Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / TrustUi / MS / Internal / documents / SigningDialog.cs / 1 / SigningDialog.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // SigningDialog is the Forms dialog that allows users to select signing parameters. // // History: // 05/03/05 - [....] created // //--------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Drawing; using System.Windows.Forms; using System.Windows.TrustUI; using System.Security.Cryptography.X509Certificates; using System.Security; // for SecurityCritical, etc. using System.Globalization; // For localization of string conversion using MS.Internal.Documents.Application; namespace MS.Internal.Documents { ////// SigningDialog is used for signing a XPS document /// internal sealed partial class SigningDialog : DialogBaseForm { #region Constructors //----------------------------------------------------- // // Constructors // //----------------------------------------------------- ////// The constructor /// ////// Critical - This is critical since we only want critical code paths creating this class. This is /// necessary to ensure that we are getting a trusted X.509 Certificate /// [SecurityCritical] internal SigningDialog(X509Certificate2 x509Certificate2, DigitalSignature digitalSignatureRequest, DocumentSignatureManager docSigManager) { if (x509Certificate2 == null) { throw new ArgumentNullException("x509Certificate2"); } if (docSigManager == null) { throw new ArgumentNullException("docSigManager"); } _docSigManager = docSigManager; _x509Certificate2 = x509Certificate2; // setting critical data. //This can be null and if so means this is a regular first signing //(not signing a request or 2nd/3rd.. regular request which can't have //signatureDefinitions.) _digitalSignatureRequest = digitalSignatureRequest; //Now we need to set all DigSig Specific Text ApplySignatureSpecificResources(); _signButton.Enabled = false; // Check DocumentManager to see if we can save package DocumentManager documentManager = DocumentManager.CreateDefault(); if (documentManager != null) { _signButton.Enabled = documentManager.CanSave; } if (DocumentRightsManagementManager.Current != null) { _signSaveAsButton.Enabled = DocumentRightsManagementManager.Current.HasPermissionToSave; } } #endregion Constructors #region Private Methods //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- ////// Method for Signing and Save/SaveAs. /// ////// Critical - Creates and modifies a DigitalSignature structure. /// TreatAsSafe - This is safe because we are creating a DigitalSignature structure based on a trusted /// certificate supplied at dialog creation time (enforced by the critical constructor) /// and the other properties of the DigitalCertificate are set based on direct user input. /// - This certificate is being used to sign a document on behalf of the user so the user /// specified data should be trusted since the user would not do something to hurt himself. /// - The user input is entered via a WinForm dialog which is not tamperable from code that /// is running in Partial Trust and Avalon based. /// [SecurityCritical, SecurityTreatAsSafe] private void SignAndSave(bool isSaveAs) { //Create new DigSig DigitalSignature digSig = null; //If this is a request, re-use the requested signature, so we update the request //to the signed version in place. if (_digitalSignatureRequest != null && _digitalSignatureRequest.GuidID != null) { digSig = _digitalSignatureRequest; } else { digSig = new DigitalSignature(); } //Get this user input and set to DigSig. digSig.Certificate = this.Certificate; // If this is a second signature that was not requested, then set the // default values for Reason and Location. if (_isSecondSignatureNotRequested) { string none = SR.Get(SRID.SignatureResourceHelperNone); digSig.Reason = none; digSig.Location = none; } else { digSig.Reason = Intent; digSig.Location = LocationText; } digSig.IsDocumentPropertiesRestricted = IsDocPropsRestricted; digSig.IsAddingSignaturesRestricted = IsDigSigRestricted; // if signing worked close because we are done // else do nothing to leave the dialog open // signing may have failed either because the user cancled or there // was a non-fatal error like the destination file was in use if (_docSigManager.SignDocument(digSig, this, isSaveAs)) { Close(); } } ////// Handler for sign and save button. /// private void _signSaveButton_Click(object sender, EventArgs e) { // To prevent launching multiple dispatcher operations that try to sign the // document simulatenously, disable both signing buttons during the operation. _signButton.Enabled = false; bool saveAsEnabled = _signSaveAsButton.Enabled; _signSaveAsButton.Enabled = false; SignAndSave(false); _signButton.Enabled = true; _signSaveAsButton.Enabled = saveAsEnabled; } ////// Handler for sign and save as button. /// private void _signSaveAsButton_Click(object sender, EventArgs e) { // To prevent launching multiple dispatcher operations that try to sign the // document simulatenously, disable both signing buttons during the operation. _signSaveAsButton.Enabled = false; bool saveEnabled = _signButton.Enabled; _signButton.Enabled = false; SignAndSave(true); _signSaveAsButton.Enabled = true; _signButton.Enabled = saveEnabled; } ////// Handler for cancel button. /// private void _cancelButton_Click(object sender, EventArgs e) { Close(); } ////// ApplySignatureSpecificResources - sets the text based on Cert and Signature. /// private void ApplySignatureSpecificResources() { _signerlabel.Text = String.Format(CultureInfo.CurrentCulture, SR.Get(SRID.SigningDialogSignerlabel), Certificate.GetNameInfo(X509NameType.SimpleName, false /*Issuer*/) ); //Check to see if this signing is a request(or second signing without request). if (_digitalSignatureRequest != null) { // For both Reason and Location, determine if this is a second signing // without a request. If this is without a request, then display // "N/A" in the textboxes. if (String.IsNullOrEmpty(_digitalSignatureRequest.Reason) && String.IsNullOrEmpty(_digitalSignatureRequest.Location)) { _isSecondSignatureNotRequested = true; string na = SR.Get(SRID.SigningDialogNA); _reasonComboBox.Text = na; _locationTextBox.Text = na; } else { // Since this is a requested signature replace any empty strings // with "" string none = SR.Get(SRID.SignatureResourceHelperNone); _reasonComboBox.Text = String.IsNullOrEmpty(_digitalSignatureRequest.Reason) ? none : _digitalSignatureRequest.Reason; _locationTextBox.Text = String.IsNullOrEmpty(_digitalSignatureRequest.Location) ? none : _digitalSignatureRequest.Location; } //This signature can't create new signatureDefinition so Enable=false to all //related UI. _reasonComboBox.Enabled = false; _reasonLabel.Enabled = false; _locationTextBox.Enabled = false; _locationLabel.Enabled = false; _addDigSigCheckBox.Enabled = false; _addDocPropCheckBox.Enabled = false; } // Regardless of the settings above, if there are any Signature Requests, // then we should disable the checkbox option that says additional signatures // will break this signature. This covers ad hoc signing when signatures requests // also exist. _addDigSigCheckBox.Enabled = _addDigSigCheckBox.Enabled & !_docSigManager.HasRequests; } #endregion Private Methods #region Protected Methods //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ /// /// ApplyResources override. Called to apply dialog resources. /// protected override void ApplyResources() { base.ApplyResources(); //Get the localized text strings. _reasonLabel.Text = SR.Get(SRID.SigningDialogReasonLabel); _locationLabel.Text = SR.Get(SRID.SigningDialogLocationLabel); _actionlabel.Text = SR.Get(SRID.SigningDialogActionlabel); _addDocPropCheckBox.Text = SR.Get(SRID.SigningDialogAddDocPropCheckBox); _addDigSigCheckBox.Text = SR.Get(SRID.SigningDialogAddDigSigCheckBox); _cancelButton.Text = SR.Get(SRID.SigningDialogCancelButton); _signButton.Text = SR.Get(SRID.SigningDialogSignButton); _signSaveAsButton.Text = SR.Get(SRID.SigningDialogSignSaveAsButton); Text = SR.Get(SRID.SigningDialogTitle); //Load the Intent/Reason combo _reasonComboBox.Items.Add(SR.Get(SRID.DigSigIntentString1)); _reasonComboBox.Items.Add(SR.Get(SRID.DigSigIntentString2)); _reasonComboBox.Items.Add(SR.Get(SRID.DigSigIntentString3)); _reasonComboBox.Items.Add(SR.Get(SRID.DigSigIntentString4)); _reasonComboBox.Items.Add(SR.Get(SRID.DigSigIntentString5)); _reasonComboBox.Items.Add(SR.Get(SRID.DigSigIntentString6)); } ////// ApplyStyle /// protected override void ApplyStyle() { base.ApplyStyle(); //Set the signer label to bold. _signerlabel.Font = new Font(System.Drawing.SystemFonts.DialogFont, System.Drawing.SystemFonts.DialogFont.Style | FontStyle.Bold); } #endregion Protected Methods #region Internal properties ////// String field Intent. /// internal string Intent { get { return _reasonComboBox.Text; } } ////// String field Location. /// internal string LocationText { get { return _locationTextBox.Text; } } ////// Is Doc Props Restricted. /// internal bool IsDocPropsRestricted { get { return _addDocPropCheckBox.Checked; } } ////// Is Dig Sigs Restricted. /// internal bool IsDigSigRestricted { get { return _addDigSigCheckBox.Checked; } } internal X509Certificate2 Certificate { ////// Critical - It returns a data piece that is marked SecurityCritical /// TreatAsSafe - We only really want to make this critical for set. It was /// supplied to us by a critical caller and is safe for reading. /// [SecurityCritical, SecurityTreatAsSafe] get { return _x509Certificate2; } } #endregion Internal properties #region Private Fields //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ private DocumentSignatureManager _docSigManager; ////// Critical - This certificate is critical for set /// [SecurityCritical] private X509Certificate2 _x509Certificate2; private DigitalSignature _digitalSignatureRequest; ////// Used to determine if this signing is for an additional signature, /// without a signature requested. /// private bool _isSecondSignatureNotRequested; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
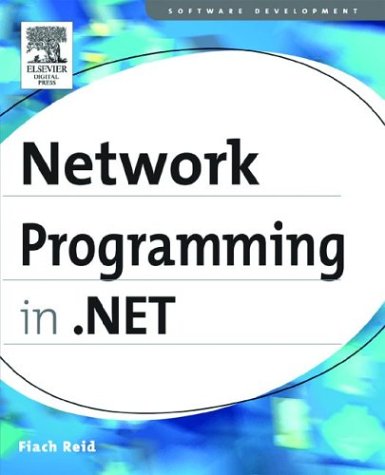
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NaturalLanguageHyphenator.cs
- NetMsmqBinding.cs
- NameValueConfigurationElement.cs
- SourceLineInfo.cs
- NavigationFailedEventArgs.cs
- ServiceDurableInstanceContextProvider.cs
- NavigationHelper.cs
- BufferModeSettings.cs
- GorillaCodec.cs
- OleDbParameter.cs
- FormsIdentity.cs
- SqlTypeConverter.cs
- EventLogSession.cs
- ConfigurationStrings.cs
- HttpListenerElement.cs
- TableCellCollection.cs
- HyperLink.cs
- ImageMap.cs
- InvalidComObjectException.cs
- Help.cs
- DockPanel.cs
- PeerNameRegistration.cs
- MarshalByRefObject.cs
- TabPage.cs
- PathSegmentCollection.cs
- FrameworkTextComposition.cs
- Encoder.cs
- SID.cs
- ChangePasswordAutoFormat.cs
- Int32CollectionConverter.cs
- OpCopier.cs
- GridViewRowPresenter.cs
- diagnosticsswitches.cs
- UIElement3D.cs
- DurationConverter.cs
- SoapSchemaImporter.cs
- ProtectedProviderSettings.cs
- SchemaMerger.cs
- ItemCheckedEvent.cs
- RowUpdatedEventArgs.cs
- RightsManagementInformation.cs
- FunctionDetailsReader.cs
- TdsValueSetter.cs
- XmlElementCollection.cs
- SQLInt16Storage.cs
- DiagnosticsConfiguration.cs
- StorageAssociationTypeMapping.cs
- TileModeValidation.cs
- MemoryMappedFileSecurity.cs
- SpeechRecognizer.cs
- controlskin.cs
- PrintingPermissionAttribute.cs
- BitArray.cs
- PropertyValueUIItem.cs
- BamlResourceDeserializer.cs
- ProtocolState.cs
- PointLightBase.cs
- WebPartMenuStyle.cs
- ImageBrush.cs
- Cell.cs
- AttributeExtensions.cs
- AssemblyAttributesGoHere.cs
- DataColumnMappingCollection.cs
- MouseGesture.cs
- DataGridViewSelectedCellCollection.cs
- OracleMonthSpan.cs
- KoreanCalendar.cs
- ControlSerializer.cs
- ViewKeyConstraint.cs
- UIElementIsland.cs
- ElapsedEventArgs.cs
- SecurityAttributeGenerationHelper.cs
- TimeSpanHelper.cs
- TextLine.cs
- DataGridGeneralPage.cs
- ImagingCache.cs
- EndOfStreamException.cs
- VisualCollection.cs
- DataGridCell.cs
- SchemaMerger.cs
- CodeDirectionExpression.cs
- EdmValidator.cs
- TagPrefixInfo.cs
- ServiceHost.cs
- HelpEvent.cs
- storagemappingitemcollection.viewdictionary.cs
- SetterBase.cs
- XmlNamespaceManager.cs
- DataGridViewCellToolTipTextNeededEventArgs.cs
- XmlCustomFormatter.cs
- ReplacementText.cs
- CodeDefaultValueExpression.cs
- DecoratedNameAttribute.cs
- AccessibleObject.cs
- WebAdminConfigurationHelper.cs
- XmlAtomErrorReader.cs
- IImplicitResourceProvider.cs
- DelegateSerializationHolder.cs
- CaseInsensitiveHashCodeProvider.cs
- ApplicationHost.cs