecimal.cs
- CacheVirtualItemsEvent.cs
- WebHttpElement.cs
- DSASignatureFormatter.cs
- RadialGradientBrush.cs
- PartitionedStream.cs
- MenuItemBindingCollection.cs
- SqlCacheDependency.cs
- GroupBoxAutomationPeer.cs
- Line.cs
- UpdateCommand.cs
- SequentialUshortCollection.cs
- AccessedThroughPropertyAttribute.cs
- WebHttpSecurityModeHelper.cs
- EdmProviderManifest.cs
- LayoutEngine.cs
- PeerApplication.cs
- ObjectStateEntry.cs
- WSAddressing10ProblemHeaderQNameFault.cs
- HostingEnvironmentSection.cs
- SqlDataReaderSmi.cs
- ReceiveDesigner.xaml.cs
- OpenTypeLayout.cs
- Parameter.cs
- XmlCollation.cs
- GridViewDeletedEventArgs.cs
- ScriptingRoleServiceSection.cs
- ContourSegment.cs
- WorkflowPersistenceService.cs
- XmlChildEnumerator.cs
- HttpApplication.cs
- PageRequestManager.cs
- FormsAuthentication.cs
- FixedNode.cs
- RefType.cs
- OracleParameterCollection.cs
- SelectedGridItemChangedEvent.cs
- ItemsPresenter.cs
- XmlObjectSerializer.cs
- MethodCallTranslator.cs
- ObjectConverter.cs
- SafeBitVector32.cs
- Line.cs
- RectAnimation.cs
- PerfService.cs
- Debug.cs
- ServiceX509SecurityTokenProvider.cs
- sortedlist.cs
- BitmapData.cs
- DataSourceComponent.cs
- MethodExpr.cs
- Cursors.cs
- XmlElementAttributes.cs
- DateTimeFormatInfo.cs
- HtmlElementErrorEventArgs.cs
- CaseInsensitiveComparer.cs
- DynamicRenderer.cs
- UnsafeNativeMethods.cs
- SimpleBitVector32.cs