Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Input / TextServicesCompartment.cs / 1 / TextServicesCompartment.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Manages Text Services Compartment. // // History: // 07/30/2003 : [....] - Ported from .net tree. // //--------------------------------------------------------------------------- using System; using System.Runtime.InteropServices; using System.Windows.Threading; using System.Security; using System.Security.Permissions; using System.Diagnostics; using System.Collections; using MS.Utility; using MS.Win32; using MS.Internal; namespace System.Windows.Input { //----------------------------------------------------- // // TextServicesCompartment class // //----------------------------------------------------- internal class TextServicesCompartment { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- ////// Critical - directly calls unmanaged code based on guid /// [SecurityCritical] internal TextServicesCompartment(Guid guid, UnsafeNativeMethods.ITfCompartmentMgr compartmentmgr) { _guid = guid; _compartmentmgr = new SecurityCriticalData(compartmentmgr); _cookie = UnsafeNativeMethods.TF_INVALID_COOKIE; } //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public Methods #endregion Public Methods //----------------------------------------------------- // // Public Operators // //------------------------------------------------------ //----------------------------------------------------- // // Public Properties // //----------------------------------------------------- //----------------------------------------------------- // // Public Events // //------------------------------------------------------ //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- #region Internal Methods /// /// Advise the notify sink of the compartment update. /// ////// Critical - wires up multiple unmanaged objects together /// [SecurityCritical] internal void AdviseNotifySink(UnsafeNativeMethods.ITfCompartmentEventSink sink) { Debug.Assert(_cookie == UnsafeNativeMethods.TF_INVALID_COOKIE, "cookie is already set."); UnsafeNativeMethods.ITfCompartment compartment = GetITfCompartment(); if (compartment == null) return; UnsafeNativeMethods.ITfSource source = compartment as UnsafeNativeMethods.ITfSource; // workaround because I can't pass a ref to a readonly constant Guid guid = UnsafeNativeMethods.IID_ITfCompartmentEventSink; source.AdviseSink(ref guid, sink, out _cookie); Marshal.ReleaseComObject(compartment); Marshal.ReleaseComObject(source); } ////// Unadvise the notify sink of the compartment update. /// ////// Critical - wires up multiple unmanaged objects together /// [SecurityCritical] internal void UnadviseNotifySink() { Debug.Assert(_cookie != UnsafeNativeMethods.TF_INVALID_COOKIE, "cookie is not set."); UnsafeNativeMethods.ITfCompartment compartment = GetITfCompartment(); if (compartment == null) return; UnsafeNativeMethods.ITfSource source = compartment as UnsafeNativeMethods.ITfSource; source.UnadviseSink(_cookie); _cookie = UnsafeNativeMethods.TF_INVALID_COOKIE; Marshal.ReleaseComObject(compartment); Marshal.ReleaseComObject(source); } ////// Retrieve ITfCompartment /// ////// Critical - returns critical resource /// [SecurityCritical] internal UnsafeNativeMethods.ITfCompartment GetITfCompartment() { UnsafeNativeMethods.ITfCompartment itfcompartment; _compartmentmgr.Value.GetCompartment(ref _guid, out itfcompartment); return itfcompartment; } #endregion Internal methods //------------------------------------------------------ // // Internal Properties // //----------------------------------------------------- #region Internal Properties ////// Cast the compartment variant to bool. /// internal bool BooleanValue { get { object obj = Value; if (obj == null) return false; if ((int)obj != 0) return true; return false; } set { Value = value ? 1 : 0; } } ////// Cast the compartment variant to int. /// internal int IntValue { get { object obj = Value; if (obj == null) return 0; return (int)obj; } set { Value = value; } } ////// Get the compartment variant. /// ////// Critical - access unmanaged code /// TreatAsSafe - returns "safe" variant based value from the store /// internal object Value { [SecurityCritical, SecurityTreatAsSafe] get { UnsafeNativeMethods.ITfCompartment compartment = GetITfCompartment(); if (compartment == null) return null; object obj; compartment.GetValue(out obj); Marshal.ReleaseComObject(compartment); return obj; } [SecurityCritical, SecurityTreatAsSafe] set { UnsafeNativeMethods.ITfCompartment compartment = GetITfCompartment(); if (compartment == null) return; compartment.SetValue(0 /* clientid */, ref value); Marshal.ReleaseComObject(compartment); } } #endregion Internal Properties //----------------------------------------------------- // // Private Methods // //----------------------------------------------------- //------------------------------------------------------ // // Private Fields // //----------------------------------------------------- #region Private Fields ////// Critical: UnsafeNativeMethods.ITfCompartmentMgr has methods with SuppressUnmanagedCodeSecurity. /// private readonly SecurityCriticalData_compartmentmgr; private Guid _guid; private int _cookie; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
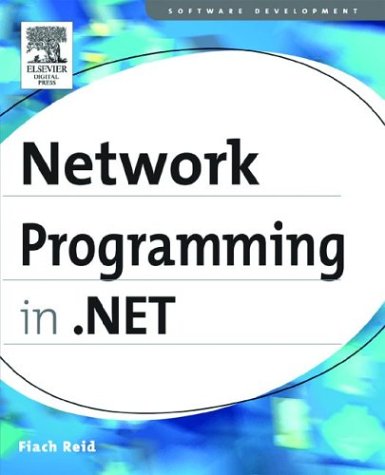
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XslTransform.cs
- MimeMapping.cs
- SoapIncludeAttribute.cs
- TextContainer.cs
- SqlCharStream.cs
- GridViewCancelEditEventArgs.cs
- SQLBytesStorage.cs
- RectangleF.cs
- BitmapEffectState.cs
- GroupItem.cs
- IPGlobalProperties.cs
- Pair.cs
- ScriptReferenceEventArgs.cs
- ListViewInsertedEventArgs.cs
- ConsoleTraceListener.cs
- ImageField.cs
- BCLDebug.cs
- StretchValidation.cs
- RuleEngine.cs
- WindowsTokenRoleProvider.cs
- LoadGrammarCompletedEventArgs.cs
- BamlLocalizabilityResolver.cs
- ValidationSummary.cs
- TraceHandlerErrorFormatter.cs
- ConstraintManager.cs
- Nullable.cs
- ParenExpr.cs
- CompiledQueryCacheKey.cs
- PropertyItemInternal.cs
- IApplicationTrustManager.cs
- ReferenceEqualityComparer.cs
- DrawingVisual.cs
- SrgsSemanticInterpretationTag.cs
- DistinctQueryOperator.cs
- RequiredFieldValidator.cs
- ObjectDataProvider.cs
- UnauthorizedWebPart.cs
- DescendantOverDescendantQuery.cs
- Border.cs
- ExceptionWrapper.cs
- HttpGetProtocolImporter.cs
- PresentationSource.cs
- TextRange.cs
- ShaderEffect.cs
- EntityKeyElement.cs
- MSAANativeProvider.cs
- Trustee.cs
- UserControl.cs
- XmlTextReaderImplHelpers.cs
- TextRange.cs
- ManagedIStream.cs
- TemplateManager.cs
- WithParamAction.cs
- CompositionTarget.cs
- EasingFunctionBase.cs
- GAC.cs
- mansign.cs
- ClientTargetCollection.cs
- MetadataAssemblyHelper.cs
- OdbcReferenceCollection.cs
- ThreadStaticAttribute.cs
- BinaryUtilClasses.cs
- GrammarBuilderWildcard.cs
- WorkflowMarkupSerializationException.cs
- EventlogProvider.cs
- XPathDocumentBuilder.cs
- RepeaterItem.cs
- Group.cs
- PasswordDeriveBytes.cs
- RelationshipDetailsRow.cs
- Wizard.cs
- _NegotiateClient.cs
- ServiceInfo.cs
- TypedElement.cs
- hwndwrapper.cs
- Attachment.cs
- OletxResourceManager.cs
- FloatUtil.cs
- TextProviderWrapper.cs
- AutomationPatternInfo.cs
- PropertyEmitterBase.cs
- _TLSstream.cs
- CryptographicAttribute.cs
- XmlSiteMapProvider.cs
- UpdateInfo.cs
- WebHttpBinding.cs
- PopupRoot.cs
- MappingMetadataHelper.cs
- TransformGroup.cs
- CompilationLock.cs
- FlowDocumentFormatter.cs
- TreeViewCancelEvent.cs
- Authorization.cs
- EventLogPermission.cs
- ObjectSpanRewriter.cs
- BitmapEffectState.cs
- SqlBuilder.cs
- HttpStaticObjectsCollectionBase.cs
- TrustLevel.cs
- InputProviderSite.cs