Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / clr / src / BCL / System / Boolean.cs / 1 / Boolean.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: Boolean ** ** ** Purpose: The boolean class serves as a wrapper for the primitive ** type boolean. ** ** ===========================================================*/ namespace System { using System; using System.Globalization; // The Boolean class provides the // object representation of the boolean primitive type. [Serializable()] [System.Runtime.InteropServices.ComVisible(true)] public struct Boolean : IComparable, IConvertible #if GENERICS_WORK , IComparable, IEquatable #endif { // // Member Variables // private bool m_value; private static char[] m_trimmableChars; // The true value. // internal const int True = 1; // The false value. // internal const int False = 0; // // Internal Constants are real consts for performance. // // The internal string representation of true. // internal const String TrueLiteral = "True"; // The internal string representation of false. // internal const String FalseLiteral = "False"; // // Public Constants // // The public string representation of true. // public static readonly String TrueString = TrueLiteral; // The public string representation of false. // public static readonly String FalseString = FalseLiteral; // // Overriden Instance Methods // /*=================================GetHashCode================================== **Args: None **Returns: 1 or 0 depending on whether this instance represents true or false. **Exceptions: None **Overriden From: Value ==============================================================================*/ // Provides a hash code for this instance. public override int GetHashCode() { return (m_value)?True:False; } /*===================================ToString=================================== **Args: None **Returns: "True" or "False" depending on the state of the boolean. **Exceptions: None. ==============================================================================*/ // Converts the boolean value of this instance to a String. public override String ToString() { if (false == m_value) { return FalseLiteral; } return TrueLiteral; } public String ToString(IFormatProvider provider) { if (false == m_value) { return FalseLiteral; } return TrueLiteral; } // Determines whether two Boolean objects are equal. public override bool Equals (Object obj) { //If it's not a boolean, we're definitely not equal if (!(obj is Boolean)) { return false; } return (m_value==((Boolean)obj).m_value); } public bool Equals(Boolean obj) { return m_value == obj; } // Compares this object to another object, returning an integer that // indicates the relationship. For booleans, false sorts before true. // null is considered to be less than any instance. // If object is not of type boolean, this method throws an ArgumentException. // // Returns a value less than zero if this object // public int CompareTo(Object obj) { if (obj==null) { return 1; } if (!(obj is Boolean)) { throw new ArgumentException (Environment.GetResourceString("Arg_MustBeBoolean")); } if (m_value==((Boolean)obj).m_value) { return 0; } else if (m_value==false) { return -1; } return 1; } public int CompareTo(Boolean value) { if (m_value==value) { return 0; } else if (m_value==false) { return -1; } return 1; } // // Static Methods // // Determines whether a String represents true or false. // public static Boolean Parse (String value) { if (value==null) throw new ArgumentNullException("value"); Boolean result = false; if (!TryParse(value, out result)) { throw new FormatException(Environment.GetResourceString("Format_BadBoolean")); } else { return result; } } // Determines whether a String represents true or false. // public static Boolean TryParse (String value, out Boolean result) { result = false; if (value==null) { return false; } // For perf reasons, let's first see if they're equal, then do the // trim to get rid of white space, and check again. if (TrueLiteral.Equals(value, StringComparison.OrdinalIgnoreCase)) { result = true; return true; } if (FalseLiteral.Equals(value,StringComparison.OrdinalIgnoreCase)) { result = false; return true; } // Special case: Trim whitespace as well as null characters. // Solution: Lazily initialize a new character array including 0x0000 if (null == m_trimmableChars) { char [] m_trimmableCharsTemp = new char[String.WhitespaceChars.Length + 1]; Array.Copy(String.WhitespaceChars, m_trimmableCharsTemp, String.WhitespaceChars.Length); m_trimmableCharsTemp[m_trimmableCharsTemp.Length - 1] = (char) 0x0000; m_trimmableChars = m_trimmableCharsTemp; } value = value.Trim(m_trimmableChars); // Remove leading & trailing white space. if (TrueLiteral.Equals(value, StringComparison.OrdinalIgnoreCase)) { result = true; return true; } if (FalseLiteral.Equals(value,StringComparison.OrdinalIgnoreCase)) { result = false; return true; } return false; } // // IValue implementation // public TypeCode GetTypeCode() { return TypeCode.Boolean; } /// bool IConvertible.ToBoolean(IFormatProvider provider) { return m_value; } /// char IConvertible.ToChar(IFormatProvider provider) { throw new InvalidCastException(String.Format(CultureInfo.CurrentCulture, Environment.GetResourceString("InvalidCast_FromTo"), "Boolean", "Char")); } /// sbyte IConvertible.ToSByte(IFormatProvider provider) { return Convert.ToSByte(m_value); } /// byte IConvertible.ToByte(IFormatProvider provider) { return Convert.ToByte(m_value); } /// short IConvertible.ToInt16(IFormatProvider provider) { return Convert.ToInt16(m_value); } /// ushort IConvertible.ToUInt16(IFormatProvider provider) { return Convert.ToUInt16(m_value); } /// int IConvertible.ToInt32(IFormatProvider provider) { return Convert.ToInt32(m_value); } /// uint IConvertible.ToUInt32(IFormatProvider provider) { return Convert.ToUInt32(m_value); } /// long IConvertible.ToInt64(IFormatProvider provider) { return Convert.ToInt64(m_value); } /// ulong IConvertible.ToUInt64(IFormatProvider provider) { return Convert.ToUInt64(m_value); } /// float IConvertible.ToSingle(IFormatProvider provider) { return Convert.ToSingle(m_value); } /// double IConvertible.ToDouble(IFormatProvider provider) { return Convert.ToDouble(m_value); } /// Decimal IConvertible.ToDecimal(IFormatProvider provider) { return Convert.ToDecimal(m_value); } /// DateTime IConvertible.ToDateTime(IFormatProvider provider) { throw new InvalidCastException(String.Format(CultureInfo.CurrentCulture, Environment.GetResourceString("InvalidCast_FromTo"), "Boolean", "DateTime")); } /// Object IConvertible.ToType(Type type, IFormatProvider provider) { return Convert.DefaultToType((IConvertible)this, type, provider); } } }
Link Menu
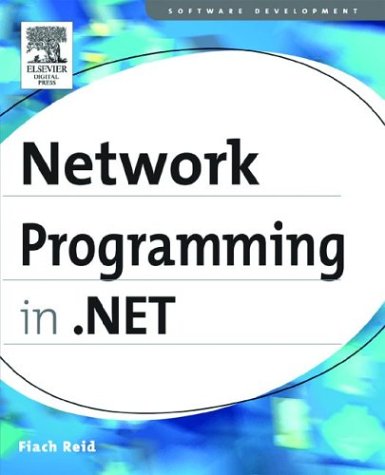
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CmsUtils.cs
- NodeFunctions.cs
- DbProviderSpecificTypePropertyAttribute.cs
- PinnedBufferMemoryStream.cs
- SqlConnectionFactory.cs
- Column.cs
- Range.cs
- PeerTransportElement.cs
- EncryptedType.cs
- RelativeSource.cs
- TextBoxAutomationPeer.cs
- CompilationLock.cs
- DataGridViewCellStyle.cs
- ByteStreamGeometryContext.cs
- InvariantComparer.cs
- CacheChildrenQuery.cs
- ErrorHandlerModule.cs
- QuaternionAnimationUsingKeyFrames.cs
- ProofTokenCryptoHandle.cs
- MetadataCacheItem.cs
- ConfigurationManagerHelperFactory.cs
- BindingRestrictions.cs
- BaseAppDomainProtocolHandler.cs
- DeclarativeCatalogPart.cs
- GridViewRowEventArgs.cs
- ReadOnlyDataSource.cs
- SmtpAuthenticationManager.cs
- Panel.cs
- SynchronizedPool.cs
- Oci.cs
- TextWriter.cs
- GridViewDeletedEventArgs.cs
- CompensationToken.cs
- ResponseStream.cs
- DataTableReader.cs
- MemberJoinTreeNode.cs
- WorkflowTraceTransfer.cs
- XmlTextEncoder.cs
- OdbcConnectionFactory.cs
- PeerToPeerException.cs
- ZipIORawDataFileBlock.cs
- UnsafeNativeMethods.cs
- NavigationCommands.cs
- SymbolDocumentInfo.cs
- ListBoxChrome.cs
- HtmlInputRadioButton.cs
- _SSPIWrapper.cs
- AnnotationHelper.cs
- BookmarkResumptionRecord.cs
- DefaultValueConverter.cs
- ObjectViewQueryResultData.cs
- UntypedNullExpression.cs
- BrowserInteropHelper.cs
- DefaultMemberAttribute.cs
- IERequestCache.cs
- ConfigXmlSignificantWhitespace.cs
- PolicyStatement.cs
- DesignerRegionCollection.cs
- SignatureToken.cs
- smtppermission.cs
- AssemblyLoader.cs
- DocumentCollection.cs
- DataGridViewColumn.cs
- XmlSchemaComplexContentExtension.cs
- RegexStringValidatorAttribute.cs
- InputScopeConverter.cs
- PersistChildrenAttribute.cs
- FontStretches.cs
- UniqueConstraint.cs
- OpenTypeMethods.cs
- ExceptionAggregator.cs
- TreeView.cs
- ZoomPercentageConverter.cs
- FontResourceCache.cs
- AssemblyName.cs
- CrossAppDomainChannel.cs
- XmlAtomicValue.cs
- WebZoneDesigner.cs
- RegistryPermission.cs
- MobileComponentEditorPage.cs
- Helper.cs
- PropertyChangedEventArgs.cs
- MetadataItemCollectionFactory.cs
- TemplateBamlRecordReader.cs
- StreamInfo.cs
- XPathBuilder.cs
- WebPartConnectionCollection.cs
- DataGridViewCheckBoxColumn.cs
- SID.cs
- StylusLogic.cs
- IntranetCredentialPolicy.cs
- ExpressionDumper.cs
- SecurityUtils.cs
- DataKey.cs
- SqlTypeSystemProvider.cs
- RegistryPermission.cs
- InputBinder.cs
- RoutingSection.cs
- GridItemCollection.cs
- ConfigurationManager.cs