Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / System / Windows / Markup / Localizer / BamlLocalizer.cs / 1 / BamlLocalizer.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2001 // // File: BamlLocalizer.cs // // Contents: BamlLocalizer class, part of Baml Localization API // // Created: 3/17/2004 [....] // History: 8/3/2004 Move to System.Windows namespace // 11/29/2004 [....] Move to System.Windows.Markup.Localization namespace // 03/24/2005 [....] Move to System.Windows.Markup.Localizer namespace // // //----------------------------------------------------------------------- using System; using System.IO; using System.Globalization; using System.Runtime.InteropServices; using System.Collections; using System.Windows.Markup; using System.Diagnostics; using System.Text; using System.Reflection; using System.Windows; using MS.Internal.Globalization; namespace System.Windows.Markup.Localizer { ////// BamlLocalizer class localizes a baml stram. /// public class BamlLocalizer { //----------------------------------- // constructor //----------------------------------- ////// Constructor /// /// source baml stream to be localized public BamlLocalizer(Stream source) : this (source, null) { } ////// Constructor /// /// source baml stream to be localized /// Localizability resolver implemented by client public BamlLocalizer( Stream source, BamlLocalizabilityResolver resolver ) : this (source, resolver, null) { } ////// constructor /// /// source baml straem to be localized /// Localizability resolver implemented by client /// TextReader to read localization comments XML public BamlLocalizer( Stream source, BamlLocalizabilityResolver resolver, TextReader comments ) { if (source == null) { throw new ArgumentNullException("source"); } _tree = BamlResourceDeserializer.LoadBaml(source); // create a Baml Localization Enumerator _bamlTreeMap = new BamlTreeMap(this, _tree, resolver, comments); } ////// Extract localizable resources from the source baml. /// ///Localizable resources returned in the form of BamlLoaclizationDictionary public BamlLocalizationDictionary ExtractResources() { return _bamlTreeMap.LocalizationDictionary.Copy(); } ////// Udpate the source baml into a target stream with all the resource udpates applied. /// /// target stream /// resource updates to be applied when generating the localized baml public void UpdateBaml( Stream target, BamlLocalizationDictionary updates ) { if (target == null) { throw new ArgumentNullException("target"); } if (updates == null) { throw new ArgumentNullException("updates"); } // we duplicate the internal baml tree here because // we will need to modify the tree to do generation // UpdateBaml can be called multiple times BamlTree _duplicateTree = _tree.Copy(); _bamlTreeMap.EnsureMap(); // Udpate the tree BamlTreeUpdater.UpdateTree( _duplicateTree, _bamlTreeMap, updates ); // Serialize the tree into Baml BamlResourceSerializer.Serialize( this, _duplicateTree, target ); } ////// Raised by BamlLocalizer when it encounters any abnormal conditions. /// ////// BamlLocalizer can normally ignore the errors and continue the localization. /// As a result, the output baml will only be partially localized. The client /// can choose to stop the BamlLocalizer on error by throw exceptions in their /// event handlers. /// public event BamlLocalizerErrorNotifyEventHandler ErrorNotify; ////// Raise ErrorNotify event /// protected virtual void OnErrorNotify(BamlLocalizerErrorNotifyEventArgs e) { BamlLocalizerErrorNotifyEventHandler handler = ErrorNotify; if (handler != null) { handler(this, e); } } //---------------------------------- // Internal method //---------------------------------- internal void RaiseErrorNotifyEvent(BamlLocalizerErrorNotifyEventArgs e) { OnErrorNotify(e); } //---------------------------------- // Private member //---------------------------------- private BamlTreeMap _bamlTreeMap; private BamlTree _tree; } ////// The delegate for the BamlLocalizer.ErrorNotify event /// public delegate void BamlLocalizerErrorNotifyEventHandler(object sender, BamlLocalizerErrorNotifyEventArgs e); } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
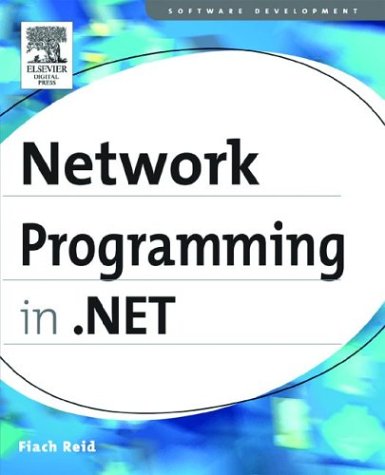
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebRequestModuleElementCollection.cs
- NotSupportedException.cs
- PartialCachingControl.cs
- HttpApplication.cs
- Separator.cs
- UTF7Encoding.cs
- ColorAnimationBase.cs
- ServiceDiscoveryBehavior.cs
- RegexMatchCollection.cs
- FtpWebRequest.cs
- ColorPalette.cs
- CheckedListBox.cs
- _OSSOCK.cs
- XmlExtensionFunction.cs
- OptimalBreakSession.cs
- Rotation3DKeyFrameCollection.cs
- EnumCodeDomSerializer.cs
- ContextMarshalException.cs
- Solver.cs
- SendMailErrorEventArgs.cs
- DocobjHost.cs
- TransportOutputChannel.cs
- PatternMatchRules.cs
- SingleStorage.cs
- NextPreviousPagerField.cs
- FixedStringLookup.cs
- MsmqNonTransactedPoisonHandler.cs
- Clock.cs
- FixedFindEngine.cs
- StatusBar.cs
- _AutoWebProxyScriptHelper.cs
- DeclarationUpdate.cs
- CodeMemberMethod.cs
- MimeWriter.cs
- ToolStripGripRenderEventArgs.cs
- TextAnchor.cs
- ArrayElementGridEntry.cs
- BindingBase.cs
- SqlFormatter.cs
- EventItfInfo.cs
- NativeMethods.cs
- PrinterSettings.cs
- TabControl.cs
- User.cs
- bidPrivateBase.cs
- GlyphShapingProperties.cs
- SqlExpressionNullability.cs
- PopupRoot.cs
- LiteralControl.cs
- UnmanagedHandle.cs
- SafeThreadHandle.cs
- NavigatingCancelEventArgs.cs
- PenLineCapValidation.cs
- WebScriptMetadataFormatter.cs
- Matrix.cs
- EdmType.cs
- ResourceWriter.cs
- ActivityTypeDesigner.xaml.cs
- ObjectDataSourceDesigner.cs
- MainMenu.cs
- DictionarySurrogate.cs
- NamedPermissionSet.cs
- FileDialog_Vista_Interop.cs
- ImageClickEventArgs.cs
- MouseActionValueSerializer.cs
- TemplateBindingExtensionConverter.cs
- Int32AnimationBase.cs
- SafeRegistryHandle.cs
- XmlTextWriter.cs
- SspiWrapper.cs
- HeaderedItemsControl.cs
- XslTransform.cs
- EpmCustomContentDeSerializer.cs
- Rfc2898DeriveBytes.cs
- WorkflowMarkupSerializationManager.cs
- Translator.cs
- ScopeElementCollection.cs
- Group.cs
- CompositeClientFormatter.cs
- _IPv4Address.cs
- log.cs
- ByteRangeDownloader.cs
- FragmentNavigationEventArgs.cs
- EncryptedData.cs
- SecurityAlgorithmSuite.cs
- ProtocolImporter.cs
- RunWorkerCompletedEventArgs.cs
- PropertyItemInternal.cs
- SignedPkcs7.cs
- ThreadInterruptedException.cs
- SdlChannelSink.cs
- UnitySerializationHolder.cs
- ThreadSafeList.cs
- SchemaNamespaceManager.cs
- SafeMarshalContext.cs
- GenerateTemporaryTargetAssembly.cs
- HMACMD5.cs
- SuppressIldasmAttribute.cs
- PerspectiveCamera.cs
- OdbcConnectionHandle.cs