Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Print / Reach / PrintConfig / PrtTicket_Public.cs / 1 / PrtTicket_Public.cs
/*++ Copyright (C) 2003-2005 Microsoft Corporation All rights reserved. Module Name: PrtTicket_Public.cs Abstract: Definition and implementation of public PrintTicket class. Author: [....] ([....]) 7/28/2003 --*/ using System; using System.Xml; using System.IO; using System.Collections; using System.Reflection; using System.Runtime.InteropServices; using System.Diagnostics; using System.Globalization; using System.Printing; using MS.Internal.Printing.Configuration; namespace MS.Internal.Printing.Configuration { ////// Represents Print Ticket settings. /// internal class InternalPrintTicket { #region Constructors ////// Constructs a new instance of the InternalPrintTicket class with no settings. /// public InternalPrintTicket() { #if _DEBUG InitTrace(); #endif _xmlDoc = new XmlDocument(); // If client doesn't provide an XML PrintTicket, we will start with the // built-in empty PrintTicket. We don't expect this loading will cause // any exceptions. _xmlDoc.LoadXml(PrintSchemaTags.Framework.EmptyPrintTicket); SetupNamespaceManager(); } ////// Constructs a new instance of the InternalPrintTicket class with settings based on the XML form of PrintTicket. /// /// Stream object containing the XML form of PrintTicket. ////// The ///parameter is null. /// /// The stream object specified by public InternalPrintTicket(Stream xmlStream) { // Verify input parameter if (xmlStream == null) { throw new ArgumentNullException("xmlStream"); } #if _DEBUG InitTrace(); #endif _xmlDoc = new XmlDocument(); try { // After reading the data from the user supplied input stream, we do NOT // reset the stream position. This allows us to provide consistent behavior // for seekable and non-seekable streams. It's trivial for application to // reset the stream position based on their own needs. _xmlDoc.Load(xmlStream); // Make sure the XML content we write out is in UTF-8. XmlDeclaration xmlDecl = _xmlDoc.FirstChild as XmlDeclaration; if (xmlDecl != null) { // XML declaration already exists in the input PrintTicket XML stream. // So enforce the UTF-8 encoding. xmlDecl.Encoding = "UTF-8"; } else { // There's no XML declaration in the input PrintTicket XML stream. // So create the XML declaration with UTF-8 encoding. xmlDecl = _xmlDoc.CreateXmlDeclaration("1.0", "UTF-8", null); _xmlDoc.InsertBefore(xmlDecl, _xmlDoc.DocumentElement); } } catch (XmlException e) { // We catch the XmlException thrown by XML parser and rethrow our own FormatException // so that our client only needs to care about FormatException for non-well-formed Print Ticket. throw NewPTFormatException(e.Message, e); } SetupNamespaceManager(); // Now the client supplied XML PrintTicket has been verified by XML // parser as well-formed XML, but we still need to further verify that // it is also well-formed PrintTicket // PrintTicketEditor.CheckIsWellFormedPrintTicket(this); PrintTicketEditor.CheckAndAddMissingStdNamespaces(this); } #endregion Constructors #region Public Methods ///parameter doesn't contain a well-formed XML PrintTicket. /// The exception object's property describes why the XML is not well-formed. /// And if not null, the exception object's property provides more details. /// /// Creates a duplicate of this InternalPrintTicket object. /// ///The duplicated InternalPrintTicket object. public InternalPrintTicket Clone() { // Design Guideline says do not implement ICloneable since that interface doesn't // specify whether the clone is a deep copy or non-deep copy. So it's recommended // that each type defines its own Clone or Copy methodology. InternalPrintTicket clonePT = new InternalPrintTicket(this.XmlStream); return clonePT; } ////// Saves this InternalPrintTicket object to the specified stream. /// /// The stream to which you want to save. ////// The public void SaveTo(Stream outStream) { // Verify input parameter if (outStream == null) { throw new ArgumentNullException("outStream"); } // After writing the data to the user supplied output stream, we do NOT // reset the stream position. This allows us to provide consistent behavior // for seekable and non-seekable streams. It's trivial for application to // reset the stream position based on their own needs. _xmlDoc.Save(outStream); } #endregion Public Methods #region Public Properties ///parameter is null. /// /// Gets a public DocumentCollateSetting DocumentCollate { get { if (_docCollate == null) { _docCollate = new DocumentCollateSetting(this); } return _docCollate; } } ///object that specifies the document collate setting. /// /// Gets a public JobDuplexSetting JobDuplex { get { if (_jobDuplex == null) { _jobDuplex = new JobDuplexSetting(this); } return _jobDuplex; } } ///object that specifies the job duplex setting. /// /// Gets a public JobNUpSetting JobNUp { get { if (_jobNUp == null) { _jobNUp = new JobNUpSetting(this); } return _jobNUp; } } ///object that specifies the job NUp setting. /// /// Gets a public JobStapleSetting JobStaple { get { if (_jobStaple == null) { _jobStaple = new JobStapleSetting(this); } return _jobStaple; } } ///object that specifies the job output stapling setting. /// /// Gets a public PageDeviceFontSubstitutionSetting PageDeviceFontSubstitution { get { if (_pageDeviceFontSubst == null) { _pageDeviceFontSubst = new PageDeviceFontSubstitutionSetting(this); } return _pageDeviceFontSubst; } } ///object that specifies the page device font substitution setting. /// /// Gets a public PageMediaSizeSetting PageMediaSize { get { if (_pageMediaSize == null) { _pageMediaSize = new PageMediaSizeSetting(this); } return _pageMediaSize; } } ///object that specifies the page media size setting. /// /// Gets a public PageMediaTypeSetting PageMediaType { get { if (_pageMediaType == null) { _pageMediaType = new PageMediaTypeSetting(this); } return _pageMediaType; } } ///object that specifies the page media type setting. /// /// Gets a public PageOrientationSetting PageOrientation { get { if (_pageOrientation == null) { _pageOrientation = new PageOrientationSetting(this); } return _pageOrientation; } } ///object that specifies the page orientation setting. /// /// Gets a public PageOutputColorSetting PageOutputColor { get { if (_pageOutputColor == null) { _pageOutputColor = new PageOutputColorSetting(this); } return _pageOutputColor; } } ///object that specifies the page output color setting. /// /// Gets a public PageResolutionSetting PageResolution { get { if (_pageResolution == null) { _pageResolution = new PageResolutionSetting(this); } return _pageResolution; } } ///object that specifies the page resolution setting. /// /// Gets a public PageScalingSetting PageScaling { get { if (_pageScaling == null) { _pageScaling = new PageScalingSetting(this); } return _pageScaling; } } ///object that specifies the page scaling setting. /// /// Gets a public PageTrueTypeFontModeSetting PageTrueTypeFontMode { get { if (_pageTrueTypeFontMode == null) { _pageTrueTypeFontMode = new PageTrueTypeFontModeSetting(this); } return _pageTrueTypeFontMode; } } ///object that specifies the page TrueType font handling mode setting. /// /// Gets a public JobPageOrderSetting JobPageOrder { get { if (_jobPageOrder == null) { _jobPageOrder = new JobPageOrderSetting(this); } return _jobPageOrder; } } ///object that specifies the job page order setting. /// /// Gets a public PagePhotoPrintingIntentSetting PagePhotoPrintingIntent { get { if (_pagePhotoIntent == null) { _pagePhotoIntent = new PagePhotoPrintingIntentSetting(this); } return _pagePhotoIntent; } } ///object that specifies the page photo printing intent setting. /// /// Gets a public PageBorderlessSetting PageBorderless { get { if (_pageBorderless == null) { _pageBorderless = new PageBorderlessSetting(this); } return _pageBorderless; } } ///object that specifies the page borderless setting. /// /// Gets a public PageOutputQualitySetting PageOutputQuality { get { if (_pageOutputQuality == null) { _pageOutputQuality = new PageOutputQualitySetting(this); } return _pageOutputQuality; } } ///object that specifies the page output quality setting. /// /// Gets a public JobInputBinSetting JobInputBin { get { if (_jobInputBin == null) { _jobInputBin = new JobInputBinSetting(this); } return _jobInputBin; } } ///object that specifies the job input bin setting. /// /// Gets a public DocumentInputBinSetting DocumentInputBin { get { if (_documentInputBin == null) { _documentInputBin = new DocumentInputBinSetting(this); } return _documentInputBin; } } ///object that specifies the document input bin setting. /// /// Gets a public PageInputBinSetting PageInputBin { get { if (_pageInputBin == null) { _pageInputBin = new PageInputBinSetting(this); } return _pageInputBin; } } ///object that specifies the page input bin setting. /// /// Gets a public JobCopyCountSetting JobCopyCount { get { if (_jobCopyCount == null) { _jobCopyCount = new JobCopyCountSetting(this); } return _jobCopyCount; } } ///object that specifies the job copy count setting. /// /// Gets a public MemoryStream XmlStream { get { MemoryStream ms = new MemoryStream(); _xmlDoc.Save(ms); ms.Position = 0; return ms; } } #endregion Public Properties #region Internal Methods ///object that contains the XML form of this PrintTicket object. /// /// Returns a new FormatException instance for not-well-formed PrintTicket XML. /// /// detailed message about the violation of well-formness ///the new FormatException instance internal static FormatException NewPTFormatException(string detailMsg) { return NewPTFormatException(detailMsg, null); } ////// Returns a new FormatException instance for not-well-formed PrintTicket XML. /// /// detailed message about the violation of well-formness /// the exception that causes the violation of well-formness ///the new FormatException instance internal static FormatException NewPTFormatException(string detailMsg, Exception innerException) { return new FormatException(String.Format(CultureInfo.CurrentCulture, "{0} {1} {2}", PrintSchemaTags.Framework.PrintTicketRoot, PTUtility.GetTextFromResource("FormatException.XMLNotWellFormed"), detailMsg), innerException); } internal PrintTicketFeature GetBasePTFeatureObject(CapabilityName feature) { switch (feature) { case CapabilityName.DocumentCollate: return this.DocumentCollate; case CapabilityName.PageDeviceFontSubstitution: return this.PageDeviceFontSubstitution; case CapabilityName.JobDuplex: return this.JobDuplex; case CapabilityName.JobInputBin: return this.JobInputBin; case CapabilityName.DocumentInputBin: return this.DocumentInputBin; case CapabilityName.PageInputBin: return this.PageInputBin; case CapabilityName.PageOutputColor: return this.PageOutputColor; case CapabilityName.PageOutputQuality: return this.PageOutputQuality; case CapabilityName.PageBorderless: return this.PageBorderless; case CapabilityName.PageMediaType: return this.PageMediaType; case CapabilityName.JobPageOrder: return this.JobPageOrder; case CapabilityName.PageOrientation: return this.PageOrientation; case CapabilityName.JobNUp: return this.JobNUp.PresentationDirection; case CapabilityName.PagePhotoPrintingIntent: return this.PagePhotoPrintingIntent; case CapabilityName.JobStaple: return this.JobStaple; case CapabilityName.PageTrueTypeFontMode: return this.PageTrueTypeFontMode; default: return null; } } #endregion internal Methods #region Internal Properties internal XmlDocument XmlDoc { get { return _xmlDoc; } } #endregion Internal Properties #region Private Methods #if _DEBUG private void InitTrace() { // direct Trace output to console Trace.Listeners.Clear(); Trace.Listeners.Add(new TextWriterTraceListener(Console.Out)); } #endif private void SetupNamespaceManager() { _nsMgr = new XmlNamespaceManager(new NameTable()); _nsMgr.AddNamespace(PrintSchemaPrefixes.Framework, PrintSchemaNamespaces.Framework); _nsMgr.AddNamespace(PrintSchemaPrefixes.StandardKeywordSet, PrintSchemaNamespaces.StandardKeywordSet); _nsMgr.AddNamespace(PrintSchemaPrefixes.xsi, PrintSchemaNamespaces.xsi); _nsMgr.AddNamespace(PrintSchemaPrefixes.xsd, PrintSchemaNamespaces.xsd); } #endregion Private Methods #region Private Fields private XmlDocument _xmlDoc; private XmlNamespaceManager _nsMgr; // Features private DocumentCollateSetting _docCollate; private JobDuplexSetting _jobDuplex; private JobNUpSetting _jobNUp; private JobStapleSetting _jobStaple; private PageDeviceFontSubstitutionSetting _pageDeviceFontSubst; private PageMediaSizeSetting _pageMediaSize; private PageMediaTypeSetting _pageMediaType; private PageOrientationSetting _pageOrientation; private PageOutputColorSetting _pageOutputColor; private PageResolutionSetting _pageResolution; private PageScalingSetting _pageScaling; private PageTrueTypeFontModeSetting _pageTrueTypeFontMode; private JobPageOrderSetting _jobPageOrder; private PagePhotoPrintingIntentSetting _pagePhotoIntent; private PageBorderlessSetting _pageBorderless; private PageOutputQualitySetting _pageOutputQuality; private JobInputBinSetting _jobInputBin; private DocumentInputBinSetting _documentInputBin; private PageInputBinSetting _pageInputBin; // Parameters private JobCopyCountSetting _jobCopyCount; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
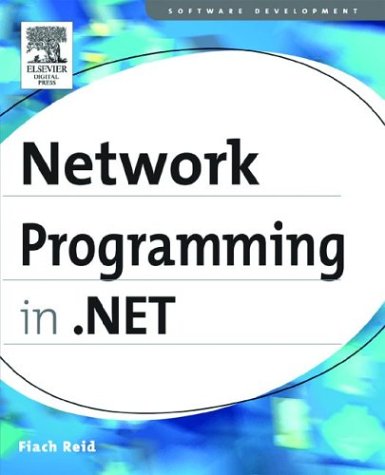
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ImageDrawing.cs
- BuilderPropertyEntry.cs
- GeneralTransformCollection.cs
- XmlFormatExtensionPrefixAttribute.cs
- ReadOnlyCollectionBase.cs
- TreeNodeCollection.cs
- SecurityContext.cs
- SystemColorTracker.cs
- SortExpressionBuilder.cs
- DocumentGrid.cs
- RelativeSource.cs
- HttpCapabilitiesEvaluator.cs
- SubclassTypeValidator.cs
- UpDownEvent.cs
- BitmapSourceSafeMILHandle.cs
- ping.cs
- VersionedStreamOwner.cs
- BooleanStorage.cs
- TextDecorationLocationValidation.cs
- JsonDataContract.cs
- MSAANativeProvider.cs
- DataGridItemEventArgs.cs
- UpdatePanel.cs
- ContextMenuAutomationPeer.cs
- OutputScope.cs
- X509Utils.cs
- MarginCollapsingState.cs
- LineProperties.cs
- ItemCollection.cs
- GuidelineCollection.cs
- XsdDuration.cs
- XPathNavigator.cs
- GroupByQueryOperator.cs
- APCustomTypeDescriptor.cs
- RenderCapability.cs
- StylusPointPropertyId.cs
- BaseAddressElement.cs
- HttpHandlersInstallComponent.cs
- ChangePassword.cs
- SqlUtils.cs
- ElementUtil.cs
- ColorTransformHelper.cs
- DesignerCategoryAttribute.cs
- ActivityCodeDomReferenceService.cs
- OleDbConnection.cs
- OptimizedTemplateContent.cs
- RequestQueryParser.cs
- HTMLTextWriter.cs
- DataGridViewCellStyle.cs
- BitmapEffectGroup.cs
- GenericArgumentsUpdater.cs
- DiscoveryOperationContext.cs
- DataGridViewRowPrePaintEventArgs.cs
- LogSwitch.cs
- BasicHttpBindingElement.cs
- SafeEventLogWriteHandle.cs
- ClassDataContract.cs
- IisTraceListener.cs
- IsolatedStorageFileStream.cs
- BrowserCapabilitiesFactoryBase.cs
- HttpRuntimeSection.cs
- BookmarkNameHelper.cs
- HighlightVisual.cs
- DocumentPage.cs
- CommentGlyph.cs
- RandomNumberGenerator.cs
- Compiler.cs
- UserControlAutomationPeer.cs
- GraphicsContext.cs
- ResourcesChangeInfo.cs
- Pens.cs
- ToolStripSettings.cs
- Instrumentation.cs
- ICspAsymmetricAlgorithm.cs
- BinaryCommonClasses.cs
- SqlExpressionNullability.cs
- UnicodeEncoding.cs
- NavigationProperty.cs
- _FtpDataStream.cs
- OperatingSystem.cs
- DataGridViewCellStyleContentChangedEventArgs.cs
- ObjectPropertyMapping.cs
- QuotedPrintableStream.cs
- MultipleCopiesCollection.cs
- AsyncSerializedWorker.cs
- ScrollBar.cs
- Italic.cs
- TagPrefixCollection.cs
- TemplatePagerField.cs
- SqlRowUpdatingEvent.cs
- ZipIOModeEnforcingStream.cs
- SupportingTokenProviderSpecification.cs
- FileFormatException.cs
- QueryInterceptorAttribute.cs
- MobileControl.cs
- XmlSchemaObjectTable.cs
- Schema.cs
- ModuleElement.cs
- CompositeDispatchFormatter.cs
- Pick.cs