Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DLinq / Dlinq / DbConvert.cs / 2 / DbConvert.cs
using System.Data.Common; using System.Data.SqlClient; using System.Linq.Expressions; using System.IO; using System.Reflection; using System.Text; using System.Runtime.Serialization.Formatters.Binary; using System.Linq; using System.Collections.Generic; using System.Collections; using System.Diagnostics.CodeAnalysis; namespace System.Data.Linq { public static class DBConvert { private static Type[] StringArg = new Type[] { typeof(string) }; [SuppressMessage("Microsoft.Design", "CA1004:GenericMethodsShouldProvideTypeParameter", Justification = "[....]: Generic parameters are required for strong-typing of the return type.")] public static T ChangeType(object value) { return (T)ChangeType(value, typeof(T)); } [SuppressMessage("Microsoft.Performance", "CA1800:DoNotCastUnnecessarily", Justification = "[....]: Cast is dependent on node type and casts do not happen unecessarily in a single code path.")] [SuppressMessage("Microsoft.Maintainability", "CA1502:AvoidExcessiveComplexity", Justification = "These issues are related to our use of if-then and case statements for node types, which adds to the complexity count however when reviewed they are easy to navigate and understand.")] public static object ChangeType(object value, Type type) { if (value == null) return null; MethodInfo mi; type = System.Data.Linq.SqlClient.TypeSystem.GetNonNullableType(type); Type oType = value.GetType(); if (type.IsAssignableFrom(value.GetType())) return value; if (type == typeof(Binary)) { if (oType == typeof(byte[])) { return new Binary((byte[])value); } else if (oType == typeof(Guid)) { return new Binary(((Guid)value).ToByteArray()); } else { BinaryFormatter formatter = new BinaryFormatter(); byte[] streamArray; using (MemoryStream stream = new MemoryStream()) { formatter.Serialize(stream, value); streamArray = stream.ToArray(); } return new Binary(streamArray); } } else if (type == typeof(byte[])) { if (oType == typeof(Binary)) { return ((Binary)value).ToArray(); } else if (oType == typeof(Guid)) { return ((Guid)value).ToByteArray(); } else { BinaryFormatter formatter = new BinaryFormatter(); byte[] returnValue; using (MemoryStream stream = new MemoryStream()) { formatter.Serialize(stream, value); returnValue = stream.ToArray(); } return returnValue; } } else if (oType == typeof(byte[])) { if (type == typeof(Guid)) { return new Guid((byte[])value); } else { BinaryFormatter formatter = new BinaryFormatter(); object returnValue; using (MemoryStream stream = new MemoryStream((byte[])value)) { returnValue = ChangeType(formatter.Deserialize(stream), type); } return returnValue; } } else if (oType == typeof(Binary)) { if (type == typeof(Guid)) { return new Guid(((Binary)value).ToArray()); } else { BinaryFormatter formatter = new BinaryFormatter(); using (MemoryStream stream = new MemoryStream(((Binary)value).ToArray(), false)) { return ChangeType(formatter.Deserialize(stream), type); } } } else if (type.IsEnum) { if (oType == typeof(string)) { string text = ((string)value).Trim(); return Enum.Parse(type, text); } else { return Enum.ToObject(type, Convert.ChangeType(value, Enum.GetUnderlyingType(type), Globalization.CultureInfo.InvariantCulture)); } } else if (oType.IsEnum) { if (type == typeof(string)) { return Enum.GetName(oType, value); } else { return Convert.ChangeType(Convert.ChangeType(value, Enum.GetUnderlyingType(oType), Globalization.CultureInfo.InvariantCulture), type, Globalization.CultureInfo.InvariantCulture); } } else if (type == typeof(TimeSpan)) { if (oType == typeof(string)) { return TimeSpan.Parse((string)value); } else if (oType == typeof(DateTime)) { return DateTime.Parse(value.ToString(), Globalization.CultureInfo.InvariantCulture).TimeOfDay; } else if (oType == typeof(DateTimeOffset)) { return DateTimeOffset.Parse(value.ToString(), Globalization.CultureInfo.InvariantCulture).TimeOfDay; } else { return new TimeSpan((long)Convert.ChangeType(value, typeof(long), Globalization.CultureInfo.InvariantCulture)); } } else if (oType == typeof(TimeSpan)) { if (type == typeof(string)) { return value.ToString(); } else if (type == typeof(DateTime)) { DateTime dt = new DateTime(); return dt.Add((TimeSpan)value); } else if (type == typeof(DateTimeOffset)) { DateTimeOffset dto = new DateTimeOffset(); return dto.Add((TimeSpan)value); } else { return Convert.ChangeType(((TimeSpan)value).Ticks, type, Globalization.CultureInfo.InvariantCulture); } } else if (type == typeof(DateTime) && oType == typeof(DateTimeOffset)) { return ((DateTimeOffset)value).DateTime; } else if (type == typeof(DateTimeOffset) && oType == typeof(DateTime)) { return new DateTimeOffset((DateTime)value); } else if (type == typeof(string) && !(typeof(IConvertible).IsAssignableFrom(oType))) { if (oType == typeof(char[])) { return new String((char[])value); } else { return value.ToString(); } } else if (oType == typeof(string)) { if (type == typeof(Guid)) { return new Guid((string)value); } else if (type == typeof(char[])) { return ((String)value).ToCharArray(); } else if (type == typeof(System.Xml.Linq.XDocument) && (string)value == string.Empty) { return new System.Xml.Linq.XDocument(); } else if (!(typeof(IConvertible).IsAssignableFrom(type)) && (mi = type.GetMethod("Parse", BindingFlags.Static | BindingFlags.Public, null, StringArg, null)) != null) { try { return mi.Invoke(null, new object[] { value }); } catch (TargetInvocationException t) { throw t.GetBaseException(); } } else { return Convert.ChangeType(value, type, Globalization.CultureInfo.InvariantCulture); } } else if (type.IsGenericType && type.GetGenericTypeDefinition() == typeof(IQueryable<>) && typeof(IEnumerable<>).MakeGenericType(type.GetGenericArguments()[0]).IsAssignableFrom(oType) ) { return Queryable.AsQueryable((IEnumerable)value); } else { try { return Convert.ChangeType(value, type, Globalization.CultureInfo.InvariantCulture); } catch (InvalidCastException) { throw Error.CouldNotConvert(value.GetType(), type); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System.Data.Common; using System.Data.SqlClient; using System.Linq.Expressions; using System.IO; using System.Reflection; using System.Text; using System.Runtime.Serialization.Formatters.Binary; using System.Linq; using System.Collections.Generic; using System.Collections; using System.Diagnostics.CodeAnalysis; namespace System.Data.Linq { public static class DBConvert { private static Type[] StringArg = new Type[] { typeof(string) }; [SuppressMessage("Microsoft.Design", "CA1004:GenericMethodsShouldProvideTypeParameter", Justification = "[....]: Generic parameters are required for strong-typing of the return type.")] public static T ChangeType (object value) { return (T)ChangeType(value, typeof(T)); } [SuppressMessage("Microsoft.Performance", "CA1800:DoNotCastUnnecessarily", Justification = "[....]: Cast is dependent on node type and casts do not happen unecessarily in a single code path.")] [SuppressMessage("Microsoft.Maintainability", "CA1502:AvoidExcessiveComplexity", Justification = "These issues are related to our use of if-then and case statements for node types, which adds to the complexity count however when reviewed they are easy to navigate and understand.")] public static object ChangeType(object value, Type type) { if (value == null) return null; MethodInfo mi; type = System.Data.Linq.SqlClient.TypeSystem.GetNonNullableType(type); Type oType = value.GetType(); if (type.IsAssignableFrom(value.GetType())) return value; if (type == typeof(Binary)) { if (oType == typeof(byte[])) { return new Binary((byte[])value); } else if (oType == typeof(Guid)) { return new Binary(((Guid)value).ToByteArray()); } else { BinaryFormatter formatter = new BinaryFormatter(); byte[] streamArray; using (MemoryStream stream = new MemoryStream()) { formatter.Serialize(stream, value); streamArray = stream.ToArray(); } return new Binary(streamArray); } } else if (type == typeof(byte[])) { if (oType == typeof(Binary)) { return ((Binary)value).ToArray(); } else if (oType == typeof(Guid)) { return ((Guid)value).ToByteArray(); } else { BinaryFormatter formatter = new BinaryFormatter(); byte[] returnValue; using (MemoryStream stream = new MemoryStream()) { formatter.Serialize(stream, value); returnValue = stream.ToArray(); } return returnValue; } } else if (oType == typeof(byte[])) { if (type == typeof(Guid)) { return new Guid((byte[])value); } else { BinaryFormatter formatter = new BinaryFormatter(); object returnValue; using (MemoryStream stream = new MemoryStream((byte[])value)) { returnValue = ChangeType(formatter.Deserialize(stream), type); } return returnValue; } } else if (oType == typeof(Binary)) { if (type == typeof(Guid)) { return new Guid(((Binary)value).ToArray()); } else { BinaryFormatter formatter = new BinaryFormatter(); using (MemoryStream stream = new MemoryStream(((Binary)value).ToArray(), false)) { return ChangeType(formatter.Deserialize(stream), type); } } } else if (type.IsEnum) { if (oType == typeof(string)) { string text = ((string)value).Trim(); return Enum.Parse(type, text); } else { return Enum.ToObject(type, Convert.ChangeType(value, Enum.GetUnderlyingType(type), Globalization.CultureInfo.InvariantCulture)); } } else if (oType.IsEnum) { if (type == typeof(string)) { return Enum.GetName(oType, value); } else { return Convert.ChangeType(Convert.ChangeType(value, Enum.GetUnderlyingType(oType), Globalization.CultureInfo.InvariantCulture), type, Globalization.CultureInfo.InvariantCulture); } } else if (type == typeof(TimeSpan)) { if (oType == typeof(string)) { return TimeSpan.Parse((string)value); } else if (oType == typeof(DateTime)) { return DateTime.Parse(value.ToString(), Globalization.CultureInfo.InvariantCulture).TimeOfDay; } else if (oType == typeof(DateTimeOffset)) { return DateTimeOffset.Parse(value.ToString(), Globalization.CultureInfo.InvariantCulture).TimeOfDay; } else { return new TimeSpan((long)Convert.ChangeType(value, typeof(long), Globalization.CultureInfo.InvariantCulture)); } } else if (oType == typeof(TimeSpan)) { if (type == typeof(string)) { return value.ToString(); } else if (type == typeof(DateTime)) { DateTime dt = new DateTime(); return dt.Add((TimeSpan)value); } else if (type == typeof(DateTimeOffset)) { DateTimeOffset dto = new DateTimeOffset(); return dto.Add((TimeSpan)value); } else { return Convert.ChangeType(((TimeSpan)value).Ticks, type, Globalization.CultureInfo.InvariantCulture); } } else if (type == typeof(DateTime) && oType == typeof(DateTimeOffset)) { return ((DateTimeOffset)value).DateTime; } else if (type == typeof(DateTimeOffset) && oType == typeof(DateTime)) { return new DateTimeOffset((DateTime)value); } else if (type == typeof(string) && !(typeof(IConvertible).IsAssignableFrom(oType))) { if (oType == typeof(char[])) { return new String((char[])value); } else { return value.ToString(); } } else if (oType == typeof(string)) { if (type == typeof(Guid)) { return new Guid((string)value); } else if (type == typeof(char[])) { return ((String)value).ToCharArray(); } else if (type == typeof(System.Xml.Linq.XDocument) && (string)value == string.Empty) { return new System.Xml.Linq.XDocument(); } else if (!(typeof(IConvertible).IsAssignableFrom(type)) && (mi = type.GetMethod("Parse", BindingFlags.Static | BindingFlags.Public, null, StringArg, null)) != null) { try { return mi.Invoke(null, new object[] { value }); } catch (TargetInvocationException t) { throw t.GetBaseException(); } } else { return Convert.ChangeType(value, type, Globalization.CultureInfo.InvariantCulture); } } else if (type.IsGenericType && type.GetGenericTypeDefinition() == typeof(IQueryable<>) && typeof(IEnumerable<>).MakeGenericType(type.GetGenericArguments()[0]).IsAssignableFrom(oType) ) { return Queryable.AsQueryable((IEnumerable)value); } else { try { return Convert.ChangeType(value, type, Globalization.CultureInfo.InvariantCulture); } catch (InvalidCastException) { throw Error.CouldNotConvert(value.GetType(), type); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
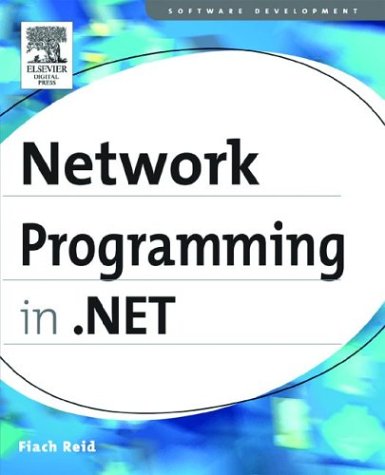
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RecognizedAudio.cs
- LineServicesCallbacks.cs
- ReliableInputConnection.cs
- ContentType.cs
- ConfigurationValues.cs
- PropertyGridView.cs
- BamlRecords.cs
- AssemblyBuilder.cs
- IdlingCommunicationPool.cs
- UmAlQuraCalendar.cs
- EntityKeyElement.cs
- SerialErrors.cs
- ProcessModelInfo.cs
- DataControlFieldHeaderCell.cs
- HtmlInputReset.cs
- BaseCodeDomTreeGenerator.cs
- EntityParameter.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- TabPage.cs
- AstTree.cs
- RangeContentEnumerator.cs
- OracleFactory.cs
- StandardTransformFactory.cs
- StrokeCollection.cs
- diagnosticsswitches.cs
- FlowLayoutSettings.cs
- KeyFrames.cs
- ViewValidator.cs
- ProfessionalColors.cs
- NumericUpDownAccelerationCollection.cs
- CodeFieldReferenceExpression.cs
- SkewTransform.cs
- TabControlAutomationPeer.cs
- DispatchWrapper.cs
- QueueSurrogate.cs
- CollectionTraceRecord.cs
- SqlConnection.cs
- ContextBase.cs
- VisualStyleInformation.cs
- XNodeValidator.cs
- SQLString.cs
- ClonableStack.cs
- PreloadedPackages.cs
- BitConverter.cs
- ProgressBarBrushConverter.cs
- SystemWebCachingSectionGroup.cs
- DeploymentExceptionMapper.cs
- WeakReferenceList.cs
- XmlComplianceUtil.cs
- MenuCommandService.cs
- DeploymentSection.cs
- InputEventArgs.cs
- AttachedPropertyBrowsableWhenAttributePresentAttribute.cs
- CmsInterop.cs
- Configuration.cs
- AsyncDataRequest.cs
- figurelengthconverter.cs
- UriParserTemplates.cs
- GridViewColumnHeader.cs
- CompressionTransform.cs
- DesignerLoader.cs
- BrowserDefinition.cs
- SynthesizerStateChangedEventArgs.cs
- ContextInformation.cs
- PathFigureCollection.cs
- CrossSiteScriptingValidation.cs
- Header.cs
- LassoSelectionBehavior.cs
- CodeMemberField.cs
- DataGridCell.cs
- HandlerWithFactory.cs
- MLangCodePageEncoding.cs
- Logging.cs
- RichTextBoxContextMenu.cs
- AuthorizationPolicyTypeElementCollection.cs
- HtmlHead.cs
- _ShellExpression.cs
- EventManager.cs
- GroupDescription.cs
- SmiGettersStream.cs
- BufferedGraphics.cs
- SafeHandles.cs
- Stylus.cs
- SelectionProviderWrapper.cs
- ProfileModule.cs
- ConsumerConnectionPoint.cs
- TextSelection.cs
- XmlAtomicValue.cs
- MenuItemStyleCollection.cs
- objectresult_tresulttype.cs
- User.cs
- _ScatterGatherBuffers.cs
- WsdlInspector.cs
- ConfigurationStrings.cs
- ListBoxItemAutomationPeer.cs
- ToolStripDropDown.cs
- SqlBooleanizer.cs
- OracleMonthSpan.cs
- Pkcs7Signer.cs
- DbConnectionPoolOptions.cs