Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataWebControlsDesign / System / Data / WebControls / Design / Util / DesignerForm.cs / 1 / DesignerForm.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //----------------------------------------------------------------------------- using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Design; using System.Diagnostics; using System.Drawing; using System.Globalization; using System.Windows.Forms; using System.Windows.Forms.Design; namespace System.Web.UI.Design.WebControls.Util { ////// Represents a form used by a designer. /// internal abstract class DesignerForm : Form { private const int SC_CONTEXTHELP = 0xF180; private const int WM_SYSCOMMAND = 0x0112; private IServiceProvider _serviceProvider; private bool _firstActivate; #if DEBUG private bool _formInitialized; #endif ////// Creates a new DesignerForm with a given service provider. /// protected DesignerForm(IServiceProvider serviceProvider) { Debug.Assert(serviceProvider != null); _serviceProvider = serviceProvider; _firstActivate = true; } ////// The service provider for the form. /// protected internal IServiceProvider ServiceProvider { get { return _serviceProvider; } } ////// Frees up resources. /// protected override void Dispose(bool disposing) { if (disposing) { _serviceProvider = null; } base.Dispose(disposing); } protected void InitializeForm() { Font dialogFont = UIHelper.GetDialogFont(ServiceProvider); if (dialogFont != null) { Font = dialogFont; } // Set RightToLeft mode based on resource file string rtlText = Strings.RTL; if (!String.Equals(rtlText, "RTL_False", StringComparison.Ordinal)) { RightToLeft = RightToLeft.Yes; RightToLeftLayout = true; } #pragma warning disable 618 AutoScaleBaseSize = new System.Drawing.Size(5, 14); #pragma warning restore 618 HelpButton = true; MinimizeBox = false; MaximizeBox = false; ShowIcon = false; ShowInTaskbar = false; FormBorderStyle = FormBorderStyle.Sizable; SizeGripStyle = System.Windows.Forms.SizeGripStyle.Show; StartPosition = System.Windows.Forms.FormStartPosition.CenterParent; MinimumSize = Size; #if DEBUG _formInitialized = true; #endif } ////// Gets a service of the desired entitySetNameItem. Returns null if the service does not exist or there is no service provider. /// protected override object GetService(Type serviceType) { if (_serviceProvider != null) { return _serviceProvider.GetService(serviceType); } return null; } protected override void OnActivated(EventArgs e) { base.OnActivated(e); if (_firstActivate) { _firstActivate = false; OnInitialActivated(e); } } ////// Returns the help topic for the form. Consult with your UE contact on /// what the appropriate help topic is for your dialog. /// protected abstract string HelpTopic { get; } protected sealed override void OnHelpRequested(HelpEventArgs hevent) { ShowHelp(); hevent.Handled = true; } ////// Raised upon first activation of the form. /// /// protected virtual void OnInitialActivated(EventArgs e) { #if DEBUG Debug.Assert(_formInitialized, "All classes deriving from DesignerForm must call InitializeForm() from within InitializeComponent()."); #endif } ////// Launches the help for this form. /// private void ShowHelp() { if (ServiceProvider != null) { IHelpService helpService = (IHelpService)ServiceProvider.GetService(typeof(IHelpService)); if (helpService != null) { helpService.ShowHelpFromKeyword(HelpTopic); } } } ////// Overridden to reroute the context-help button to our own handler. /// protected override void WndProc(ref Message m) { if ((m.Msg == WM_SYSCOMMAND) && ((int)m.WParam == SC_CONTEXTHELP)) { ShowHelp(); return; } else { base.WndProc(ref m); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //----------------------------------------------------------------------------- using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Design; using System.Diagnostics; using System.Drawing; using System.Globalization; using System.Windows.Forms; using System.Windows.Forms.Design; namespace System.Web.UI.Design.WebControls.Util { ////// Represents a form used by a designer. /// internal abstract class DesignerForm : Form { private const int SC_CONTEXTHELP = 0xF180; private const int WM_SYSCOMMAND = 0x0112; private IServiceProvider _serviceProvider; private bool _firstActivate; #if DEBUG private bool _formInitialized; #endif ////// Creates a new DesignerForm with a given service provider. /// protected DesignerForm(IServiceProvider serviceProvider) { Debug.Assert(serviceProvider != null); _serviceProvider = serviceProvider; _firstActivate = true; } ////// The service provider for the form. /// protected internal IServiceProvider ServiceProvider { get { return _serviceProvider; } } ////// Frees up resources. /// protected override void Dispose(bool disposing) { if (disposing) { _serviceProvider = null; } base.Dispose(disposing); } protected void InitializeForm() { Font dialogFont = UIHelper.GetDialogFont(ServiceProvider); if (dialogFont != null) { Font = dialogFont; } // Set RightToLeft mode based on resource file string rtlText = Strings.RTL; if (!String.Equals(rtlText, "RTL_False", StringComparison.Ordinal)) { RightToLeft = RightToLeft.Yes; RightToLeftLayout = true; } #pragma warning disable 618 AutoScaleBaseSize = new System.Drawing.Size(5, 14); #pragma warning restore 618 HelpButton = true; MinimizeBox = false; MaximizeBox = false; ShowIcon = false; ShowInTaskbar = false; FormBorderStyle = FormBorderStyle.Sizable; SizeGripStyle = System.Windows.Forms.SizeGripStyle.Show; StartPosition = System.Windows.Forms.FormStartPosition.CenterParent; MinimumSize = Size; #if DEBUG _formInitialized = true; #endif } ////// Gets a service of the desired entitySetNameItem. Returns null if the service does not exist or there is no service provider. /// protected override object GetService(Type serviceType) { if (_serviceProvider != null) { return _serviceProvider.GetService(serviceType); } return null; } protected override void OnActivated(EventArgs e) { base.OnActivated(e); if (_firstActivate) { _firstActivate = false; OnInitialActivated(e); } } ////// Returns the help topic for the form. Consult with your UE contact on /// what the appropriate help topic is for your dialog. /// protected abstract string HelpTopic { get; } protected sealed override void OnHelpRequested(HelpEventArgs hevent) { ShowHelp(); hevent.Handled = true; } ////// Raised upon first activation of the form. /// /// protected virtual void OnInitialActivated(EventArgs e) { #if DEBUG Debug.Assert(_formInitialized, "All classes deriving from DesignerForm must call InitializeForm() from within InitializeComponent()."); #endif } ////// Launches the help for this form. /// private void ShowHelp() { if (ServiceProvider != null) { IHelpService helpService = (IHelpService)ServiceProvider.GetService(typeof(IHelpService)); if (helpService != null) { helpService.ShowHelpFromKeyword(HelpTopic); } } } ////// Overridden to reroute the context-help button to our own handler. /// protected override void WndProc(ref Message m) { if ((m.Msg == WM_SYSCOMMAND) && ((int)m.WParam == SC_CONTEXTHELP)) { ShowHelp(); return; } else { base.WndProc(ref m); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
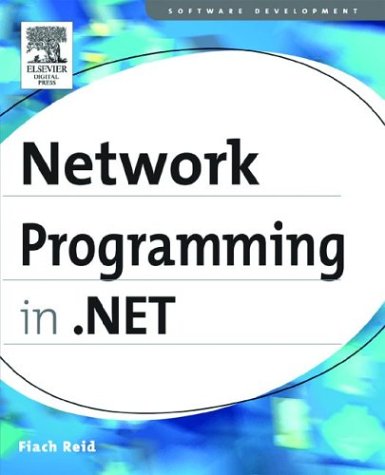
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextServicesManager.cs
- CodeCatchClauseCollection.cs
- CompilationPass2TaskInternal.cs
- ListBox.cs
- SplitterCancelEvent.cs
- EncoderParameters.cs
- RoutedCommand.cs
- HttpConfigurationSystem.cs
- DataSourceCacheDurationConverter.cs
- PreProcessor.cs
- Simplifier.cs
- HuffModule.cs
- CustomPopupPlacement.cs
- MemberInfoSerializationHolder.cs
- ObjectManager.cs
- ExeConfigurationFileMap.cs
- SrgsElement.cs
- XmlSortKeyAccumulator.cs
- EntityModelBuildProvider.cs
- ObjectStateEntryBaseUpdatableDataRecord.cs
- _Semaphore.cs
- SqlFormatter.cs
- FlowDocumentFormatter.cs
- PrintDialogException.cs
- ValidationErrorEventArgs.cs
- ActivityBindForm.Designer.cs
- WebSysDisplayNameAttribute.cs
- _ShellExpression.cs
- OdbcCommand.cs
- Int32KeyFrameCollection.cs
- RawMouseInputReport.cs
- KeyManager.cs
- SafeWaitHandle.cs
- FilterEventArgs.cs
- RequestDescription.cs
- Bits.cs
- Message.cs
- Knowncolors.cs
- PackUriHelper.cs
- ReadOnlyPermissionSet.cs
- SubqueryRules.cs
- NonVisualControlAttribute.cs
- OciEnlistContext.cs
- DataGridViewRowConverter.cs
- CultureInfoConverter.cs
- HttpChannelHelper.cs
- Privilege.cs
- DataTableReader.cs
- QuaternionKeyFrameCollection.cs
- ViewSimplifier.cs
- AuthenticationServiceManager.cs
- ContentType.cs
- PropertyGridCommands.cs
- CharacterHit.cs
- RegexReplacement.cs
- DataGridPreparingCellForEditEventArgs.cs
- GAC.cs
- XmlNodeComparer.cs
- StringToken.cs
- Shape.cs
- GPPOINTF.cs
- AnimatedTypeHelpers.cs
- InputReport.cs
- SHA256.cs
- ChildrenQuery.cs
- ValuePatternIdentifiers.cs
- DesignRelationCollection.cs
- ConfigXmlElement.cs
- Themes.cs
- SortQueryOperator.cs
- UnrecognizedAssertionsBindingElement.cs
- ConnectionInterfaceCollection.cs
- UDPClient.cs
- SecureStringHasher.cs
- Number.cs
- IpcManager.cs
- ProfileBuildProvider.cs
- Wildcard.cs
- NodeCounter.cs
- x509store.cs
- MatrixTransform3D.cs
- XPathNodeIterator.cs
- CorrelationKey.cs
- RegularExpressionValidator.cs
- WSDualHttpSecurity.cs
- OAVariantLib.cs
- ControlUtil.cs
- SqlBuilder.cs
- InputDevice.cs
- DataDocumentXPathNavigator.cs
- LineMetrics.cs
- UnicodeEncoding.cs
- ByteAnimationUsingKeyFrames.cs
- SerializationException.cs
- Camera.cs
- TextMetrics.cs
- RegexGroup.cs
- DataViewListener.cs
- ObjectDataSourceEventArgs.cs
- Stopwatch.cs