Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / Xml / System / Xml / XPath / XPathNodeIterator.cs / 1 / XPathNodeIterator.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.XPath { using System; using System.Collections; using System.Diagnostics; using System.Text; [DebuggerDisplay("Position={CurrentPosition}, Current={debuggerDisplayProxy}")] public abstract class XPathNodeIterator : ICloneable, IEnumerable { internal int count = -1; object ICloneable.Clone() { return this.Clone(); } public abstract XPathNodeIterator Clone(); public abstract bool MoveNext(); public abstract XPathNavigator Current { get; } public abstract int CurrentPosition { get; } public virtual int Count { get { if (count == -1) { XPathNodeIterator clone = this.Clone(); while(clone.MoveNext()) ; count = clone.CurrentPosition; } return count; } } public virtual IEnumerator GetEnumerator() { return new Enumerator(this); } private object debuggerDisplayProxy { get { return Current == null ? null : (object)new XPathNavigator.DebuggerDisplayProxy(Current); } } ////// Implementation of a resetable enumerator that is linked to the XPathNodeIterator used to create it. /// private class Enumerator : IEnumerator { private XPathNodeIterator original; // Keep original XPathNodeIterator in case Reset() is called private XPathNodeIterator current; private bool iterationStarted; public Enumerator(XPathNodeIterator original) { this.original = original.Clone(); } public virtual object Current { get { // 1. Do not reuse the XPathNavigator, as we do in XPathNodeIterator // 2. Throw exception if current position is before first node or after the last node if (this.iterationStarted) { // Current is null if iterator is positioned after the last node if (this.current == null) throw new InvalidOperationException(Res.GetString(Res.Sch_EnumFinished, string.Empty)); return this.current.Current.Clone(); } // User must call MoveNext before accessing Current property throw new InvalidOperationException(Res.GetString(Res.Sch_EnumNotStarted, string.Empty)); } } public virtual bool MoveNext() { // Delegate to XPathNodeIterator if (!this.iterationStarted) { // Reset iteration to original position this.current = this.original.Clone(); this.iterationStarted = true; } if (this.current == null || !this.current.MoveNext()) { // Iteration complete this.current = null; return false; } return true; } public virtual void Reset() { this.iterationStarted = false; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.XPath { using System; using System.Collections; using System.Diagnostics; using System.Text; [DebuggerDisplay("Position={CurrentPosition}, Current={debuggerDisplayProxy}")] public abstract class XPathNodeIterator : ICloneable, IEnumerable { internal int count = -1; object ICloneable.Clone() { return this.Clone(); } public abstract XPathNodeIterator Clone(); public abstract bool MoveNext(); public abstract XPathNavigator Current { get; } public abstract int CurrentPosition { get; } public virtual int Count { get { if (count == -1) { XPathNodeIterator clone = this.Clone(); while(clone.MoveNext()) ; count = clone.CurrentPosition; } return count; } } public virtual IEnumerator GetEnumerator() { return new Enumerator(this); } private object debuggerDisplayProxy { get { return Current == null ? null : (object)new XPathNavigator.DebuggerDisplayProxy(Current); } } ////// Implementation of a resetable enumerator that is linked to the XPathNodeIterator used to create it. /// private class Enumerator : IEnumerator { private XPathNodeIterator original; // Keep original XPathNodeIterator in case Reset() is called private XPathNodeIterator current; private bool iterationStarted; public Enumerator(XPathNodeIterator original) { this.original = original.Clone(); } public virtual object Current { get { // 1. Do not reuse the XPathNavigator, as we do in XPathNodeIterator // 2. Throw exception if current position is before first node or after the last node if (this.iterationStarted) { // Current is null if iterator is positioned after the last node if (this.current == null) throw new InvalidOperationException(Res.GetString(Res.Sch_EnumFinished, string.Empty)); return this.current.Current.Clone(); } // User must call MoveNext before accessing Current property throw new InvalidOperationException(Res.GetString(Res.Sch_EnumNotStarted, string.Empty)); } } public virtual bool MoveNext() { // Delegate to XPathNodeIterator if (!this.iterationStarted) { // Reset iteration to original position this.current = this.original.Clone(); this.iterationStarted = true; } if (this.current == null || !this.current.MoveNext()) { // Iteration complete this.current = null; return false; } return true; } public virtual void Reset() { this.iterationStarted = false; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
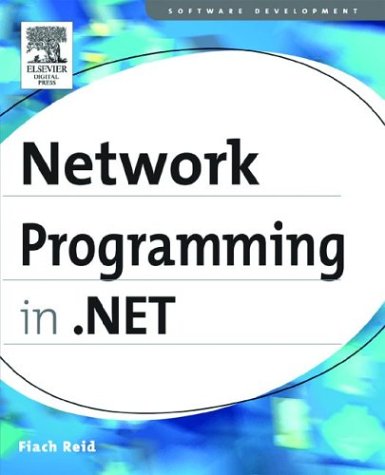
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NumericExpr.cs
- NavigationPropertySingletonExpression.cs
- CompModHelpers.cs
- HtmlTableRowCollection.cs
- EncryptedType.cs
- TreeView.cs
- UpdateInfo.cs
- EventManager.cs
- StateWorkerRequest.cs
- DataObjectPastingEventArgs.cs
- CqlErrorHelper.cs
- ProvideValueServiceProvider.cs
- BooleanProjectedSlot.cs
- InfoCardRequestException.cs
- EntityClientCacheEntry.cs
- TextBreakpoint.cs
- OraclePermissionAttribute.cs
- BypassElementCollection.cs
- HttpServerUtilityBase.cs
- ButtonFlatAdapter.cs
- ViewManager.cs
- TextAutomationPeer.cs
- TextDpi.cs
- Profiler.cs
- BrowserDefinitionCollection.cs
- ServiceOperationListItemList.cs
- DefaultTextStore.cs
- XPathParser.cs
- ImageListImageEditor.cs
- PersistenceProvider.cs
- RecognizedWordUnit.cs
- ImageIndexConverter.cs
- SqlDataSourceDesigner.cs
- DataGridState.cs
- SoapIgnoreAttribute.cs
- ObjectItemCachedAssemblyLoader.cs
- ProtocolElementCollection.cs
- CustomValidator.cs
- DnsPermission.cs
- NumberSubstitution.cs
- FixedPosition.cs
- ScriptRegistrationManager.cs
- PrivilegedConfigurationManager.cs
- BinaryObjectInfo.cs
- MainMenu.cs
- Rect.cs
- DesignRelation.cs
- ProvidersHelper.cs
- RegexCharClass.cs
- ImageEditor.cs
- ListBox.cs
- NameTable.cs
- ISessionStateStore.cs
- InkCanvasSelectionAdorner.cs
- DataSourceView.cs
- MissingMethodException.cs
- ConfigurationException.cs
- LinqDataSourceUpdateEventArgs.cs
- DataGridCell.cs
- WebConfigurationManager.cs
- OraclePermission.cs
- DataObjectSettingDataEventArgs.cs
- Activity.cs
- HttpCapabilitiesBase.cs
- Item.cs
- HandlerMappingMemo.cs
- XPathNodePointer.cs
- HandlerBase.cs
- NegatedConstant.cs
- FontUnit.cs
- DSASignatureDeformatter.cs
- FeatureSupport.cs
- XslVisitor.cs
- FileVersionInfo.cs
- ItemCheckEvent.cs
- InputScope.cs
- OdbcConnectionHandle.cs
- Control.cs
- CultureTableRecord.cs
- NetworkStream.cs
- MimeBasePart.cs
- ZipIOZip64EndOfCentralDirectoryLocatorBlock.cs
- HtmlToClrEventProxy.cs
- PlainXmlSerializer.cs
- MasterPage.cs
- SystemFonts.cs
- BooleanAnimationUsingKeyFrames.cs
- XmlEntity.cs
- TextDecorationCollection.cs
- BamlWriter.cs
- AdornerPresentationContext.cs
- BinHexEncoding.cs
- _NtlmClient.cs
- SecurityCriticalDataForSet.cs
- AssertFilter.cs
- ClientScriptManagerWrapper.cs
- MenuItem.cs
- PackageStore.cs
- DocumentPageHost.cs
- BrowsableAttribute.cs