Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / fx / src / xsp / System / Web / UI / WebControls / AccessDataSource.cs / 2 / AccessDataSource.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Data; using System.Data.Common; using System.Data.OleDb; using System.Diagnostics; using System.Drawing; using System.Drawing.Design; using System.IO; using System.Security.Permissions; using System.Text; using System.Web.Caching; using System.Web.UI; ////// Allows a user to create a declarative connection to an Access database in a .aspx page. /// [ Designer("System.Web.UI.Design.WebControls.AccessDataSourceDesigner, " + AssemblyRef.SystemDesign), ToolboxBitmap(typeof(AccessDataSource)), WebSysDescription(SR.AccessDataSource_Description), WebSysDisplayName(SR.AccessDataSource_DisplayName) ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class AccessDataSource : SqlDataSource { private const string OleDbProviderName = "System.Data.OleDb"; private FileDataSourceCache _cache; private string _connectionString; private string _dataFile; private string _physicalDataFile; ////// Creates a new instance of AccessDataSource. /// public AccessDataSource() : base() { } ////// Creates a new instance of AccessDataSource with a specified connection string and select command. /// public AccessDataSource(string dataFile, string selectCommand) : base() { if (String.IsNullOrEmpty(dataFile)) { throw new ArgumentNullException("dataFile"); } DataFile = dataFile; SelectCommand = selectCommand; } ////// Specifies the cache settings for this data source. For the cache to /// work, the DataSourceMode must be set to DataSet. /// internal override DataSourceCache Cache { get { if (_cache == null) { _cache = new FileDataSourceCache(); } return _cache; } } ////// Gets the connection string for the AccessDataSource. This property is auto-generated and cannot be set. /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ] public override string ConnectionString { get { if (_connectionString == null) { _connectionString = CreateConnectionString(); } return _connectionString; } set { throw new InvalidOperationException(SR.GetString(SR.AccessDataSource_CannotSetConnectionString)); } } ////// The name of an Access database file. /// This property is not stored in ViewState. /// [ DefaultValue(""), Editor("System.Web.UI.Design.MdbDataFileEditor, " + AssemblyRef.SystemDesign, typeof(UITypeEditor)), UrlProperty(), WebCategory("Data"), WebSysDescription(SR.AccessDataSource_DataFile), ] public string DataFile { get { return (_dataFile == null) ? String.Empty : _dataFile; } set { if (DataFile != value) { _dataFile = value; _connectionString = null; _physicalDataFile = null; RaiseDataSourceChangedEvent(EventArgs.Empty); } } } ////// Gets the file data source cache object. /// private FileDataSourceCache FileDataSourceCache { get { FileDataSourceCache fileCache = Cache as FileDataSourceCache; Debug.Assert(fileCache != null, "Cache object should be a FileDataSourceCache"); return fileCache; } } ////// Gets the Physical path of the data file. /// private string PhysicalDataFile { get { if (_physicalDataFile == null) { _physicalDataFile = GetPhysicalDataFilePath(); } return _physicalDataFile; } } ////// Gets/sets the ADO.net managed provider name. This property is restricted to the OLE DB provider and cannot be set. /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ] public override string ProviderName { get { return OleDbProviderName; } set { throw new InvalidOperationException(SR.GetString(SR.AccessDataSource_CannotSetProvider, ID)); } } ////// A semi-colon delimited string indicating which databases to use for the dependency in the format "database1:table1;database2:table2". /// [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ] public override string SqlCacheDependency { get { throw new NotSupportedException(SR.GetString(SR.AccessDataSource_SqlCacheDependencyNotSupported, ID)); } set { throw new NotSupportedException(SR.GetString(SR.AccessDataSource_SqlCacheDependencyNotSupported, ID)); } } private void AddCacheFileDependency() { FileDataSourceCache.FileDependencies.Clear(); string filename = PhysicalDataFile; if (filename.Length > 0) { FileDataSourceCache.FileDependencies.Add(filename); } } ////// Creates a connection string for an Access database connection. /// The JET provider is automatically used, and the filename, username, password, and /// share mode are are set. /// private string CreateConnectionString() { return "Provider=Microsoft.Jet.OLEDB.4.0; Data Source=" + PhysicalDataFile; } ////// Creates a new AccessDataSourceView. /// protected override SqlDataSourceView CreateDataSourceView(string viewName) { return new AccessDataSourceView(this, viewName, Context); } protected override DbProviderFactory GetDbProviderFactory() { return OleDbFactory.Instance; } ////// Gets the appropriate mapped path for the DataFile. /// private string GetPhysicalDataFilePath() { string filename = DataFile; if (filename.Length == 0) { return null; } if (!System.Web.Util.UrlPath.IsAbsolutePhysicalPath(filename)) { // Root relative path if (DesignMode) { // This exception should never be thrown - the designer always maps paths // before using the runtime control. throw new NotSupportedException(SR.GetString(SR.AccessDataSource_DesignTimeRelativePathsNotSupported, ID)); } filename = Context.Request.MapPath(filename, AppRelativeTemplateSourceDirectory, true); } HttpRuntime.CheckFilePermission(filename, true); // We also need to if (!HttpRuntime.HasPathDiscoveryPermission(filename)) { throw new HttpException(SR.GetString(SR.AccessDataSource_NoPathDiscoveryPermission, HttpRuntime.GetSafePath(filename), ID)); } return filename; } ////// Saves data to the cache. /// internal override void SaveDataToCache(int startRowIndex, int maximumRows, object data, CacheDependency dependency) { AddCacheFileDependency(); base.SaveDataToCache(startRowIndex, maximumRows, data, dependency); } /*internal override void SaveTotalRowCountToCache(int totalRowCount) { AddCacheFileDependency(); base.SaveTotalRowCountToCache(totalRowCount); }*/ } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
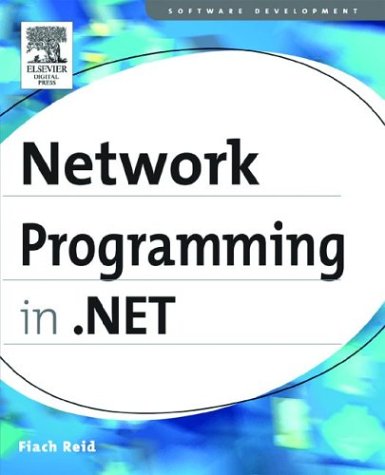
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlReader.cs
- NetDataContractSerializer.cs
- BaseComponentEditor.cs
- PropertyIdentifier.cs
- NameValueConfigurationCollection.cs
- Splitter.cs
- WindowsUpDown.cs
- XPathScanner.cs
- XmlMemberMapping.cs
- IDReferencePropertyAttribute.cs
- SafePEFileHandle.cs
- DtdParser.cs
- XmlIgnoreAttribute.cs
- CryptoKeySecurity.cs
- GenericAuthenticationEventArgs.cs
- BitmapScalingModeValidation.cs
- DataGridItemEventArgs.cs
- SoapExtensionTypeElement.cs
- BuildResult.cs
- FormParameter.cs
- HttpModuleAction.cs
- ClientRolePrincipal.cs
- KnownColorTable.cs
- UriTemplateCompoundPathSegment.cs
- HeaderUtility.cs
- HandleCollector.cs
- ObjectDataSourceFilteringEventArgs.cs
- CustomAttributeBuilder.cs
- FormatException.cs
- RepeatButtonAutomationPeer.cs
- ProcessThreadCollection.cs
- Camera.cs
- TTSEngineProxy.cs
- KeysConverter.cs
- SessionEndingCancelEventArgs.cs
- AnimatedTypeHelpers.cs
- PropertyEmitterBase.cs
- TextHidden.cs
- Graphics.cs
- IPEndPoint.cs
- LineServices.cs
- CompleteWizardStep.cs
- SessionStateItemCollection.cs
- TcpSocketManager.cs
- ConcurrentStack.cs
- CustomErrorsSectionWrapper.cs
- InputLangChangeRequestEvent.cs
- DbTransaction.cs
- MarkupProperty.cs
- Vertex.cs
- TypeConverterMarkupExtension.cs
- InstanceCreationEditor.cs
- DropDownList.cs
- TemplatedControlDesigner.cs
- ServiceContractViewControl.cs
- Pts.cs
- DefaultShape.cs
- TimerElapsedEvenArgs.cs
- Variant.cs
- SwitchLevelAttribute.cs
- OutputCacheProfile.cs
- Util.cs
- PointIndependentAnimationStorage.cs
- _HelperAsyncResults.cs
- _HTTPDateParse.cs
- ObjectDataSourceFilteringEventArgs.cs
- StructuredTypeEmitter.cs
- UnsafeNativeMethods.cs
- ScriptComponentDescriptor.cs
- XmlSchemaDocumentation.cs
- CompleteWizardStep.cs
- RtfToXamlReader.cs
- _IPv4Address.cs
- PassportAuthenticationModule.cs
- UrlUtility.cs
- DataBindingCollection.cs
- ExpressionBuilder.cs
- AccessDataSource.cs
- CompilerResults.cs
- QueryCacheManager.cs
- PreloadHost.cs
- SpecialNameAttribute.cs
- StrokeSerializer.cs
- CursorInteropHelper.cs
- HtmlLink.cs
- M3DUtil.cs
- PageBreakRecord.cs
- DrawTreeNodeEventArgs.cs
- Binding.cs
- ResourceExpression.cs
- TransformerTypeCollection.cs
- ExpressionBinding.cs
- SharedUtils.cs
- DetailsViewRow.cs
- UIElement3D.cs
- NamedPipeChannelFactory.cs
- TextTabProperties.cs
- BitmapPalette.cs
- ColorAnimationBase.cs
- TableLayoutSettingsTypeConverter.cs