Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / CommonUI / System / Drawing / SystemPens.cs / 1 / SystemPens.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Drawing { using System.Diagnostics; using System; ////// /// Pens for select Windows system-wide colors. Whenever possible, try to use /// SystemPens and SystemBrushes rather than SystemColors. /// public sealed class SystemPens { static readonly object SystemPensKey = new object(); private SystemPens() { } ////// /// Pen is the color of the filled area of an active window border. /// public static Pen ActiveBorder { get { return FromSystemColor(SystemColors.ActiveBorder); } } ////// /// Pen is the color of the background of an active title bar caption. /// public static Pen ActiveCaption { get { return FromSystemColor(SystemColors.ActiveCaption); } } ////// /// Pen is the color of the active window's caption text. /// public static Pen ActiveCaptionText { get { return FromSystemColor(SystemColors.ActiveCaptionText); } } ////// /// Pen is the color of the application workspace. The application workspace /// is the area in a multiple document view that is not being occupied /// by documents. /// public static Pen AppWorkspace { get { return FromSystemColor(SystemColors.AppWorkspace); } } ////// /// Pen for the ButtonFace system color. /// public static Pen ButtonFace { get { return FromSystemColor(SystemColors.ButtonFace); } } ////// /// Pen for the ButtonHighlight system color. /// public static Pen ButtonHighlight { get { return FromSystemColor(SystemColors.ButtonHighlight); } } ////// /// Pen for the ButtonShadow system color. /// public static Pen ButtonShadow { get { return FromSystemColor(SystemColors.ButtonShadow); } } ////// /// Pen is the color of a button or control. /// public static Pen Control { get { return FromSystemColor(SystemColors.Control); } } ////// /// Pen is the color of the text on a button or control. /// public static Pen ControlText { get { return FromSystemColor(SystemColors.ControlText); } } ////// /// Pen is the color of the shadow part of a 3D element /// public static Pen ControlDark { get { return FromSystemColor(SystemColors.ControlDark); } } ////// /// Pen is the color of the darkest part of a 3D element /// public static Pen ControlDarkDark { get { return FromSystemColor(SystemColors.ControlDarkDark); } } ////// /// public static Pen ControlLight { get { return FromSystemColor(SystemColors.ControlLight); } } ///[To be supplied.] ////// /// Pen is the color of the lightest part of a 3D element /// public static Pen ControlLightLight { get { return FromSystemColor(SystemColors.ControlLightLight); } } ////// /// Pen is the color of the desktop. /// public static Pen Desktop { get { return FromSystemColor(SystemColors.Desktop); } } ////// /// Pen for the GradientActiveCaption system color. /// public static Pen GradientActiveCaption { get { return FromSystemColor(SystemColors.GradientActiveCaption); } } ////// /// Pen for the GradientInactiveCaption system color. /// public static Pen GradientInactiveCaption { get { return FromSystemColor(SystemColors.GradientInactiveCaption); } } ////// /// Pen is the color of disabled text. /// public static Pen GrayText { get { return FromSystemColor(SystemColors.GrayText); } } ////// /// Pen is the color of a highlighted background. /// public static Pen Highlight { get { return FromSystemColor(SystemColors.Highlight); } } ////// /// Pen is the color of highlighted text. /// public static Pen HighlightText { get { return FromSystemColor(SystemColors.HighlightText); } } ////// /// Pen is the color used to represent hot tracking. /// public static Pen HotTrack { get { return FromSystemColor(SystemColors.HotTrack); } } ////// /// Pen is the color if an inactive window border. /// public static Pen InactiveBorder { get { return FromSystemColor(SystemColors.InactiveBorder); } } ////// /// Pen is the color of an inactive caption bar. /// public static Pen InactiveCaption { get { return FromSystemColor(SystemColors.InactiveCaption); } } ////// /// Pen is the color of an inactive window's caption text. /// public static Pen InactiveCaptionText { get { return FromSystemColor(SystemColors.InactiveCaptionText); } } ////// /// Pen is the color of the info tooltip's background. /// public static Pen Info { get { return FromSystemColor(SystemColors.Info); } } ////// /// Pen is the color of the info tooltip's text. /// public static Pen InfoText { get { return FromSystemColor(SystemColors.InfoText); } } ////// /// Pen is the color of the background of a menu. /// public static Pen Menu { get { return FromSystemColor(SystemColors.Menu); } } ////// /// Pen is the color of the background of a menu bar. /// public static Pen MenuBar { get { return FromSystemColor(SystemColors.MenuBar); } } ////// /// Pen for the MenuHighlight system color. /// public static Pen MenuHighlight { get { return FromSystemColor(SystemColors.MenuHighlight); } } ////// /// Pen is the color of the menu text. /// public static Pen MenuText { get { return FromSystemColor(SystemColors.MenuText); } } ////// /// Pen is the color of the scroll bar area that is not being used by the /// thumb button. /// public static Pen ScrollBar { get { return FromSystemColor(SystemColors.ScrollBar); } } ////// /// Pen is the color of the client area of a window. /// public static Pen Window { get { return FromSystemColor(SystemColors.Window); } } ////// /// Pen is the color of the window frame. /// public static Pen WindowFrame { get { return FromSystemColor(SystemColors.WindowFrame); } } ////// /// Pen is the color of a window's text. /// public static Pen WindowText { get { return FromSystemColor(SystemColors.WindowText); } } ////// /// Retrieves a pen given a system color. An error will be raised /// if the color provide is not a system color. /// public static Pen FromSystemColor(Color c) { if (!c.IsSystemColor) { throw new ArgumentException(SR.GetString(SR.ColorNotSystemColor, c.ToString())); } Pen[] systemPens = (Pen[])SafeNativeMethods.Gdip.ThreadData[SystemPensKey]; if (systemPens == null) { systemPens = new Pen[(int)KnownColor.WindowText + (int)KnownColor.MenuHighlight - (int)KnownColor.YellowGreen]; SafeNativeMethods.Gdip.ThreadData[SystemPensKey] = systemPens; } int idx = (int)c.ToKnownColor(); if (idx > (int)KnownColor.YellowGreen) { idx -= (int)KnownColor.YellowGreen - (int)KnownColor.WindowText; } idx--; Debug.Assert(idx >= 0 && idx < systemPens.Length, "System colors have been added but our system color array has not been expanded."); if (systemPens[idx] == null) { systemPens[idx] = new Pen(c, true); } return systemPens[idx]; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
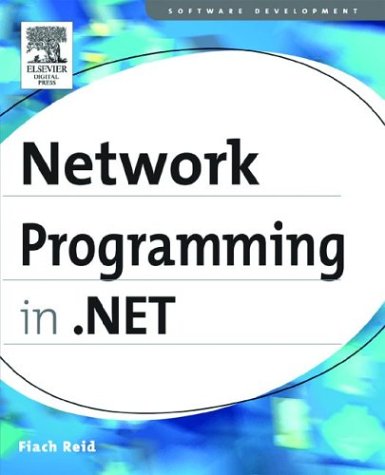
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DtrList.cs
- MailAddress.cs
- PaintEvent.cs
- Version.cs
- ProxyHelper.cs
- EditorPartCollection.cs
- ApplicationInfo.cs
- WebPartRestoreVerb.cs
- InputScopeAttribute.cs
- SqlCacheDependency.cs
- recordstate.cs
- _DigestClient.cs
- PriorityQueue.cs
- HttpRuntime.cs
- XhtmlConformanceSection.cs
- _AutoWebProxyScriptHelper.cs
- ProfileSettings.cs
- ListViewEditEventArgs.cs
- Repeater.cs
- LexicalChunk.cs
- Point3DValueSerializer.cs
- ComponentGlyph.cs
- QilPatternVisitor.cs
- DesignerSerializationOptionsAttribute.cs
- KnownColorTable.cs
- XmlDomTextWriter.cs
- InstanceDataCollection.cs
- SystemNetworkInterface.cs
- DeferredReference.cs
- CodeTypeReferenceExpression.cs
- UpdateExpressionVisitor.cs
- BuildProviderAppliesToAttribute.cs
- ExtendLockAsyncResult.cs
- WizardStepBase.cs
- SqlResolver.cs
- ListViewItem.cs
- XsdDataContractImporter.cs
- XmlChildNodes.cs
- XmlSerializerOperationFormatter.cs
- OleDbConnectionFactory.cs
- ProtocolsSection.cs
- ComponentResourceKeyConverter.cs
- ResourcePermissionBase.cs
- DesignerHelpers.cs
- SqlInfoMessageEvent.cs
- CallSiteOps.cs
- TimeZone.cs
- RawAppCommandInputReport.cs
- EntityTypeEmitter.cs
- EncryptedType.cs
- ConfigXmlSignificantWhitespace.cs
- TraceHwndHost.cs
- StateMachine.cs
- TextViewSelectionProcessor.cs
- OutputCache.cs
- ReachPrintTicketSerializer.cs
- MobileErrorInfo.cs
- DbSetClause.cs
- XmlValidatingReader.cs
- SqlProviderManifest.cs
- DocumentSequenceHighlightLayer.cs
- MessageHeaderT.cs
- RuleConditionDialog.Designer.cs
- DtrList.cs
- TypeSystemHelpers.cs
- Int64AnimationBase.cs
- Int16KeyFrameCollection.cs
- PropertyKey.cs
- AmbiguousMatchException.cs
- SqlDataReader.cs
- DataGridHeaderBorder.cs
- IPAddressCollection.cs
- SoapServerProtocol.cs
- AspProxy.cs
- XmlSerializerFactory.cs
- SmtpFailedRecipientsException.cs
- arabicshape.cs
- GlyphTypeface.cs
- DataGridLinkButton.cs
- HtmlTableRowCollection.cs
- TabRenderer.cs
- PrimitiveList.cs
- VirtualDirectoryMapping.cs
- SeverityFilter.cs
- FrugalMap.cs
- DefaultProxySection.cs
- HotCommands.cs
- X509SecurityTokenParameters.cs
- XmlnsDictionary.cs
- XPathItem.cs
- ExternalCalls.cs
- BaseCollection.cs
- BoundField.cs
- FixUp.cs
- DbConnectionPoolGroup.cs
- GridViewCancelEditEventArgs.cs
- PropertyInformation.cs
- EditingScope.cs
- NullNotAllowedCollection.cs
- TransformedBitmap.cs