Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WF / RunTime / TimerEventSubscriptionCollection.cs / 1305376 / TimerEventSubscriptionCollection.cs
using System; using System.Collections.Generic; using System.Text; using System.Workflow.Runtime.Hosting; using System.Workflow.ComponentModel; using System.Diagnostics; using System.Collections; namespace System.Workflow.Runtime { [Serializable] public class TimerEventSubscriptionCollection : ICollection { public readonly static DependencyProperty TimerCollectionProperty = DependencyProperty.RegisterAttached("TimerCollection", typeof(TimerEventSubscriptionCollection), typeof(TimerEventSubscriptionCollection)); private object locker = new Object(); private KeyedPriorityQueuequeue = new KeyedPriorityQueue (); #pragma warning disable 0414 private bool suspended = false; // no longer used but required for binary compatibility of serialization format #pragma warning restore 0414 [NonSerialized] private IWorkflowCoreRuntime executor; private Guid instanceId; internal TimerEventSubscriptionCollection(IWorkflowCoreRuntime executor, Guid instanceId) { this.executor = executor; this.instanceId = instanceId; WorkflowTrace.Host.TraceEvent(TraceEventType.Information, 0, "TimerEventSubscriptionQueue: {0} Created", instanceId); this.queue.FirstElementChanged += OnFirstElementChanged; } internal void Enqueue(TimerEventSubscription timerEventSubscription) { lock (locker) { WorkflowTrace.Host.TraceEvent(TraceEventType.Information, 0, "TimerEventSubscriptionQueue: {0} Enqueue Timer {1} for {2} ", instanceId, timerEventSubscription.SubscriptionId, timerEventSubscription.ExpiresAt); queue.Enqueue(timerEventSubscription.SubscriptionId, timerEventSubscription, timerEventSubscription.ExpiresAt); } } internal IWorkflowCoreRuntime Executor { get { return executor; } set { executor = value; } } public TimerEventSubscription Peek() { lock (locker) { return queue.Peek(); } } internal TimerEventSubscription Dequeue() { lock (locker) { TimerEventSubscription retval = queue.Dequeue(); if(retval != null) WorkflowTrace.Host.TraceEvent(TraceEventType.Information, 0, "TimerEventSubscriptionQueue: {0} Dequeue Timer {1} for {2} ", instanceId, retval.SubscriptionId, retval.ExpiresAt); return retval; } } public void Remove(Guid timerSubscriptionId) { lock (locker) { WorkflowTrace.Host.TraceEvent(TraceEventType.Information, 0, "TimerEventSubscriptionQueue: {0} Remove Timer {1}", instanceId, timerSubscriptionId); queue.Remove(timerSubscriptionId); } } private void OnFirstElementChanged(object source, KeyedPriorityQueueHeadChangedEventArgs e) { lock (locker) { ITimerService timerService = this.executor.GetService(typeof(ITimerService)) as ITimerService; if (e.NewFirstElement != null && executor != null) { WorkflowTrace.Host.TraceEvent(TraceEventType.Information, 0, "TimerEventSubscriptionQueue: {0} Schedule Timer {1} for {2} ", instanceId, e.NewFirstElement.SubscriptionId, e.NewFirstElement.ExpiresAt); timerService.ScheduleTimer(executor.ProcessTimersCallback, e.NewFirstElement.WorkflowInstanceId, e.NewFirstElement.ExpiresAt, e.NewFirstElement.SubscriptionId); } if (e.OldFirstElement != null) { WorkflowTrace.Host.TraceEvent(TraceEventType.Information, 0, "TimerEventSubscriptionQueue: {0} Unschedule Timer {1} for {2} ", instanceId, e.OldFirstElement.SubscriptionId, e.OldFirstElement.ExpiresAt); timerService.CancelTimer(e.OldFirstElement.SubscriptionId); } } } internal void SuspendDelivery() { lock (locker) { WorkflowTrace.Host.TraceEvent(TraceEventType.Information, 0, "TimerEventSubscriptionQueue: {0} Suspend", instanceId); WorkflowSchedulerService schedulerService = this.executor.GetService(typeof(WorkflowSchedulerService)) as WorkflowSchedulerService; TimerEventSubscription sub = queue.Peek(); if (sub != null) { schedulerService.Cancel(sub.SubscriptionId); } } } internal void ResumeDelivery() { lock (locker) { WorkflowTrace.Host.TraceEvent(TraceEventType.Information, 0, "TimerEventSubscriptionQueue: {0} Resume", instanceId); WorkflowSchedulerService schedulerService = this.executor.GetService(typeof(WorkflowSchedulerService)) as WorkflowSchedulerService; TimerEventSubscription sub = queue.Peek(); if (sub != null) { schedulerService.Schedule(executor.ProcessTimersCallback, sub.WorkflowInstanceId, sub.ExpiresAt, sub.SubscriptionId); } } } public void Add(TimerEventSubscription item) { if (item == null) throw new ArgumentNullException("item"); this.Enqueue(item); } public void Remove(TimerEventSubscription item) { if (item == null) throw new ArgumentNullException("item"); this.Remove(item.SubscriptionId); } #region ICollection Members public void CopyTo(Array array, int index) { TimerEventSubscription[] tes = null; lock (locker) { tes = new TimerEventSubscription[queue.Count]; queue.Values.CopyTo(tes, 0); } if(tes != null) tes.CopyTo(array, index); } public int Count { get { return queue.Count; } } public bool IsSynchronized { get { return true; } } public object SyncRoot { get { return locker; } } #endregion #region IEnumerable Members public IEnumerator GetEnumerator() { return queue.Values.GetEnumerator(); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections.Generic; using System.Text; using System.Workflow.Runtime.Hosting; using System.Workflow.ComponentModel; using System.Diagnostics; using System.Collections; namespace System.Workflow.Runtime { [Serializable] public class TimerEventSubscriptionCollection : ICollection { public readonly static DependencyProperty TimerCollectionProperty = DependencyProperty.RegisterAttached("TimerCollection", typeof(TimerEventSubscriptionCollection), typeof(TimerEventSubscriptionCollection)); private object locker = new Object(); private KeyedPriorityQueue queue = new KeyedPriorityQueue (); #pragma warning disable 0414 private bool suspended = false; // no longer used but required for binary compatibility of serialization format #pragma warning restore 0414 [NonSerialized] private IWorkflowCoreRuntime executor; private Guid instanceId; internal TimerEventSubscriptionCollection(IWorkflowCoreRuntime executor, Guid instanceId) { this.executor = executor; this.instanceId = instanceId; WorkflowTrace.Host.TraceEvent(TraceEventType.Information, 0, "TimerEventSubscriptionQueue: {0} Created", instanceId); this.queue.FirstElementChanged += OnFirstElementChanged; } internal void Enqueue(TimerEventSubscription timerEventSubscription) { lock (locker) { WorkflowTrace.Host.TraceEvent(TraceEventType.Information, 0, "TimerEventSubscriptionQueue: {0} Enqueue Timer {1} for {2} ", instanceId, timerEventSubscription.SubscriptionId, timerEventSubscription.ExpiresAt); queue.Enqueue(timerEventSubscription.SubscriptionId, timerEventSubscription, timerEventSubscription.ExpiresAt); } } internal IWorkflowCoreRuntime Executor { get { return executor; } set { executor = value; } } public TimerEventSubscription Peek() { lock (locker) { return queue.Peek(); } } internal TimerEventSubscription Dequeue() { lock (locker) { TimerEventSubscription retval = queue.Dequeue(); if(retval != null) WorkflowTrace.Host.TraceEvent(TraceEventType.Information, 0, "TimerEventSubscriptionQueue: {0} Dequeue Timer {1} for {2} ", instanceId, retval.SubscriptionId, retval.ExpiresAt); return retval; } } public void Remove(Guid timerSubscriptionId) { lock (locker) { WorkflowTrace.Host.TraceEvent(TraceEventType.Information, 0, "TimerEventSubscriptionQueue: {0} Remove Timer {1}", instanceId, timerSubscriptionId); queue.Remove(timerSubscriptionId); } } private void OnFirstElementChanged(object source, KeyedPriorityQueueHeadChangedEventArgs e) { lock (locker) { ITimerService timerService = this.executor.GetService(typeof(ITimerService)) as ITimerService; if (e.NewFirstElement != null && executor != null) { WorkflowTrace.Host.TraceEvent(TraceEventType.Information, 0, "TimerEventSubscriptionQueue: {0} Schedule Timer {1} for {2} ", instanceId, e.NewFirstElement.SubscriptionId, e.NewFirstElement.ExpiresAt); timerService.ScheduleTimer(executor.ProcessTimersCallback, e.NewFirstElement.WorkflowInstanceId, e.NewFirstElement.ExpiresAt, e.NewFirstElement.SubscriptionId); } if (e.OldFirstElement != null) { WorkflowTrace.Host.TraceEvent(TraceEventType.Information, 0, "TimerEventSubscriptionQueue: {0} Unschedule Timer {1} for {2} ", instanceId, e.OldFirstElement.SubscriptionId, e.OldFirstElement.ExpiresAt); timerService.CancelTimer(e.OldFirstElement.SubscriptionId); } } } internal void SuspendDelivery() { lock (locker) { WorkflowTrace.Host.TraceEvent(TraceEventType.Information, 0, "TimerEventSubscriptionQueue: {0} Suspend", instanceId); WorkflowSchedulerService schedulerService = this.executor.GetService(typeof(WorkflowSchedulerService)) as WorkflowSchedulerService; TimerEventSubscription sub = queue.Peek(); if (sub != null) { schedulerService.Cancel(sub.SubscriptionId); } } } internal void ResumeDelivery() { lock (locker) { WorkflowTrace.Host.TraceEvent(TraceEventType.Information, 0, "TimerEventSubscriptionQueue: {0} Resume", instanceId); WorkflowSchedulerService schedulerService = this.executor.GetService(typeof(WorkflowSchedulerService)) as WorkflowSchedulerService; TimerEventSubscription sub = queue.Peek(); if (sub != null) { schedulerService.Schedule(executor.ProcessTimersCallback, sub.WorkflowInstanceId, sub.ExpiresAt, sub.SubscriptionId); } } } public void Add(TimerEventSubscription item) { if (item == null) throw new ArgumentNullException("item"); this.Enqueue(item); } public void Remove(TimerEventSubscription item) { if (item == null) throw new ArgumentNullException("item"); this.Remove(item.SubscriptionId); } #region ICollection Members public void CopyTo(Array array, int index) { TimerEventSubscription[] tes = null; lock (locker) { tes = new TimerEventSubscription[queue.Count]; queue.Values.CopyTo(tes, 0); } if(tes != null) tes.CopyTo(array, index); } public int Count { get { return queue.Count; } } public bool IsSynchronized { get { return true; } } public object SyncRoot { get { return locker; } } #endregion #region IEnumerable Members public IEnumerator GetEnumerator() { return queue.Values.GetEnumerator(); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
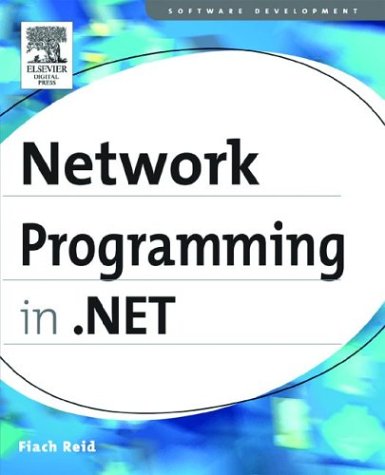
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SafeTimerHandle.cs
- XPathBinder.cs
- MetafileHeaderWmf.cs
- Privilege.cs
- UnitySerializationHolder.cs
- EllipticalNodeOperations.cs
- DataKey.cs
- CodeSubDirectoriesCollection.cs
- TableChangeProcessor.cs
- WebConfigurationManager.cs
- SplitContainerDesigner.cs
- CompilationPass2Task.cs
- EventManager.cs
- BitConverter.cs
- Span.cs
- DBConnection.cs
- VisualStyleRenderer.cs
- HebrewNumber.cs
- XmlSchemaAnnotation.cs
- DebugView.cs
- InternalRelationshipCollection.cs
- SqlResolver.cs
- StickyNoteContentControl.cs
- FileFormatException.cs
- DataMisalignedException.cs
- RolePrincipal.cs
- StringValidator.cs
- Pair.cs
- PropertyReferenceSerializer.cs
- httpapplicationstate.cs
- EntityDataSourceWizardForm.cs
- XmlProcessingInstruction.cs
- HtmlUtf8RawTextWriter.cs
- SoundPlayer.cs
- HuffCodec.cs
- Int32Rect.cs
- ExportException.cs
- SqlTypeConverter.cs
- PropertyPathConverter.cs
- RijndaelManagedTransform.cs
- OutputCacheSettingsSection.cs
- StringTraceRecord.cs
- CompModSwitches.cs
- ControlEvent.cs
- DefaultWorkflowTransactionService.cs
- OdbcCommand.cs
- XmlSchemaSimpleTypeRestriction.cs
- ExpressionBuilderContext.cs
- FormCollection.cs
- RewritingProcessor.cs
- RecordsAffectedEventArgs.cs
- DataTablePropertyDescriptor.cs
- LongValidator.cs
- StructuredTypeEmitter.cs
- Parser.cs
- GestureRecognizer.cs
- CellParaClient.cs
- ToolStripItemBehavior.cs
- FilterQueryOptionExpression.cs
- IdentitySection.cs
- JulianCalendar.cs
- ProviderSettings.cs
- OutputCache.cs
- IFlowDocumentViewer.cs
- ColumnMapCopier.cs
- XmlRawWriterWrapper.cs
- FixedPage.cs
- BaseProcessor.cs
- parserscommon.cs
- Encoder.cs
- DecimalConverter.cs
- MenuItem.cs
- ObjectParameterCollection.cs
- CaseCqlBlock.cs
- CompositeTypefaceMetrics.cs
- Base64Decoder.cs
- CompilationLock.cs
- ImageFormatConverter.cs
- DataGridAddNewRow.cs
- Helpers.cs
- EntityTypeEmitter.cs
- Win32Native.cs
- DataProtection.cs
- DynamicDataRouteHandler.cs
- RemoteWebConfigurationHostServer.cs
- MenuItem.cs
- MatrixCamera.cs
- PinnedBufferMemoryStream.cs
- PeerCollaboration.cs
- TemplatedAdorner.cs
- XmlArrayItemAttribute.cs
- OLEDB_Util.cs
- X509AsymmetricSecurityKey.cs
- AnimationStorage.cs
- Italic.cs
- BitmapMetadata.cs
- KeyValueConfigurationCollection.cs
- safemediahandle.cs
- OrCondition.cs
- PaperSize.cs