Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Media / Animation / PointAnimationUsingPath.cs / 1 / PointAnimationUsingPath.cs
//------------------------------------------------------------------------------ //// Copyright (C) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using MS.Internal; using System; using System.IO; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Reflection; using System.Runtime.InteropServices; using System.Windows; using System.Windows.Media; using System.Windows.Markup; using System.Windows.Media.Animation; using System.Windows.Media.Composition; namespace System.Windows.Media.Animation { ////// This animation can be used inside of a MatrixAnimationCollection to move /// a visual object along a path. /// public class PointAnimationUsingPath : PointAnimationBase { #region Data private bool _isValid; ////// If IsCumulative is set to true, this value represents the value that /// is accumulated with each repeat. It is the end value of the path /// output value for the path. /// private Vector _accumulatingVector = new Vector(); #endregion #region Constructors ////// Creates a new PathPointAnimation class. /// ////// There is no default PathGeometry so the user must specify one. /// public PointAnimationUsingPath() : base() { } #endregion #region Public ////// PathGeometry Property /// public static readonly DependencyProperty PathGeometryProperty = DependencyProperty.Register( "PathGeometry", typeof(PathGeometry), typeof(PointAnimationUsingPath), new PropertyMetadata( (PathGeometry)null)); ////// This geometry specifies the path. /// public PathGeometry PathGeometry { get { return (PathGeometry)GetValue(PathGeometryProperty); } set { SetValue(PathGeometryProperty, value); } } #endregion #region Freezable ////// Creates a copy of this PointAnimationUsingPath. /// ///The copy. public new PointAnimationUsingPath Clone() { return (PointAnimationUsingPath)base.Clone(); } ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new PointAnimationUsingPath(); } ////// Implementation of protected override void OnChanged() { _isValid = false; base.OnChanged(); } #endregion #region PointAnimationBase ///Freezable.OnChanged . ////// Calculates the value this animation believes should be the current value for the property. /// /// /// This value is the suggested origin value provided to the animation /// to be used if the animation does not have its own concept of a /// start value. If this animation is the first in a composition chain /// this value will be the snapshot value if one is available or the /// base property value if it is not; otherise this value will be the /// value returned by the previous animation in the chain with an /// animationClock that is not Stopped. /// /// /// This value is the suggested destination value provided to the animation /// to be used if the animation does not have its own concept of an /// end value. This value will be the base value if the animation is /// in the first composition layer of animations on a property; /// otherwise this value will be the output value from the previous /// composition layer of animations for the property. /// /// /// This is the animationClock which can generate the CurrentTime or /// CurrentProgress value to be used by the animation to generate its /// output value. /// ////// The value this animation believes should be the current value for the property. /// protected override Point GetCurrentValueCore(Point defaultOriginValue, Point defaultDestinationValue, AnimationClock animationClock) { Debug.Assert(animationClock.CurrentState != ClockState.Stopped); PathGeometry pathGeometry = PathGeometry; if (pathGeometry == null) { return defaultDestinationValue; } if (!_isValid) { Validate(); } Point pathPoint; Point pathTangent; pathGeometry.GetPointAtFractionLength(animationClock.CurrentProgress.Value, out pathPoint, out pathTangent); double currentRepeat = (double)(animationClock.CurrentIteration - 1); if ( IsCumulative && currentRepeat > 0) { pathPoint = pathPoint + (_accumulatingVector * currentRepeat); } if (IsAdditive) { return defaultOriginValue + (Vector)pathPoint; } else { return pathPoint; } } ////// IsAdditive /// public bool IsAdditive { get { return (bool)GetValue(IsAdditiveProperty); } set { SetValue(IsAdditiveProperty, value); } } ////// IsCumulative /// public bool IsCumulative { get { return (bool)GetValue(IsCumulativeProperty); } set { SetValue(IsCumulativeProperty, value); } } #endregion #region Private Methods private void Validate() { Debug.Assert(!_isValid); if (IsCumulative) { Point startPoint; Point startTangent; Point endPoint; Point endTangent; PathGeometry pathGeometry = PathGeometry; // Get values at the beginning of the path. pathGeometry.GetPointAtFractionLength(0.0, out startPoint, out startTangent); // Get values at the end of the path. pathGeometry.GetPointAtFractionLength(1.0, out endPoint, out endTangent); _accumulatingVector.X = endPoint.X - startPoint.X; _accumulatingVector.Y = endPoint.Y - startPoint.Y; } _isValid = true; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ //// Copyright (C) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using MS.Internal; using System; using System.IO; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Reflection; using System.Runtime.InteropServices; using System.Windows; using System.Windows.Media; using System.Windows.Markup; using System.Windows.Media.Animation; using System.Windows.Media.Composition; namespace System.Windows.Media.Animation { ////// This animation can be used inside of a MatrixAnimationCollection to move /// a visual object along a path. /// public class PointAnimationUsingPath : PointAnimationBase { #region Data private bool _isValid; ////// If IsCumulative is set to true, this value represents the value that /// is accumulated with each repeat. It is the end value of the path /// output value for the path. /// private Vector _accumulatingVector = new Vector(); #endregion #region Constructors ////// Creates a new PathPointAnimation class. /// ////// There is no default PathGeometry so the user must specify one. /// public PointAnimationUsingPath() : base() { } #endregion #region Public ////// PathGeometry Property /// public static readonly DependencyProperty PathGeometryProperty = DependencyProperty.Register( "PathGeometry", typeof(PathGeometry), typeof(PointAnimationUsingPath), new PropertyMetadata( (PathGeometry)null)); ////// This geometry specifies the path. /// public PathGeometry PathGeometry { get { return (PathGeometry)GetValue(PathGeometryProperty); } set { SetValue(PathGeometryProperty, value); } } #endregion #region Freezable ////// Creates a copy of this PointAnimationUsingPath. /// ///The copy. public new PointAnimationUsingPath Clone() { return (PointAnimationUsingPath)base.Clone(); } ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new PointAnimationUsingPath(); } ////// Implementation of protected override void OnChanged() { _isValid = false; base.OnChanged(); } #endregion #region PointAnimationBase ///Freezable.OnChanged . ////// Calculates the value this animation believes should be the current value for the property. /// /// /// This value is the suggested origin value provided to the animation /// to be used if the animation does not have its own concept of a /// start value. If this animation is the first in a composition chain /// this value will be the snapshot value if one is available or the /// base property value if it is not; otherise this value will be the /// value returned by the previous animation in the chain with an /// animationClock that is not Stopped. /// /// /// This value is the suggested destination value provided to the animation /// to be used if the animation does not have its own concept of an /// end value. This value will be the base value if the animation is /// in the first composition layer of animations on a property; /// otherwise this value will be the output value from the previous /// composition layer of animations for the property. /// /// /// This is the animationClock which can generate the CurrentTime or /// CurrentProgress value to be used by the animation to generate its /// output value. /// ////// The value this animation believes should be the current value for the property. /// protected override Point GetCurrentValueCore(Point defaultOriginValue, Point defaultDestinationValue, AnimationClock animationClock) { Debug.Assert(animationClock.CurrentState != ClockState.Stopped); PathGeometry pathGeometry = PathGeometry; if (pathGeometry == null) { return defaultDestinationValue; } if (!_isValid) { Validate(); } Point pathPoint; Point pathTangent; pathGeometry.GetPointAtFractionLength(animationClock.CurrentProgress.Value, out pathPoint, out pathTangent); double currentRepeat = (double)(animationClock.CurrentIteration - 1); if ( IsCumulative && currentRepeat > 0) { pathPoint = pathPoint + (_accumulatingVector * currentRepeat); } if (IsAdditive) { return defaultOriginValue + (Vector)pathPoint; } else { return pathPoint; } } ////// IsAdditive /// public bool IsAdditive { get { return (bool)GetValue(IsAdditiveProperty); } set { SetValue(IsAdditiveProperty, value); } } ////// IsCumulative /// public bool IsCumulative { get { return (bool)GetValue(IsCumulativeProperty); } set { SetValue(IsCumulativeProperty, value); } } #endregion #region Private Methods private void Validate() { Debug.Assert(!_isValid); if (IsCumulative) { Point startPoint; Point startTangent; Point endPoint; Point endTangent; PathGeometry pathGeometry = PathGeometry; // Get values at the beginning of the path. pathGeometry.GetPointAtFractionLength(0.0, out startPoint, out startTangent); // Get values at the end of the path. pathGeometry.GetPointAtFractionLength(1.0, out endPoint, out endTangent); _accumulatingVector.X = endPoint.X - startPoint.X; _accumulatingVector.Y = endPoint.Y - startPoint.Y; } _isValid = true; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
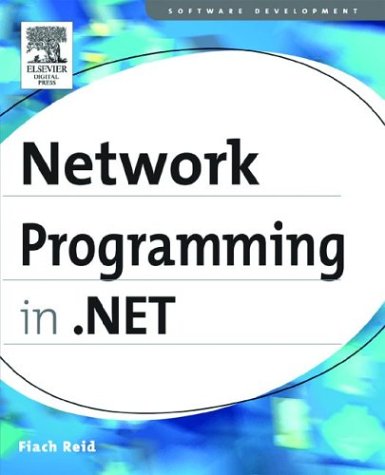
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GradientStopCollection.cs
- PrefixHandle.cs
- ManualResetEvent.cs
- InstanceKeyCollisionException.cs
- EditBehavior.cs
- DynamicDataRouteHandler.cs
- Fonts.cs
- OutputScopeManager.cs
- AsyncResult.cs
- ClusterRegistryConfigurationProvider.cs
- Resources.Designer.cs
- Margins.cs
- ProfileService.cs
- StringValidator.cs
- OleDbTransaction.cs
- X509Extension.cs
- Point3DConverter.cs
- StateDesigner.LayoutSelectionGlyph.cs
- FunctionQuery.cs
- TablePatternIdentifiers.cs
- CollaborationHelperFunctions.cs
- KeyValuePairs.cs
- HTMLTagNameToTypeMapper.cs
- CfgRule.cs
- TrackingProfileDeserializationException.cs
- XdrBuilder.cs
- SiteMapSection.cs
- LambdaCompiler.Generated.cs
- HtmlSelect.cs
- ProcessProtocolHandler.cs
- AutomationFocusChangedEventArgs.cs
- StringValidator.cs
- GestureRecognitionResult.cs
- RequestQueryParser.cs
- SemanticResultValue.cs
- IListConverters.cs
- Completion.cs
- ContextMenuService.cs
- RoutedEventConverter.cs
- VisualSerializer.cs
- _NegotiateClient.cs
- OuterGlowBitmapEffect.cs
- FindCriteria.cs
- UserControlBuildProvider.cs
- InvalidOperationException.cs
- ResourceReferenceExpressionConverter.cs
- SiteMapNodeItemEventArgs.cs
- SchemaSetCompiler.cs
- ConversionHelper.cs
- Int32AnimationBase.cs
- QueuePathEditor.cs
- Stack.cs
- WorkflowTraceTransfer.cs
- PlainXmlWriter.cs
- WebPartVerb.cs
- TraceRecord.cs
- documentsequencetextview.cs
- RegisteredArrayDeclaration.cs
- ToolStripStatusLabel.cs
- EncodingInfo.cs
- TargetPerspective.cs
- SmiContextFactory.cs
- AutomationAttributeInfo.cs
- X509Chain.cs
- WebEvents.cs
- StringWriter.cs
- SecurityHelper.cs
- DataServiceBuildProvider.cs
- PageThemeCodeDomTreeGenerator.cs
- IsolatedStorageException.cs
- LayoutExceptionEventArgs.cs
- EventBookmark.cs
- SafeCertificateStore.cs
- FileReader.cs
- CompositeControl.cs
- CompoundFileStreamReference.cs
- ZoneButton.cs
- MergeEnumerator.cs
- X509Utils.cs
- Selection.cs
- NumberFunctions.cs
- ProtectedConfigurationProviderCollection.cs
- TypeElementCollection.cs
- DataGridViewColumnCollection.cs
- ParameterSubsegment.cs
- JsonServiceDocumentSerializer.cs
- ThreadSafeMessageFilterTable.cs
- GridViewUpdateEventArgs.cs
- EntityDataSourceSelectedEventArgs.cs
- CellConstant.cs
- UiaCoreProviderApi.cs
- StringInfo.cs
- RegexTree.cs
- XmlNamedNodeMap.cs
- SafeCryptHandles.cs
- HttpHandler.cs
- Misc.cs
- ToolStripPanelSelectionBehavior.cs
- EllipseGeometry.cs
- DurableInstanceManager.cs