Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / MS / Internal / Ink / InkSerializedFormat / Codec.cs / 1 / Codec.cs
using MS.Utility; using System; using System.Runtime.InteropServices; using System.Security; using System.Globalization; using System.Windows; using System.Windows.Input; using System.Windows.Ink; using MS.Internal.Ink.InkSerializedFormat; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace MS.Internal.Ink.InkSerializedFormat { ////// A Math helper class. /// internal static class MathHelper { ////// Returns the absolute value of a 32-bit signed integer. /// Unlike Math.Abs, this method doesn't throw OverflowException /// when the signed integer equals int.MinValue (-2,147,483,648/0x80000000). /// It will return the same value (-2,147,483,648). /// In this case, value can be casted to unsigned value which will be positive (2,147,483,648) /// /// ///internal static int AbsNoThrow(int data) { // This behavior is desired for ISF decoder. Please refer to the below macro in old native code (codec.h). // template{typename DataType} // inline DataType Abs(DataType data) { return (data < 0) ? -data : data; }; return (data < 0) ? -data : data; } /// /// Returns the absolute value of a 64-bit signed integer. /// Unlike Math.Abs, this method doesn't throw OverflowException /// when the signed integer equals int.MinValue (-9,223,372,036,854,775,808/0x8000000000000000). /// It will return the same value -9,223,372,036,854,775,808 instead. /// In this case, value can be casted to unsigned value which will be positive (9,223,372,036,854,775,808) /// /// ///internal static long AbsNoThrow(long data) { // This behavior is desired for ISF decoder. Please refer to the below macro in old native code (codec.h). // template{typename DataType} // inline DataType Abs(DataType data) { return (data < 0) ? -data : data; }; return (data < 0) ? -data : data; } } /// /// Abstact base class for DeltaDelta and some others /// internal abstract class DataXform { internal abstract void Transform(int data, ref int xfData, ref int extra); internal abstract void ResetState(); internal abstract int InverseTransform(int xfData, int extra); } ////// Oddly named because we have unmanged code we keep in sync with this that /// has this name. /// internal class DeltaDelta : DataXform { private long _d_i_1 = 0; private long _d_i_2 = 0; internal DeltaDelta() { } ////// Your guess is as good as mine /// /// /// /// internal override void Transform(int data, ref int xfData, ref int extra) { // Find out the delta delta of the number // Its absolute value could potentially be more than LONG_MAX long llxfData = (data + _d_i_2 - (_d_i_1 << 1)); // Save the state info for next number _d_i_2 = _d_i_1; _d_i_1 = data; // Most of the cases, the delta delta will be less than LONG_MAX if ( Int32.MaxValue >= MathHelper.AbsNoThrow(llxfData) ) { // In those cases, we set 0 to nExtra and // assign the delta delta to xfData extra = 0; xfData = (int)llxfData; } else { long absLxfData = MathHelper.AbsNoThrow(llxfData); // Additional bits in most significant 32 bits extra = (int)(absLxfData >> (sizeof(int) << 3)); // Left sift one bit and append sign bit the the LSB extra = (extra << 1) | ((llxfData < 0) ? 1 : 0); // Save least significant 32 bits in xfData xfData = (int)((unchecked((uint)~0 & absLxfData))); } } ////// /// internal override void ResetState() { _d_i_1 = 0; _d_i_2 = 0; } ////// Your guess is as good as mine /// /// /// ///internal override int InverseTransform(int xfData, int extra) { long llxfData; // Find out whether the original delta delta exceeded the limit if (0 != extra) { // Yes, we had |delta delta| more than LONG_MAX // Find out the original delta delta was negative bool negative = ((extra & 0x01) != 0); // Construct the |DelDel| from xfData and nExtra llxfData = (((long)extra >> 1) << (sizeof(int) << 3)) | (unchecked((uint)~0) & xfData); // Do the sign adjustment llxfData = (negative) ? -llxfData : llxfData; } else { llxfData = xfData; } // Reconstruct the number from delta delta long orgData = (llxfData - _d_i_2 + (_d_i_1 << 1)); _d_i_2 = _d_i_1; _d_i_1 = orgData; // Typecast to LONG and return it return (int)orgData; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
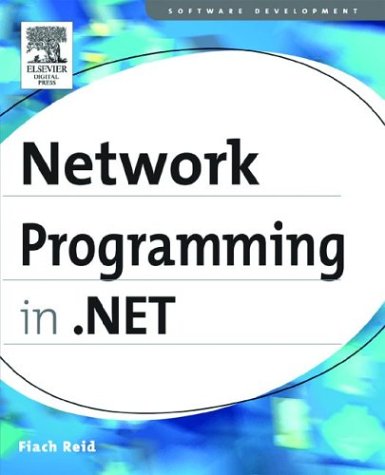
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SHA384Managed.cs
- ObjectCache.cs
- Rijndael.cs
- TextElementEnumerator.cs
- Command.cs
- SocketInformation.cs
- EventPrivateKey.cs
- RecoverInstanceLocksCommand.cs
- ScaleTransform3D.cs
- ContainsRowNumberChecker.cs
- ModifiableIteratorCollection.cs
- XsltLoader.cs
- WmlControlAdapter.cs
- SqlErrorCollection.cs
- ExpressionQuoter.cs
- ListParaClient.cs
- IBuiltInEvidence.cs
- ProjectionCamera.cs
- NullableIntMinMaxAggregationOperator.cs
- BrowserPolicyValidator.cs
- EventLogger.cs
- AttributeSetAction.cs
- UnicodeEncoding.cs
- XmlDocumentType.cs
- SubpageParagraph.cs
- BindableAttribute.cs
- FilteredReadOnlyMetadataCollection.cs
- ConcatQueryOperator.cs
- OperationAbortedException.cs
- FunctionCommandText.cs
- PtsHost.cs
- ExchangeUtilities.cs
- WebBrowserNavigatingEventHandler.cs
- DuplicateWaitObjectException.cs
- Run.cs
- DoubleCollectionValueSerializer.cs
- IsolatedStorageFile.cs
- ResetableIterator.cs
- StorageSetMapping.cs
- HelpEvent.cs
- FormClosingEvent.cs
- VectorAnimation.cs
- DesignerDataSchemaClass.cs
- ItemCheckedEvent.cs
- ViewService.cs
- TripleDESCryptoServiceProvider.cs
- DataErrorValidationRule.cs
- FullTextState.cs
- XmlSchemaSequence.cs
- CTreeGenerator.cs
- MarshalByValueComponent.cs
- ComplusTypeValidator.cs
- PropertyValidationContext.cs
- SqlBinder.cs
- Site.cs
- RowBinding.cs
- PropertyDescriptorComparer.cs
- ClosableStream.cs
- WebEventTraceProvider.cs
- EntityDataSourceContextCreatingEventArgs.cs
- odbcmetadatafactory.cs
- LocalBuilder.cs
- PowerStatus.cs
- DesignerActionItemCollection.cs
- ImageKeyConverter.cs
- ProfileSection.cs
- ComplexObject.cs
- SelectManyQueryOperator.cs
- DeleteHelper.cs
- ColorConverter.cs
- TextBoxBase.cs
- SqlInternalConnectionTds.cs
- ObjectDataSourceWizardForm.cs
- DataTableReaderListener.cs
- EntitySetBaseCollection.cs
- XamlFrame.cs
- BufferedGraphicsContext.cs
- InputLanguageCollection.cs
- EnvironmentPermission.cs
- XmlNamedNodeMap.cs
- ProtocolElementCollection.cs
- XmlIterators.cs
- XmlHierarchicalEnumerable.cs
- PackageRelationshipSelector.cs
- MenuItemStyleCollection.cs
- BitStream.cs
- Properties.cs
- invalidudtexception.cs
- CellRelation.cs
- Process.cs
- ParamArrayAttribute.cs
- PerformanceCounterManager.cs
- Merger.cs
- AutoGeneratedField.cs
- ExecutedRoutedEventArgs.cs
- QilXmlWriter.cs
- ConfigurationStrings.cs
- CatalogZone.cs
- JsonEncodingStreamWrapper.cs
- AuthenticationManager.cs