Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / Xml / System / Xml / schema / XmlSchemaComplexType.cs / 1 / XmlSchemaComplexType.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Schema { using System.Collections; using System.ComponentModel; using System.Xml.Serialization; ////// /// public class XmlSchemaComplexType : XmlSchemaType { XmlSchemaDerivationMethod block = XmlSchemaDerivationMethod.None; XmlSchemaContentModel contentModel; XmlSchemaParticle particle; XmlSchemaObjectCollection attributes; XmlSchemaAnyAttribute anyAttribute; XmlSchemaParticle contentTypeParticle = XmlSchemaParticle.Empty; XmlSchemaDerivationMethod blockResolved; XmlSchemaObjectTable localElements; XmlSchemaObjectTable attributeUses; XmlSchemaAnyAttribute attributeWildcard; static XmlSchemaComplexType anyTypeLax; static XmlSchemaComplexType anyTypeSkip; static XmlSchemaComplexType untypedAnyType; //additional info for Partial validation byte pvFlags; const byte wildCardMask = 0x01; const byte dupDeclMask = 0x02; const byte isMixedMask = 0x04; const byte isAbstractMask = 0x08; static XmlSchemaComplexType() { anyTypeLax = CreateAnyType(XmlSchemaContentProcessing.Lax); anyTypeSkip = CreateAnyType(XmlSchemaContentProcessing.Skip); // Create xdt:untypedAny untypedAnyType = new XmlSchemaComplexType(); untypedAnyType.SetQualifiedName(new XmlQualifiedName("untypedAny", XmlReservedNs.NsXQueryDataType)); untypedAnyType.IsMixed = true; untypedAnyType.SetContentTypeParticle(anyTypeLax.ContentTypeParticle); untypedAnyType.SetContentType(XmlSchemaContentType.Mixed); untypedAnyType.ElementDecl = SchemaElementDecl.CreateAnyTypeElementDecl(); untypedAnyType.ElementDecl.SchemaType = untypedAnyType; untypedAnyType.ElementDecl.ContentValidator = AnyTypeContentValidator; } static XmlSchemaComplexType CreateAnyType(XmlSchemaContentProcessing processContents) { XmlSchemaComplexType localAnyType = new XmlSchemaComplexType(); localAnyType.SetQualifiedName(DatatypeImplementation.QnAnyType); XmlSchemaAny anyElement = new XmlSchemaAny(); anyElement.MinOccurs = decimal.Zero; anyElement.MaxOccurs = decimal.MaxValue; anyElement.ProcessContents = processContents; anyElement.BuildNamespaceList(null); XmlSchemaSequence seq = new XmlSchemaSequence(); seq.Items.Add(anyElement); localAnyType.SetContentTypeParticle(seq); localAnyType.SetContentType(XmlSchemaContentType.Mixed); localAnyType.ElementDecl = SchemaElementDecl.CreateAnyTypeElementDecl(); localAnyType.ElementDecl.SchemaType = localAnyType; //Create contentValidator for Any ParticleContentValidator contentValidator = new ParticleContentValidator(XmlSchemaContentType.Mixed); contentValidator.Start(); contentValidator.OpenGroup(); contentValidator.AddNamespaceList(anyElement.NamespaceList, anyElement); contentValidator.AddStar(); contentValidator.CloseGroup(); ContentValidator anyContentValidator = contentValidator.Finish(true); localAnyType.ElementDecl.ContentValidator = anyContentValidator; XmlSchemaAnyAttribute anyAttribute = new XmlSchemaAnyAttribute(); anyAttribute.ProcessContents = processContents; anyAttribute.BuildNamespaceList(null); localAnyType.SetAttributeWildcard(anyAttribute); localAnyType.ElementDecl.AnyAttribute = anyAttribute; return localAnyType; } ///[To be supplied.] ////// /// public XmlSchemaComplexType() { } [XmlIgnore] internal static XmlSchemaComplexType AnyType { get { return anyTypeLax; } } [XmlIgnore] internal static XmlSchemaComplexType UntypedAnyType { get { return untypedAnyType; } } [XmlIgnore] internal static XmlSchemaComplexType AnyTypeSkip { get { return anyTypeSkip; } } internal static ContentValidator AnyTypeContentValidator { get { return anyTypeLax.ElementDecl.ContentValidator; } } ///[To be supplied.] ////// /// [XmlAttribute("abstract"), DefaultValue(false)] public bool IsAbstract { get { return (pvFlags & isAbstractMask) != 0; } set { if (value) { pvFlags = (byte)(pvFlags | isAbstractMask); } else { pvFlags = (byte)(pvFlags & ~isAbstractMask); } } } ///[To be supplied.] ////// /// [XmlAttribute("block"), DefaultValue(XmlSchemaDerivationMethod.None)] public XmlSchemaDerivationMethod Block { get { return block; } set { block = value; } } ///[To be supplied.] ////// /// [XmlAttribute("mixed"), DefaultValue(false)] public override bool IsMixed { get { return (pvFlags & isMixedMask) != 0; } set { if (value) { pvFlags = (byte)(pvFlags | isMixedMask); } else { pvFlags = (byte)(pvFlags & ~isMixedMask); } } } ///[To be supplied.] ////// /// [XmlElement("simpleContent", typeof(XmlSchemaSimpleContent)), XmlElement("complexContent", typeof(XmlSchemaComplexContent))] public XmlSchemaContentModel ContentModel { get { return contentModel; } set { contentModel = value; } } ///[To be supplied.] ////// /// [XmlElement("group", typeof(XmlSchemaGroupRef)), XmlElement("choice", typeof(XmlSchemaChoice)), XmlElement("all", typeof(XmlSchemaAll)), XmlElement("sequence", typeof(XmlSchemaSequence))] public XmlSchemaParticle Particle { get { return particle; } set { particle = value; } } ///[To be supplied.] ////// /// [XmlElement("attribute", typeof(XmlSchemaAttribute)), XmlElement("attributeGroup", typeof(XmlSchemaAttributeGroupRef))] public XmlSchemaObjectCollection Attributes { get { if (attributes == null) { attributes = new XmlSchemaObjectCollection(); } return attributes; } } ///[To be supplied.] ////// /// [XmlElement("anyAttribute")] public XmlSchemaAnyAttribute AnyAttribute { get { return anyAttribute; } set { anyAttribute = value; } } ///[To be supplied.] ////// /// [XmlIgnore] public XmlSchemaContentType ContentType { get { return SchemaContentType; } } ///[To be supplied.] ////// /// [XmlIgnore] public XmlSchemaParticle ContentTypeParticle { get { return contentTypeParticle; } } ///[To be supplied.] ////// /// [XmlIgnore] public XmlSchemaDerivationMethod BlockResolved { get { return blockResolved; } } ///[To be supplied.] ////// /// [XmlIgnore] public XmlSchemaObjectTable AttributeUses { get { if (attributeUses == null) { attributeUses = new XmlSchemaObjectTable(); } return attributeUses; } } ///[To be supplied.] ////// /// [XmlIgnore] public XmlSchemaAnyAttribute AttributeWildcard { get { return attributeWildcard; } } ///[To be supplied.] ////// /// [XmlIgnore] internal XmlSchemaObjectTable LocalElements { get { if (localElements == null) { localElements = new XmlSchemaObjectTable(); } return localElements; } } internal void SetContentTypeParticle(XmlSchemaParticle value) { contentTypeParticle = value; } internal void SetBlockResolved(XmlSchemaDerivationMethod value) { blockResolved = value; } internal void SetAttributeWildcard(XmlSchemaAnyAttribute value) { attributeWildcard = value; } internal bool HasWildCard { get { return (pvFlags & wildCardMask) != 0; } set { if (value) { pvFlags = (byte)(pvFlags | wildCardMask); } else { pvFlags = (byte)(pvFlags & ~wildCardMask); } } } internal bool HasDuplicateDecls { get { return (pvFlags & dupDeclMask) != 0; } set { if (value) { pvFlags = (byte)(pvFlags | dupDeclMask); } else { pvFlags = (byte)(pvFlags & ~dupDeclMask); } } } internal override XmlQualifiedName DerivedFrom { get { if (contentModel == null) { // type derived from anyType return XmlQualifiedName.Empty; } if (contentModel.Content is XmlSchemaComplexContentRestriction) return ((XmlSchemaComplexContentRestriction)contentModel.Content).BaseTypeName; else if (contentModel.Content is XmlSchemaComplexContentExtension) return ((XmlSchemaComplexContentExtension)contentModel.Content).BaseTypeName; else if (contentModel.Content is XmlSchemaSimpleContentRestriction) return ((XmlSchemaSimpleContentRestriction)contentModel.Content).BaseTypeName; else if (contentModel.Content is XmlSchemaSimpleContentExtension) return ((XmlSchemaSimpleContentExtension)contentModel.Content).BaseTypeName; else return XmlQualifiedName.Empty; } } internal void SetAttributes(XmlSchemaObjectCollection newAttributes) { attributes = newAttributes; } internal bool ContainsIdAttribute(bool findAll) { int idCount = 0; foreach(XmlSchemaAttribute attribute in this.AttributeUses.Values) { if (attribute.Use != XmlSchemaUse.Prohibited) { XmlSchemaDatatype datatype = attribute.Datatype; if (datatype != null && datatype.TypeCode == XmlTypeCode.Id) { idCount++; if (idCount > 1) { //two or more attributes is error break; } } } } return findAll ? (idCount > 1) : (idCount > 0); } internal override XmlSchemaObject Clone() { XmlSchemaComplexType complexType = (XmlSchemaComplexType)MemberwiseClone(); //Deep clone the QNames as these will be updated on chameleon includes if (complexType.ContentModel != null) { //simpleContent or complexContent XmlSchemaSimpleContent simpleContent = complexType.ContentModel as XmlSchemaSimpleContent; if (simpleContent != null) { XmlSchemaSimpleContent newSimpleContent = (XmlSchemaSimpleContent)simpleContent.Clone(); XmlSchemaSimpleContentExtension simpleExt = simpleContent.Content as XmlSchemaSimpleContentExtension; if (simpleExt != null) { XmlSchemaSimpleContentExtension newSimpleExt = (XmlSchemaSimpleContentExtension)simpleExt.Clone(); newSimpleExt.BaseTypeName = simpleExt.BaseTypeName.Clone(); newSimpleExt.SetAttributes(CloneAttributes(simpleExt.Attributes)); newSimpleContent.Content = newSimpleExt; } else { //simpleContent.Content is XmlSchemaSimpleContentRestriction XmlSchemaSimpleContentRestriction simpleRest = (XmlSchemaSimpleContentRestriction)simpleContent.Content; XmlSchemaSimpleContentRestriction newSimpleRest = (XmlSchemaSimpleContentRestriction)simpleRest.Clone(); newSimpleRest.BaseTypeName = simpleRest.BaseTypeName.Clone(); newSimpleRest.SetAttributes(CloneAttributes(simpleRest.Attributes)); newSimpleContent.Content = newSimpleRest; } complexType.ContentModel = newSimpleContent; } else { // complexType.ContentModel is XmlSchemaComplexContent XmlSchemaComplexContent complexContent = (XmlSchemaComplexContent)complexType.ContentModel; XmlSchemaComplexContent newComplexContent = (XmlSchemaComplexContent)complexContent.Clone(); XmlSchemaComplexContentExtension complexExt = complexContent.Content as XmlSchemaComplexContentExtension; if (complexExt != null) { XmlSchemaComplexContentExtension newComplexExt = (XmlSchemaComplexContentExtension)complexExt.Clone(); newComplexExt.BaseTypeName = complexExt.BaseTypeName.Clone(); newComplexExt.SetAttributes(CloneAttributes(complexExt.Attributes)); if (HasParticleRef(complexExt.Particle)) { newComplexExt.Particle = CloneParticle(complexExt.Particle); } newComplexContent.Content = newComplexExt; } else { // complexContent.Content is XmlSchemaComplexContentRestriction XmlSchemaComplexContentRestriction complexRest = complexContent.Content as XmlSchemaComplexContentRestriction; XmlSchemaComplexContentRestriction newComplexRest = (XmlSchemaComplexContentRestriction)complexRest.Clone(); newComplexRest.BaseTypeName = complexRest.BaseTypeName.Clone(); newComplexRest.SetAttributes(CloneAttributes(complexRest.Attributes)); if (HasParticleRef(newComplexRest.Particle)) { newComplexRest.Particle = CloneParticle(newComplexRest.Particle); } newComplexContent.Content = newComplexRest; } complexType.ContentModel = newComplexContent; } } else { //equals XmlSchemaComplexContent with baseType is anyType if (HasParticleRef(complexType.Particle)) { complexType.Particle = CloneParticle(complexType.Particle); } complexType.SetAttributes(CloneAttributes(complexType.Attributes)); } complexType.ClearCompiledState(); return complexType; } private void ClearCompiledState() { //Re-set post-compiled state for cloned object this.attributeUses = null; this.localElements = null; this.attributeWildcard = null; this.contentTypeParticle = XmlSchemaParticle.Empty; this.blockResolved = XmlSchemaDerivationMethod.None; } internal static XmlSchemaObjectCollection CloneAttributes(XmlSchemaObjectCollection attributes) { if (HasAttributeQNameRef(attributes)) { XmlSchemaObjectCollection newAttributes = attributes.Clone(); XmlSchemaAttributeGroupRef attributeGroupRef; XmlSchemaAttributeGroupRef newAttGroupRef; XmlSchemaObject xso; XmlSchemaAttribute att; for (int i = 0; i < attributes.Count; i++) { xso = attributes[i]; attributeGroupRef = xso as XmlSchemaAttributeGroupRef; if (attributeGroupRef != null) { newAttGroupRef = (XmlSchemaAttributeGroupRef)attributeGroupRef.Clone(); newAttGroupRef.RefName = attributeGroupRef.RefName.Clone(); newAttributes[i] = newAttGroupRef; } else { //Its XmlSchemaAttribute att = xso as XmlSchemaAttribute; if (!att.RefName.IsEmpty || !att.SchemaTypeName.IsEmpty) { newAttributes[i] = att.Clone(); } } } return newAttributes; } return attributes; } private static XmlSchemaObjectCollection CloneGroupBaseParticles(XmlSchemaObjectCollection groupBaseParticles) { XmlSchemaObjectCollection newParticles = groupBaseParticles.Clone(); for (int i = 0; i < groupBaseParticles.Count; i++) { XmlSchemaParticle p = (XmlSchemaParticle)groupBaseParticles[i]; newParticles[i] = CloneParticle(p); } return newParticles; } internal static XmlSchemaParticle CloneParticle(XmlSchemaParticle particle) { XmlSchemaGroupBase groupBase = particle as XmlSchemaGroupBase; if (groupBase != null && ! (groupBase is XmlSchemaAll)) { //Choice or sequence XmlSchemaGroupBase newGroupBase = groupBase; XmlSchemaObjectCollection newGroupbaseParticles = CloneGroupBaseParticles(groupBase.Items); newGroupBase = (XmlSchemaGroupBase)groupBase.Clone(); newGroupBase.SetItems(newGroupbaseParticles); return newGroupBase; } else if (particle is XmlSchemaGroupRef) { // group ref XmlSchemaGroupRef newGroupRef = (XmlSchemaGroupRef)particle.Clone(); newGroupRef.RefName = newGroupRef.RefName.Clone(); return newGroupRef; } else { XmlSchemaElement oldElem = particle as XmlSchemaElement; if (oldElem != null && (!oldElem.RefName.IsEmpty || !oldElem.SchemaTypeName.IsEmpty)) { //Its element ref or type name is present XmlSchemaElement newElem = (XmlSchemaElement)oldElem.Clone(); return newElem; } } return particle; } internal static bool HasParticleRef(XmlSchemaParticle particle) { XmlSchemaGroupBase groupBase = particle as XmlSchemaGroupBase; if (groupBase != null && ! (groupBase is XmlSchemaAll)) { bool foundRef = false; int i = 0; while (i < groupBase.Items.Count && !foundRef) { XmlSchemaParticle p = (XmlSchemaParticle)groupBase.Items[i++]; if (p is XmlSchemaGroupRef) { foundRef = true; } else { XmlSchemaElement elem = p as XmlSchemaElement; if (elem != null && (!elem.RefName.IsEmpty || !elem.SchemaTypeName.IsEmpty)) { foundRef = true; } else { foundRef = HasParticleRef(p); } } } return foundRef; } else if (particle is XmlSchemaGroupRef) { return true; } return false; } internal static bool HasAttributeQNameRef(XmlSchemaObjectCollection attributes) { foreach (XmlSchemaObject xso in attributes) { if (xso is XmlSchemaAttributeGroupRef) { return true; } else { XmlSchemaAttribute attribute = xso as XmlSchemaAttribute; if (!attribute.RefName.IsEmpty || !attribute.SchemaTypeName.IsEmpty) { return true; } } } return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Schema { using System.Collections; using System.ComponentModel; using System.Xml.Serialization; ////// /// public class XmlSchemaComplexType : XmlSchemaType { XmlSchemaDerivationMethod block = XmlSchemaDerivationMethod.None; XmlSchemaContentModel contentModel; XmlSchemaParticle particle; XmlSchemaObjectCollection attributes; XmlSchemaAnyAttribute anyAttribute; XmlSchemaParticle contentTypeParticle = XmlSchemaParticle.Empty; XmlSchemaDerivationMethod blockResolved; XmlSchemaObjectTable localElements; XmlSchemaObjectTable attributeUses; XmlSchemaAnyAttribute attributeWildcard; static XmlSchemaComplexType anyTypeLax; static XmlSchemaComplexType anyTypeSkip; static XmlSchemaComplexType untypedAnyType; //additional info for Partial validation byte pvFlags; const byte wildCardMask = 0x01; const byte dupDeclMask = 0x02; const byte isMixedMask = 0x04; const byte isAbstractMask = 0x08; static XmlSchemaComplexType() { anyTypeLax = CreateAnyType(XmlSchemaContentProcessing.Lax); anyTypeSkip = CreateAnyType(XmlSchemaContentProcessing.Skip); // Create xdt:untypedAny untypedAnyType = new XmlSchemaComplexType(); untypedAnyType.SetQualifiedName(new XmlQualifiedName("untypedAny", XmlReservedNs.NsXQueryDataType)); untypedAnyType.IsMixed = true; untypedAnyType.SetContentTypeParticle(anyTypeLax.ContentTypeParticle); untypedAnyType.SetContentType(XmlSchemaContentType.Mixed); untypedAnyType.ElementDecl = SchemaElementDecl.CreateAnyTypeElementDecl(); untypedAnyType.ElementDecl.SchemaType = untypedAnyType; untypedAnyType.ElementDecl.ContentValidator = AnyTypeContentValidator; } static XmlSchemaComplexType CreateAnyType(XmlSchemaContentProcessing processContents) { XmlSchemaComplexType localAnyType = new XmlSchemaComplexType(); localAnyType.SetQualifiedName(DatatypeImplementation.QnAnyType); XmlSchemaAny anyElement = new XmlSchemaAny(); anyElement.MinOccurs = decimal.Zero; anyElement.MaxOccurs = decimal.MaxValue; anyElement.ProcessContents = processContents; anyElement.BuildNamespaceList(null); XmlSchemaSequence seq = new XmlSchemaSequence(); seq.Items.Add(anyElement); localAnyType.SetContentTypeParticle(seq); localAnyType.SetContentType(XmlSchemaContentType.Mixed); localAnyType.ElementDecl = SchemaElementDecl.CreateAnyTypeElementDecl(); localAnyType.ElementDecl.SchemaType = localAnyType; //Create contentValidator for Any ParticleContentValidator contentValidator = new ParticleContentValidator(XmlSchemaContentType.Mixed); contentValidator.Start(); contentValidator.OpenGroup(); contentValidator.AddNamespaceList(anyElement.NamespaceList, anyElement); contentValidator.AddStar(); contentValidator.CloseGroup(); ContentValidator anyContentValidator = contentValidator.Finish(true); localAnyType.ElementDecl.ContentValidator = anyContentValidator; XmlSchemaAnyAttribute anyAttribute = new XmlSchemaAnyAttribute(); anyAttribute.ProcessContents = processContents; anyAttribute.BuildNamespaceList(null); localAnyType.SetAttributeWildcard(anyAttribute); localAnyType.ElementDecl.AnyAttribute = anyAttribute; return localAnyType; } ///[To be supplied.] ////// /// public XmlSchemaComplexType() { } [XmlIgnore] internal static XmlSchemaComplexType AnyType { get { return anyTypeLax; } } [XmlIgnore] internal static XmlSchemaComplexType UntypedAnyType { get { return untypedAnyType; } } [XmlIgnore] internal static XmlSchemaComplexType AnyTypeSkip { get { return anyTypeSkip; } } internal static ContentValidator AnyTypeContentValidator { get { return anyTypeLax.ElementDecl.ContentValidator; } } ///[To be supplied.] ////// /// [XmlAttribute("abstract"), DefaultValue(false)] public bool IsAbstract { get { return (pvFlags & isAbstractMask) != 0; } set { if (value) { pvFlags = (byte)(pvFlags | isAbstractMask); } else { pvFlags = (byte)(pvFlags & ~isAbstractMask); } } } ///[To be supplied.] ////// /// [XmlAttribute("block"), DefaultValue(XmlSchemaDerivationMethod.None)] public XmlSchemaDerivationMethod Block { get { return block; } set { block = value; } } ///[To be supplied.] ////// /// [XmlAttribute("mixed"), DefaultValue(false)] public override bool IsMixed { get { return (pvFlags & isMixedMask) != 0; } set { if (value) { pvFlags = (byte)(pvFlags | isMixedMask); } else { pvFlags = (byte)(pvFlags & ~isMixedMask); } } } ///[To be supplied.] ////// /// [XmlElement("simpleContent", typeof(XmlSchemaSimpleContent)), XmlElement("complexContent", typeof(XmlSchemaComplexContent))] public XmlSchemaContentModel ContentModel { get { return contentModel; } set { contentModel = value; } } ///[To be supplied.] ////// /// [XmlElement("group", typeof(XmlSchemaGroupRef)), XmlElement("choice", typeof(XmlSchemaChoice)), XmlElement("all", typeof(XmlSchemaAll)), XmlElement("sequence", typeof(XmlSchemaSequence))] public XmlSchemaParticle Particle { get { return particle; } set { particle = value; } } ///[To be supplied.] ////// /// [XmlElement("attribute", typeof(XmlSchemaAttribute)), XmlElement("attributeGroup", typeof(XmlSchemaAttributeGroupRef))] public XmlSchemaObjectCollection Attributes { get { if (attributes == null) { attributes = new XmlSchemaObjectCollection(); } return attributes; } } ///[To be supplied.] ////// /// [XmlElement("anyAttribute")] public XmlSchemaAnyAttribute AnyAttribute { get { return anyAttribute; } set { anyAttribute = value; } } ///[To be supplied.] ////// /// [XmlIgnore] public XmlSchemaContentType ContentType { get { return SchemaContentType; } } ///[To be supplied.] ////// /// [XmlIgnore] public XmlSchemaParticle ContentTypeParticle { get { return contentTypeParticle; } } ///[To be supplied.] ////// /// [XmlIgnore] public XmlSchemaDerivationMethod BlockResolved { get { return blockResolved; } } ///[To be supplied.] ////// /// [XmlIgnore] public XmlSchemaObjectTable AttributeUses { get { if (attributeUses == null) { attributeUses = new XmlSchemaObjectTable(); } return attributeUses; } } ///[To be supplied.] ////// /// [XmlIgnore] public XmlSchemaAnyAttribute AttributeWildcard { get { return attributeWildcard; } } ///[To be supplied.] ////// /// [XmlIgnore] internal XmlSchemaObjectTable LocalElements { get { if (localElements == null) { localElements = new XmlSchemaObjectTable(); } return localElements; } } internal void SetContentTypeParticle(XmlSchemaParticle value) { contentTypeParticle = value; } internal void SetBlockResolved(XmlSchemaDerivationMethod value) { blockResolved = value; } internal void SetAttributeWildcard(XmlSchemaAnyAttribute value) { attributeWildcard = value; } internal bool HasWildCard { get { return (pvFlags & wildCardMask) != 0; } set { if (value) { pvFlags = (byte)(pvFlags | wildCardMask); } else { pvFlags = (byte)(pvFlags & ~wildCardMask); } } } internal bool HasDuplicateDecls { get { return (pvFlags & dupDeclMask) != 0; } set { if (value) { pvFlags = (byte)(pvFlags | dupDeclMask); } else { pvFlags = (byte)(pvFlags & ~dupDeclMask); } } } internal override XmlQualifiedName DerivedFrom { get { if (contentModel == null) { // type derived from anyType return XmlQualifiedName.Empty; } if (contentModel.Content is XmlSchemaComplexContentRestriction) return ((XmlSchemaComplexContentRestriction)contentModel.Content).BaseTypeName; else if (contentModel.Content is XmlSchemaComplexContentExtension) return ((XmlSchemaComplexContentExtension)contentModel.Content).BaseTypeName; else if (contentModel.Content is XmlSchemaSimpleContentRestriction) return ((XmlSchemaSimpleContentRestriction)contentModel.Content).BaseTypeName; else if (contentModel.Content is XmlSchemaSimpleContentExtension) return ((XmlSchemaSimpleContentExtension)contentModel.Content).BaseTypeName; else return XmlQualifiedName.Empty; } } internal void SetAttributes(XmlSchemaObjectCollection newAttributes) { attributes = newAttributes; } internal bool ContainsIdAttribute(bool findAll) { int idCount = 0; foreach(XmlSchemaAttribute attribute in this.AttributeUses.Values) { if (attribute.Use != XmlSchemaUse.Prohibited) { XmlSchemaDatatype datatype = attribute.Datatype; if (datatype != null && datatype.TypeCode == XmlTypeCode.Id) { idCount++; if (idCount > 1) { //two or more attributes is error break; } } } } return findAll ? (idCount > 1) : (idCount > 0); } internal override XmlSchemaObject Clone() { XmlSchemaComplexType complexType = (XmlSchemaComplexType)MemberwiseClone(); //Deep clone the QNames as these will be updated on chameleon includes if (complexType.ContentModel != null) { //simpleContent or complexContent XmlSchemaSimpleContent simpleContent = complexType.ContentModel as XmlSchemaSimpleContent; if (simpleContent != null) { XmlSchemaSimpleContent newSimpleContent = (XmlSchemaSimpleContent)simpleContent.Clone(); XmlSchemaSimpleContentExtension simpleExt = simpleContent.Content as XmlSchemaSimpleContentExtension; if (simpleExt != null) { XmlSchemaSimpleContentExtension newSimpleExt = (XmlSchemaSimpleContentExtension)simpleExt.Clone(); newSimpleExt.BaseTypeName = simpleExt.BaseTypeName.Clone(); newSimpleExt.SetAttributes(CloneAttributes(simpleExt.Attributes)); newSimpleContent.Content = newSimpleExt; } else { //simpleContent.Content is XmlSchemaSimpleContentRestriction XmlSchemaSimpleContentRestriction simpleRest = (XmlSchemaSimpleContentRestriction)simpleContent.Content; XmlSchemaSimpleContentRestriction newSimpleRest = (XmlSchemaSimpleContentRestriction)simpleRest.Clone(); newSimpleRest.BaseTypeName = simpleRest.BaseTypeName.Clone(); newSimpleRest.SetAttributes(CloneAttributes(simpleRest.Attributes)); newSimpleContent.Content = newSimpleRest; } complexType.ContentModel = newSimpleContent; } else { // complexType.ContentModel is XmlSchemaComplexContent XmlSchemaComplexContent complexContent = (XmlSchemaComplexContent)complexType.ContentModel; XmlSchemaComplexContent newComplexContent = (XmlSchemaComplexContent)complexContent.Clone(); XmlSchemaComplexContentExtension complexExt = complexContent.Content as XmlSchemaComplexContentExtension; if (complexExt != null) { XmlSchemaComplexContentExtension newComplexExt = (XmlSchemaComplexContentExtension)complexExt.Clone(); newComplexExt.BaseTypeName = complexExt.BaseTypeName.Clone(); newComplexExt.SetAttributes(CloneAttributes(complexExt.Attributes)); if (HasParticleRef(complexExt.Particle)) { newComplexExt.Particle = CloneParticle(complexExt.Particle); } newComplexContent.Content = newComplexExt; } else { // complexContent.Content is XmlSchemaComplexContentRestriction XmlSchemaComplexContentRestriction complexRest = complexContent.Content as XmlSchemaComplexContentRestriction; XmlSchemaComplexContentRestriction newComplexRest = (XmlSchemaComplexContentRestriction)complexRest.Clone(); newComplexRest.BaseTypeName = complexRest.BaseTypeName.Clone(); newComplexRest.SetAttributes(CloneAttributes(complexRest.Attributes)); if (HasParticleRef(newComplexRest.Particle)) { newComplexRest.Particle = CloneParticle(newComplexRest.Particle); } newComplexContent.Content = newComplexRest; } complexType.ContentModel = newComplexContent; } } else { //equals XmlSchemaComplexContent with baseType is anyType if (HasParticleRef(complexType.Particle)) { complexType.Particle = CloneParticle(complexType.Particle); } complexType.SetAttributes(CloneAttributes(complexType.Attributes)); } complexType.ClearCompiledState(); return complexType; } private void ClearCompiledState() { //Re-set post-compiled state for cloned object this.attributeUses = null; this.localElements = null; this.attributeWildcard = null; this.contentTypeParticle = XmlSchemaParticle.Empty; this.blockResolved = XmlSchemaDerivationMethod.None; } internal static XmlSchemaObjectCollection CloneAttributes(XmlSchemaObjectCollection attributes) { if (HasAttributeQNameRef(attributes)) { XmlSchemaObjectCollection newAttributes = attributes.Clone(); XmlSchemaAttributeGroupRef attributeGroupRef; XmlSchemaAttributeGroupRef newAttGroupRef; XmlSchemaObject xso; XmlSchemaAttribute att; for (int i = 0; i < attributes.Count; i++) { xso = attributes[i]; attributeGroupRef = xso as XmlSchemaAttributeGroupRef; if (attributeGroupRef != null) { newAttGroupRef = (XmlSchemaAttributeGroupRef)attributeGroupRef.Clone(); newAttGroupRef.RefName = attributeGroupRef.RefName.Clone(); newAttributes[i] = newAttGroupRef; } else { //Its XmlSchemaAttribute att = xso as XmlSchemaAttribute; if (!att.RefName.IsEmpty || !att.SchemaTypeName.IsEmpty) { newAttributes[i] = att.Clone(); } } } return newAttributes; } return attributes; } private static XmlSchemaObjectCollection CloneGroupBaseParticles(XmlSchemaObjectCollection groupBaseParticles) { XmlSchemaObjectCollection newParticles = groupBaseParticles.Clone(); for (int i = 0; i < groupBaseParticles.Count; i++) { XmlSchemaParticle p = (XmlSchemaParticle)groupBaseParticles[i]; newParticles[i] = CloneParticle(p); } return newParticles; } internal static XmlSchemaParticle CloneParticle(XmlSchemaParticle particle) { XmlSchemaGroupBase groupBase = particle as XmlSchemaGroupBase; if (groupBase != null && ! (groupBase is XmlSchemaAll)) { //Choice or sequence XmlSchemaGroupBase newGroupBase = groupBase; XmlSchemaObjectCollection newGroupbaseParticles = CloneGroupBaseParticles(groupBase.Items); newGroupBase = (XmlSchemaGroupBase)groupBase.Clone(); newGroupBase.SetItems(newGroupbaseParticles); return newGroupBase; } else if (particle is XmlSchemaGroupRef) { // group ref XmlSchemaGroupRef newGroupRef = (XmlSchemaGroupRef)particle.Clone(); newGroupRef.RefName = newGroupRef.RefName.Clone(); return newGroupRef; } else { XmlSchemaElement oldElem = particle as XmlSchemaElement; if (oldElem != null && (!oldElem.RefName.IsEmpty || !oldElem.SchemaTypeName.IsEmpty)) { //Its element ref or type name is present XmlSchemaElement newElem = (XmlSchemaElement)oldElem.Clone(); return newElem; } } return particle; } internal static bool HasParticleRef(XmlSchemaParticle particle) { XmlSchemaGroupBase groupBase = particle as XmlSchemaGroupBase; if (groupBase != null && ! (groupBase is XmlSchemaAll)) { bool foundRef = false; int i = 0; while (i < groupBase.Items.Count && !foundRef) { XmlSchemaParticle p = (XmlSchemaParticle)groupBase.Items[i++]; if (p is XmlSchemaGroupRef) { foundRef = true; } else { XmlSchemaElement elem = p as XmlSchemaElement; if (elem != null && (!elem.RefName.IsEmpty || !elem.SchemaTypeName.IsEmpty)) { foundRef = true; } else { foundRef = HasParticleRef(p); } } } return foundRef; } else if (particle is XmlSchemaGroupRef) { return true; } return false; } internal static bool HasAttributeQNameRef(XmlSchemaObjectCollection attributes) { foreach (XmlSchemaObject xso in attributes) { if (xso is XmlSchemaAttributeGroupRef) { return true; } else { XmlSchemaAttribute attribute = xso as XmlSchemaAttribute; if (!attribute.RefName.IsEmpty || !attribute.SchemaTypeName.IsEmpty) { return true; } } } return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
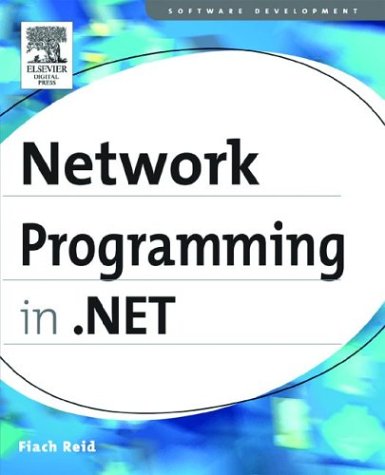
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Transform3DCollection.cs
- TemplateXamlTreeBuilder.cs
- XPathNavigator.cs
- DataGridViewCellToolTipTextNeededEventArgs.cs
- Rect3D.cs
- VideoDrawing.cs
- StickyNoteAnnotations.cs
- SoapReflectionImporter.cs
- ObjectDisposedException.cs
- BufferedResponseStream.cs
- IChannel.cs
- DataGridViewHeaderCell.cs
- XmlILAnnotation.cs
- HttpWebRequest.cs
- ToolStripGripRenderEventArgs.cs
- TableItemStyle.cs
- TemplateColumn.cs
- SQLBytes.cs
- ProcessInputEventArgs.cs
- DBSqlParserColumnCollection.cs
- ColorConvertedBitmap.cs
- TriggerCollection.cs
- ZipPackage.cs
- FixedFindEngine.cs
- TemplateField.cs
- ImageBrush.cs
- HttpStaticObjectsCollectionWrapper.cs
- CustomWebEventKey.cs
- EdmProviderManifest.cs
- CodeCommentStatement.cs
- BinaryObjectWriter.cs
- ScriptBehaviorDescriptor.cs
- Scene3D.cs
- DurableInstanceContextProvider.cs
- Object.cs
- ReachSerializationCacheItems.cs
- PersistenceTypeAttribute.cs
- RolePrincipal.cs
- categoryentry.cs
- ArrayElementGridEntry.cs
- Walker.cs
- InputLanguage.cs
- ToolStripAdornerWindowService.cs
- ButtonColumn.cs
- MbpInfo.cs
- DefaultProxySection.cs
- SqlGenericUtil.cs
- ISO2022Encoding.cs
- SafeNativeMethodsOther.cs
- XmlNullResolver.cs
- BoundColumn.cs
- UpdateRecord.cs
- MediaTimeline.cs
- XComponentModel.cs
- TaiwanLunisolarCalendar.cs
- Style.cs
- DrawingGroupDrawingContext.cs
- DesignerProperties.cs
- SHA1Managed.cs
- ReturnType.cs
- AsymmetricKeyExchangeDeformatter.cs
- MailMessageEventArgs.cs
- XmlCompatibilityReader.cs
- columnmapkeybuilder.cs
- GradientStop.cs
- TabItemWrapperAutomationPeer.cs
- XmlUrlResolver.cs
- externdll.cs
- SQLInt64Storage.cs
- ClientTarget.cs
- VBIdentifierNameEditor.cs
- DataGridCheckBoxColumn.cs
- DateTimeHelper.cs
- XmlNamespaceMapping.cs
- Rect3DConverter.cs
- FormViewPageEventArgs.cs
- DelegateHelpers.Generated.cs
- RecipientServiceModelSecurityTokenRequirement.cs
- UrlMappingsSection.cs
- Model3DCollection.cs
- DoubleConverter.cs
- MailSettingsSection.cs
- HitTestWithGeometryDrawingContextWalker.cs
- XmlNavigatorFilter.cs
- PromptStyle.cs
- ReadOnlyState.cs
- Stopwatch.cs
- OperationInvokerTrace.cs
- Peer.cs
- InvokeProviderWrapper.cs
- FileSystemEventArgs.cs
- DocumentPage.cs
- BlockingCollection.cs
- SqlDataReaderSmi.cs
- OleDbPropertySetGuid.cs
- storepermissionattribute.cs
- StyleTypedPropertyAttribute.cs
- ProfileManager.cs
- ElementAtQueryOperator.cs
- GradientStopCollection.cs