Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Controls / CalendarBlackoutDatesCollection.cs / 1305600 / CalendarBlackoutDatesCollection.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Collections.ObjectModel; using System.ComponentModel; using System.Diagnostics; using System.Threading; namespace System.Windows.Controls { ////// Represents a collection of DateTimeRanges. /// public sealed class CalendarBlackoutDatesCollection : ObservableCollection{ #region Data private Thread _dispatcherThread; private Calendar _owner; #endregion Data /// /// Initializes a new instance of the CalendarBlackoutDatesCollection class. /// /// public CalendarBlackoutDatesCollection(Calendar owner) { _owner = owner; this._dispatcherThread = Thread.CurrentThread; } #region Public Methods ////// Dates that are in the past are added to the BlackoutDates. /// public void AddDatesInPast() { this.Add(new CalendarDateRange(DateTime.MinValue, DateTime.Today.AddDays(-1))); } ////// Checks if a DateTime is in the Collection /// /// ///public bool Contains(DateTime date) { return null != GetContainingDateRange(date); } /// /// Checks if a Range is in the collection /// /// /// ///public bool Contains(DateTime start, DateTime end) { DateTime rangeStart, rangeEnd; int n = Count; if (DateTime.Compare(end, start) > -1) { rangeStart = DateTimeHelper.DiscardTime(start).Value; rangeEnd = DateTimeHelper.DiscardTime(end).Value; } else { rangeStart = DateTimeHelper.DiscardTime(end).Value; rangeEnd = DateTimeHelper.DiscardTime(start).Value; } for (int i = 0; i < n; i++) { if (DateTime.Compare(this[i].Start, rangeStart) == 0 && DateTime.Compare(this[i].End, rangeEnd) == 0) { return true; } } return false; } /// /// Returns true if any day in the given DateTime range is contained in the BlackOutDays. /// /// CalendarDateRange that is searched in BlackOutDays ///true if at least one day in the range is included in the BlackOutDays public bool ContainsAny(CalendarDateRange range) { foreach (CalendarDateRange item in this) { if (item.ContainsAny(range)) { return true; } } return false; } ////// This finds the next date that is not blacked out in a certian direction. /// /// /// ///internal DateTime? GetNonBlackoutDate(DateTime? requestedDate, int dayInterval) { Debug.Assert(dayInterval != 0); DateTime? currentDate = requestedDate; CalendarDateRange range = null; if (requestedDate == null) { return null; } if ((range = GetContainingDateRange((DateTime)currentDate)) == null) { return requestedDate; } do { if (dayInterval > 0) { // Moving Forwards. // The DateRanges require start <= end currentDate = DateTimeHelper.AddDays(range.End, dayInterval ); } else { //Moving backwards. currentDate = DateTimeHelper.AddDays(range.Start, dayInterval ); } } while (currentDate != null && ((range = GetContainingDateRange((DateTime)currentDate)) != null)); return currentDate; } #endregion Public Methods #region Protected Methods /// /// All the items in the collection are removed. /// protected override void ClearItems() { if (!IsValidThread()) { throw new NotSupportedException(SR.Get(SRID.CalendarCollection_MultiThreadedCollectionChangeNotSupported)); } foreach (CalendarDateRange item in Items) { UnRegisterItem(item); } base.ClearItems(); this._owner.UpdateCellItems(); } ////// The item is inserted in the specified place in the collection. /// /// /// protected override void InsertItem(int index, CalendarDateRange item) { if (!IsValidThread()) { throw new NotSupportedException(SR.Get(SRID.CalendarCollection_MultiThreadedCollectionChangeNotSupported)); } if (IsValid(item)) { RegisterItem(item); base.InsertItem(index, item); _owner.UpdateCellItems(); } else { throw new ArgumentOutOfRangeException(SR.Get(SRID.Calendar_UnSelectableDates)); } } ////// The item in the specified index is removed from the collection. /// /// protected override void RemoveItem(int index) { if (!IsValidThread()) { throw new NotSupportedException(SR.Get(SRID.CalendarCollection_MultiThreadedCollectionChangeNotSupported)); } if (index >= 0 && index < this.Count) { UnRegisterItem(Items[index]); } base.RemoveItem(index); _owner.UpdateCellItems(); } ////// The object in the specified index is replaced with the provided item. /// /// /// protected override void SetItem(int index, CalendarDateRange item) { if (!IsValidThread()) { throw new NotSupportedException(SR.Get(SRID.CalendarCollection_MultiThreadedCollectionChangeNotSupported)); } if (IsValid(item)) { CalendarDateRange oldItem = null; if (index >= 0 && index < this.Count) { oldItem = Items[index]; } base.SetItem(index, item); UnRegisterItem(oldItem); RegisterItem(Items[index]); _owner.UpdateCellItems(); } else { throw new ArgumentOutOfRangeException(SR.Get(SRID.Calendar_UnSelectableDates)); } } #endregion Protected Methods #region Private Methods ////// Registers for change notification on date ranges /// /// private void RegisterItem(CalendarDateRange item) { if (item != null) { item.Changing += new EventHandler(Item_Changing); item.PropertyChanged += new PropertyChangedEventHandler(Item_PropertyChanged); } } /// /// Un registers for change notification on date ranges /// private void UnRegisterItem(CalendarDateRange item) { if (item != null) { item.Changing -= new EventHandler(Item_Changing); item.PropertyChanged -= new PropertyChangedEventHandler(Item_PropertyChanged); } } /// /// Reject date range changes that would make the blackout dates collection invalid /// /// /// private void Item_Changing(object sender, CalendarDateRangeChangingEventArgs e) { CalendarDateRange item = sender as CalendarDateRange; if (item != null) { if (!IsValid(e.Start, e.End)) { throw new ArgumentOutOfRangeException(SR.Get(SRID.Calendar_UnSelectableDates)); } } } ////// Update the calendar view to reflect the new blackout dates /// /// /// private void Item_PropertyChanged(object sender, PropertyChangedEventArgs e) { if (sender is CalendarDateRange) { _owner.UpdateCellItems(); } } ////// Tests to see if a date range is not already selected /// /// date range to test ///True if no selected day falls in the given date range private bool IsValid(CalendarDateRange item) { return IsValid(item.Start, item.End); } ////// Tests to see if a date range is not already selected /// /// First day of date range to test /// Last day of date range to test ///True if no selected day falls between start and end private bool IsValid(DateTime start, DateTime end) { foreach (object child in _owner.SelectedDates) { DateTime? day = child as DateTime?; Debug.Assert(day != null); if (DateTimeHelper.InRange(day.Value, start, end)) { return false; } } return true; } private bool IsValidThread() { return Thread.CurrentThread == this._dispatcherThread; } ////// Gets the DateRange that contains the date. /// /// ///private CalendarDateRange GetContainingDateRange(DateTime date) { if (date == null) return null; for (int i = 0; i < Count; i++) { if (DateTimeHelper.InRange(date, this[i])) { return this[i]; } } return null; } #endregion Private Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
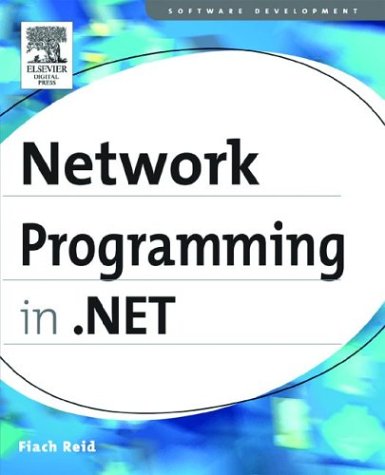
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlArrayAttribute.cs
- XsltException.cs
- HMACSHA1.cs
- XmlByteStreamReader.cs
- ZipFileInfo.cs
- regiisutil.cs
- PartitionResolver.cs
- MarkupExtensionParser.cs
- WebPartHeaderCloseVerb.cs
- SpinWait.cs
- DataStorage.cs
- LineBreak.cs
- dataobject.cs
- BaseDataList.cs
- GlobalItem.cs
- OneOfTypeConst.cs
- DataGridViewRowEventArgs.cs
- IssuerInformation.cs
- TextBox.cs
- UnionCodeGroup.cs
- CompiledELinqQueryState.cs
- PropertyRecord.cs
- IndexOutOfRangeException.cs
- MiniAssembly.cs
- CharacterMetricsDictionary.cs
- KeyValuePair.cs
- ObjectQueryState.cs
- MultiBinding.cs
- GeneratedCodeAttribute.cs
- MessageQueuePermission.cs
- URIFormatException.cs
- DataBindingCollectionConverter.cs
- TextServicesCompartment.cs
- HeaderCollection.cs
- ToolBar.cs
- SoapClientProtocol.cs
- EventLogEntry.cs
- UrlMappingsModule.cs
- _HeaderInfo.cs
- QueryTreeBuilder.cs
- DecoderFallback.cs
- SqlDataReader.cs
- ExtentKey.cs
- IChannel.cs
- DocumentViewerAutomationPeer.cs
- ProcessModule.cs
- Msec.cs
- LineGeometry.cs
- RichTextBox.cs
- Point4DConverter.cs
- NotificationContext.cs
- Typeface.cs
- WindowsStatusBar.cs
- AsyncContentLoadedEventArgs.cs
- ArcSegment.cs
- IHttpResponseInternal.cs
- HtmlTableCellCollection.cs
- DataGridLinkButton.cs
- IdentitySection.cs
- SqlXmlStorage.cs
- SecurityElement.cs
- PackagePart.cs
- JsonGlobals.cs
- Component.cs
- DetailsViewRowCollection.cs
- SerialReceived.cs
- XPathMessageFilterElement.cs
- IndexedString.cs
- NetNamedPipeBindingElement.cs
- CategoryValueConverter.cs
- SqlNotificationEventArgs.cs
- StringSorter.cs
- LogLogRecordHeader.cs
- DataGridViewHitTestInfo.cs
- altserialization.cs
- CompositeControl.cs
- WebPartTracker.cs
- WindowAutomationPeer.cs
- GACIdentityPermission.cs
- SrgsDocument.cs
- ConstrainedGroup.cs
- Command.cs
- BorderGapMaskConverter.cs
- CoreSwitches.cs
- MainMenu.cs
- DataTableExtensions.cs
- Icon.cs
- EditorAttributeInfo.cs
- ClientSettingsProvider.cs
- UInt16Storage.cs
- PackagingUtilities.cs
- ListControlConvertEventArgs.cs
- OleDbRowUpdatingEvent.cs
- TypeElementCollection.cs
- TrustLevelCollection.cs
- ActivityExecutor.cs
- SubpageParaClient.cs
- GcSettings.cs
- TypeToken.cs
- DirtyTextRange.cs