Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Media / ArcSegment.cs / 1 / ArcSegment.cs
//-------------------------------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: Impelementation of ArcSegment // // History: // //------------------------------------------------------------------------------------------------- using MS.Internal; using MS.Internal.PresentationCore; using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Text; using System.Security; using System.Security.Permissions; using System.Text.RegularExpressions; using System.Windows.Media; using System.Windows; using System.Windows.Media.Composition; using System.Windows.Media.Animation; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// ArcSegment /// public sealed partial class ArcSegment : PathSegment { #region Constructors ////// /// public ArcSegment() { } ////// /// public ArcSegment( Point point, Size size, double rotationAngle, bool isLargeArc, SweepDirection sweepDirection, bool isStroked) { Size = size; RotationAngle = rotationAngle; IsLargeArc = isLargeArc; SweepDirection = sweepDirection; Point = point; IsStroked = isStroked; } #endregion #region AddToFigure ////// Critical: This code calls into MilUtility_ArcToBezier which has an unmanaged code elevation /// TreatAsSafe: Adding a figure is considered safe in partial trust. /// [SecurityCritical,SecurityTreatAsSafe] internal override void AddToFigure( Matrix matrix, // The transformation matrid PathFigure figure, // The figure to add to ref Point current) // In: Segment start point, Out: Segment endpoint, neither transformed { Point endPoint = Point; if (matrix.IsIdentity) { figure.Segments.Add(this); } else { // The arc segment is approximated by up to 4 Bezier segments unsafe { int count; Point* points = stackalloc Point[12]; Size size = Size; Double rotation = RotationAngle; MilMatrix3x2D mat3X2 = CompositionResourceManager.MatrixToMilMatrix3x2D(ref matrix); Composition.MilCoreApi.MilUtility_ArcToBezier( current, // =start point size, rotation, IsLargeArc, SweepDirection, endPoint, &mat3X2, points, out count); // = number of Bezier segments Invariant.Assert(count <= 4); // To ensure no buffer overflows count = Math.Min(count, 4); bool isStroked = IsStroked; bool isSmoothJoin = IsSmoothJoin; // Add the segments if (count > 0) { for (int i = 0; i < count; i++) { figure.Segments.Add(new BezierSegment( points[3*i], points[3*i + 1], points[3*i + 2], isStroked, (i < count - 1) || isSmoothJoin)); // Smooth join between arc pieces } } else if (count == 0) { figure.Segments.Add(new LineSegment(points[0], isStroked, isSmoothJoin)); } } // Update the last point current = endPoint; } } #endregion ////// SerializeData - Serialize the contents of this Segment to the provided context. /// internal override void SerializeData(StreamGeometryContext ctx) { ctx.ArcTo(Point, Size, RotationAngle, IsLargeArc, SweepDirection, IsStroked, IsSmoothJoin); } internal override bool IsCurved() { return true; } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// internal override string ConvertToString(string format, IFormatProvider provider) { // Helper to get the numeric list separator for a given culture. char separator = MS.Internal.TokenizerHelper.GetNumericListSeparator(provider); return String.Format(provider, "A{1:" + format + "}{0}{2:" + format + "}{0}{3}{0}{4}{0}{5:" + format + "}", separator, Size, RotationAngle, IsLargeArc ? "1" : "0", SweepDirection == SweepDirection.Clockwise ? "1" : "0", Point); } // // This property // 1. Finds the correct initial size for the _effectiveValues store on the current DependencyObject // 2. This is a performance optimization // internal override int EffectiveValuesInitialSize { get { return 6; } } ////// For the purposes of this class, Size.Empty should be treated as if it were Size(0,0). /// private static object CoerceSize(DependencyObject d, object value) { if (((Size)value).IsEmpty) { return new Size(0,0); } else { return value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //-------------------------------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: Impelementation of ArcSegment // // History: // //------------------------------------------------------------------------------------------------- using MS.Internal; using MS.Internal.PresentationCore; using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Text; using System.Security; using System.Security.Permissions; using System.Text.RegularExpressions; using System.Windows.Media; using System.Windows; using System.Windows.Media.Composition; using System.Windows.Media.Animation; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// ArcSegment /// public sealed partial class ArcSegment : PathSegment { #region Constructors ////// /// public ArcSegment() { } ////// /// public ArcSegment( Point point, Size size, double rotationAngle, bool isLargeArc, SweepDirection sweepDirection, bool isStroked) { Size = size; RotationAngle = rotationAngle; IsLargeArc = isLargeArc; SweepDirection = sweepDirection; Point = point; IsStroked = isStroked; } #endregion #region AddToFigure ////// Critical: This code calls into MilUtility_ArcToBezier which has an unmanaged code elevation /// TreatAsSafe: Adding a figure is considered safe in partial trust. /// [SecurityCritical,SecurityTreatAsSafe] internal override void AddToFigure( Matrix matrix, // The transformation matrid PathFigure figure, // The figure to add to ref Point current) // In: Segment start point, Out: Segment endpoint, neither transformed { Point endPoint = Point; if (matrix.IsIdentity) { figure.Segments.Add(this); } else { // The arc segment is approximated by up to 4 Bezier segments unsafe { int count; Point* points = stackalloc Point[12]; Size size = Size; Double rotation = RotationAngle; MilMatrix3x2D mat3X2 = CompositionResourceManager.MatrixToMilMatrix3x2D(ref matrix); Composition.MilCoreApi.MilUtility_ArcToBezier( current, // =start point size, rotation, IsLargeArc, SweepDirection, endPoint, &mat3X2, points, out count); // = number of Bezier segments Invariant.Assert(count <= 4); // To ensure no buffer overflows count = Math.Min(count, 4); bool isStroked = IsStroked; bool isSmoothJoin = IsSmoothJoin; // Add the segments if (count > 0) { for (int i = 0; i < count; i++) { figure.Segments.Add(new BezierSegment( points[3*i], points[3*i + 1], points[3*i + 2], isStroked, (i < count - 1) || isSmoothJoin)); // Smooth join between arc pieces } } else if (count == 0) { figure.Segments.Add(new LineSegment(points[0], isStroked, isSmoothJoin)); } } // Update the last point current = endPoint; } } #endregion ////// SerializeData - Serialize the contents of this Segment to the provided context. /// internal override void SerializeData(StreamGeometryContext ctx) { ctx.ArcTo(Point, Size, RotationAngle, IsLargeArc, SweepDirection, IsStroked, IsSmoothJoin); } internal override bool IsCurved() { return true; } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// internal override string ConvertToString(string format, IFormatProvider provider) { // Helper to get the numeric list separator for a given culture. char separator = MS.Internal.TokenizerHelper.GetNumericListSeparator(provider); return String.Format(provider, "A{1:" + format + "}{0}{2:" + format + "}{0}{3}{0}{4}{0}{5:" + format + "}", separator, Size, RotationAngle, IsLargeArc ? "1" : "0", SweepDirection == SweepDirection.Clockwise ? "1" : "0", Point); } // // This property // 1. Finds the correct initial size for the _effectiveValues store on the current DependencyObject // 2. This is a performance optimization // internal override int EffectiveValuesInitialSize { get { return 6; } } ////// For the purposes of this class, Size.Empty should be treated as if it were Size(0,0). /// private static object CoerceSize(DependencyObject d, object value) { if (((Size)value).IsEmpty) { return new Size(0,0); } else { return value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
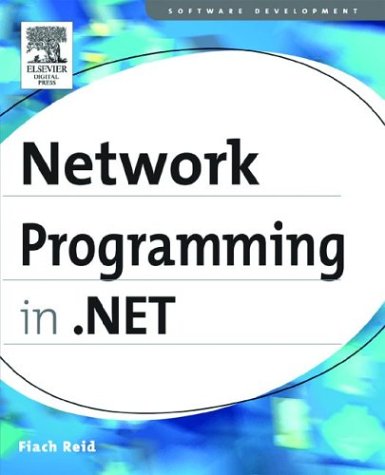
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UIElementAutomationPeer.cs
- BinaryMessageFormatter.cs
- RequestCachingSection.cs
- DateTimeOffsetStorage.cs
- SQLCharsStorage.cs
- Int64Storage.cs
- _CommandStream.cs
- EventProxy.cs
- HashMembershipCondition.cs
- HttpProtocolReflector.cs
- UdpDiscoveryMessageFilter.cs
- KeyInfo.cs
- TextServicesContext.cs
- DependencyPropertyValueSerializer.cs
- DecoderReplacementFallback.cs
- codemethodreferenceexpression.cs
- ApplicationHost.cs
- WorkflowValidationFailedException.cs
- UpdatePanelTriggerCollection.cs
- PanelStyle.cs
- ApplicationContext.cs
- FrameworkTextComposition.cs
- ListItemConverter.cs
- MainMenu.cs
- LoadRetryConstantStrategy.cs
- ContextMarshalException.cs
- SecUtil.cs
- DbConnectionPoolIdentity.cs
- PreservationFileWriter.cs
- Stack.cs
- CommonXSendMessage.cs
- CompositionAdorner.cs
- LambdaCompiler.Generated.cs
- xml.cs
- HttpApplicationFactory.cs
- AssociationType.cs
- XPathScanner.cs
- SqlProfileProvider.cs
- DeflateStream.cs
- Avt.cs
- DataGridViewSortCompareEventArgs.cs
- AppSettingsExpressionBuilder.cs
- SystemTcpConnection.cs
- UnicodeEncoding.cs
- OperationAbortedException.cs
- AssemblyBuilder.cs
- Transactions.cs
- XamlFilter.cs
- ContextStaticAttribute.cs
- ListViewItem.cs
- BamlBinaryWriter.cs
- Vector3DCollectionValueSerializer.cs
- NullExtension.cs
- WebPartMinimizeVerb.cs
- RelationshipEndCollection.cs
- HideDisabledControlAdapter.cs
- ListViewUpdateEventArgs.cs
- SqlProfileProvider.cs
- PageOrientation.cs
- TdsParserSafeHandles.cs
- ReachDocumentReferenceCollectionSerializerAsync.cs
- PolicyFactory.cs
- InputReport.cs
- DesignerDataParameter.cs
- LinkUtilities.cs
- WebPartTransformerAttribute.cs
- SecureStringHasher.cs
- TerminateSequenceResponse.cs
- SqlDataSourceConfigureSortForm.cs
- ApplicationSecurityInfo.cs
- StreamUpdate.cs
- FrameworkElement.cs
- NonParentingControl.cs
- InfoCardSymmetricCrypto.cs
- BooleanConverter.cs
- SubMenuStyleCollection.cs
- ExpressionVisitor.cs
- OrElse.cs
- Token.cs
- Win32NamedPipes.cs
- LogicalExpressionTypeConverter.cs
- AssociationSetMetadata.cs
- CustomErrorsSectionWrapper.cs
- CodeAccessPermission.cs
- ListViewInsertEventArgs.cs
- FunctionCommandText.cs
- StorageScalarPropertyMapping.cs
- ArgumentOutOfRangeException.cs
- PerfCounterSection.cs
- NavigationCommands.cs
- GeometryCombineModeValidation.cs
- CodeDefaultValueExpression.cs
- XsltInput.cs
- RecordConverter.cs
- loginstatus.cs
- _AcceptOverlappedAsyncResult.cs
- GetReadStreamResult.cs
- HtmlTitle.cs
- ControlCollection.cs
- FileDataSourceCache.cs