Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / clr / src / BCL / System / UIntPtr.cs / 1 / UIntPtr.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: UIntPtr ** ** ** Purpose: Platform independent integer ** ** ===========================================================*/ namespace System { using System; using System.Globalization; using System.Runtime.Serialization; [Serializable(),CLSCompliant(false)] [System.Runtime.InteropServices.ComVisible(true)] public struct UIntPtr : ISerializable { unsafe private void* m_value; public static readonly UIntPtr Zero; public unsafe UIntPtr(uint value) { m_value = (void *)value; } public unsafe UIntPtr(ulong value) { #if WIN32 m_value = (void *)checked((uint)value); #else m_value = (void *)value; #endif } [CLSCompliant(false)] public unsafe UIntPtr(void* value) { m_value = value; } private unsafe UIntPtr(SerializationInfo info, StreamingContext context) { ulong l = info.GetUInt64("value"); if (Size==4 && l>UInt32.MaxValue) { throw new ArgumentException(Environment.GetResourceString("Serialization_InvalidPtrValue")); } m_value = (void *)l; } unsafe void ISerializable.GetObjectData(SerializationInfo info, StreamingContext context) { if (info==null) { throw new ArgumentNullException("info"); } info.AddValue("value", (ulong)m_value); } public unsafe override bool Equals(Object obj) { if (obj is UIntPtr) { return (m_value == ((UIntPtr)obj).m_value); } return false; } public unsafe override int GetHashCode() { return unchecked((int)((long)m_value)) & 0x7fffffff; } public unsafe uint ToUInt32() { #if WIN32 return (uint)m_value; #else return checked((uint)m_value); #endif } public unsafe ulong ToUInt64() { return (ulong)m_value; } public unsafe override String ToString() { #if WIN32 return ((uint)m_value).ToString(CultureInfo.InvariantCulture); #else return ((ulong)m_value).ToString(CultureInfo.InvariantCulture); #endif } public static explicit operator UIntPtr (uint value) { return new UIntPtr(value); } public static explicit operator UIntPtr (ulong value) { return new UIntPtr(value); } public unsafe static explicit operator uint (UIntPtr value) { #if WIN32 return (uint)value.m_value; #else return checked((uint)value.m_value); #endif } public unsafe static explicit operator ulong (UIntPtr value) { return (ulong)value.m_value; } [CLSCompliant(false)] public static unsafe explicit operator UIntPtr (void* value) { return new UIntPtr(value); } [CLSCompliant(false)] public static unsafe explicit operator void* (UIntPtr value) { return value.ToPointer(); } public unsafe static bool operator == (UIntPtr value1, UIntPtr value2) { return value1.m_value == value2.m_value; } public unsafe static bool operator != (UIntPtr value1, UIntPtr value2) { return value1.m_value != value2.m_value; } public static int Size { get { #if WIN32 return 4; #else return 8; #endif } } [CLSCompliant(false)] public unsafe void* ToPointer() { return m_value; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: UIntPtr ** ** ** Purpose: Platform independent integer ** ** ===========================================================*/ namespace System { using System; using System.Globalization; using System.Runtime.Serialization; [Serializable(),CLSCompliant(false)] [System.Runtime.InteropServices.ComVisible(true)] public struct UIntPtr : ISerializable { unsafe private void* m_value; public static readonly UIntPtr Zero; public unsafe UIntPtr(uint value) { m_value = (void *)value; } public unsafe UIntPtr(ulong value) { #if WIN32 m_value = (void *)checked((uint)value); #else m_value = (void *)value; #endif } [CLSCompliant(false)] public unsafe UIntPtr(void* value) { m_value = value; } private unsafe UIntPtr(SerializationInfo info, StreamingContext context) { ulong l = info.GetUInt64("value"); if (Size==4 && l>UInt32.MaxValue) { throw new ArgumentException(Environment.GetResourceString("Serialization_InvalidPtrValue")); } m_value = (void *)l; } unsafe void ISerializable.GetObjectData(SerializationInfo info, StreamingContext context) { if (info==null) { throw new ArgumentNullException("info"); } info.AddValue("value", (ulong)m_value); } public unsafe override bool Equals(Object obj) { if (obj is UIntPtr) { return (m_value == ((UIntPtr)obj).m_value); } return false; } public unsafe override int GetHashCode() { return unchecked((int)((long)m_value)) & 0x7fffffff; } public unsafe uint ToUInt32() { #if WIN32 return (uint)m_value; #else return checked((uint)m_value); #endif } public unsafe ulong ToUInt64() { return (ulong)m_value; } public unsafe override String ToString() { #if WIN32 return ((uint)m_value).ToString(CultureInfo.InvariantCulture); #else return ((ulong)m_value).ToString(CultureInfo.InvariantCulture); #endif } public static explicit operator UIntPtr (uint value) { return new UIntPtr(value); } public static explicit operator UIntPtr (ulong value) { return new UIntPtr(value); } public unsafe static explicit operator uint (UIntPtr value) { #if WIN32 return (uint)value.m_value; #else return checked((uint)value.m_value); #endif } public unsafe static explicit operator ulong (UIntPtr value) { return (ulong)value.m_value; } [CLSCompliant(false)] public static unsafe explicit operator UIntPtr (void* value) { return new UIntPtr(value); } [CLSCompliant(false)] public static unsafe explicit operator void* (UIntPtr value) { return value.ToPointer(); } public unsafe static bool operator == (UIntPtr value1, UIntPtr value2) { return value1.m_value == value2.m_value; } public unsafe static bool operator != (UIntPtr value1, UIntPtr value2) { return value1.m_value != value2.m_value; } public static int Size { get { #if WIN32 return 4; #else return 8; #endif } } [CLSCompliant(false)] public unsafe void* ToPointer() { return m_value; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
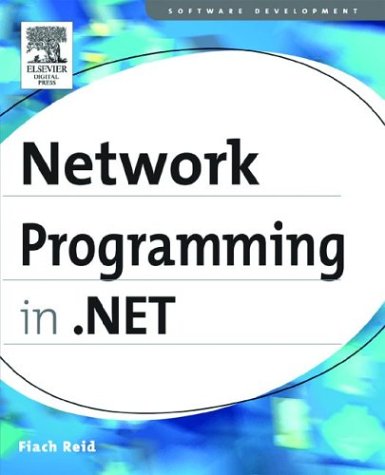
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProviderConnectionPointCollection.cs
- BehaviorEditorPart.cs
- SqlWriter.cs
- ExceptionRoutedEventArgs.cs
- MessageSecurityVersion.cs
- ElementHostAutomationPeer.cs
- IssuanceLicense.cs
- RelatedView.cs
- QilLoop.cs
- ExpandedProjectionNode.cs
- HitTestFilterBehavior.cs
- TextEditorMouse.cs
- MessagePartDescriptionCollection.cs
- ScrollBarRenderer.cs
- _NestedSingleAsyncResult.cs
- Parameter.cs
- TokenizerHelper.cs
- StsCommunicationException.cs
- WindowsFormsLinkLabel.cs
- BitmapData.cs
- EntityDataSourceDataSelection.cs
- EventMappingSettings.cs
- GB18030Encoding.cs
- KeyTime.cs
- XmlDownloadManager.cs
- PropertyManager.cs
- AccessDataSourceWizardForm.cs
- DataServices.cs
- TdsRecordBufferSetter.cs
- TextEditorThreadLocalStore.cs
- ContextProperty.cs
- AttributeEmitter.cs
- BufferManager.cs
- SocketException.cs
- TransformedBitmap.cs
- EngineSiteSapi.cs
- SqlConnectionPoolProviderInfo.cs
- ICspAsymmetricAlgorithm.cs
- BCLDebug.cs
- WebScriptServiceHostFactory.cs
- entityreference_tresulttype.cs
- CommandPlan.cs
- TypeExtensionConverter.cs
- UInt32Converter.cs
- RolePrincipal.cs
- ProcessProtocolHandler.cs
- TreeNodeBindingCollection.cs
- XmlSchemaInfo.cs
- Expressions.cs
- FrameworkContentElement.cs
- X509PeerCertificateElement.cs
- MutexSecurity.cs
- PolyBezierSegment.cs
- HandledEventArgs.cs
- DatePickerDateValidationErrorEventArgs.cs
- ReadOnlyDataSourceView.cs
- PropertyChangingEventArgs.cs
- SerializationObjectManager.cs
- TextSpan.cs
- InfoCardRSAPKCS1SignatureDeformatter.cs
- LongAverageAggregationOperator.cs
- ParameterModifier.cs
- WebPartPersonalization.cs
- UpdatePanelControlTrigger.cs
- ToolStripPanel.cs
- TimeSpanSecondsOrInfiniteConverter.cs
- MimeObjectFactory.cs
- CorrelationTokenTypeConvertor.cs
- PreservationFileReader.cs
- DisposableCollectionWrapper.cs
- FrameworkContentElementAutomationPeer.cs
- XmlNodeList.cs
- BitmapEffectCollection.cs
- Accessible.cs
- UnionCodeGroup.cs
- DoubleStorage.cs
- RemotingException.cs
- TreeViewDataItemAutomationPeer.cs
- MenuTracker.cs
- EmbeddedMailObjectsCollection.cs
- XmlTextReaderImpl.cs
- WindowsEditBoxRange.cs
- VBIdentifierTrimConverter.cs
- DataSvcMapFile.cs
- CharAnimationUsingKeyFrames.cs
- SendMailErrorEventArgs.cs
- BinHexDecoder.cs
- TabControlCancelEvent.cs
- XmlSchemaImport.cs
- PropertyGeneratedEventArgs.cs
- ErrorEventArgs.cs
- GCHandleCookieTable.cs
- CodePageEncoding.cs
- ExpressionConverter.cs
- ListViewItem.cs
- TreeNode.cs
- PropertyEmitterBase.cs
- DataServiceSaveChangesEventArgs.cs
- ScriptResourceMapping.cs
- DesignerSerializerAttribute.cs